Intro
Unlock the power of data analysis with VBA pivot tables. Learn 5 efficient methods to create pivot tables with VBA, including dynamic range selection, data manipulation, and pivot cache optimization. Master pivot table automation, data summarization, and Excel dashboard creation with these expert VBA techniques.
Mastering pivot tables in Excel is a powerful skill for data analysis, and automating their creation with VBA (Visual Basic for Applications) can save you a tremendous amount of time and effort. Pivot tables are a versatile tool that allows you to summarize and analyze large datasets in a flexible and interactive way. By combining the functionality of pivot tables with the automation capabilities of VBA, you can create reports, summaries, and data visualizations more efficiently than ever before.
Pivot tables are particularly useful because they enable you to look at data from different angles, easily rotating and reorganizing the data to ask different questions. This flexibility makes pivot tables a key tool for anyone working with data, from business analysts and financial forecasters to marketers and researchers. By automating the creation of pivot tables with VBA, you can perform repetitive tasks quickly, ensure consistency across reports, and focus on interpreting and acting on your data insights.
Here, we'll explore five ways to create pivot tables with VBA, covering both the basics and some advanced techniques. Whether you're new to VBA or looking to enhance your skills, this guide will help you automate the creation of pivot tables to streamline your data analysis workflow.
Understanding Pivot Tables
Before diving into VBA, it's essential to have a solid understanding of pivot tables themselves. A pivot table is a data summarization tool that helps you extract and analyze data patterns. It's called a "pivot" table because you can rotate (or pivot) the data to look at it from different angles.
Creating Pivot Tables Manually
Before automating with VBA, let's briefly touch on how to create a pivot table manually:
- Select a cell where you want your pivot table to appear.
- Go to the "Insert" tab in the ribbon.
- Click on "PivotTable."
- Choose a table or range as your data source.
- Click "OK" to create the pivot table.
Manual creation is straightforward, but repetitive and time-consuming, especially when dealing with multiple datasets or when the same type of pivot table needs to be created regularly.
5 Ways to Create Pivot Tables with VBA
1. Basic Pivot Table Creation
Sub CreatePivotTableBasic()
Dim ws As Worksheet
Dim pc As PivotCache
Dim pt As PivotTable
' Set the worksheet where the pivot table will be created
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Create a new PivotCache object
Set pc = ThisWorkbook.PivotCaches.Create(SourceType:=xlDatabase, SourceData:=ws.Range("A1").CurrentRegion)
' Create a new PivotTable object
Set pt = pc.CreatePivotTable(TableDestination:=ws.Range("E1"), TableName:="SalesPivot")
' Add fields to the pivot table
pt.PivotFields("Region").Orientation = xlRowField
pt.PivotFields("Product").Orientation = xlColumnField
pt.AddDataField pt.PivotFields("Sales"), "Sum of Sales", xlSum
' Refresh the pivot table
pt.RefreshTable
End Sub
This script demonstrates the basic steps of creating a pivot table with VBA: setting up the environment, creating a PivotCache
object to define the data source, generating the pivot table itself, and then adding fields to it.
2. Advanced Pivot Table with Custom Layout
Sub CreatePivotTableAdvanced()
Dim ws As Worksheet
Dim pc As PivotCache
Dim pt As PivotTable
Dim pf As PivotField
' Set the worksheet where the pivot table will be created
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Create a new PivotCache object
Set pc = ThisWorkbook.PivotCaches.Create(SourceType:=xlDatabase, SourceData:=ws.Range("A1").CurrentRegion)
' Create a new PivotTable object with a custom layout
Set pt = pc.CreatePivotTable(TableDestination:=ws.Range("E1"), TableName:="AdvancedPivot", TableStyle:= "PivotStyleMedium9")
' Add fields to the pivot table
Set pf = pt.PivotFields("Date")
pf.Orientation = xlPageField
pt.AddDataField pt.PivotFields("Sales"), "Sum of Sales", xlSum
' Customize the layout
pt.PivotFields("Region").PivotItems("North").Visible = False
pt.DisplayFieldCaptions = False
pt.TableStyle2 = "PivotStyleMedium10"
' Refresh the pivot table
pt.RefreshTable
End Sub
This example shows how to create a pivot table with a custom layout, including setting the table style and hiding specific pivot items. It also demonstrates how to add a data field with a custom name.
3. Creating Pivot Tables Dynamically Based on Worksheet Data
Sub CreatePivotTableDynamic()
Dim ws As Worksheet
Dim pc As PivotCache
Dim pt As PivotTable
Dim lastRow As Long
Dim lastCol As Long
' Set the worksheet where the pivot table will be created
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Find the last row and column with data
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
lastCol = ws.Cells(1, ws.Columns.Count).End(xlToLeft).Column
' Create a new PivotCache object
Set pc = ThisWorkbook.PivotCaches.Create(SourceType:=xlDatabase, SourceData:=ws.Range(ws.Cells(1, 1), ws.Cells(lastRow, lastCol)))
' Create a new PivotTable object
Set pt = pc.CreatePivotTable(TableDestination:=ws.Range("E1"), TableName:="DynamicPivot")
' Add fields to the pivot table dynamically
For Each pf In pt.PivotFields
If pf.Name <> "Date" Then
pf.Orientation = xlColumnField
Else
pf.Orientation = xlPageField
End If
Next pf
' Refresh the pivot table
pt.RefreshTable
End Sub
This script dynamically creates a pivot table based on the data in the worksheet, automatically including all fields in the dataset.
4. Using External Data Sources for Pivot Tables
Sub CreatePivotTableExternalData()
Dim ws As Worksheet
Dim pc As PivotCache
Dim pt As PivotTable
Dim CN As ADODB.Connection
Dim RS As ADODB.Recordset
' Set the worksheet where the pivot table will be created
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Set up the external data source (SQL Server in this example)
Set CN = New ADODB.Connection
CN.Open "DRIVER={ODBC Driver 17 for SQL Server};" & _
"SERVER=YourServerName;" & _
"DATABASE=YourDatabaseName;" & _
"UID=YourUsername;" & _
"PWD=YourPassword"
' Execute a query to get the data
Set RS = New ADODB.Recordset
RS.Open "SELECT * FROM YourTableName", CN
' Create a new PivotCache object
Set pc = ThisWorkbook.PivotCaches.Create(SourceType:=xlExternal, SourceData:=RS)
' Create a new PivotTable object
Set pt = pc.CreatePivotTable(TableDestination:=ws.Range("E1"), TableName:="ExternalDataPivot")
' Add fields to the pivot table
pt.PivotFields("Region").Orientation = xlRowField
pt.PivotFields("Product").Orientation = xlColumnField
pt.AddDataField pt.PivotFields("Sales"), "Sum of Sales", xlSum
' Refresh the pivot table
pt.RefreshTable
' Clean up
RS.Close
CN.Close
Set RS = Nothing
Set CN = Nothing
End Sub
This example shows how to create a pivot table using an external data source (a SQL Server database in this case), demonstrating the flexibility of VBA in accessing and manipulating external data.
5. Scheduling Pivot Table Updates
Sub SchedulePivotTableUpdate()
Dim ws As Worksheet
Dim pt As PivotTable
' Set the worksheet where the pivot table is located
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Set the pivot table object
Set pt = ws.PivotTables("YourPivotTableName")
' Schedule the update (in this case, every hour)
Application.OnTime TimeValue("00:00"), "UpdatePivotTable"
' Function to update the pivot table
Sub UpdatePivotTable()
pt.RefreshTable
End Sub
End Sub
This script sets up a schedule to automatically update a pivot table at regular intervals, ensuring that the data remains current and up-to-date.
Gallery of Pivot Table Creation with VBA
Pivot Table Creation with VBA Image Gallery
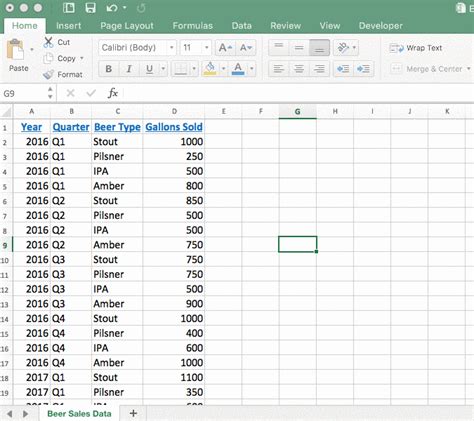
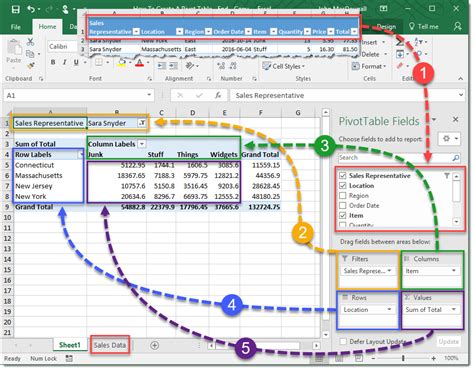
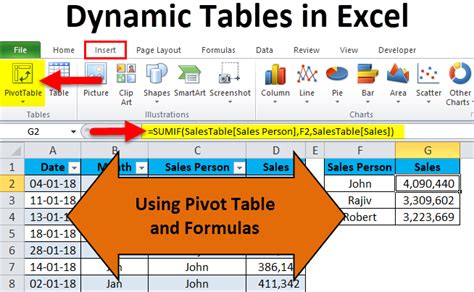
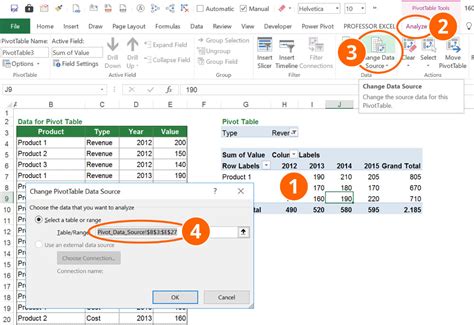
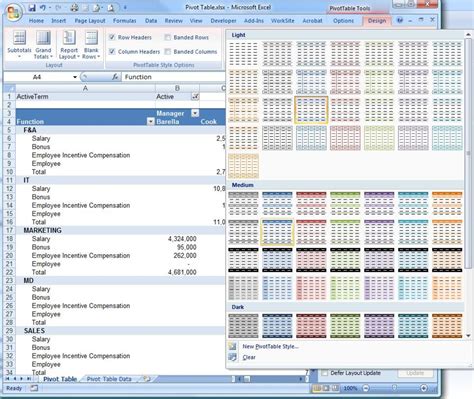
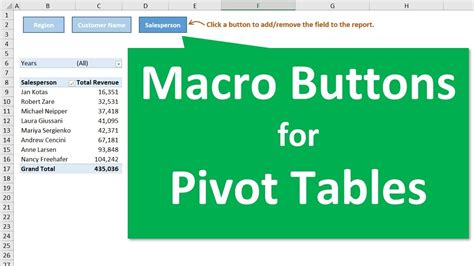
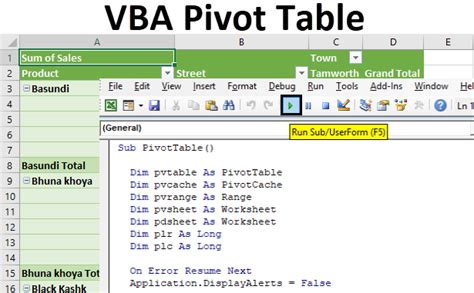
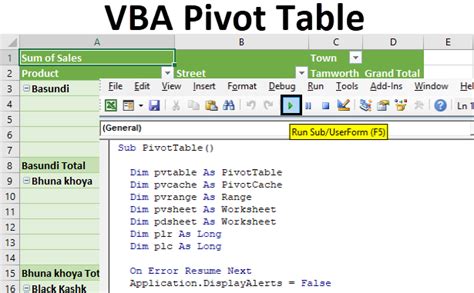
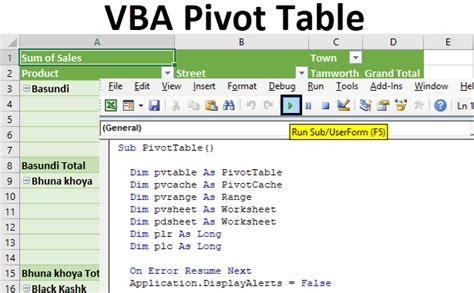
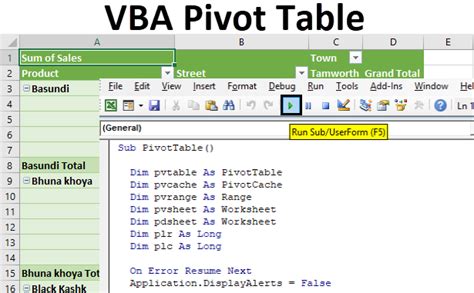
Final Thoughts
Automating the creation of pivot tables with VBA can significantly enhance your data analysis workflow, saving time and reducing the risk of human error. By mastering the techniques outlined in this article, you can unlock the full potential of pivot tables, making complex data insights more accessible than ever before.
Whether you're working with small datasets or handling large-scale data analytics projects, the ability to automate pivot table creation with VBA can be a game-changer. So, take the first step today and start automating your pivot table creation with VBA!
Share Your Thoughts!
Have you ever used VBA to create pivot tables? What challenges did you face, and how did you overcome them? Share your experiences and tips in the comments below. Let's learn from each other and take our data analysis skills to the next level!