Intro
Master Excel VBA with 3 simple ways to check if a sheet exists. Learn how to verify sheet existence using VBA code, avoiding errors and optimizing your macros. Discover the power of WorksheetExists, Sheets collection, and error handling techniques to improve your VBA skills and streamline your workflow.
Working with Excel Sheets in VBA: Checking if a Sheet Exists
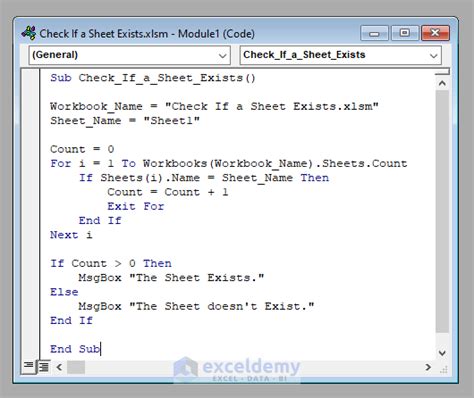
When automating tasks in Excel using VBA, it's not uncommon to need to verify whether a specific sheet exists in your workbook before attempting to manipulate it. This verification step is crucial for preventing errors and ensuring your code runs smoothly. In this article, we'll explore three different methods to check if a sheet exists in VBA.
Method 1: Using the On Error Resume Next Statement
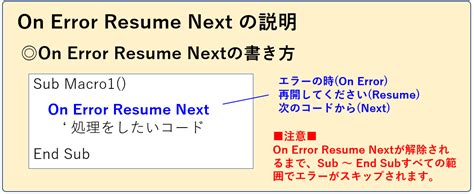
This method leverages VBA's error handling capabilities to detect if a sheet exists. The basic idea is to attempt to select the sheet; if it doesn't exist, VBA will throw an error, which you can then handle to determine the outcome.
Sub CheckSheetExists_OnError()
Dim ws As Worksheet
Dim sheetName As String
sheetName = "YourSheetName"
On Error Resume Next
Set ws = ThisWorkbook.Worksheets(sheetName)
On Error GoTo 0
If ws Is Nothing Then
MsgBox "The sheet '" & sheetName & "' does not exist."
Else
MsgBox "The sheet '" & sheetName & "' exists."
End If
End Sub
How It Works
- Define your sheet name in the
sheetName
variable. - Use
On Error Resume Next
to instruct VBA to continue executing the code even if it encounters an error. - Attempt to set a worksheet object (
ws
) to the sheet with the specified name. If the sheet does not exist, this line will throw an error, andws
will remainNothing
. - Immediately turn off error handling with
On Error GoTo 0
to ensure errors are handled normally for the rest of your code. - Check if
ws
isNothing
. If it is, the sheet does not exist.
Method 2: Looping Through Worksheets
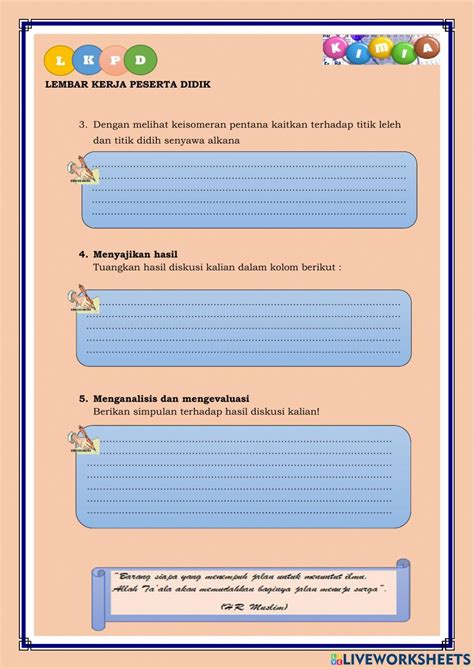
Another approach is to loop through all the worksheets in your workbook and check each sheet's name to see if it matches the one you're looking for.
Sub CheckSheetExists_Loop()
Dim ws As Worksheet
Dim sheetName As String
sheetName = "YourSheetName"
For Each ws In ThisWorkbook.Worksheets
If ws.Name = sheetName Then
MsgBox "The sheet '" & sheetName & "' exists."
Exit Sub
End If
Next ws
MsgBox "The sheet '" & sheetName & "' does not exist."
End Sub
How It Works
- Define the sheet name you're looking for in the
sheetName
variable. - Loop through each worksheet in
ThisWorkbook.Worksheets
. - Inside the loop, check if the current worksheet's name (
ws.Name
) matches the target sheet name. - If a match is found, display a message indicating the sheet exists and exit the subroutine.
- If the loop completes without finding a match, display a message indicating the sheet does not exist.
Method 3: Using the WorksheetFunction.CountIf Method
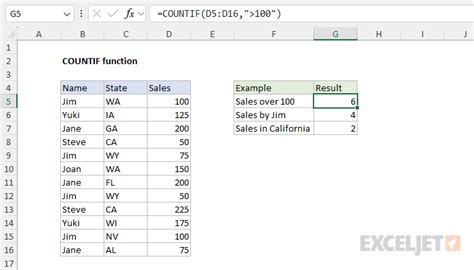
This method is a bit more indirect but leverages the WorksheetFunction.CountIf
method to check if a sheet name exists among the names of all worksheets.
Sub CheckSheetExists_CountIf()
Dim sheetName As String
sheetName = "YourSheetName"
Dim count As Long
count = Application.WorksheetFunction.CountIf(ThisWorkbook.Worksheets, sheetName)
If count > 0 Then
MsgBox "The sheet '" & sheetName & "' exists."
Else
MsgBox "The sheet '" & sheetName & "' does not exist."
End If
End Sub
How It Works
- Define the target sheet name in the
sheetName
variable. - Use
Application.WorksheetFunction.CountIf
to count how many worksheets inThisWorkbook.Worksheets
have the name specified insheetName
. - If the count is greater than 0, it means the sheet exists.
- Display a message based on the existence of the sheet.
Checking Sheet Existence in VBA Gallery
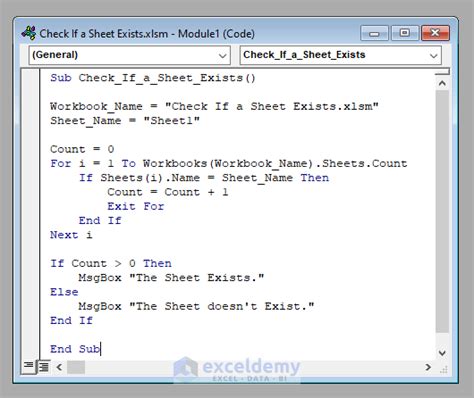
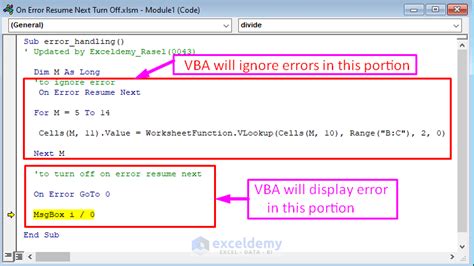
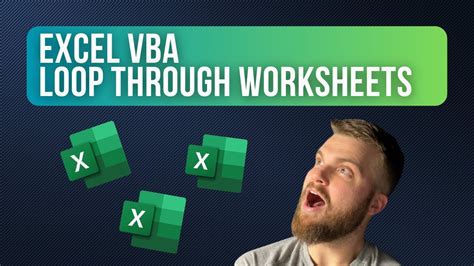
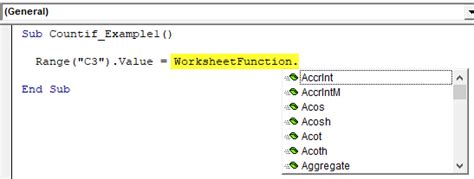
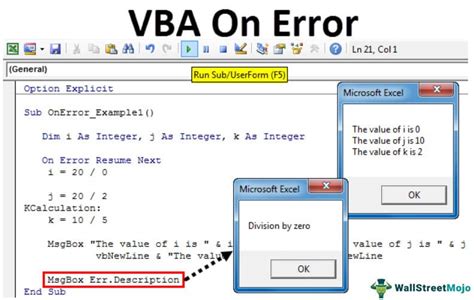
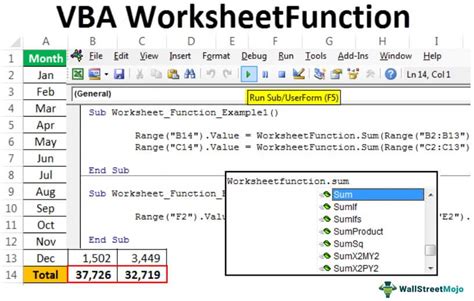
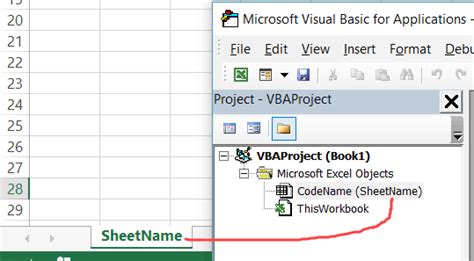
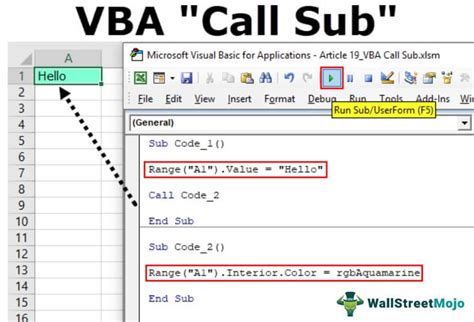
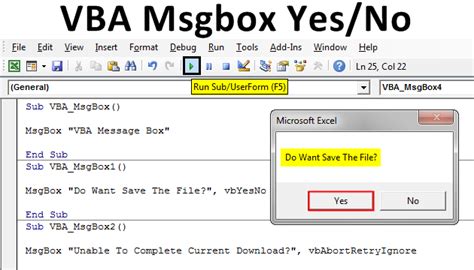
Choosing the Right Method
Each method for checking if a sheet exists in VBA has its own merits. The choice of method depends on your specific needs, such as the structure of your workbook, the number of sheets, and personal preference in coding style.
Conclusion
Verifying the existence of a sheet in VBA is a fundamental task that can help prevent errors and make your macros more robust. By understanding and applying these three methods—using On Error Resume Next
, looping through worksheets, and utilizing the WorksheetFunction.CountIf
method—you can efficiently check for sheet existence and improve your VBA coding skills.
We hope this comprehensive guide has been informative and helpful. Whether you're a beginner or an advanced VBA user, these methods will surely enhance your macro development capabilities.