Intro
Master Excel VBA with our step-by-step guide on how to count rows in Excel VBA. Learn efficient methods to automate row counting, using Excel VBA techniques such as loops, ranges, and worksheet functions. Improve data analysis and processing with expert VBA coding tips and best practices.
Counting rows in Excel is a common task that can be accomplished using various methods, including Excel VBA. In this article, we will explore the different ways to count rows in Excel VBA, including using the Rows.Count
property, the Range.Rows.Count
property, and the WorksheetFunction.CountA
method.
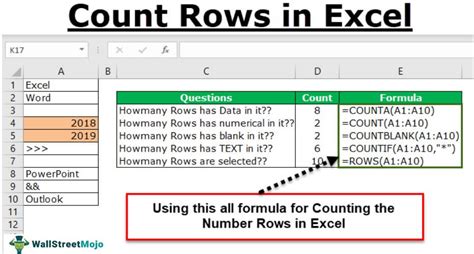
Why Count Rows in Excel VBA?
Counting rows in Excel VBA is useful in a variety of situations, such as:
- Determining the number of rows in a dataset
- Looping through a range of cells
- Creating dynamic charts and graphs
- Performing data analysis and manipulation
Using the Rows.Count Property
The Rows.Count
property returns the number of rows in a worksheet. This property is useful when you need to count the total number of rows in a worksheet, including blank rows.
Sub CountRowsUsingRowsCount()
Dim rowCount As Long
rowCount = ActiveSheet.Rows.Count
MsgBox "The number of rows in the worksheet is: " & rowCount
End Sub
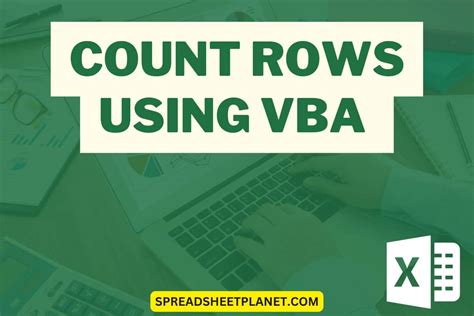
Using the Range.Rows.Count Property
The Range.Rows.Count
property returns the number of rows in a specific range. This property is useful when you need to count the number of rows in a specific range of cells.
Sub CountRowsUsingRangeRowsCount()
Dim rowCount As Long
rowCount = Range("A1:A10").Rows.Count
MsgBox "The number of rows in the range is: " & rowCount
End Sub
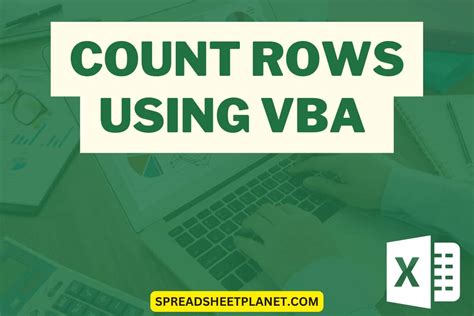
Using the WorksheetFunction.CountA Method
The WorksheetFunction.CountA
method returns the number of non-blank cells in a range. This method is useful when you need to count the number of rows in a range that contain data.
Sub CountRowsUsingWorksheetFunctionCountA()
Dim rowCount As Long
rowCount = WorksheetFunction.CountA(Range("A1:A10"))
MsgBox "The number of non-blank rows in the range is: " & rowCount
End Sub
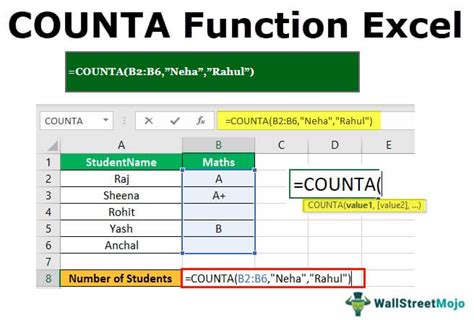
Best Practices for Counting Rows in Excel VBA
- Use the
Rows.Count
property to count the total number of rows in a worksheet. - Use the
Range.Rows.Count
property to count the number of rows in a specific range. - Use the
WorksheetFunction.CountA
method to count the number of non-blank rows in a range. - Avoid using the
COUNTA
function in Excel VBA, as it can be slow and inefficient. - Use variables to store the row count, rather than hard-coding the value.
Common Errors When Counting Rows in Excel VBA
- Using the
COUNTA
function instead of theWorksheetFunction.CountA
method. - Forgetting to specify the range when using the
Range.Rows.Count
property. - Using the
Rows.Count
property instead of theRange.Rows.Count
property.
Conclusion
Counting rows in Excel VBA is a common task that can be accomplished using various methods. By using the Rows.Count
property, the Range.Rows.Count
property, and the WorksheetFunction.CountA
method, you can efficiently and accurately count the number of rows in a worksheet or range. Remember to follow best practices and avoid common errors to ensure that your code runs smoothly and efficiently.
Count Rows in Excel VBA Image Gallery
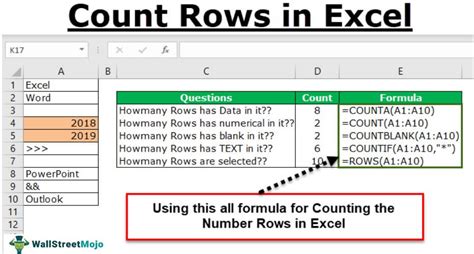
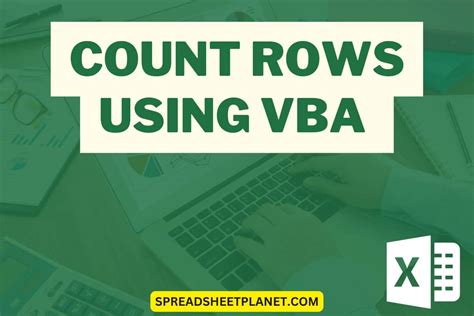
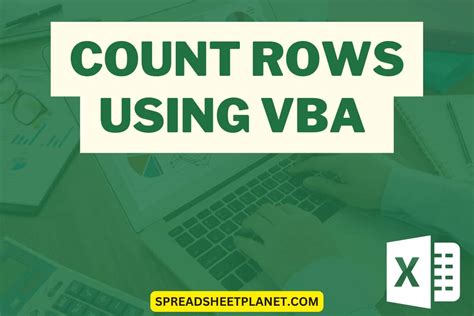
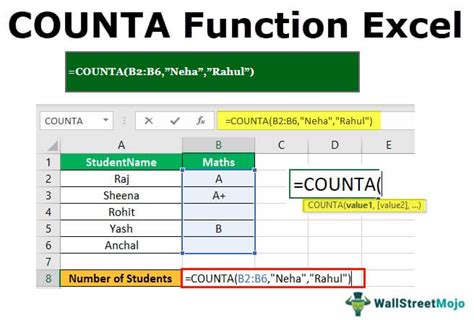
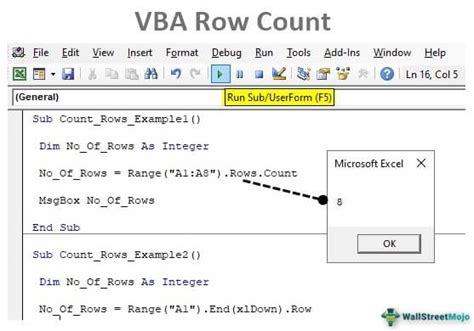
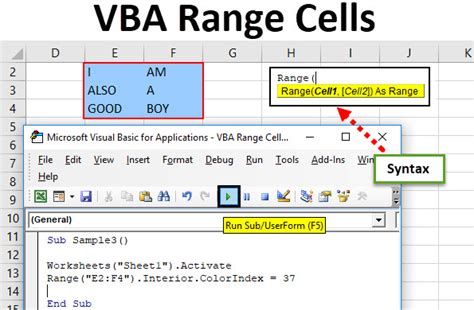
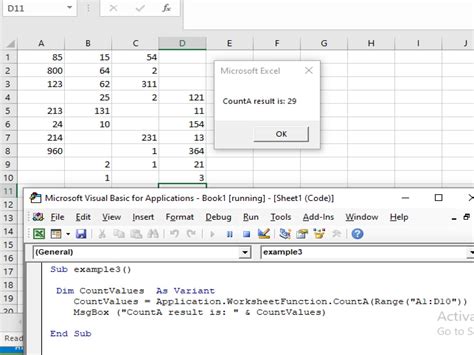
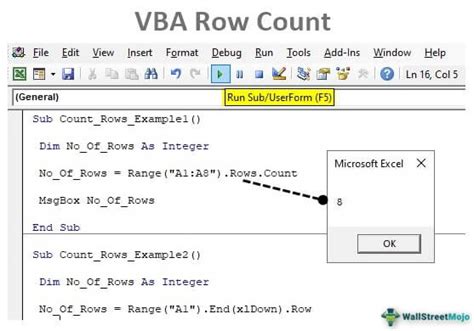
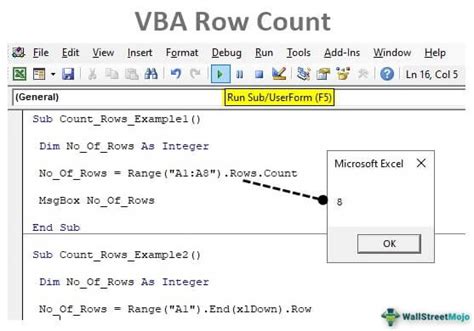
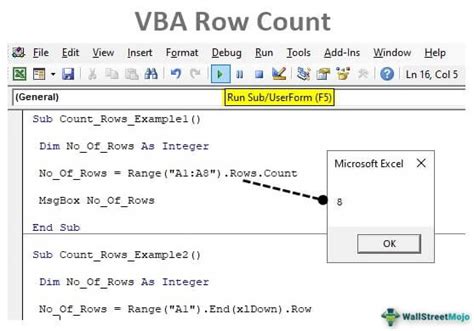
We hope this article has been helpful in teaching you how to count rows in Excel VBA. If you have any questions or need further assistance, please don't hesitate to ask.