Intro
Discover the power of VBA table manipulation. Learn 5 efficient ways to clear a table in VBA without deleting it, using methods such as clearing cell contents, resetting table ranges, and utilizing VBA loops. Improve your Excel automation skills and boost productivity with these expert-approved techniques.
When working with tables in VBA, there are instances where you might need to clear the content of a table without deleting it. This is particularly useful when you want to reuse the table structure but remove all the existing data. Deleting a table and then recreating it can be cumbersome, especially if the table has a complex structure or is tied to other elements in your spreadsheet. Here are five ways to clear a table in VBA without deleting it:
Method 1: Clearing a Table Using the `ClearContents` Method
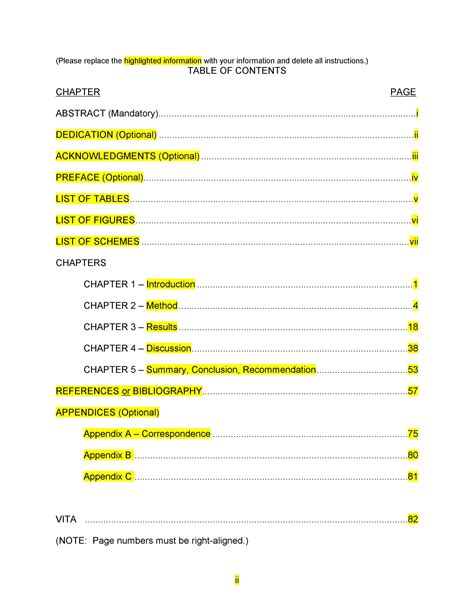
One of the simplest methods to clear the contents of a table is by using the ClearContents
method. This method removes all data from the cells in the specified range but leaves the formatting intact.
Sub ClearTableContents()
Dim tbl As ListObject
Set tbl = ThisWorkbook.Worksheets("YourSheetName").ListObjects("YourTableName")
tbl.DataBodyRange.ClearContents
End Sub
How It Works
ThisWorkbook.Worksheets("YourSheetName")
refers to the worksheet where your table is located. Replace"YourSheetName"
with the actual name of your worksheet.ListObjects("YourTableName")
refers to the table you want to clear. Replace"YourTableName"
with the actual name of your table.DataBodyRange
refers to the range of cells containing the data in the table, excluding the header row.ClearContents
clears the data from the specified range.
Method 2: Using the `Clear` Method
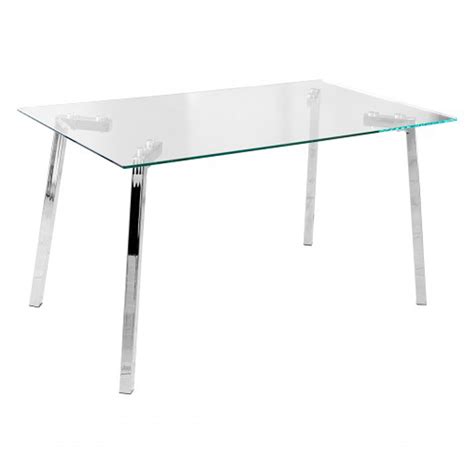
Similar to the ClearContents
method, the Clear
method removes all data from the cells, but it also removes any formatting.
Sub ClearTable()
Dim tbl As ListObject
Set tbl = ThisWorkbook.Worksheets("YourSheetName").ListObjects("YourTableName")
tbl.DataBodyRange.Clear
End Sub
Key Differences
- Unlike
ClearContents
,Clear
removes both data and formatting.
Method 3: Looping Through Each Cell
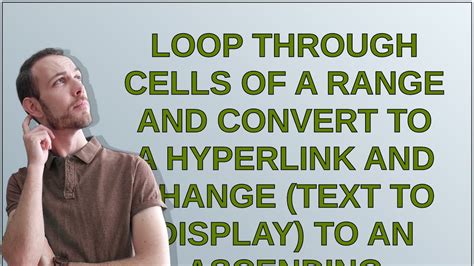
For a more granular approach, you can loop through each cell in the table and clear its contents.
Sub LoopAndClear()
Dim tbl As ListObject
Dim cell As Range
Set tbl = ThisWorkbook.Worksheets("YourSheetName").ListObjects("YourTableName")
For Each cell In tbl.DataBodyRange
cell.ClearContents
Next cell
End Sub
Advantages
- Allows for checking or manipulating each cell individually before clearing.
Method 4: Using `Range` Object
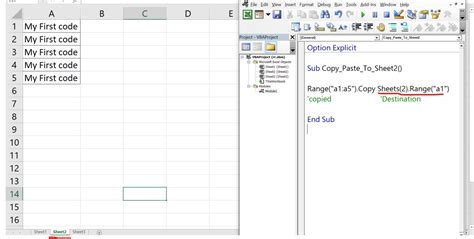
You can also directly reference the range of the table's data body and clear it.
Sub ClearRange()
Dim rng As Range
Set rng = ThisWorkbook.Worksheets("YourSheetName").ListObjects("YourTableName").DataBodyRange
rng.ClearContents
End Sub
Method 5: Using `Delete` Method with `Shift` Parameter
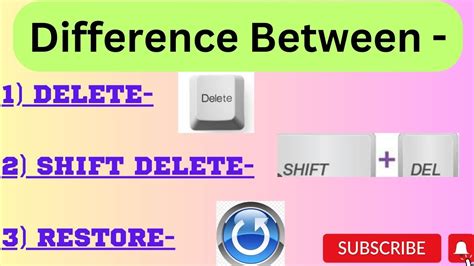
Another approach is to delete the contents by shifting cells up.
Sub DeleteAndShift()
Dim tbl As ListObject
Set tbl = ThisWorkbook.Worksheets("YourSheetName").ListObjects("YourTableName")
tbl.DataBodyRange.Delete Shift:=xlUp
End Sub
Considerations
- This method physically deletes cells, which might affect other data or formulas referencing these cells.
Clearing Table Contents Gallery
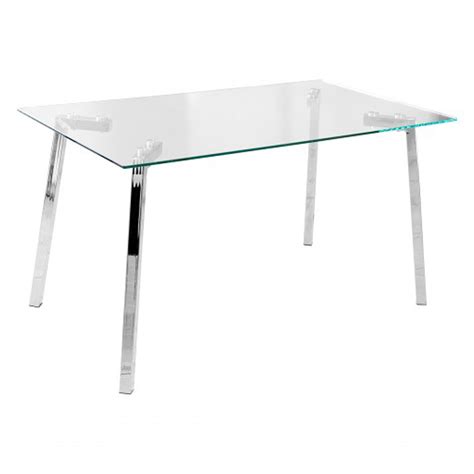
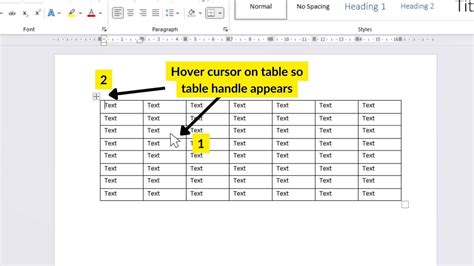
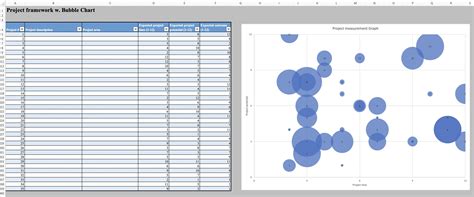
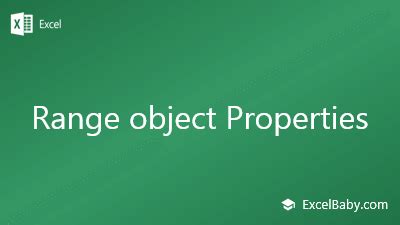
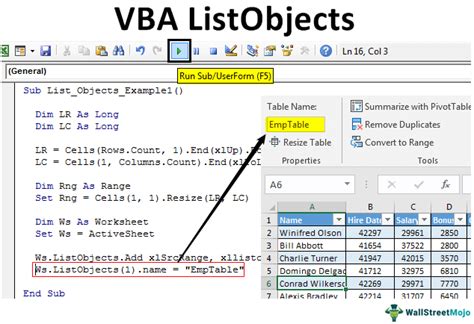
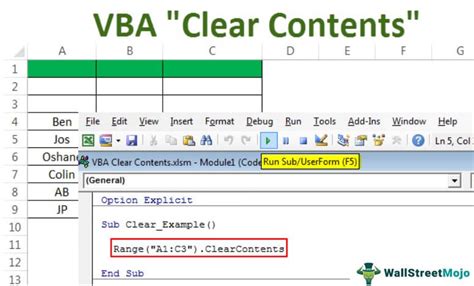
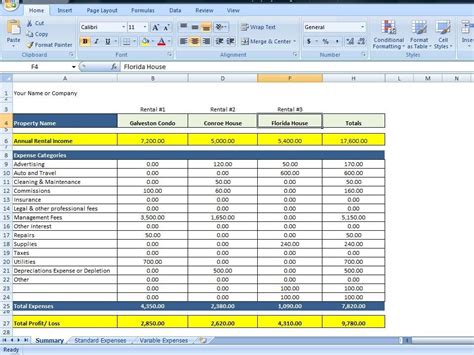
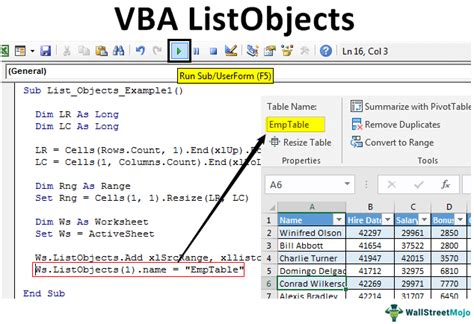
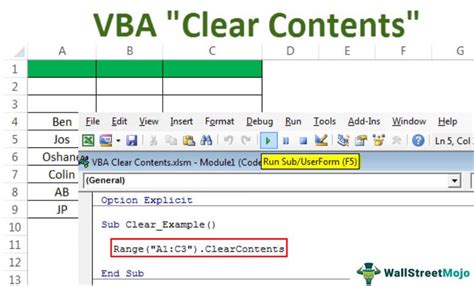
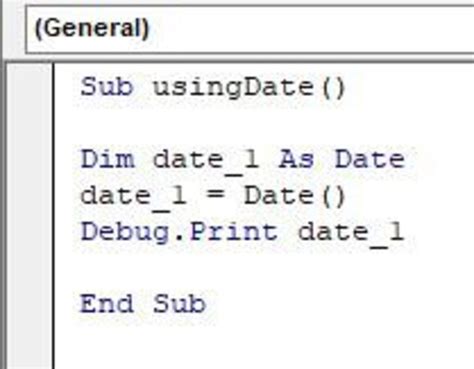
Whether you're automating reports, managing data entry templates, or simply need to reset a table for new data, these methods provide flexible ways to clear table contents in VBA without deleting the table itself. Remember to adjust the code snippets to fit your specific worksheet and table names.