Intro
Learn how to copy an Excel sheet to a new workbook using VBA. Discover the step-by-step process of duplicating worksheets, transferring data, and formatting using VBA macros. Master the art of Excel automation and increase productivity with this tutorial on VBA sheet copying, Excel worksheet duplication, and workbook manipulation.
Excel is a powerful tool for data analysis and manipulation, and sometimes, we need to copy an entire sheet to a new workbook to work on a separate project or to share data with others. While this can be done manually, using VBA (Visual Basic for Applications) can automate the process, making it faster and more efficient. In this article, we will explore how to copy an Excel sheet to a new workbook using VBA.
Why Use VBA to Copy an Excel Sheet?
There are several reasons why you might want to use VBA to copy an Excel sheet:
- Automation: VBA allows you to automate repetitive tasks, such as copying a sheet to a new workbook, with just a few lines of code.
- Speed: VBA can perform tasks much faster than manual methods, especially when working with large datasets.
- Accuracy: VBA reduces the risk of human error, ensuring that the copy is accurate and complete.
How to Copy an Excel Sheet to a New Workbook using VBA
To copy an Excel sheet to a new workbook using VBA, follow these steps:
Step 1: Open the Visual Basic Editor
To access the Visual Basic Editor, press Alt + F11
or navigate to Developer
> Visual Basic
in the ribbon.
Step 2: Insert a New Module
In the Visual Basic Editor, click Insert
> Module
to insert a new module.
Step 3: Write the VBA Code
In the new module, paste the following code:
Sub CopySheetToNewWorkbook()
Dim ws As Worksheet
Dim newWB As Workbook
' Set the worksheet to copy
Set ws = ThisWorkbook.Sheets("YourSheetName")
' Create a new workbook
Set newWB = Workbooks.Add
' Copy the worksheet to the new workbook
ws.Copy Before:=newWB.Sheets(1)
' Save the new workbook
newWB.SaveAs "NewWorkbook.xlsx"
' Clean up
Set ws = Nothing
Set newWB = Nothing
End Sub
Explanation of the Code
Sub CopySheetToNewWorkbook()
: This line defines a new subroutine calledCopySheetToNewWorkbook
.Dim ws As Worksheet
andDim newWB As Workbook
: These lines declare two variables,ws
andnewWB
, to hold references to the worksheet and new workbook, respectively.Set ws = ThisWorkbook.Sheets("YourSheetName")
: This line sets thews
variable to the worksheet you want to copy. Replace "YourSheetName" with the actual name of the worksheet.Set newWB = Workbooks.Add
: This line creates a new workbook and sets thenewWB
variable to it.ws.Copy Before:=newWB.Sheets(1)
: This line copies the worksheet to the new workbook, placing it before the first sheet in the new workbook.newWB.SaveAs "NewWorkbook.xlsx"
: This line saves the new workbook with the file name "NewWorkbook.xlsx".Set ws = Nothing
andSet newWB = Nothing
: These lines release the memory used by thews
andnewWB
variables.
Step 4: Run the VBA Code
To run the VBA code, click Run
> Run Sub/UserForm
or press F5
.
Step 5: Verify the Results
After running the code, verify that the worksheet has been copied to a new workbook.
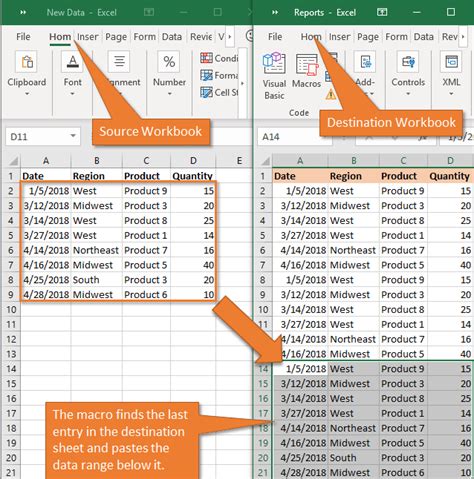
Tips and Variations
- Copy Multiple Sheets: To copy multiple sheets, simply repeat the
ws.Copy
line for each sheet you want to copy. - Copy to a Specific Location: To copy the worksheet to a specific location in the new workbook, use the
Before
parameter of theCopy
method. For example, to copy the worksheet to the second sheet in the new workbook, usews.Copy Before:=newWB.Sheets(2)
. - Use a Different File Name: To save the new workbook with a different file name, simply change the file name in the
SaveAs
method. For example, to save the new workbook as "MyNewWorkbook.xlsx", usenewWB.SaveAs "MyNewWorkbook.xlsx"
.
Gallery of Excel VBA Examples
Excel VBA Examples
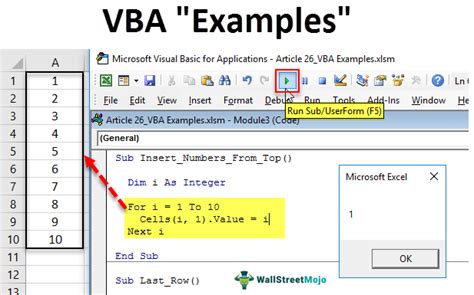
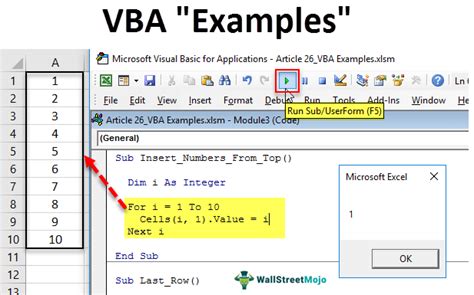
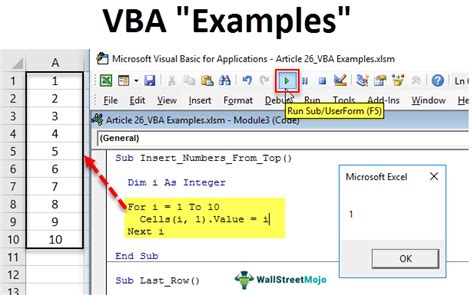
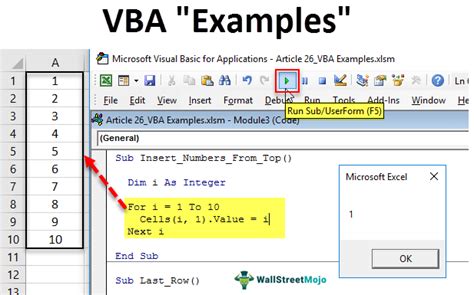
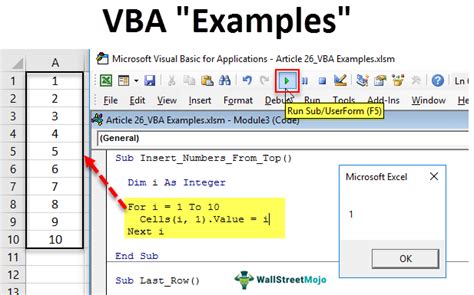
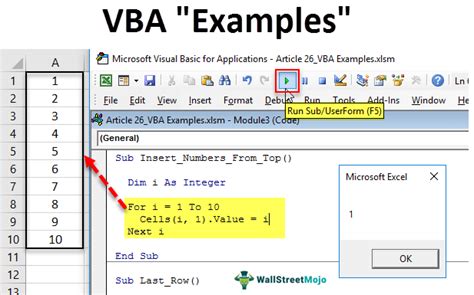
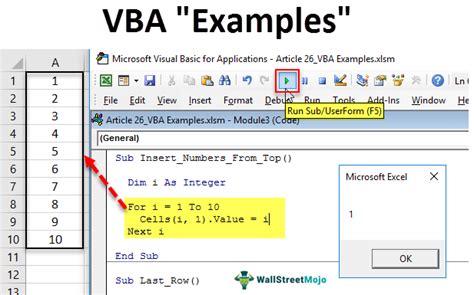
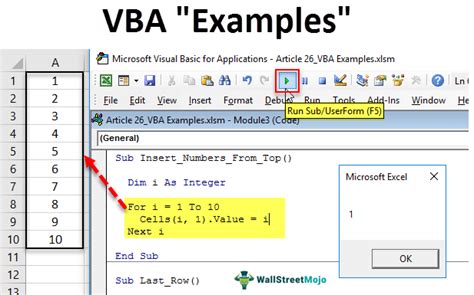
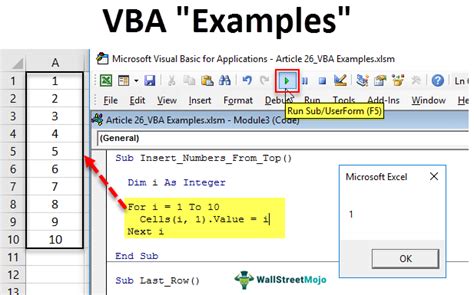
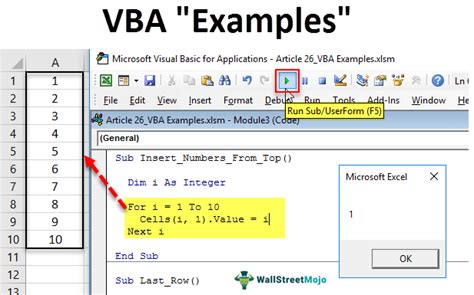
Conclusion
In this article, we have explored how to copy an Excel sheet to a new workbook using VBA. By following the steps outlined above, you can automate this task and make your workflow more efficient. Whether you are a beginner or an experienced VBA user, this tutorial should provide you with a solid foundation for copying Excel sheets to new workbooks using VBA.
Share Your Thoughts
Have you used VBA to copy Excel sheets to new workbooks? Share your experiences and tips in the comments below!