Intro
Activating a specific sheet in Excel using VBA is a common task that can be accomplished with a few lines of code. In this article, we will explore the different ways to activate a sheet using VBA, including using the sheet's name, index, or codename.
Why Activate a Sheet?
Activating a sheet is necessary when you want to perform actions on a specific sheet, such as selecting cells, formatting data, or running formulas. By default, VBA will perform actions on the active sheet, which may not be the sheet you intend to work with. By activating the correct sheet, you can ensure that your code runs on the intended sheet.
Using the Sheet's Name
One way to activate a sheet is by using its name. You can use the Worksheets
collection to access a sheet by its name.
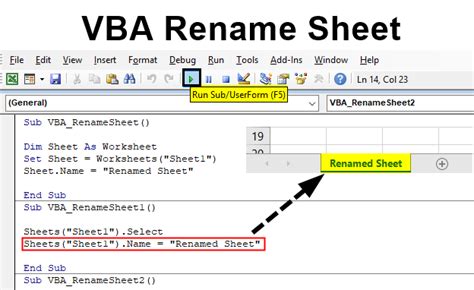
Sub ActivateSheetByName()
Worksheets("Sheet1").Activate
End Sub
In this example, the Worksheets
collection is used to access the sheet named "Sheet1". The Activate
method is then used to activate the sheet.
Using the Sheet's Index
Another way to activate a sheet is by using its index. The index of a sheet refers to its position in the workbook. The first sheet in the workbook has an index of 1, the second sheet has an index of 2, and so on.
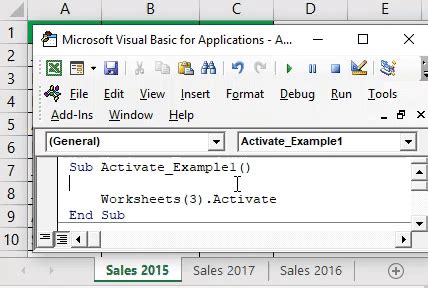
Sub ActivateSheetByIndex()
Worksheets(1).Activate
End Sub
In this example, the Worksheets
collection is used to access the first sheet in the workbook (index 1). The Activate
method is then used to activate the sheet.
Using the Sheet's Codename
A sheet's codename is a unique identifier that is assigned to the sheet when it is created. The codename is used internally by Excel and is not visible to the user.
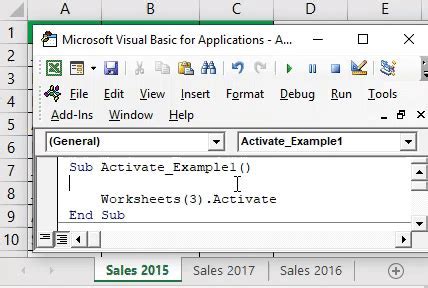
Sub ActivateSheetByCodename()
Sheet1.Activate
End Sub
In this example, the codename "Sheet1" is used to access the sheet. The Activate
method is then used to activate the sheet.
Error Handling
When activating a sheet, it's a good idea to include error handling to ensure that the code runs smoothly. For example, you can use the On Error
statement to handle errors that may occur when trying to activate a sheet.
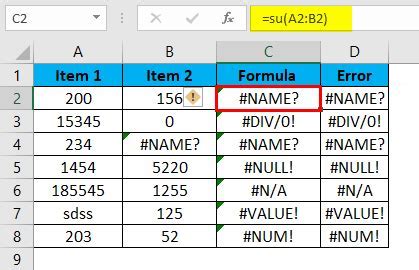
Sub ActivateSheetWithErrorHandling()
On Error GoTo ErrorHandler
Worksheets("Sheet1").Activate
Exit Sub
ErrorHandler:
MsgBox "Error activating sheet: " & Err.Description
End Sub
In this example, the On Error
statement is used to handle errors that may occur when trying to activate the sheet. If an error occurs, the code will jump to the ErrorHandler
label and display an error message.
Gallery of Activate Sheet
Activate Sheet Image Gallery
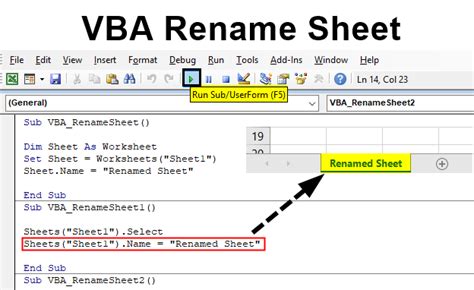
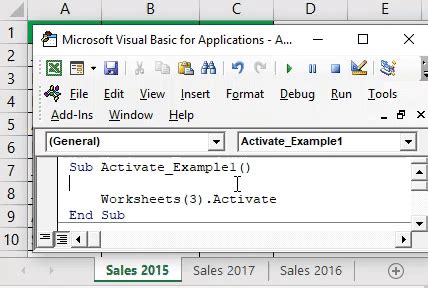
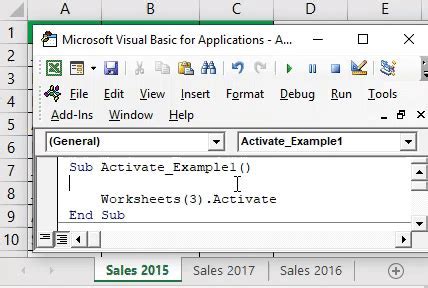
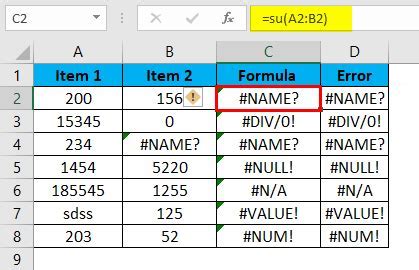
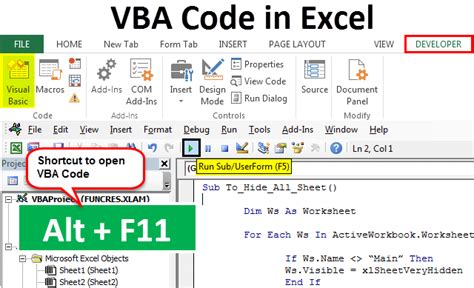
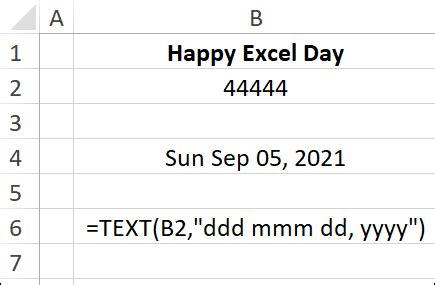
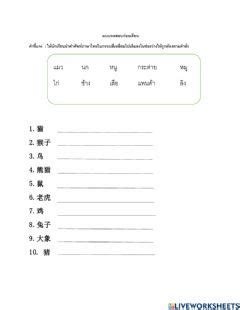
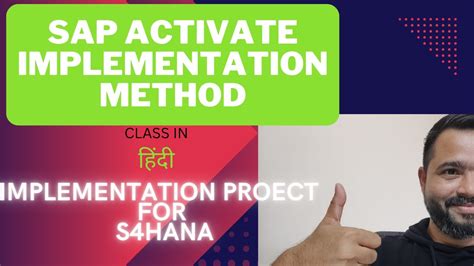
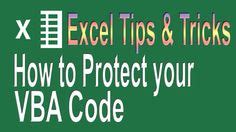
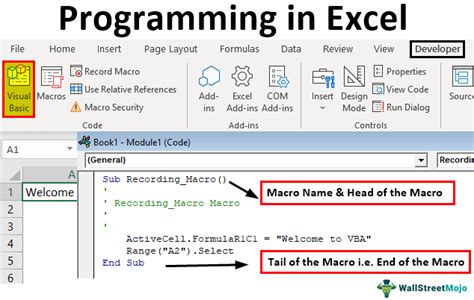
We hope this article has provided you with a comprehensive understanding of how to activate a specific sheet in Excel using VBA. Whether you're using the sheet's name, index, or codename, we've covered the different methods and provided examples to help you get started.
If you have any questions or need further assistance, please don't hesitate to leave a comment below. We'd be happy to help!