Intro
Master file name manipulation with VBA. Discover 5 efficient ways to change file names using Visual Basic for Applications, including renaming files in a folder, using loops, and handling errors. Learn VBA file management techniques, automate file renaming tasks, and boost productivity with these expert-approved methods.
Renaming files in bulk can be a tedious task, especially when dealing with a large number of files. Fortunately, Visual Basic for Applications (VBA) provides a powerful tool to automate this process. In this article, we will explore five ways to change file names with VBA, making it easier to manage your files.
The Importance of Renaming Files
Renaming files is a common task in various industries, including data analysis, software development, and digital marketing. It helps in organizing files, making them easier to identify and access. Moreover, renaming files can improve data integrity, reduce errors, and enhance collaboration among team members.
Method 1: Using the FileSystemObject
The FileSystemObject (FSO) is a built-in VBA object that allows you to interact with the file system. You can use it to rename files using the following code:
Sub RenameFileUsingFSO()
Dim fso As FileSystemObject
Set fso = New FileSystemObject
Dim filePath As String
filePath = "C:\Example\OldFileName.txt"
Dim newFileName As String
newFileName = "NewFileName.txt"
fso.MoveFile filePath, "C:\Example\" & newFileName
End Sub
Method 2: Using the Dir Function
The Dir function is a VBA function that returns the name of a file or directory. You can use it to rename files using the following code:
Sub RenameFileUsingDir()
Dim filePath As String
filePath = "C:\Example\OldFileName.txt"
Dim newFileName As String
newFileName = "NewFileName.txt"
Name filePath As "C:\Example\" & newFileName
End Sub
Method 3: Using the Kill Statement
The Kill statement is a VBA statement that deletes a file. You can use it to rename files by deleting the old file and creating a new one with the same content. Here's an example:
Sub RenameFileUsingKill()
Dim filePath As String
filePath = "C:\Example\OldFileName.txt"
Dim newFileName As String
newFileName = "NewFileName.txt"
Kill filePath
Dim fileContent As String
fileContent = "This is the content of the file."
Open "C:\Example\" & newFileName For Output As #1
Write #1, fileContent
Close #1
End Sub
Method 4: Using the Rename Method
The Rename method is a VBA method that renames a file or directory. You can use it to rename files using the following code:
Sub RenameFileUsingRename()
Dim filePath As String
filePath = "C:\Example\OldFileName.txt"
Dim newFileName As String
newFileName = "NewFileName.txt"
Rename filePath, "C:\Example\" & newFileName
End Sub
Method 5: Using the Scripting Runtime Library
The Scripting Runtime Library is a VBA library that provides additional functionality for working with files and directories. You can use it to rename files using the following code:
Sub RenameFileUsingScriptingRuntime()
Dim fso As New Scripting.FileSystemObject
Dim filePath As String
filePath = "C:\Example\OldFileName.txt"
Dim newFileName As String
newFileName = "NewFileName.txt"
fso.MoveFile filePath, "C:\Example\" & newFileName
End Sub
Gallery of VBA File Rename Methods
VBA File Rename Methods
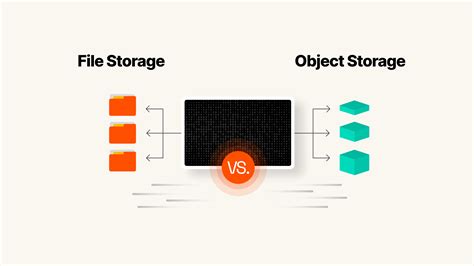
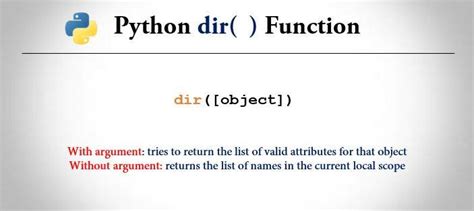
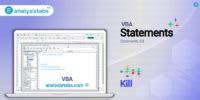
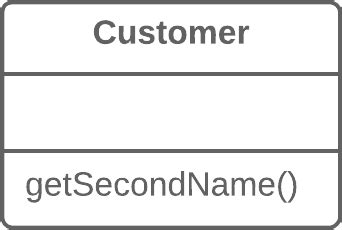
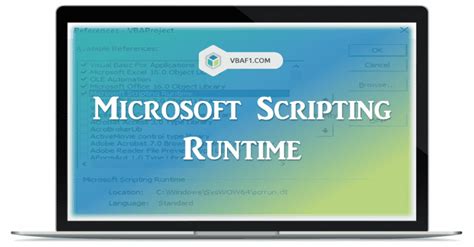
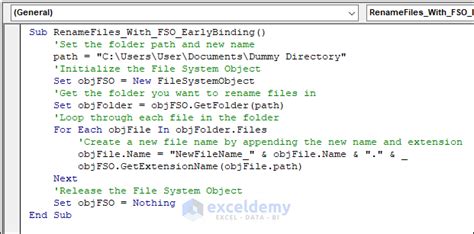
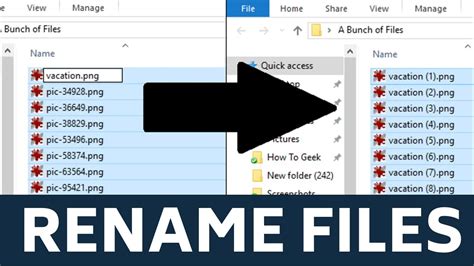
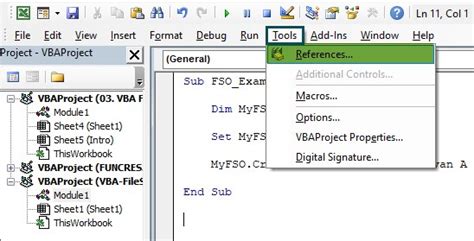
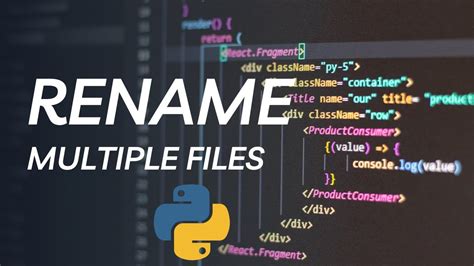
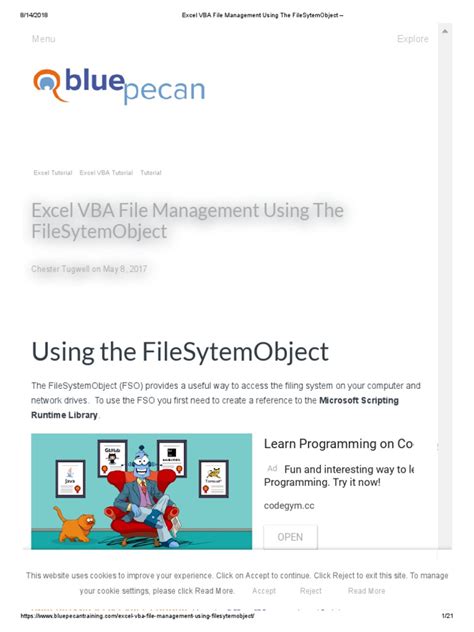
We hope this article has provided you with a comprehensive guide on how to change file names with VBA. Whether you're a beginner or an advanced user, these methods will help you automate file renaming tasks and improve your productivity. Don't hesitate to ask if you have any questions or need further assistance.
Feel free to share your thoughts and experiences with VBA file renaming methods in the comments section below.