Intro
Discover the 4 effective ways to check if a file exists in VBA. Learn how to use the Dir function, FileExist method, and other techniques to verify file existence in Visual Basic for Applications. Improve your VBA skills and error-handling with these practical examples and code snippets. Master file management in VBA.
When working with files in VBA, it's essential to verify if a file exists before attempting to open, read, or write to it. This helps prevent errors and ensures your code runs smoothly. In this article, we'll explore four ways to check if a file exists in VBA, each with its own strengths and use cases.
The Importance of File Existence Checks
Before diving into the methods, let's discuss why checking if a file exists is crucial in VBA. Here are a few reasons:
- Prevent errors: Trying to open or manipulate a non-existent file can lead to runtime errors, which can be frustrating to debug.
- Improve code reliability: By verifying the existence of a file, you can ensure your code behaves as expected and doesn't fail unexpectedly.
- Enhance user experience: Checking if a file exists before attempting to use it can help you provide a better user experience by avoiding errors and providing meaningful feedback.
Method 1: Using the Dir
Function
The Dir
function is a simple way to check if a file exists in VBA. This function returns a string representing the name of the file if it exists, or an empty string if it doesn't.
Sub CheckFileExists_Dir()
Dim filePath As String
filePath = "C:\path\to\your\file.txt"
If Dir(filePath) <> "" Then
MsgBox "File exists"
Else
MsgBox "File does not exist"
End If
End Sub
The Dir
function is easy to use, but it has some limitations. For example, it can return an empty string if the file is hidden or if the path is incorrect.
Method 2: Using the FileExists
Method of the FileSystemObject
The FileSystemObject
(FSO) is a powerful tool in VBA that provides a more robust way to interact with the file system. The FileExists
method of the FSO returns a boolean value indicating whether a file exists.
Sub CheckFileExists_FSO()
Dim fso As Object
Dim filePath As String
Set fso = CreateObject("Scripting.FileSystemObject")
filePath = "C:\path\to\your\file.txt"
If fso.FileExists(filePath) Then
MsgBox "File exists"
Else
MsgBox "File does not exist"
End If
Set fso = Nothing
End Sub
The FileExists
method is more reliable than the Dir
function, as it can handle hidden files and incorrect paths more effectively.
Method 3: Using the GetAttr
Function
The GetAttr
function returns the attributes of a file, including whether it exists. This method is useful when you need to check the existence of a file and also retrieve its attributes.
Sub CheckFileExists_GetAttr()
Dim filePath As String
filePath = "C:\path\to\your\file.txt"
If GetAttr(filePath) <> 0 Then
MsgBox "File exists"
Else
MsgBox "File does not exist"
End If
End Sub
The GetAttr
function returns an integer value representing the attributes of the file. If the file exists, the value will be non-zero.
Method 4: Using the Open
Statement
The Open
statement can be used to check if a file exists by attempting to open it. If the file does not exist, the Open
statement will raise an error.
Sub CheckFileExists_Open()
Dim filePath As String
filePath = "C:\path\to\your\file.txt"
On Error Resume Next
Open filePath For Input As #1
If Err.Number = 0 Then
MsgBox "File exists"
Else
MsgBox "File does not exist"
End If
On Error GoTo 0
Close #1
End Sub
This method is not recommended, as it can lead to unexpected behavior if the file is not found.
Conclusion
In this article, we've explored four ways to check if a file exists in VBA. Each method has its own strengths and weaknesses, and the choice of method depends on the specific requirements of your project. By using these methods, you can ensure that your VBA code is robust and reliable, and provides a better user experience.
Gallery of VBA File Existence Checking Methods
VBA File Existence Checking Methods
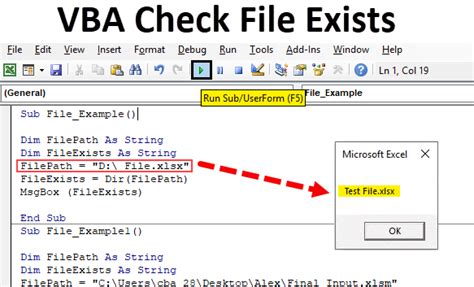
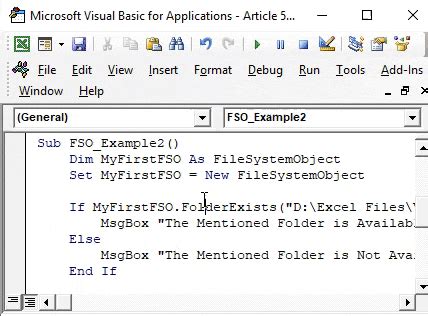
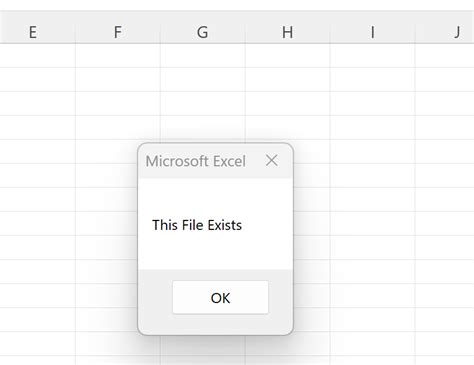
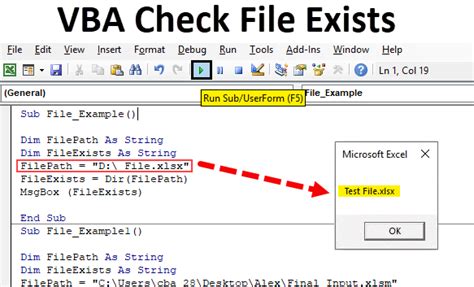
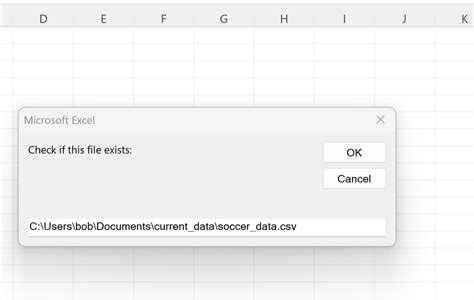
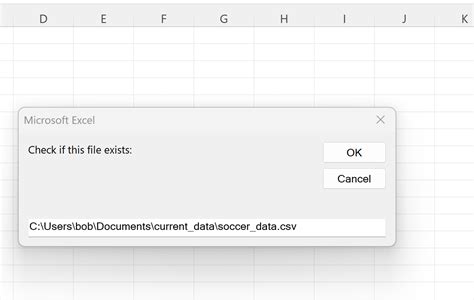
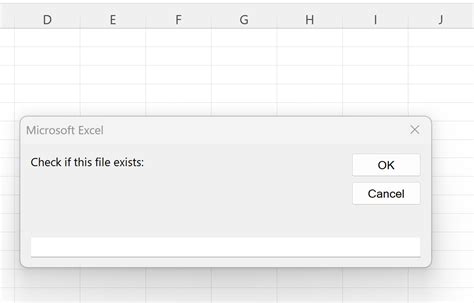
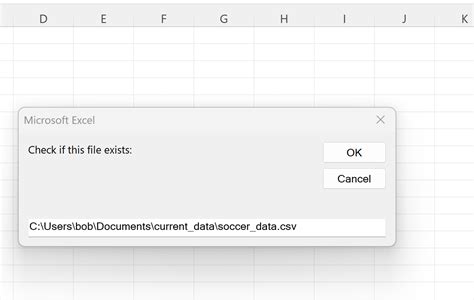
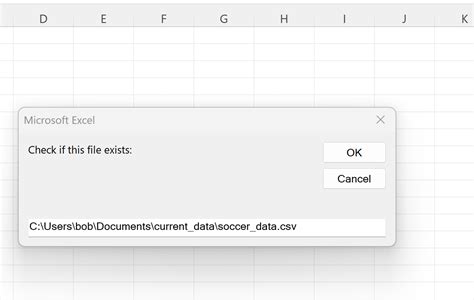
We hope this article has been helpful in exploring the different methods for checking if a file exists in VBA. If you have any questions or need further assistance, please don't hesitate to ask.