Intro
Unlock the power of VBA workbooks with our expert guide. Discover 5 simple ways to create a new workbook in VBA, including using the Workbooks.Add method, copying existing workbooks, and more. Master VBA workbook creation and boost your productivity with our step-by-step tutorials and code examples.
Creating a new workbook in VBA (Visual Basic for Applications) is a fundamental task that can be accomplished in several ways, depending on your specific needs and the context of your project. Workbooks are the core component of Excel, serving as containers for worksheets that hold your data. Here are five distinct methods to create a new workbook in VBA, each with its unique application and benefits.
Method 1: Using the Workbooks.Add Method
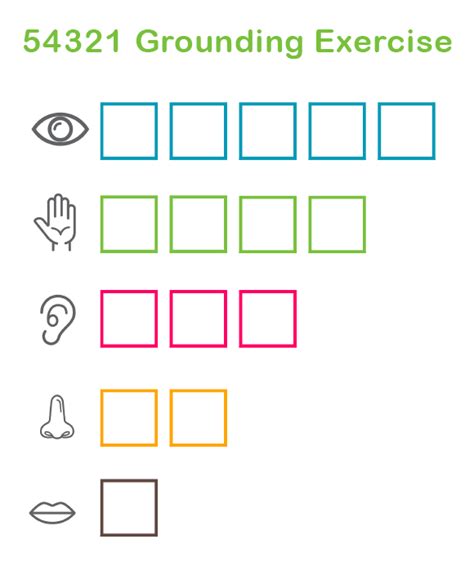
The Workbooks.Add
method is the most straightforward way to create a new workbook in VBA. This method adds a new workbook to the workbooks collection and returns a Workbook object. The new workbook becomes the active workbook.
Sub CreateNewWorkbook()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
' Further operations on the new workbook can be performed here
End Sub
Benefits and Considerations
- Ease of Use: This method is simple and direct, requiring minimal code.
- Flexibility: After creating the workbook, you can manipulate it as needed (e.g., adding worksheets, entering data).
- Limitations: It always creates a workbook with the default template and settings.
Method 2: Creating a Workbook from a Template
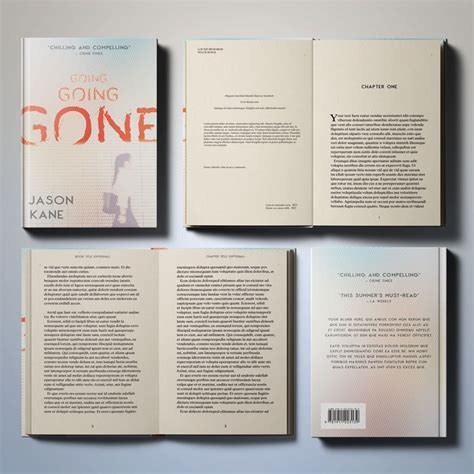
If you frequently create workbooks for specific tasks or formats, using a template can save time and ensure consistency. VBA allows you to create a new workbook based on an existing template.
Sub CreateWorkbookFromTemplate()
Dim templatePath As String
templatePath = "C:\Path\To\Your\Template.xltm" ' Update this path
Workbooks.Add templatePath
End Sub
Benefits and Considerations
- Customization: Templates can be tailored to your needs, including pre-formatted worksheets and predefined formulas.
- Efficiency: Reduces the time spent setting up new workbooks.
- Availability: Requires access to the template file.
Method 3: Copying an Existing Workbook
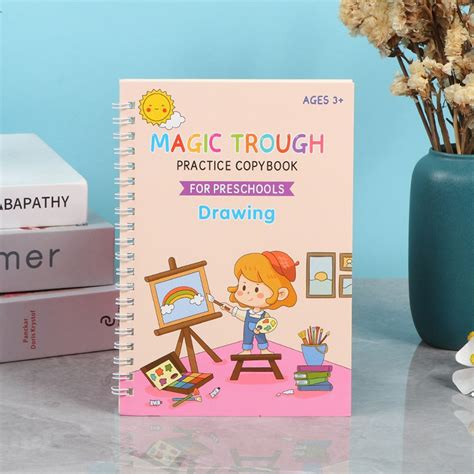
Sometimes, you might want to create a new workbook that is identical to an existing one, perhaps for testing or archival purposes. VBA can copy an entire workbook.
Sub CopyExistingWorkbook()
Dim originalWorkbook As Workbook
Set originalWorkbook = Workbooks("OriginalWorkbook.xlsx") ' Update the workbook name
originalWorkbook.Copy
End Sub
Benefits and Considerations
- Accuracy: Ensures the new workbook is an exact copy, including all worksheets and data.
- Convenience: Quick method for duplicating complex workbooks.
- Size: The new workbook will be as large as the original, which might be a consideration for storage or performance.
Method 4: Using Late Binding
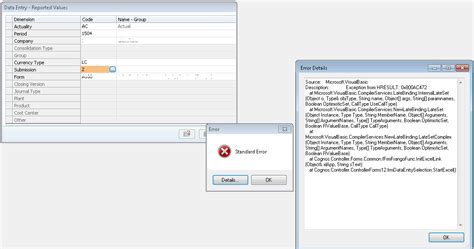
Late binding involves creating an object variable without setting its type until runtime. This approach can be useful when the Excel object library is not referenced in your project, or for compatibility reasons.
Sub CreateWorkbookLateBinding()
Dim excelApp As Object
Dim newWorkbook As Object
Set excelApp = CreateObject("Excel.Application")
excelApp.Visible = True
Set newWorkbook = excelApp.Workbooks.Add
End Sub
Benefits and Considerations
- Flexibility: Works without requiring a reference to the Excel library.
- Compatibility: Can be more compatible with different Excel versions or systems.
- Performance: May be slower due to the late binding mechanism.
Method 5: Automating Workbook Creation through UserForms
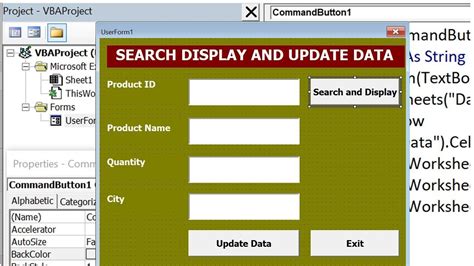
For more complex or user-driven scenarios, you can create a UserForm that prompts users for the necessary details and then automates the workbook creation based on their input.
Sub CreateWorkbookFromUserForm()
' Assume a UserForm named "frmNewWorkbook" with necessary controls
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
' Populate the workbook based on UserForm input
End Sub
Benefits and Considerations
- Customization: Allows for tailored workbook creation based on user input.
- User Experience: Provides a friendly interface for non-technical users.
- Complexity: Requires more code and form design effort.
Workbook Creation Gallery
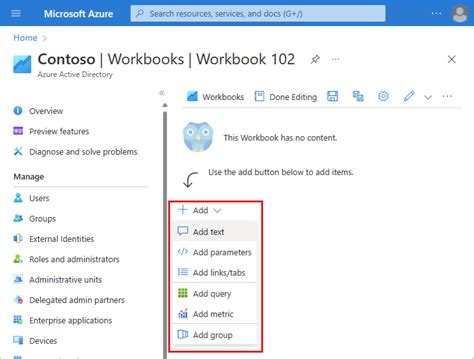
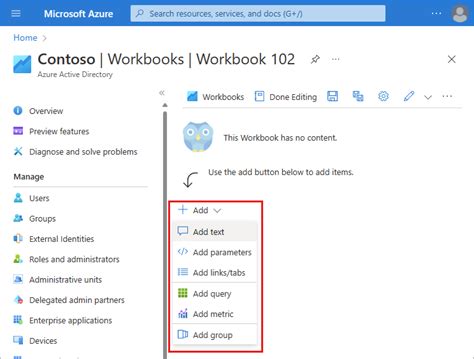
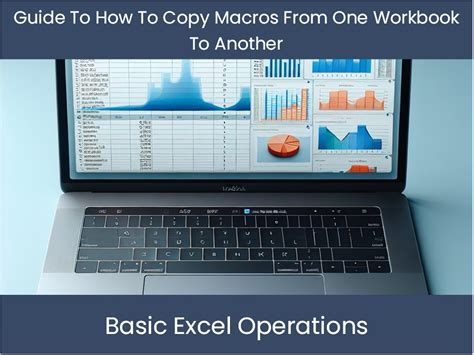
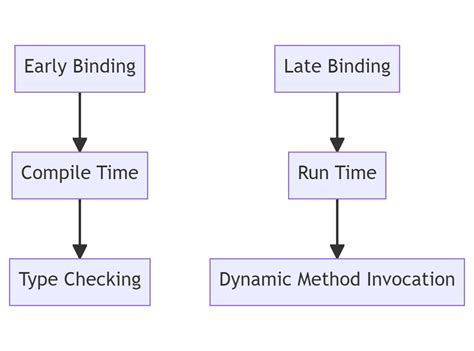
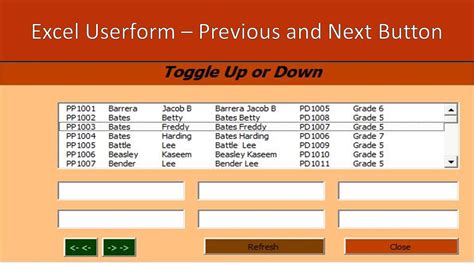
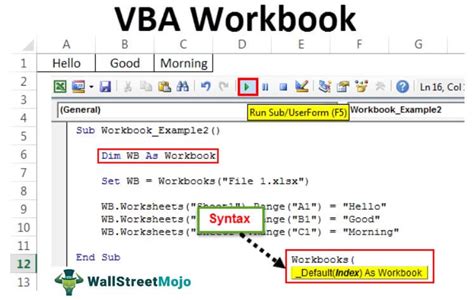
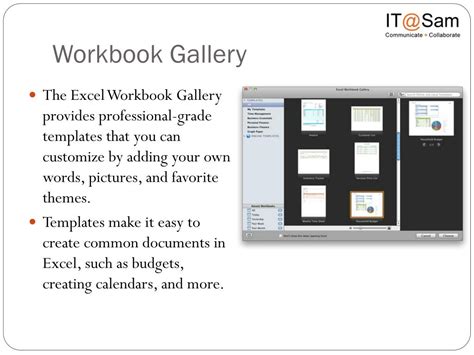
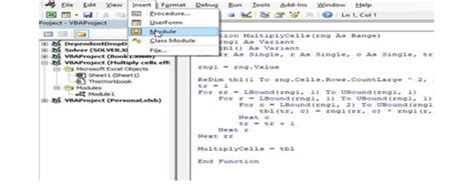
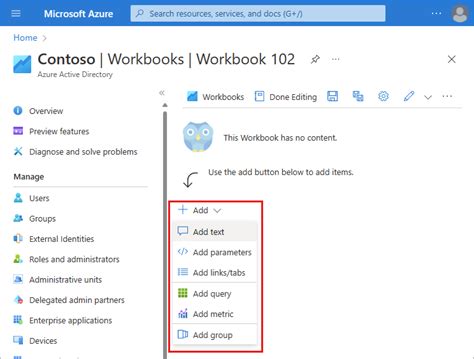
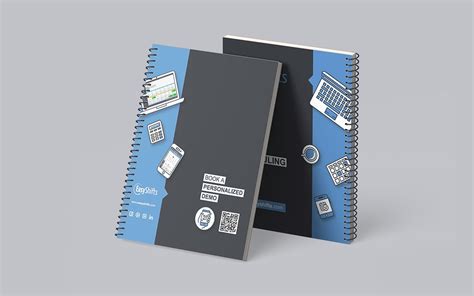
Creating a new workbook in VBA is a versatile process that can be tailored to various scenarios and needs. Whether you're looking for simplicity, customization, or automation, there's a method that suits your project requirements. By mastering these techniques, you can efficiently manage and create workbooks within your VBA applications, enhancing productivity and user experience.