Intro
Discover how to automate column width adjustments in Excel using VBA code. Learn 5 efficient methods to autofit column width, including using Range, Cells, and Worksheet events. Improve your spreadsheet management with these expert-approved VBA code snippets and optimize your column widths for better data visualization.
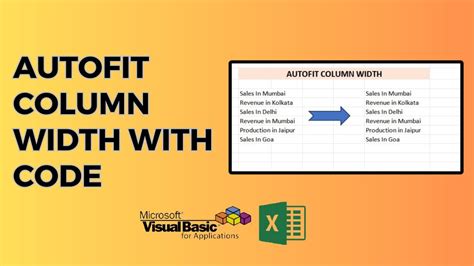
Excel is a powerful tool for data analysis, and when working with large datasets, it's essential to ensure that the column widths are adjusted correctly for better readability. Autofitting column widths can be done manually, but with VBA code, you can automate this process and save time. In this article, we'll explore five ways to autofit column widths using VBA code.
What is Autofit?
Autofit is a feature in Excel that allows you to automatically adjust the width of columns or rows to fit the contents. This feature is useful when you have large datasets and want to ensure that the column widths are adjusted correctly to prevent data from being truncated or hidden.
Why Use VBA Code for Autofit?
While Excel provides a built-in autofit feature, using VBA code can offer more flexibility and control over the autofit process. With VBA code, you can automate the autofit process, apply it to specific ranges or worksheets, and even schedule it to run at regular intervals.
Method 1: Autofit Entire Worksheet
Autofit Entire Worksheet
To autofit the entire worksheet using VBA code, you can use the following code:
Sub AutofitEntireWorksheet()
Dim ws As Worksheet
Set ws = ActiveSheet
ws.Cells.EntireColumn.AutoFit
End Sub
This code sets the active worksheet as the target worksheet and then uses the AutoFit
method to adjust the column widths.
Method 2: Autofit Specific Range
Autofit Specific Range
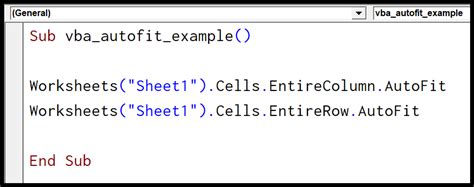
To autofit a specific range of cells using VBA code, you can use the following code:
Sub AutofitSpecificRange()
Dim rng As Range
Set rng = Range("A1:E10") ' adjust the range as needed
rng.Columns.AutoFit
End Sub
This code sets the target range as A1:E10 and then uses the AutoFit
method to adjust the column widths.
Method 3: Autofit Based on Cell Contents
Autofit Based on Cell Contents
To autofit column widths based on cell contents using VBA code, you can use the following code:
Sub AutofitBasedOnCellContents()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim rng As Range
Set rng = ws.UsedRange
rng.Columns.AutoFit
End Sub
This code sets the target range as the used range of the active worksheet and then uses the AutoFit
method to adjust the column widths based on the cell contents.
Method 4: Autofit with Multiple Criteria
Autofit with Multiple Criteria
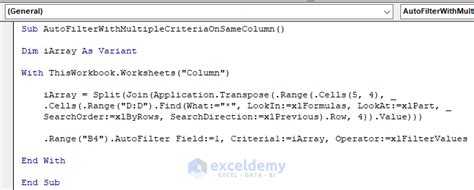
To autofit column widths based on multiple criteria using VBA code, you can use the following code:
Sub AutofitMultipleCriteria()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim rng As Range
Set rng = Range("A1:E10") ' adjust the range as needed
Dim crit1 As Variant
crit1 = Array(" Criteria 1", "Criteria 2")
Dim crit2 As Variant
crit2 = Array(" Criteria 3", "Criteria 4")
rng.Columns.AutoFit
For Each cell In rng
If cell.Value Like crit1(0) Or cell.Value Like crit1(1) Then
cell.Columns.AutoFit
ElseIf cell.Value Like crit2(0) Or cell.Value Like crit2(1) Then
cell.Columns.AutoFit
End If
Next cell
End Sub
This code sets the target range as A1:E10 and then uses the AutoFit
method to adjust the column widths based on multiple criteria.
Method 5: Autofit with Dynamic Range
Autofit with Dynamic Range
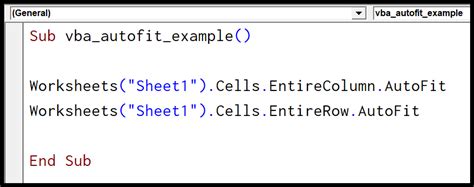
To autofit column widths with a dynamic range using VBA code, you can use the following code:
Sub AutofitDynamicRange()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim rng As Range
Set rng = ws.Range("A1").CurrentRegion
rng.Columns.AutoFit
End Sub
This code sets the target range as the current region of cell A1 and then uses the AutoFit
method to adjust the column widths.
Gallery of Autofit VBA Code Examples
Autofit VBA Code Examples
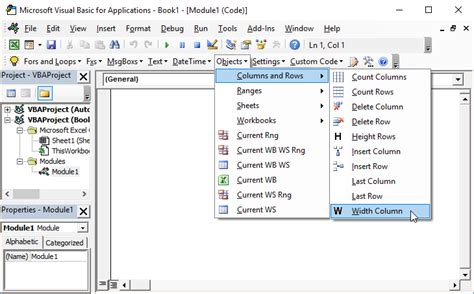
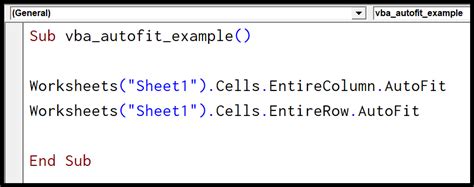
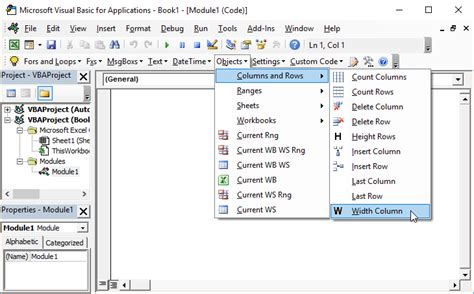
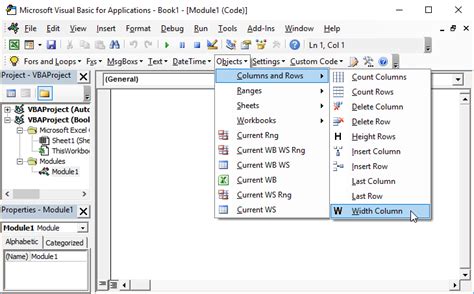
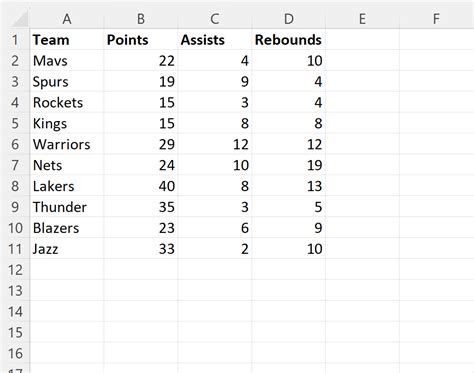
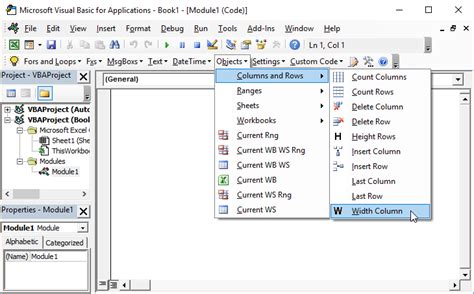
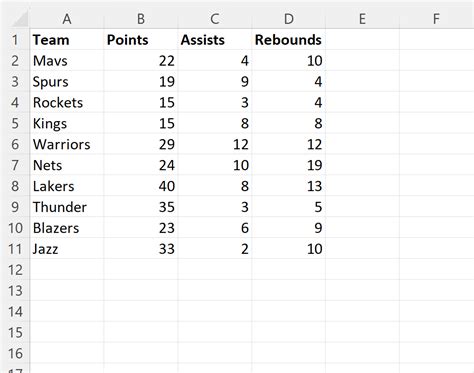
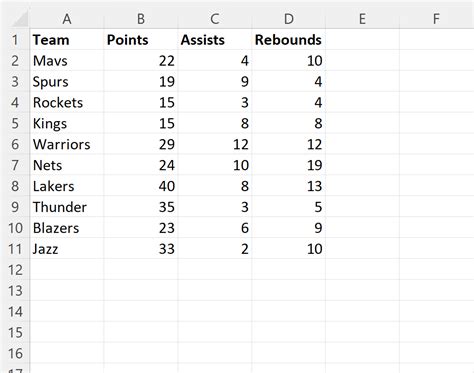
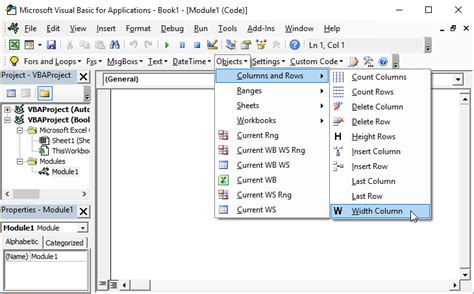
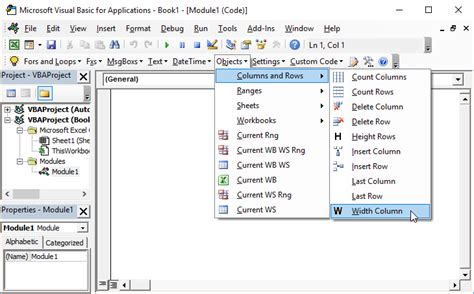
We hope you find these examples helpful in learning how to autofit column widths with VBA code. Do you have any questions or need further assistance? Please leave a comment below, and we'll be happy to help!