Intro
Discover how to select a sheet with VBA code in Excel. Learn 5 efficient methods to automate sheet selection, including using sheet index, name, and active sheet. Master VBA techniques for worksheet manipulation, error handling, and optimization. Boost productivity and streamline your workflow with these expert-approved VBA code snippets.
Working with Excel sheets using VBA (Visual Basic for Applications) can greatly enhance your productivity and automate tasks. One of the fundamental actions you might want to perform is selecting a specific sheet. This can be particularly useful when you have multiple sheets in your workbook and you want to manipulate data on a specific one. Here are five ways to select a sheet using VBA code, each with its own utility depending on your needs.
1. Selecting a Sheet by Name
This is one of the most straightforward methods. You can select a sheet by directly referencing its name. If you know the exact name of the sheet you want to select, this method is very efficient.
Sub SelectSheetByName()
Worksheets("Sheet1").Select
End Sub
Replace "Sheet1"
with the name of the sheet you want to select. Be cautious with this method because if the sheet name is misspelled or does not exist, VBA will throw an error.
2. Selecting a Sheet by Index
Every sheet in a workbook has an index number, which is its position in the workbook. You can select a sheet by its index, starting from 1 for the first sheet.
Sub SelectSheetByIndex()
Worksheets(1).Select
End Sub
This code selects the first sheet in the workbook. This method is useful if you're working with a dynamic set of sheets where names might change but the order remains the same.
3. Looping Through All Sheets
If you need to perform an action on all sheets in your workbook, you can loop through them. This method doesn't exactly "select" a sheet in the traditional sense but allows you to interact with each sheet in turn.
Sub LoopThroughSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
'Your code here
ws.Range("A1").Value = "Processed"
Next ws
End Sub
This example changes the value of cell A1 in each sheet to "Processed". You can replace the code inside the loop with whatever action you need to perform on each sheet.
4. Selecting a Sheet Based on a Condition
Sometimes, you might want to select a sheet based on a specific condition, such as its name containing a certain word or its index being within a certain range. You can use an IF statement to achieve this.
Sub SelectSheetByCondition()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If InStr(ws.Name, "Report") > 0 Then
ws.Select
Exit For
End If
Next ws
End Sub
This code selects the first sheet whose name contains "Report". You can adjust the condition to fit your needs.
5. Selecting a Sheet Using InputBox
If you want the user to select a sheet from a list, you can use an InputBox to prompt them to choose. This method is interactive and requires the user's input.
Sub SelectSheetViaInputBox()
Dim wsName As String
wsName = InputBox("Enter the sheet name to select:", "Sheet Selector")
Worksheets(wsName).Select
End Sub
This code prompts the user to enter a sheet name, then selects that sheet. Be aware that this method will throw an error if the user enters a name that does not match any sheet.
Conclusion
Each of these methods has its place depending on your specific needs. Whether you're working with a static set of sheets, a dynamic set, or need user interaction, there's a way to select sheets in VBA that fits your scenario.
Gallery of VBA and Excel
VBA and Excel Gallery
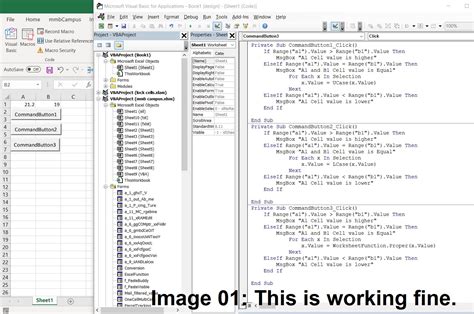
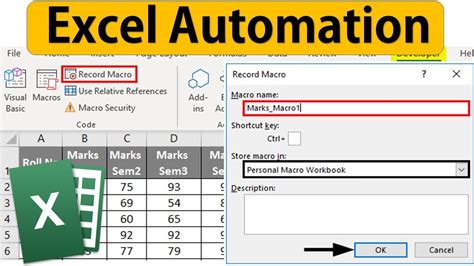
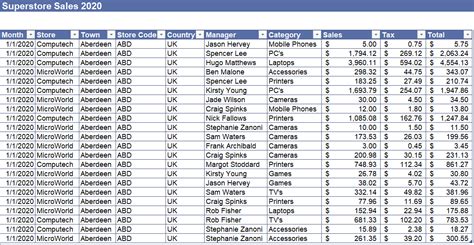
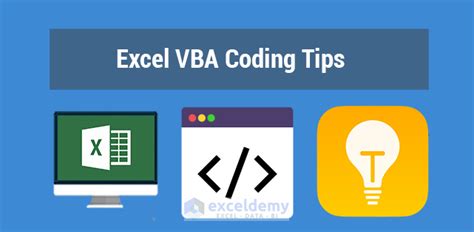
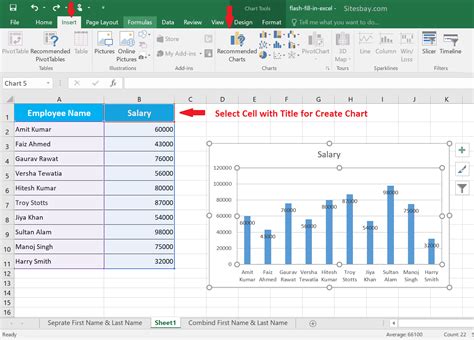
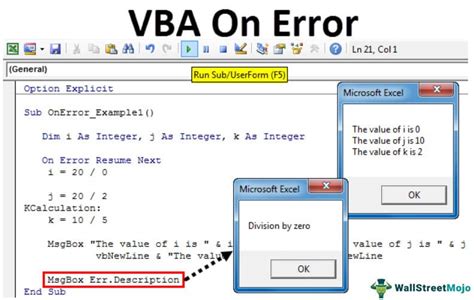
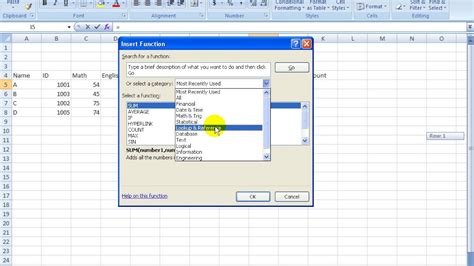
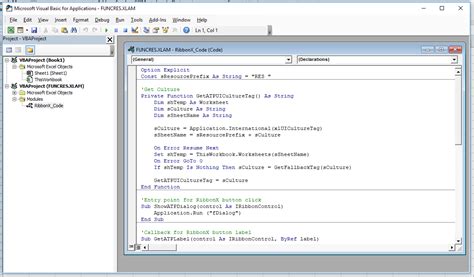
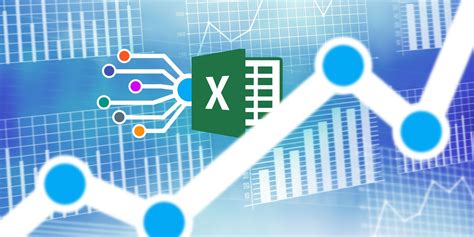
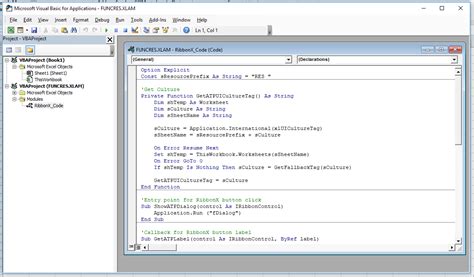
Feel free to share your experiences or ask questions about using VBA to select sheets in Excel in the comments below!