Intro
Master Excel automation with VBA! Learn how to check if a worksheet exists using VBA, including error handling and worksheet enumeration. Discover how to avoid runtime errors and efficiently manage your worksheets with this essential VBA tutorial. Improve your Excel VBA skills and optimize your workflows today!
Working with worksheets in Excel VBA can be a bit tricky, especially when you need to check if a specific worksheet exists in a workbook. In this article, we'll explore how to check if a worksheet exists in Excel VBA, and provide a comprehensive guide on how to do it efficiently.
Why Check if a Worksheet Exists?
Before we dive into the code, let's quickly discuss why checking if a worksheet exists is important. Here are a few scenarios where this check is crucial:
- Error handling: When working with worksheets, you might encounter errors if you try to access a worksheet that doesn't exist. By checking if the worksheet exists, you can avoid these errors and provide a better user experience.
- Dynamic worksheet creation: In some cases, you might need to create worksheets dynamically based on user input or other factors. Checking if a worksheet exists helps you avoid creating duplicate worksheets.
- Data validation: When working with data, you might need to check if a specific worksheet exists to validate the data or perform calculations.
Checking if a Worksheet Exists
Now, let's get to the code! There are a few ways to check if a worksheet exists in Excel VBA. Here are the most common methods:
Method 1: Using the Worksheets
Collection
You can use the Worksheets
collection to check if a worksheet exists. Here's an example:
Sub CheckWorksheetExists()
Dim ws As Worksheet
Set ws = Worksheets("MyWorksheet")
If Not ws Is Nothing Then
MsgBox "Worksheet exists!"
Else
MsgBox "Worksheet does not exist!"
End If
End Sub
In this example, we try to set the ws
variable to the worksheet named "MyWorksheet". If the worksheet exists, ws
will not be Nothing
, and we can display a message indicating that the worksheet exists. If the worksheet does not exist, ws
will be Nothing
, and we can display a message indicating that the worksheet does not exist.
Method 2: Using the On Error Resume Next
Statement
Another way to check if a worksheet exists is to use the On Error Resume Next
statement. Here's an example:
Sub CheckWorksheetExists()
On Error Resume Next
Dim ws As Worksheet
Set ws = Worksheets("MyWorksheet")
If Err.Number = 0 Then
MsgBox "Worksheet exists!"
Else
MsgBox "Worksheet does not exist!"
End If
On Error GoTo 0
End Sub
In this example, we use the On Error Resume Next
statement to ignore any errors that might occur when trying to set the ws
variable to the worksheet named "MyWorksheet". If the worksheet exists, the Err.Number
property will be 0, and we can display a message indicating that the worksheet exists. If the worksheet does not exist, the Err.Number
property will not be 0, and we can display a message indicating that the worksheet does not exist.
Method 3: Using the Worksheets.Exists
Method (Excel 2013 and later)
In Excel 2013 and later, you can use the Worksheets.Exists
method to check if a worksheet exists. Here's an example:
Sub CheckWorksheetExists()
If Worksheets.Exists("MyWorksheet") Then
MsgBox "Worksheet exists!"
Else
MsgBox "Worksheet does not exist!"
End If
End Sub
In this example, we use the Worksheets.Exists
method to check if a worksheet named "MyWorksheet" exists. If the worksheet exists, the method returns True
, and we can display a message indicating that the worksheet exists. If the worksheet does not exist, the method returns False
, and we can display a message indicating that the worksheet does not exist.
Conclusion
Checking if a worksheet exists is an important task in Excel VBA, and there are several ways to do it. We've explored three common methods: using the Worksheets
collection, using the On Error Resume Next
statement, and using the Worksheets.Exists
method (Excel 2013 and later). By using these methods, you can ensure that your code is robust and error-free.
Gallery of Worksheet-Related Images
Worksheet-Related Images
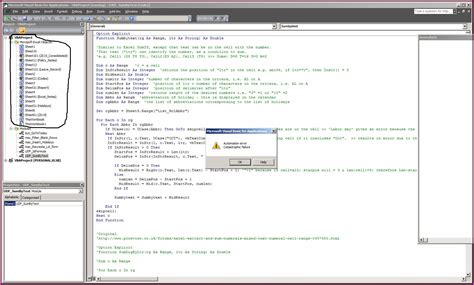
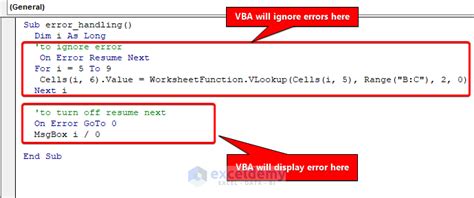
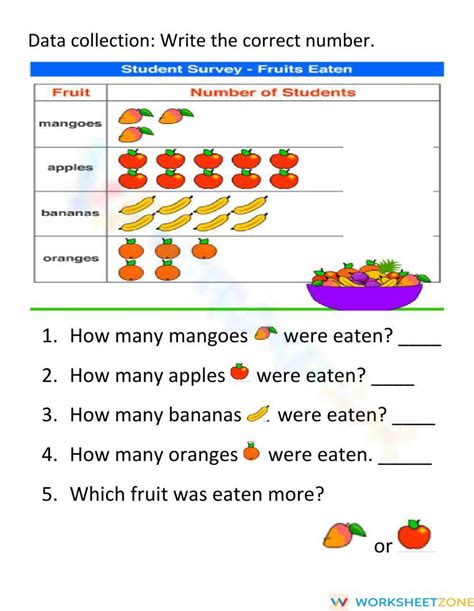
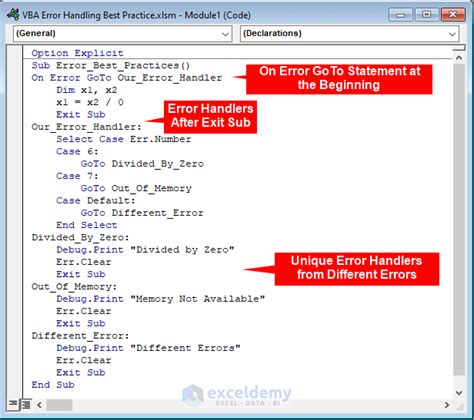
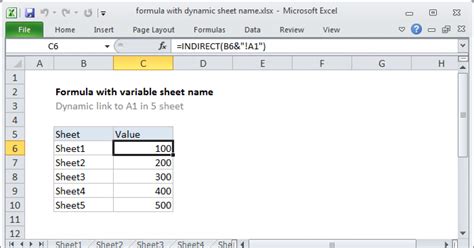
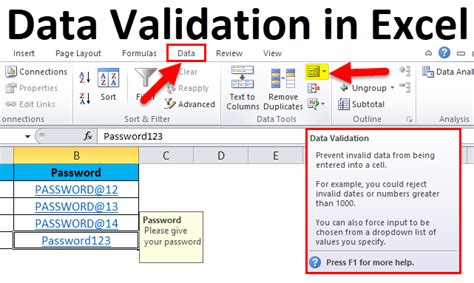
Frequently Asked Questions
- Q: How do I check if a worksheet exists in Excel VBA?
A: You can use the
Worksheets
collection, theOn Error Resume Next
statement, or theWorksheets.Exists
method (Excel 2013 and later) to check if a worksheet exists. - Q: Why is it important to check if a worksheet exists? A: Checking if a worksheet exists helps you avoid errors, ensure data validation, and perform dynamic worksheet creation.
- Q: Can I use the
Worksheets.Exists
method in earlier versions of Excel? A: No, theWorksheets.Exists
method is only available in Excel 2013 and later. In earlier versions, you can use theWorksheets
collection or theOn Error Resume Next
statement to check if a worksheet exists.