Intro
Master VBA coding with 5 efficient ways to comment block code. Learn how to use the block, comment, and REM statements to streamline your VBA projects. Discover the difference between commenting and uncommenting code, and how to use VBAs built-in features to improve code readability and maintainability.
As a programmer, you understand the importance of commenting your code. Comments help explain the purpose and functionality of your code, making it easier for others (and yourself) to understand. In VBA, commenting a block of code is a straightforward process. Here are five ways to comment a block of code in VBA:
Why Comment Your Code?
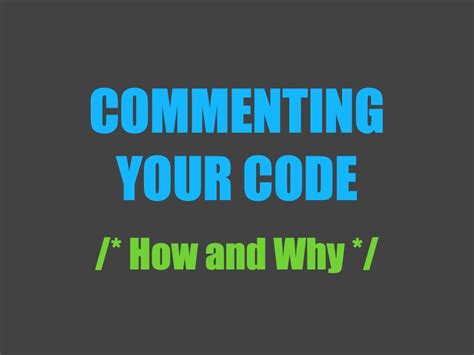
Before we dive into the methods, let's quickly discuss why commenting your code is crucial. Comments:
- Improve code readability
- Help others understand your code
- Serve as a reminder for yourself
- Facilitate debugging and troubleshooting
- Enhance collaboration and knowledge sharing
Method 1: Using the apostrophe (')
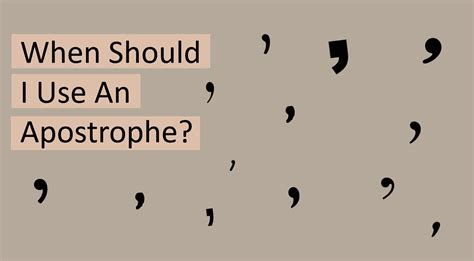
The most common way to comment a line of code in VBA is by using the apostrophe (') symbol. Simply place the apostrophe at the beginning of the line, and everything that follows will be considered a comment.
' This is a commented line
To comment a block of code, place the apostrophe at the beginning of each line:
' This is the first line of commented code
' This is the second line of commented code
' This is the third line of commented code
Method 2: Using the REM statement
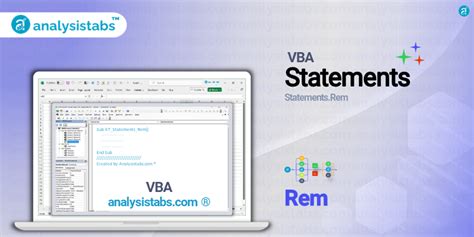
Another way to comment a line of code is by using the REM statement. REM is short for "remark," and it serves the same purpose as the apostrophe.
REM This is a commented line
To comment a block of code, use the REM statement at the beginning of each line:
REM This is the first line of commented code
REM This is the second line of commented code
REM This is the third line of commented code
Method 3: Using the '''''' delimiter
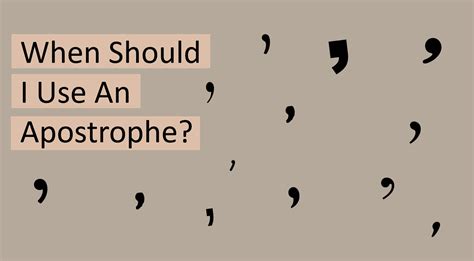
You can also use the double apostrophe ('') delimiter to comment a block of code. This method is useful when you need to comment multiple lines of code.
'''' This is a commented block of code
'''' Everything within the double apostrophes is commented
'''' You can write as many lines as needed
'''' And they will all be considered comments
'''' This is the end of the commented block
Method 4: Using the '''''' delimiter with REM
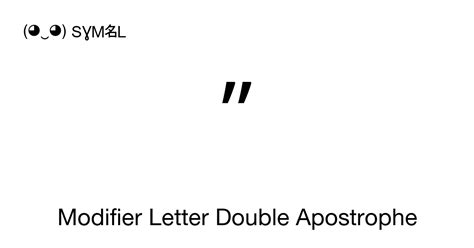
You can combine the double apostrophe delimiter with the REM statement to comment a block of code.
'''' REM This is a commented block of code
'''' REM Everything within the double apostrophes is commented
'''' REM You can write as many lines as needed
'''' REM And they will all be considered comments
'''' REM This is the end of the commented block
Method 5: Using the Visual Basic Editor's Comment Block feature
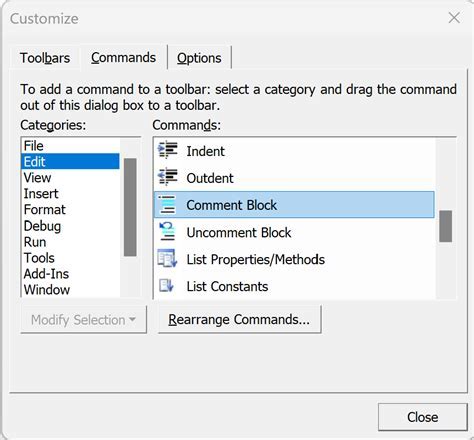
The Visual Basic Editor provides a built-in feature to comment and uncomment blocks of code. To use this feature, select the block of code you want to comment, then go to the "Edit" menu and select "Comment Block" (or press Ctrl+R).
' This is a commented block of code
' The Visual Basic Editor will automatically add the apostrophes
' For each line of code in the selected block
Conclusion
Commenting your code is an essential part of programming. By using one of these five methods, you can effectively comment your code and make it easier for others to understand. Remember to use comments to explain the purpose and functionality of your code, and don't be afraid to use them liberally throughout your projects.
VBA Commenting Image Gallery
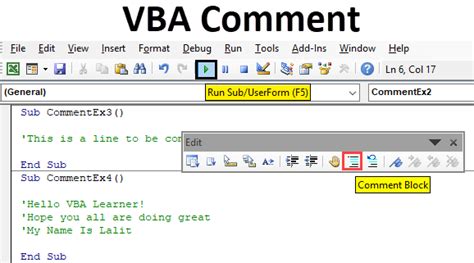
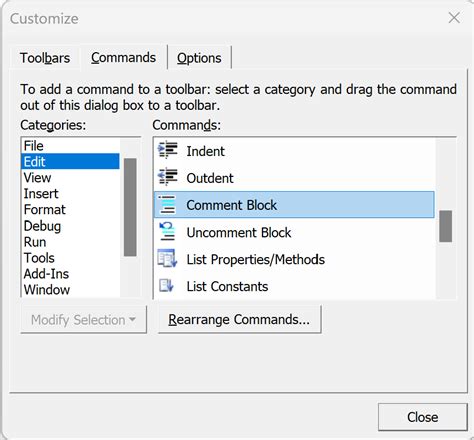
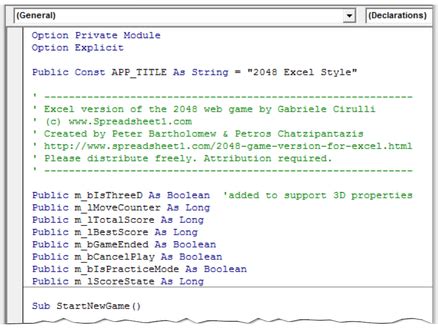
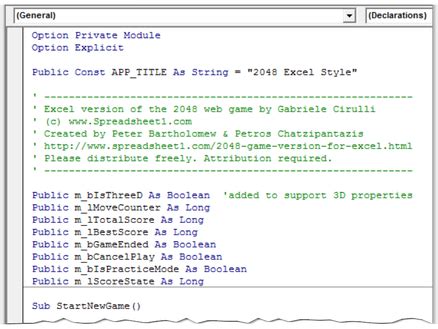
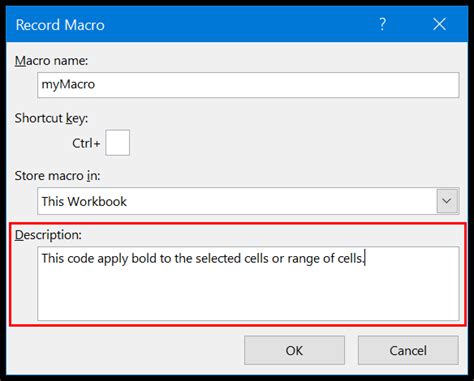
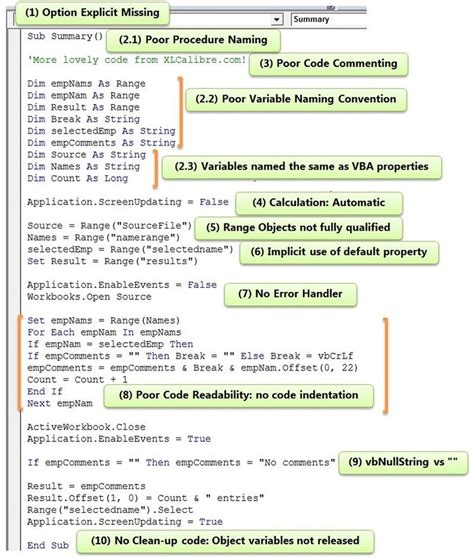
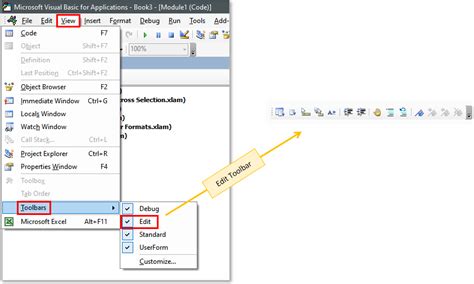
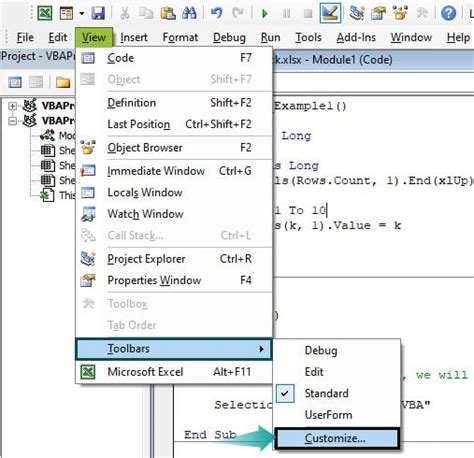
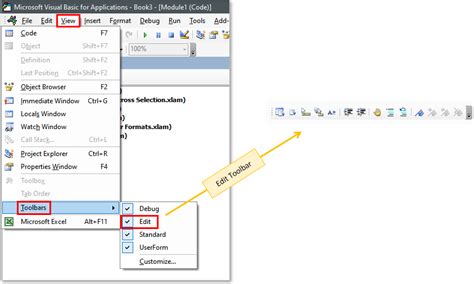