Intro
Learn how to copy pivot table values with formatting in VBA using 5 efficient methods. Master Excel VBA programming and discover techniques for preserving formatting, including number formatting, conditional formatting, and more. Improve your data analysis and reporting skills with these actionable VBA code examples and best practices.
The pivot table - a powerful tool in Excel that helps us summarize and analyze large datasets. But have you ever tried to copy the values from a pivot table with their formatting intact? It can be a real challenge. Fortunately, VBA (Visual Basic for Applications) is here to save the day. In this article, we'll explore five ways to copy pivot table values with formatting using VBA.
The Importance of Copying Pivot Table Values with Formatting
When working with pivot tables, we often need to copy the values to another worksheet or even another workbook. But simply copying the values can lead to a loss of formatting, which can be frustrating. The formatting of the pivot table values is what makes them easy to read and understand. By copying the values with their formatting intact, we can ensure that the data is presented in a clear and concise manner.
Method 1: Using the PasteSpecial
Method
One way to copy pivot table values with formatting is to use the PasteSpecial
method. This method allows us to paste the values of the pivot table into another range while preserving the formatting.
Sub CopyPivotTableValuesWithFormatting()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTable1")
' Copy the pivot table values with formatting
pt.TableRange2.Copy
Range("A1").PasteSpecial xlPasteValuesAndNumberFormats
Application.CutCopyMode = False
End Sub
In this code, we first set a reference to the pivot table using the ActiveSheet.PivotTables
collection. We then copy the pivot table values using the TableRange2
property, which returns a Range object that represents the entire pivot table. Finally, we paste the values into cell A1 using the PasteSpecial
method, specifying xlPasteValuesAndNumberFormats
to preserve the formatting.
Method 2: Using the Format
Property
Another way to copy pivot table values with formatting is to use the Format
property. This property allows us to apply a specific format to the values of the pivot table.
Sub CopyPivotTableValuesWithFormatting()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTable1")
' Copy the pivot table values with formatting
Dim targetRange As Range
Set targetRange = Range("A1")
pt.TableRange2.Copy
With targetRange
.PasteSpecial xlPasteValues
.NumberFormat = pt.TableRange2.NumberFormat
.Font.Name = pt.TableRange2.Font.Name
.Font.Size = pt.TableRange2.Font.Size
End With
Application.CutCopyMode = False
End Sub
In this code, we first copy the pivot table values using the TableRange2
property. We then set a reference to the target range using the Range
object. We apply the formatting of the pivot table values to the target range using the NumberFormat
, Font.Name
, and Font.Size
properties.
Method 3: Using the Paste
Method with xlPasteAll
We can also use the Paste
method with the xlPasteAll
argument to copy the pivot table values with formatting.
Sub CopyPivotTableValuesWithFormatting()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTable1")
' Copy the pivot table values with formatting
pt.TableRange2.Copy
Range("A1").Paste xlPasteAll
Application.CutCopyMode = False
End Sub
In this code, we copy the pivot table values using the TableRange2
property and then paste them into cell A1 using the Paste
method with the xlPasteAll
argument.
Method 4: Using the CreatePivotCache
Method
We can also use the CreatePivotCache
method to create a new pivot cache and then copy the pivot table values with formatting.
Sub CopyPivotTableValuesWithFormatting()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTable1")
' Create a new pivot cache
Dim pc As PivotCache
Set pc = ActiveWorkbook.PivotCaches.Create(pt.TableRange2)
' Copy the pivot table values with formatting
Dim targetRange As Range
Set targetRange = Range("A1")
pc.CreatePivotTable TableDestination:=targetRange, TableName:="PivotTable2"
Application.CutCopyMode = False
End Sub
In this code, we create a new pivot cache using the CreatePivotCache
method and then create a new pivot table using the CreatePivotTable
method. We can then copy the pivot table values with formatting.
Method 5: Using the Copy
Method with xlCopyFormats
Finally, we can use the Copy
method with the xlCopyFormats
argument to copy the pivot table values with formatting.
Sub CopyPivotTableValuesWithFormatting()
Dim pt As PivotTable
Set pt = ActiveSheet.PivotTables("PivotTable1")
' Copy the pivot table values with formatting
pt.TableRange2.Copy xlCopyFormats
Range("A1").PasteSpecial xlPasteValues
Application.CutCopyMode = False
End Sub
In this code, we copy the pivot table values using the TableRange2
property and then paste them into cell A1 using the PasteSpecial
method with the xlPasteValues
argument.
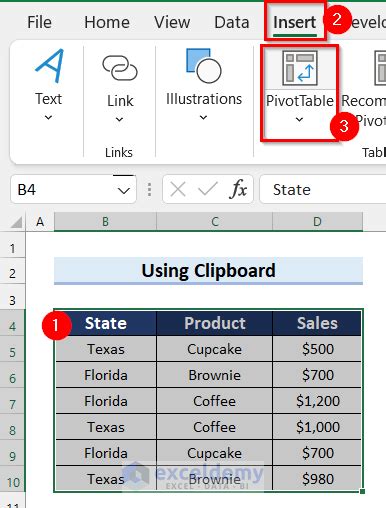
Gallery of Pivot Table Values with Formatting
Pivot Table Values with Formatting Image Gallery
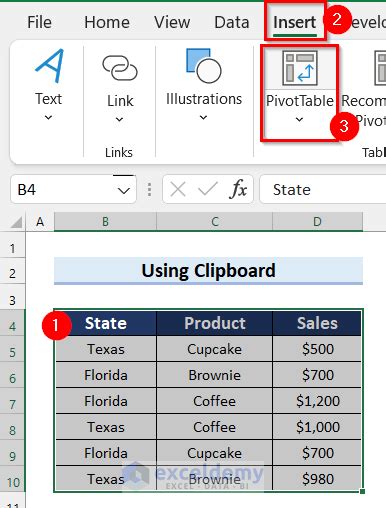
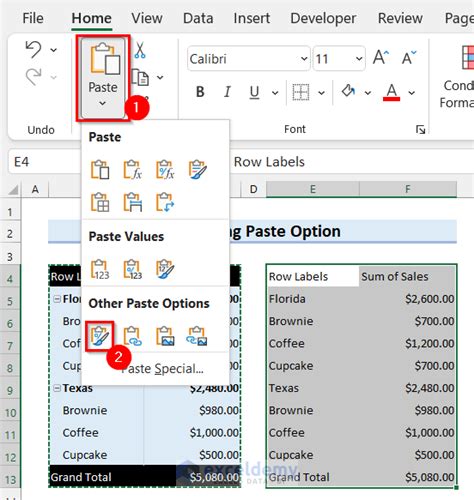
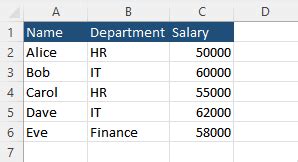
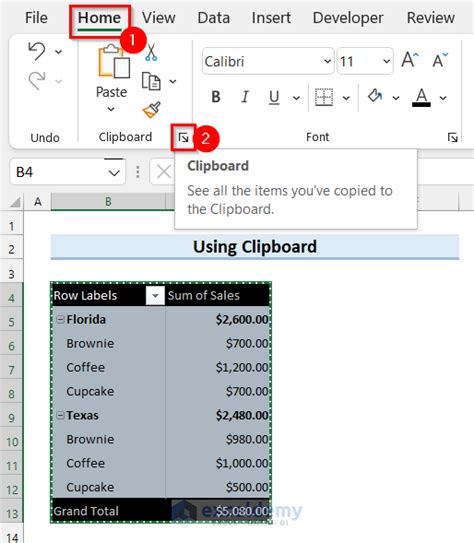
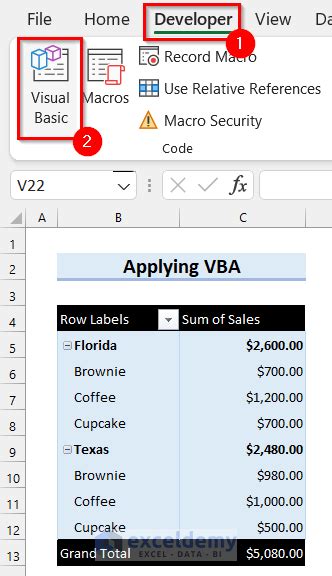
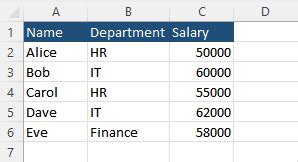
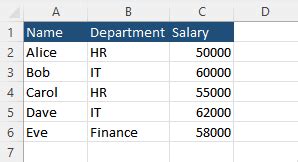
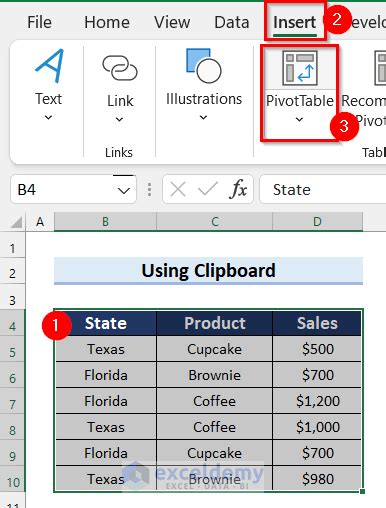
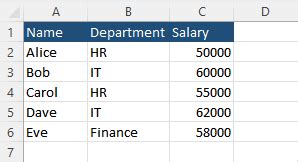
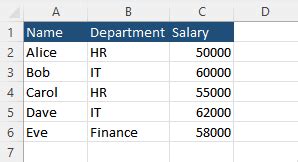
We hope this article has been helpful in providing you with five ways to copy pivot table values with formatting using VBA. Whether you're a seasoned developer or just starting out, these methods will help you to efficiently copy pivot table values with their formatting intact. So go ahead, give them a try, and see how they can help you in your Excel development journey!