Intro
Discover the top 5 methods to convert string to date in VBA, including using the DateValue function, CDate function, and more. Master date conversion techniques and efficiently handle dates in your Excel VBA projects. Learn how to parse dates from strings, handle formatting issues, and optimize your VBA code for accurate date manipulation.
When working with dates in VBA, it's essential to ensure that the data is in a format that can be recognized and manipulated by the program. Converting strings to dates is a common task, and there are several ways to achieve this in VBA. In this article, we'll explore five methods to convert strings to dates in VBA.
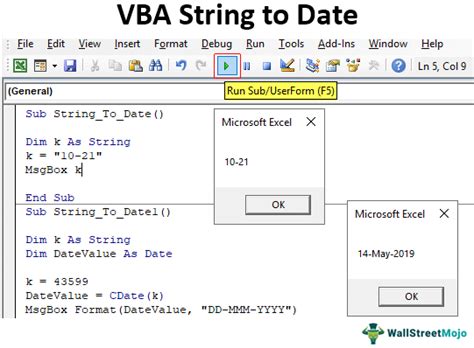
Understanding Date Formats in VBA
Before we dive into the methods, it's crucial to understand how VBA interprets dates. VBA stores dates as serial numbers, where each number represents a unique date. The serial number is calculated based on the number of days since December 30, 1899, which is considered day 1 in the VBA calendar.
VBA can recognize dates in various formats, including MM/DD/YYYY, DD/MM/YYYY, and YYYY/MM/DD. However, the default format used by VBA is MM/DD/YYYY. When working with dates, it's essential to ensure that the format is consistent to avoid errors.
Method 1: Using the CDate Function
The CDate function is the most straightforward method to convert a string to a date in VBA. This function takes a string as an argument and returns a date value.
Dim dateString As String
Dim dateValue As Date
dateString = "2022-07-25"
dateValue = CDate(dateString)
The CDate function can recognize most date formats, including MM/DD/YYYY, DD/MM/YYYY, and YYYY/MM/DD.
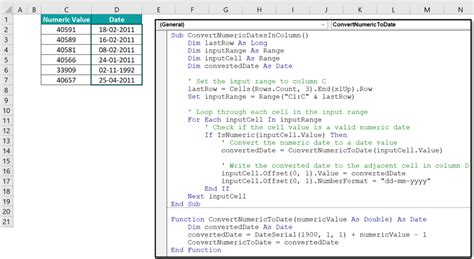
Method 2: Using the DateValue Function
The DateValue function is similar to the CDate function, but it's more flexible. This function takes a string as an argument and returns a date value.
Dim dateString As String
Dim dateValue As Date
dateString = "2022-07-25"
dateValue = DateValue(dateString)
The DateValue function can recognize most date formats, including MM/DD/YYYY, DD/MM/YYYY, and YYYY/MM/DD.
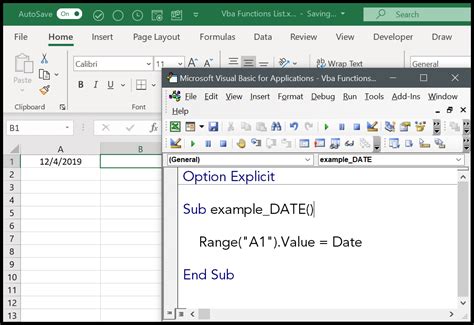
Method 3: Using the Format Function
The Format function can be used to convert a string to a date by specifying the format of the date string.
Dim dateString As String
Dim dateValue As Date
dateString = "2022-07-25"
dateValue = Format(dateString, "yyyy-mm-dd")
The Format function requires the date string to be in the specified format. If the date string is not in the correct format, the function will return an error.
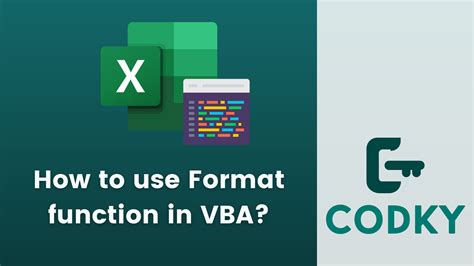
Method 4: Using the Split Function
The Split function can be used to convert a string to a date by splitting the string into its component parts (year, month, and day).
Dim dateString As String
Dim dateParts() As String
Dim dateValue As Date
dateString = "2022-07-25"
dateParts = Split(dateString, "-")
dateValue = DateSerial(dateParts(0), dateParts(1), dateParts(2))
The Split function requires the date string to be in a format that can be split into its component parts.
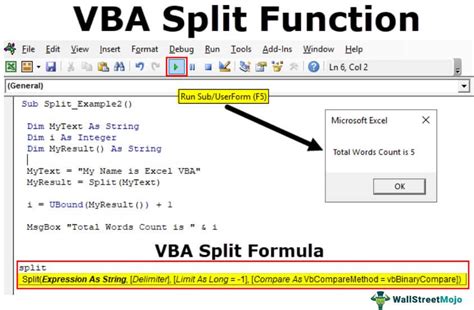
Method 5: Using the DateSerial Function
The DateSerial function can be used to convert a string to a date by specifying the year, month, and day components of the date.
Dim dateString As String
Dim dateValue As Date
dateString = "2022-07-25"
dateValue = DateSerial(2022, 7, 25)
The DateSerial function requires the year, month, and day components of the date to be specified.
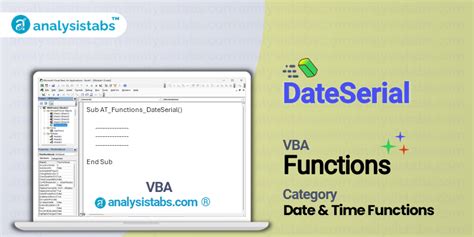
Gallery of VBA Date Conversion Methods
VBA Date Conversion Methods
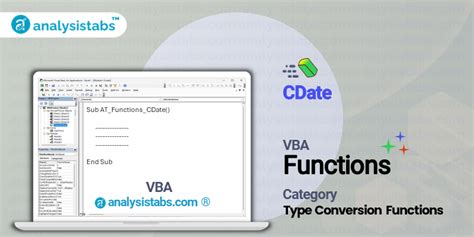
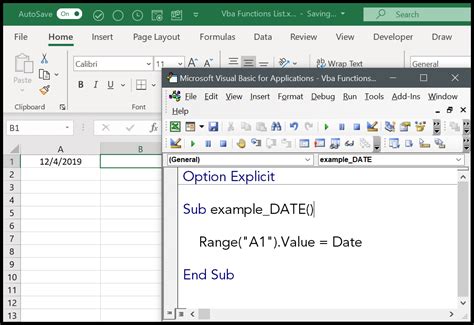
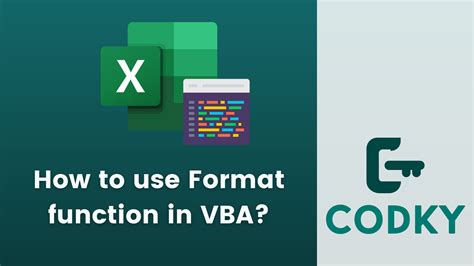
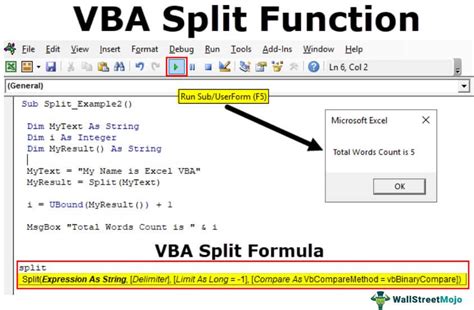
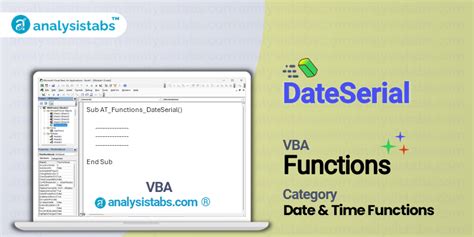
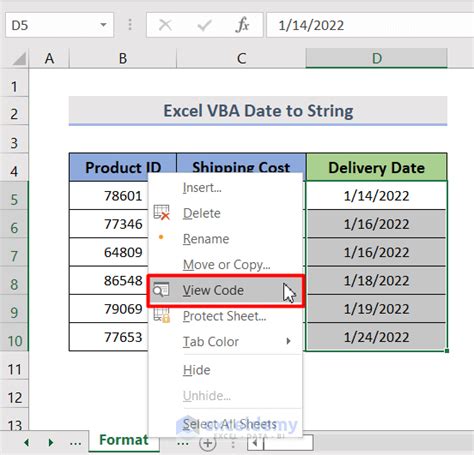
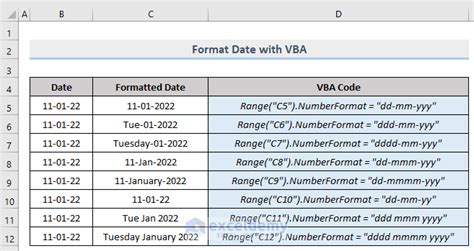
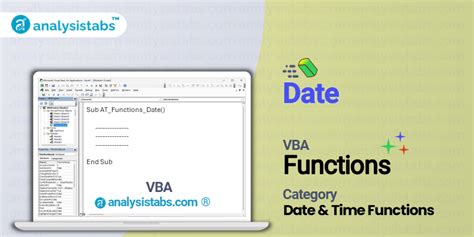
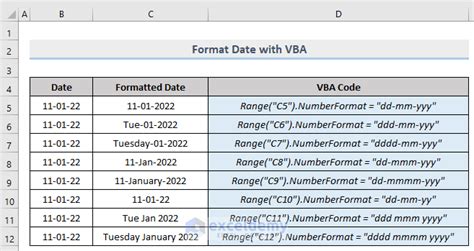
Conclusion
Converting strings to dates in VBA is a common task that can be achieved using various methods. The CDate function, DateValue function, Format function, Split function, and DateSerial function are all useful methods for converting strings to dates. Each method has its own advantages and disadvantages, and the choice of method depends on the specific requirements of the task.
By understanding the different methods available for converting strings to dates in VBA, developers can write more efficient and effective code that accurately handles date data.
We hope this article has been helpful in explaining the different methods for converting strings to dates in VBA. If you have any questions or need further clarification, please don't hesitate to ask.