Intro
Learn how to easily check if a file exists in VBA with code examples. Discover the most efficient methods to verify file existence, including Dir() function, FileSystemObject, and error handling techniques. Master VBA file management with our step-by-step guide, covering file paths, file types, and file system errors.
The importance of checking if a file exists in VBA (Visual Basic for Applications) cannot be overstated. This simple yet crucial task is a building block for more complex operations, such as file manipulation, data import/export, and automation of various processes. When working with files in VBA, whether it's reading from, writing to, or manipulating them in any way, knowing if the file exists beforehand can save you from runtime errors and make your code more robust.
Checking if a file exists can also serve as a preliminary step for validation, ensuring that your program doesn't attempt to access a file that doesn't exist, which could otherwise lead to errors and crashes. In this article, we will delve into the different methods available in VBA to check if a file exists, providing code examples to make it easy to implement in your projects.
Why Check If a File Exists in VBA?
Before diving into the "how," it's essential to understand the "why." Checking if a file exists is fundamental for several reasons:
- Error Prevention: Attempting to open or manipulate a non-existent file will result in runtime errors. Checking the file's existence beforehand helps prevent these errors.
- Validation: It's a crucial step in validating user input or expected file paths.
- Efficiency: Your program can take alternative actions or provide informative messages to the user if the file does not exist, improving the overall user experience.
Methods to Check If a File Exists in VBA
VBA provides several methods to check if a file exists. Each has its own advantages and is suited for different scenarios.
1. Using the Dir
Function
The Dir
function is one of the simplest ways to check if a file exists. It returns the name of the file if it exists; otherwise, it returns an empty string.
Sub CheckFileExistsUsingDir()
Dim filePath As String
filePath = "C:\path\to\your\file.txt"
If Dir(filePath) <> "" Then
MsgBox "File exists."
Else
MsgBox "File does not exist."
End If
End Sub
2. Using the FileExists
Method from the FileSystemObject
The FileSystemObject
(FSO) provides a more modern and flexible way to interact with the file system. It includes a FileExists
method that directly checks for the existence of a file.
First, you need to set a reference to the Microsoft Scripting Runtime
library in your VBA project. To do this:
- Open the VBA Editor (Press
Alt+F11
in Excel). - Right-click on any of the objects for your workbook listed in the "Project Explorer" window on the left side of the VBA Editor.
- Choose
View Code
. - From the
Tools
menu, chooseReferences
. - Scroll down and check
Microsoft Scripting Runtime
. - Click
OK
.
Sub CheckFileExistsUsingFSO()
Dim fso As New FileSystemObject
Dim filePath As String
filePath = "C:\path\to\your\file.txt"
If fso.FileExists(filePath) Then
MsgBox "File exists."
Else
MsgBox "File does not exist."
End If
End Sub
3. Using Late Binding with the FileSystemObject
If setting an early reference to the FileSystemObject
is not feasible due to deployment reasons or otherwise, you can use late binding. This method does not require setting a reference in the VBA editor.
Sub CheckFileExistsUsingLateBinding()
Dim fso As Object
Dim filePath As String
filePath = "C:\path\to\your\file.txt"
Set fso = CreateObject("Scripting.FileSystemObject")
If fso.FileExists(filePath) Then
MsgBox "File exists."
Else
MsgBox "File does not exist."
End If
Set fso = Nothing
End Sub
Choosing the Right Method
- For simplicity and backward compatibility, the
Dir
function is straightforward and doesn't require any additional references. - For more functionality and modern code, using the
FileSystemObject
provides a wide range of file system operations beyond just checking if a file exists. If you're performing multiple file system operations, this is likely your best choice. - For flexibility in deployment, late binding with the
FileSystemObject
might be preferable if you're distributing your VBA project to users who might not have theMicrosoft Scripting Runtime
library referenced.
Gallery of VBA File Existence Checks
VBA File Existence Check Gallery
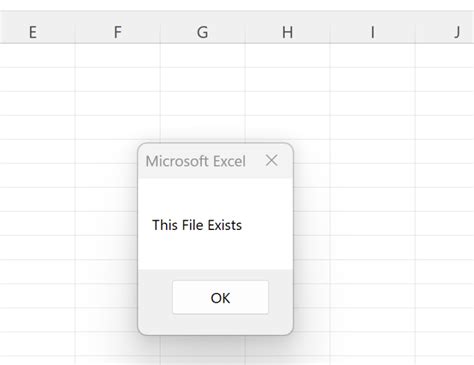
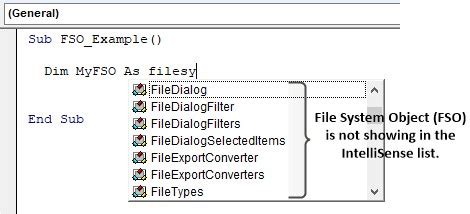
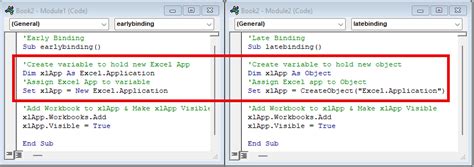
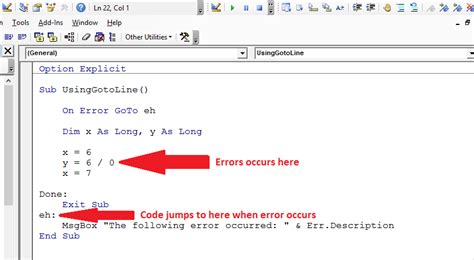
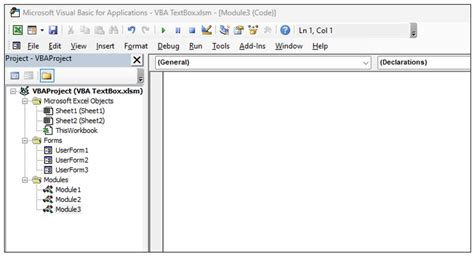
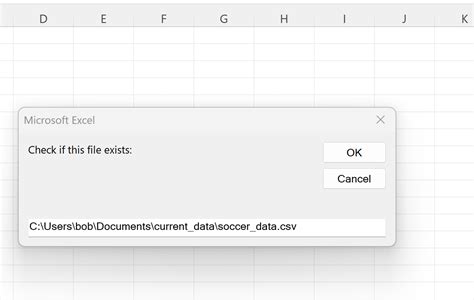
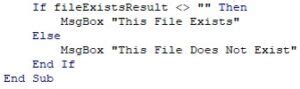
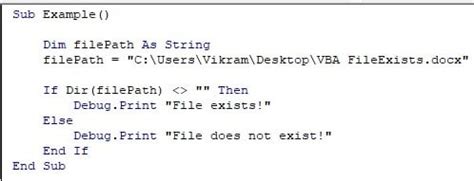
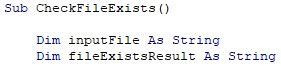
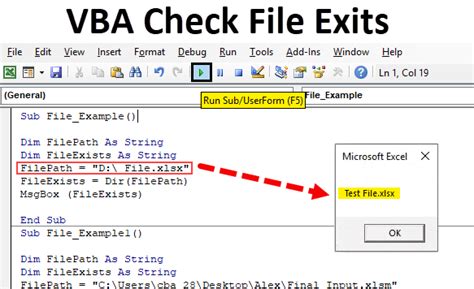
We've covered the importance of checking if a file exists in VBA, along with methods to implement this check using the Dir
function, the FileSystemObject
, and late binding. Each method has its own strengths and is suited for different scenarios, depending on your project's needs and your personal preference.
As you continue to work with files in VBA, remember the value of preliminary checks to ensure your code runs smoothly and efficiently. Whether you're automating tasks, reading/writing data, or simply validating file paths, checking if a file exists is a crucial step that should not be overlooked.
Feel free to share your experiences, ask questions, or discuss best practices in the comments section below.