Intro
Discover how to convert text to numbers in VBA with our expert guide. Learn 5 efficient methods to convert text to numerical values, including using the Value function, multiplying by 1, and utilizing the CLNG function. Improve your Excel skills and optimize your VBA code with these practical solutions for text-to-number conversions.
Converting text to numbers is a common task in Excel VBA programming. Whether you're working with user input, data imports, or formula calculations, ensuring that numbers are treated as numbers rather than text is crucial for accurate computations and data analysis. In Excel VBA, there are several methods to convert text to numbers, each with its own set of circumstances under which it is most appropriately used. Here, we'll explore five primary ways to achieve this conversion.
Understanding the Need for Conversion
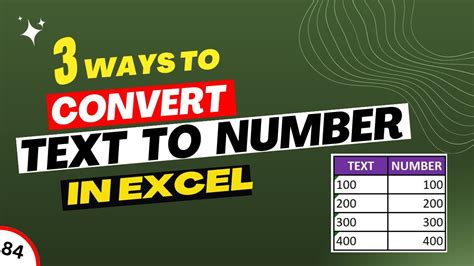
Before diving into the methods, it's essential to understand why text-to-number conversion is necessary. In Excel, a value can be treated as text even if it appears to be a number. This often happens when data is imported from external sources or when users enter numbers in a format that Excel doesn't recognize as numeric (e.g., numbers enclosed in quotes). Performing mathematical operations on these "text-numbers" can lead to errors or unexpected results.
Method 1: Using the `VAL()` Function
One of the simplest ways to convert text to numbers in VBA is by using the VAL()
function. This function attempts to convert a string to a number, stopping at the first character that cannot be interpreted as part of a number.
Sub TextToNumberUsingVAL()
Dim textValue As String
textValue = "123.45"
Dim numericValue As Double
numericValue = Val(textValue)
MsgBox numericValue
End Sub
Method 2: Utilizing the `CDbl()` Function
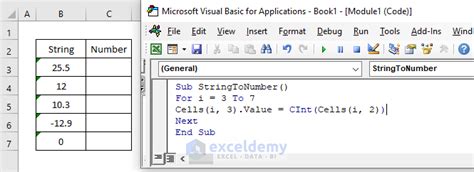
The CDbl()
function is used to convert a value to a Double data type, which is a common numeric data type in VBA. This method is more robust than VAL()
as it can handle decimal points and negative numbers but will throw an error if the conversion fails.
Sub TextToNumberUsingCDbl()
Dim textValue As String
textValue = "-123.45"
Dim numericValue As Double
numericValue = CDbl(textValue)
MsgBox numericValue
End Sub
Method 3: Applying the `CLng()` Function
If you're dealing with whole numbers, the CLng()
function is a good choice for conversion. It converts a value to a Long data type, which is a 32-bit integer.
Sub TextToNumberUsingCLng()
Dim textValue As String
textValue = "123"
Dim numericValue As Long
numericValue = CLng(textValue)
MsgBox numericValue
End Sub
Method 4: Leveraging the `CInt()` Function
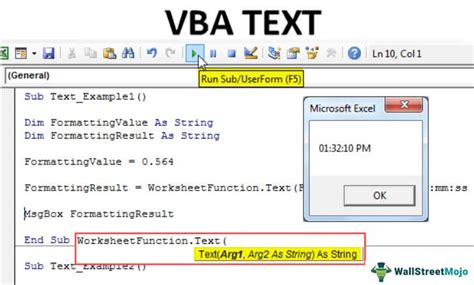
Similar to CLng()
, the CInt()
function converts a value to an Integer data type, which is a 16-bit integer. This is suitable for smaller whole numbers.
Sub TextToNumberUsingCInt()
Dim textValue As String
textValue = "123"
Dim numericValue As Integer
numericValue = CInt(textValue)
MsgBox numericValue
End Sub
Method 5: Using Range.Value2 Property
For values stored in Excel cells, you can use the Value2
property of the Range
object to convert text to numbers. This method is particularly useful when dealing with data in worksheets.
Sub TextToNumberUsingValue2()
Range("A1").Value = "123.45"
Dim numericValue As Double
numericValue = Range("A1").Value2
MsgBox numericValue
End Sub
Gallery of VBA Text to Number Conversion
VBA Text to Number Conversion Gallery
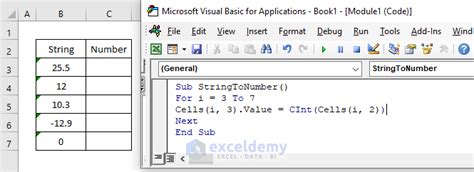
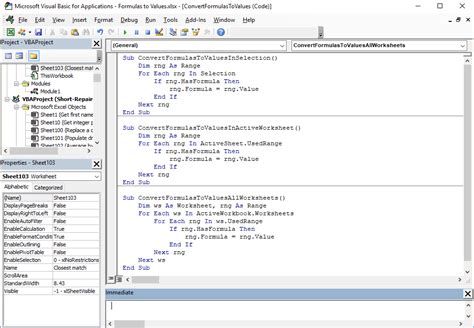
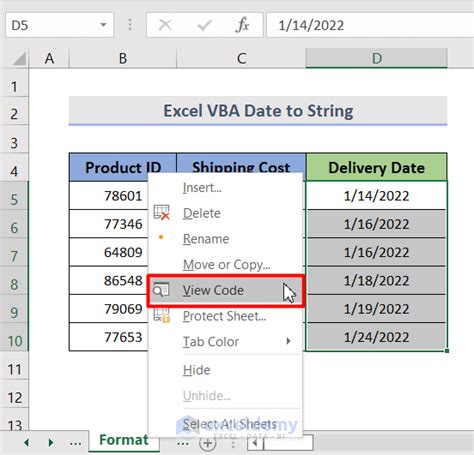
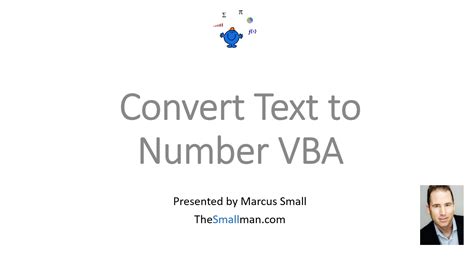
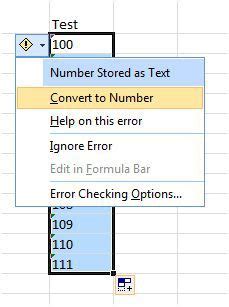
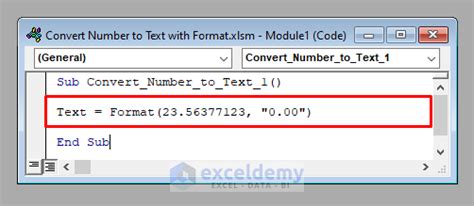
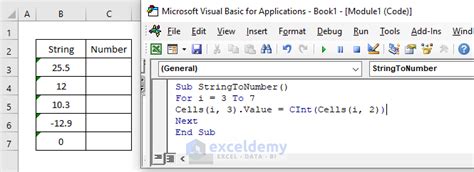
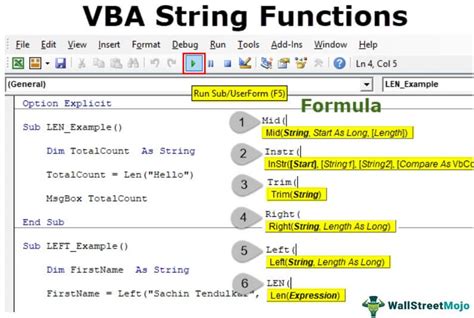
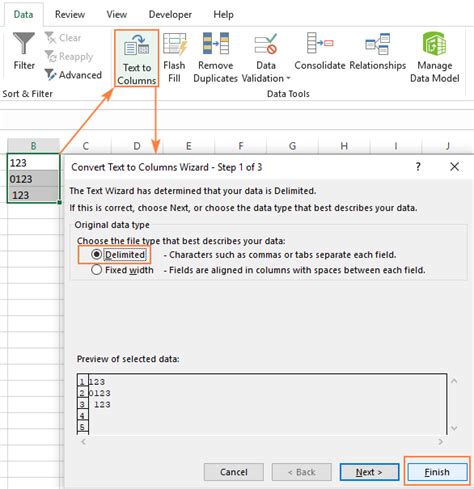
By understanding and applying these methods appropriately, you can ensure that your VBA applications handle numeric data correctly, leading to more accurate calculations and reliable results.