Intro
Copying a range in VBA is a fundamental skill that can be applied to a wide range of tasks, from data manipulation to report generation. Whether you're a beginner or an experienced VBA developer, understanding the different ways to copy a range is essential for efficient and effective coding.
The Microsoft Visual Basic for Applications (VBA) environment provides several methods to copy a range, each with its own strengths and weaknesses. In this article, we'll explore five ways to copy a range in VBA, including their syntax, examples, and best practices.
Method 1: Using the Range.Copy Method
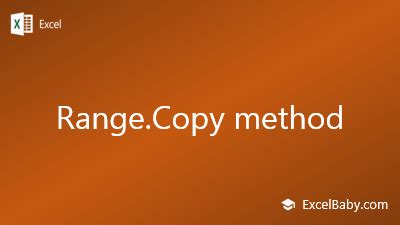
The Range.Copy method is the most straightforward way to copy a range in VBA. This method copies the entire range, including its values, formulas, and formatting.
Syntax:
Range("SourceRange").Copy Destination:=Range("TargetRange")
Example:
Sub CopyRangeUsingCopyMethod()
Range("A1:B2").Copy Destination:=Range("C1:D2")
End Sub
This code copies the range A1:B2 to the range C1:D2.
Method 1.1: Copying a Range to a Variable
You can also copy a range to a variable using the Range.Copy method.
Sub CopyRangeToVariable()
Dim MyRange As Variant
MyRange = Range("A1:B2").Value
Range("C1:D2").Value = MyRange
End Sub
This code copies the values of the range A1:B2 to the variable MyRange
and then assigns those values to the range C1:D2.
Method 2: Using the Range.Value Property
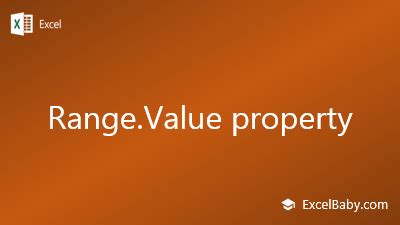
The Range.Value property allows you to copy a range by assigning its values to another range. This method only copies the values, not the formatting or formulas.
Syntax:
Range("TargetRange").Value = Range("SourceRange").Value
Example:
Sub CopyRangeUsingValueProperty()
Range("C1:D2").Value = Range("A1:B2").Value
End Sub
This code copies the values of the range A1:B2 to the range C1:D2.
Method 2.1: Copying a Range to an Array
You can also copy a range to an array using the Range.Value property.
Sub CopyRangeToArray()
Dim MyArray() As Variant
MyArray = Range("A1:B2").Value
Range("C1:D2").Value = MyArray
End Sub
This code copies the values of the range A1:B2 to the array MyArray
and then assigns those values to the range C1:D2.
Method 3: Using the Worksheet.Paste Method
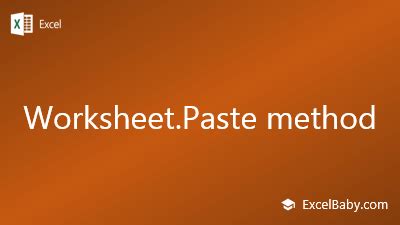
The Worksheet.Paste method copies a range to the clipboard and then pastes it to a target range. This method can be useful when you need to copy a range with formatting and formulas.
Syntax:
Range("SourceRange").Copy
Range("TargetRange").Worksheet.Paste
Application.CutCopyMode = False
Example:
Sub CopyRangeUsingPasteMethod()
Range("A1:B2").Copy
Range("C1:D2").Worksheet.Paste
Application.CutCopyMode = False
End Sub
This code copies the range A1:B2 to the clipboard, pastes it to the range C1:D2, and then clears the clipboard.
Method 4: Using the Range.FormulaArray Property
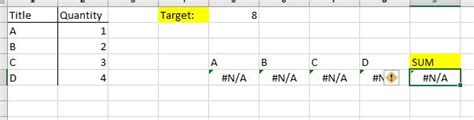
The Range.FormulaArray property allows you to copy a range by assigning its formulas to another range. This method only copies the formulas, not the values or formatting.
Syntax:
Range("TargetRange").FormulaArray = Range("SourceRange").FormulaArray
Example:
Sub CopyRangeUsingFormulaArrayProperty()
Range("C1:D2").FormulaArray = Range("A1:B2").FormulaArray
End Sub
This code copies the formulas of the range A1:B2 to the range C1:D2.
Method 5: Using the Range.AutoFill Method
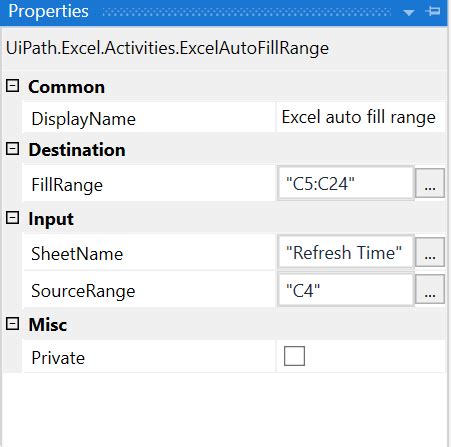
The Range.AutoFill method copies a range by filling a target range with the values, formulas, or formatting of the source range. This method is useful when you need to copy a range with a specific pattern.
Syntax:
Range("SourceRange").AutoFill Destination:=Range("TargetRange")
Example:
Sub CopyRangeUsingAutoFillMethod()
Range("A1:B2").AutoFill Destination:=Range("C1:D2")
End Sub
This code copies the range A1:B2 to the range C1:D2 using the AutoFill method.
Copy a Range in VBA Image Gallery
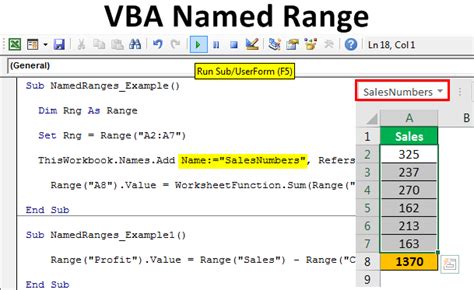
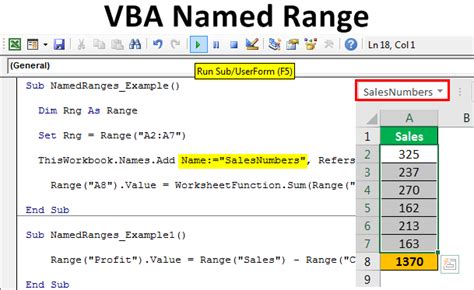
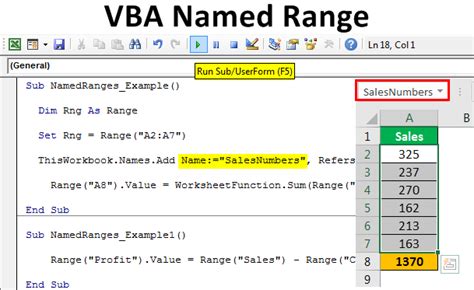
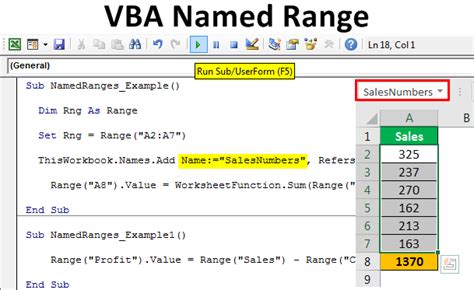
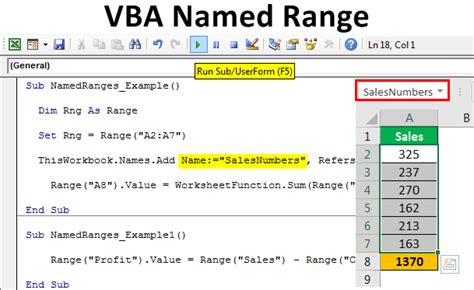
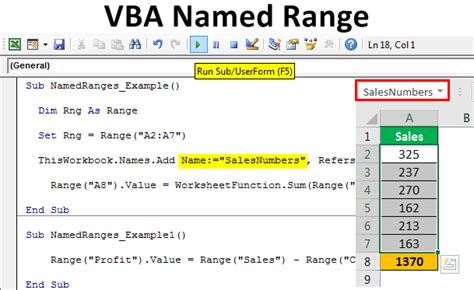
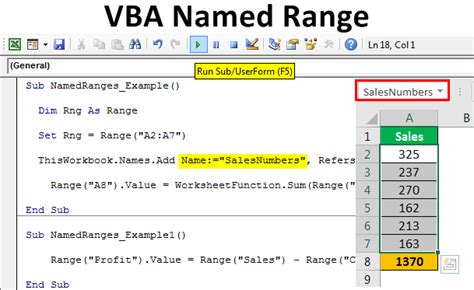
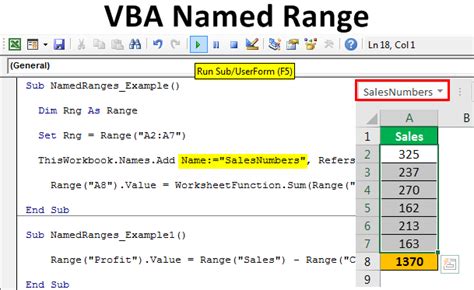
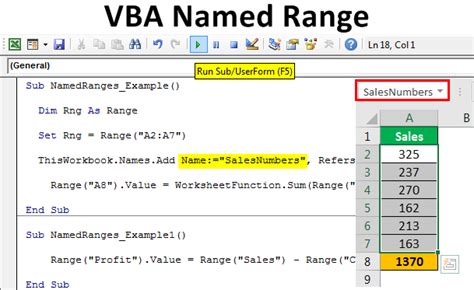
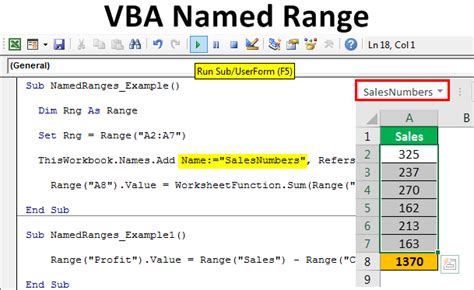
In conclusion, copying a range in VBA can be achieved using various methods, each with its own strengths and weaknesses. The Range.Copy method is the most straightforward way to copy a range, while the Range.Value property and Worksheet.Paste method offer more flexibility. The Range.FormulaArray property and Range.AutoFill method provide advanced features for copying ranges with specific patterns. By mastering these methods, you can become more proficient in VBA and automate tasks with ease.
We hope this article has been informative and helpful in your VBA journey. If you have any questions or need further assistance, please don't hesitate to ask. Share your thoughts and experiences in the comments below, and don't forget to subscribe to our blog for more VBA tutorials and tips!