Intro
Boost productivity with VBA! Learn how to copy worksheets to new workbooks with ease using simple yet powerful VBA code. Discover how to automate tasks, streamline workflows, and increase efficiency. Master Excel VBA and take your spreadsheet skills to the next level with our easy-to-follow guide.
Working with multiple workbooks and worksheets in Excel can be a challenging task, especially when you need to copy data from one workbook to another. Fortunately, VBA (Visual Basic for Applications) provides a simple and efficient way to accomplish this task. In this article, we will explore how to copy a worksheet to a new workbook using VBA.
Why Use VBA to Copy Worksheets?
Before we dive into the code, let's discuss why you might want to use VBA to copy worksheets. Here are a few reasons:
- Efficiency: VBA allows you to automate repetitive tasks, such as copying worksheets, with just a few lines of code.
- Accuracy: By using VBA, you can ensure that the copy process is accurate and consistent, reducing the risk of human error.
- Flexibility: VBA provides a wide range of options for customizing the copy process, such as specifying the worksheet to copy, the new workbook name, and more.
Basic VBA Code to Copy Worksheet
To get started, let's take a look at a basic VBA code snippet that copies a worksheet to a new workbook:
Sub CopyWorksheetToNewWorkbook()
Dim sourceWorkbook As Workbook
Dim sourceWorksheet As Worksheet
Dim newWorkbook As Workbook
Set sourceWorkbook = ThisWorkbook
Set sourceWorksheet = sourceWorkbook.Sheets("Sheet1")
' Create a new workbook
Set newWorkbook = Workbooks.Add
' Copy the worksheet to the new workbook
sourceWorksheet.Copy Before:=newWorkbook.Sheets(1)
' Save the new workbook
newWorkbook.SaveAs "NewWorkbook.xlsx"
End Sub
This code snippet assumes that you have a worksheet named "Sheet1" in the active workbook, and you want to copy it to a new workbook named "NewWorkbook.xlsx".
How the Code Works
Here's a step-by-step explanation of how the code works:
- Declare variables: We declare three variables:
sourceWorkbook
,sourceWorksheet
, andnewWorkbook
. These variables will hold references to the source workbook, source worksheet, and new workbook, respectively. - Set source workbook and worksheet: We set the
sourceWorkbook
variable to the active workbook usingThisWorkbook
, and set thesourceWorksheet
variable to the worksheet named "Sheet1" usingsourceWorkbook.Sheets("Sheet1")
. - Create a new workbook: We create a new workbook using
Workbooks.Add
and set thenewWorkbook
variable to the new workbook. - Copy the worksheet: We copy the source worksheet to the new workbook using
sourceWorksheet.Copy Before:=newWorkbook.Sheets(1)
. This line of code copies the entire worksheet, including all data, formulas, and formatting. - Save the new workbook: Finally, we save the new workbook using
newWorkbook.SaveAs "NewWorkbook.xlsx"
.
Customizing the Code
The basic code snippet above provides a good starting point, but you may want to customize it to suit your specific needs. Here are a few examples:
- Specify a different worksheet: To copy a different worksheet, simply change the
sourceWorksheet
variable to the desired worksheet name. - Use a different new workbook name: To use a different new workbook name, change the
SaveAs
method to specify the desired file name. - Copy multiple worksheets: To copy multiple worksheets, simply loop through the worksheets collection and repeat the copy process for each worksheet.
Common Errors and Troubleshooting
When working with VBA code, it's not uncommon to encounter errors. Here are a few common errors you may encounter when copying worksheets:
- Runtime error 9: This error occurs when the specified worksheet does not exist in the source workbook. Make sure to verify the worksheet name and spelling.
- Runtime error 1004: This error occurs when the new workbook cannot be saved due to a file name conflict or permission issue. Try changing the file name or saving the workbook to a different location.
Conclusion
In conclusion, copying a worksheet to a new workbook using VBA is a simple and efficient process. By using the basic code snippet above and customizing it to suit your specific needs, you can automate this task and reduce the risk of human error.
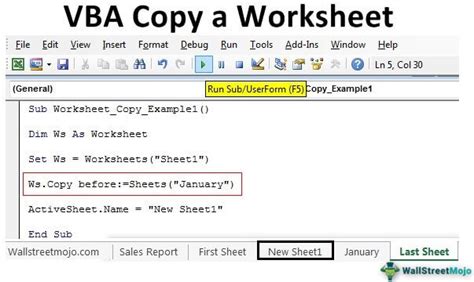
We hope this article has been helpful in showing you how to copy a worksheet to a new workbook using VBA. Do you have any questions or need further assistance? Please leave a comment below.
Gallery of VBA Code Examples
VBA Code Examples Gallery
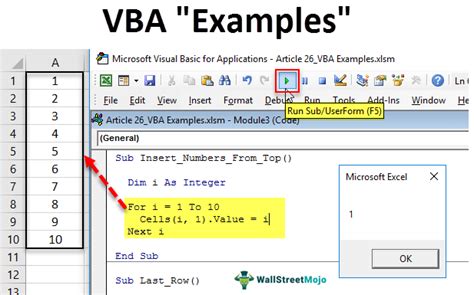
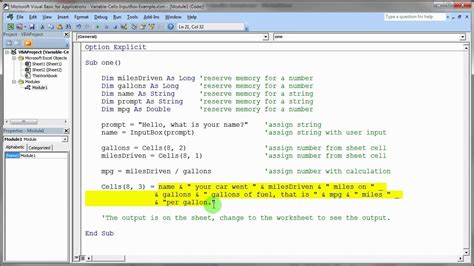
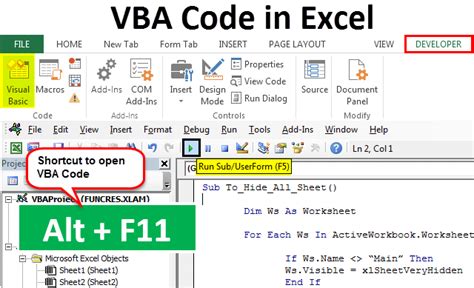
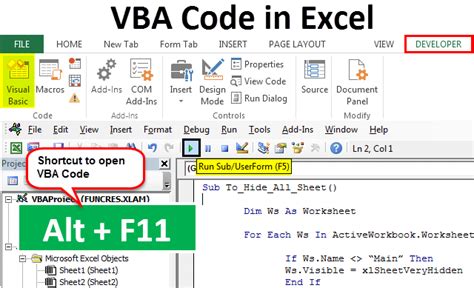
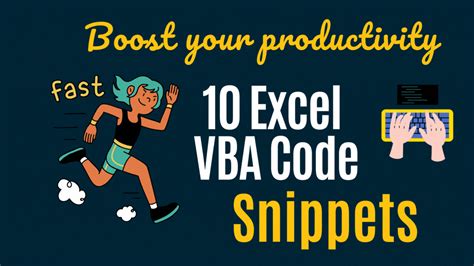
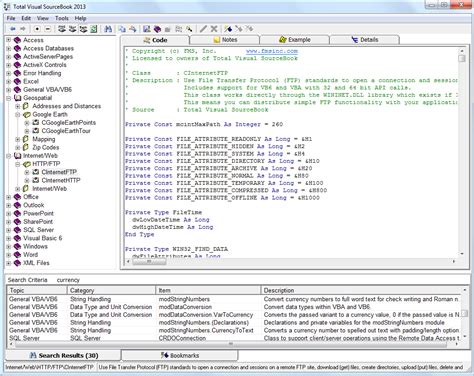
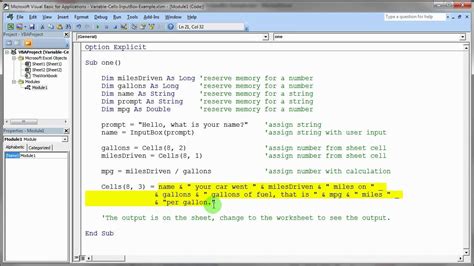
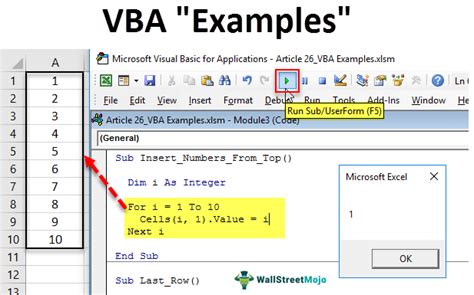
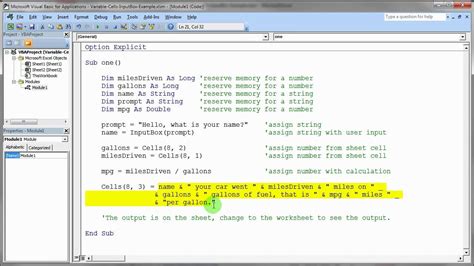
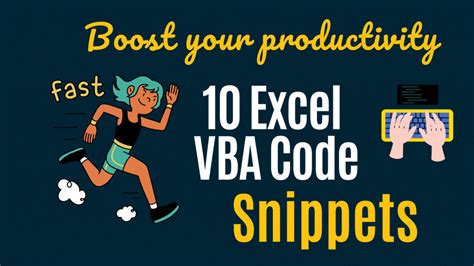