Intro
Discover how to create a new workbook in Excel with ease using VBA macro code. Learn the step-by-step process of creating a new Excel workbook, setting up worksheets, and customizing your workbook with VBA macros. Master Excel automation and streamline your workflow with this easy-to-follow guide on VBA Excel workbook creation.
Creating a new workbook in Excel can be a repetitive task, especially when you need to start from scratch on a regular basis. However, with the help of Visual Basic for Applications (VBA), you can automate this process and save yourself some time. In this article, we'll explore how to create a new workbook in Excel using VBA macro code.
Why Use VBA?
VBA is a powerful programming language that allows you to automate tasks in Excel. By using VBA, you can create custom macros that can perform a wide range of tasks, from simple operations like creating a new workbook to complex tasks like data analysis and reporting. With VBA, you can streamline your workflow, reduce errors, and increase productivity.
Creating a New Workbook with VBA
To create a new workbook using VBA, you'll need to open the Visual Basic Editor in Excel. To do this, press Alt + F11
or navigate to Developer
> Visual Basic
in the ribbon. Once the Visual Basic Editor is open, you'll see a blank module. In this module, you can write your VBA code.
Here's a simple example of VBA code that creates a new workbook:
Sub CreateNewWorkbook()
Workbooks.Add
End Sub
This code creates a new workbook with a single worksheet. You can run this macro by clicking Run
> Run Sub/UserForm
in the Visual Basic Editor or by pressing F5
.
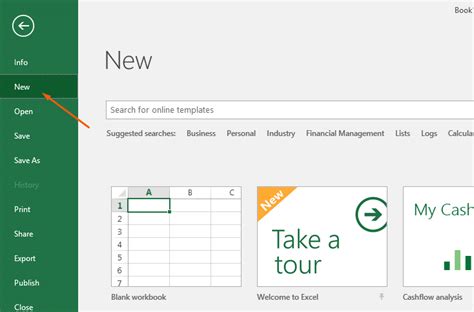
Customizing the New Workbook
While the previous code creates a new workbook, you may want to customize the new workbook further. For example, you may want to add multiple worksheets, set the worksheet names, or even format the worksheets. Here's an example of VBA code that creates a new workbook with multiple worksheets and customizes the worksheet names:
Sub CreateNewWorkbookWithMultipleWorksheets()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
' Add multiple worksheets
newWorkbook.Worksheets.Add Count:=2
' Set worksheet names
newWorkbook.Worksheets(1).Name = "Sheet1"
newWorkbook.Worksheets(2).Name = "Sheet2"
newWorkbook.Worksheets(3).Name = "Sheet3"
End Sub
This code creates a new workbook with three worksheets and sets the worksheet names to "Sheet1", "Sheet2", and "Sheet3".
Saving the New Workbook
Once you've created a new workbook, you may want to save it to a specific location. Here's an example of VBA code that saves the new workbook to a specific location:
Sub SaveNewWorkbook()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
' Save the new workbook
newWorkbook.SaveAs Filename:="C:\Users\Username\Documents\NewWorkbook.xlsx"
End Sub
This code saves the new workbook to the "C:\Users\Username\Documents" folder with the file name "NewWorkbook.xlsx".
Best Practices for Creating a New Workbook with VBA
When creating a new workbook with VBA, there are several best practices to keep in mind:
- Use descriptive variable names: Use descriptive variable names to make your code easier to read and understand.
- Use error handling: Use error handling to catch any errors that may occur when creating a new workbook.
- Use relative paths: Use relative paths when saving the new workbook to avoid hardcoding file paths.
- Test your code: Test your code thoroughly to ensure it works as expected.
Common Errors When Creating a New Workbook with VBA
When creating a new workbook with VBA, you may encounter several common errors. Here are some of the most common errors and how to fix them:
- Error 76: Path not found: This error occurs when the file path specified in the
SaveAs
method does not exist. To fix this error, ensure the file path is correct and the folder exists. - Error 1004: Method 'SaveAs' of object 'Workbook' failed: This error occurs when the
SaveAs
method fails. To fix this error, ensure the file name and path are correct and the folder has write permission.
Create New Workbook Gallery
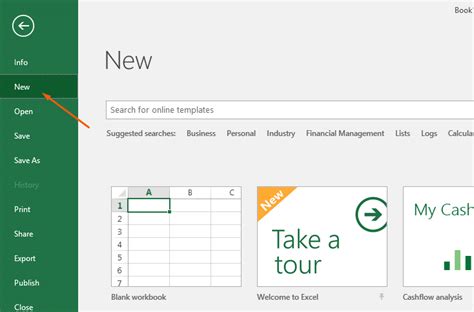
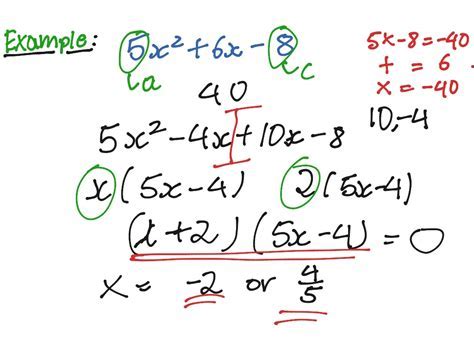
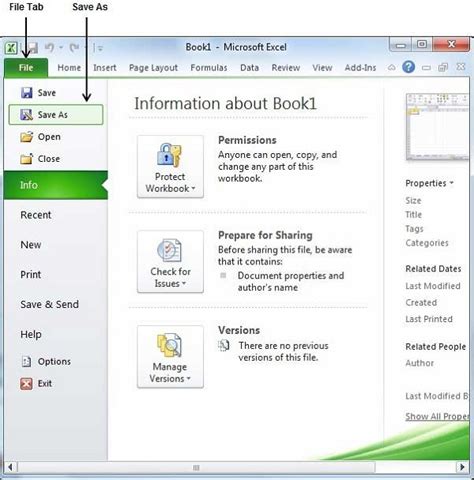
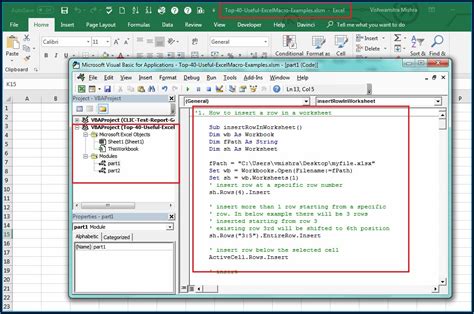
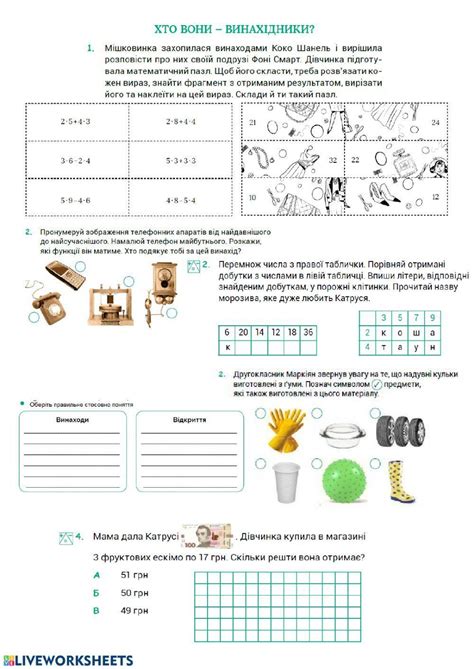
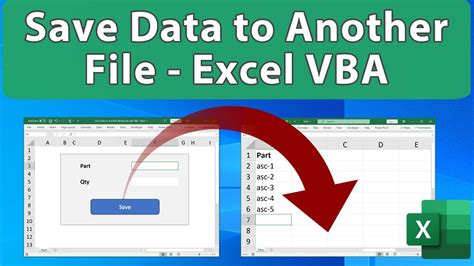
We hope this article has helped you understand how to create a new workbook with VBA. By following the examples and best practices outlined in this article, you can automate the process of creating a new workbook and save yourself time and effort. If you have any questions or need further assistance, please don't hesitate to ask.