Intro
Finding the last row in Excel with VBA is a fundamental task that can be used in a variety of applications, such as data manipulation, reporting, and automation. In this article, we will explore the different methods to find the last row in Excel using VBA, including their advantages and disadvantages.
Why Find the Last Row in Excel?
Before we dive into the methods, let's discuss why finding the last row in Excel is important. In many cases, you may need to perform operations on a range of data, such as formatting, data validation, or calculations. Knowing the last row of your data range is crucial to ensure that your operations are applied correctly and efficiently.
Method 1: Using the `UsedRange` Property
One of the simplest ways to find the last row in Excel using VBA is by using the UsedRange
property. This property returns a range object that represents the used range of the worksheet.
Sub FindLastRowUsedRange()
Dim lastRow As Long
lastRow = ActiveSheet.UsedRange.Rows.Count
MsgBox "The last row is: " & lastRow
End Sub
This method is quick and easy to implement, but it has some limitations. The UsedRange
property may not always return the last row of your data range, especially if there are blank rows or columns in your worksheet.
Method 2: Using the `Find` Method
Another method to find the last row in Excel using VBA is by using the Find
method. This method searches for a specific value in a range and returns the address of the first occurrence.
Sub FindLastRowFindMethod()
Dim lastRow As Long
lastRow = ActiveSheet.Cells.Find("*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
MsgBox "The last row is: " & lastRow
End Sub
This method is more reliable than the UsedRange
property, but it may still return incorrect results if there are blank rows or columns in your worksheet.
Method 3: Using the `End` Property
The End
property is another way to find the last row in Excel using VBA. This property returns a range object that represents the cell at the end of the worksheet.
Sub FindLastRowEndProperty()
Dim lastRow As Long
lastRow = ActiveSheet.Cells(1, 1).End(xlDown).Row
MsgBox "The last row is: " & lastRow
End Sub
This method is similar to the Find
method, but it searches for the last non-blank cell in the column.
Method 4: Using the `SpecialCells` Method
The SpecialCells
method is a more advanced way to find the last row in Excel using VBA. This method returns a range object that represents the cells that match a specific criteria.
Sub FindLastRowSpecialCells()
Dim lastRow As Long
lastRow = ActiveSheet.Cells.SpecialCells(xlCellTypeLastCell).Row
MsgBox "The last row is: " & lastRow
End Sub
This method is the most reliable way to find the last row in Excel using VBA, as it searches for the last non-blank cell in the worksheet.
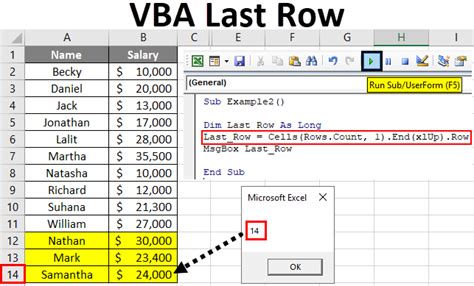
Conclusion
Finding the last row in Excel using VBA is an essential task that can be achieved using different methods. While the UsedRange
property is quick and easy to implement, it may not always return the correct results. The Find
method, End
property, and SpecialCells
method are more reliable ways to find the last row in Excel using VBA.
We hope this article has provided you with the necessary knowledge to find the last row in Excel using VBA. If you have any questions or need further assistance, please don't hesitate to ask.
Gallery of Finding Last Row in Excel VBA
Find Last Row in Excel VBA Image Gallery
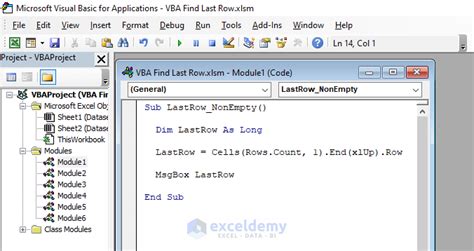
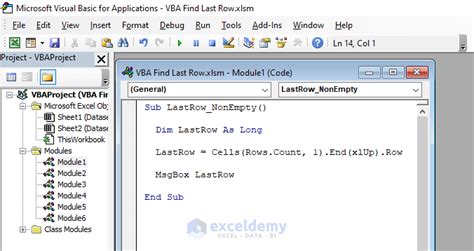
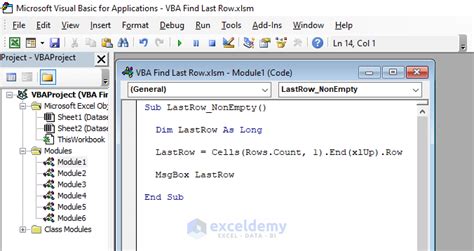
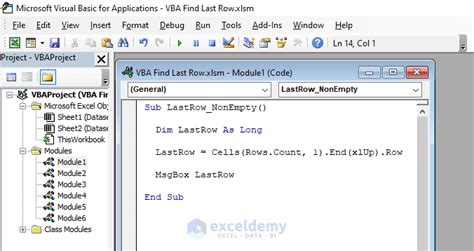
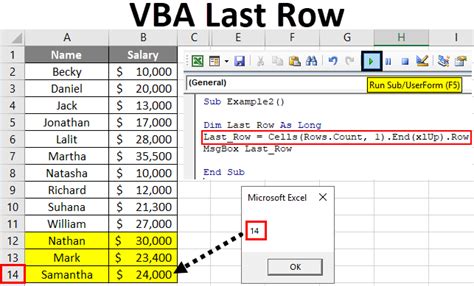
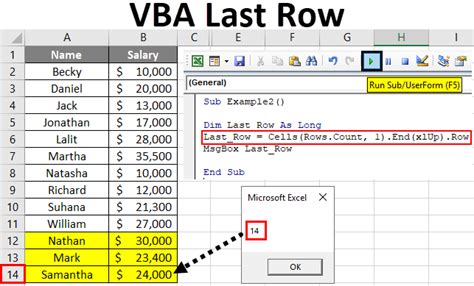
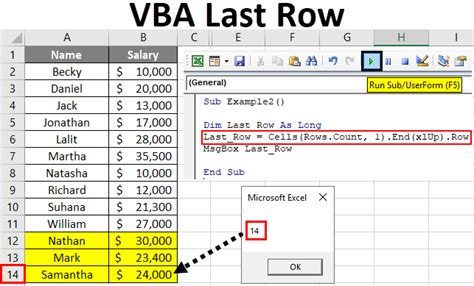
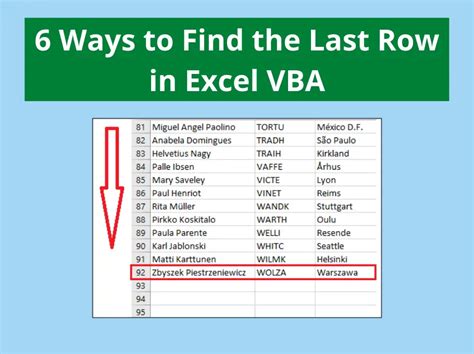
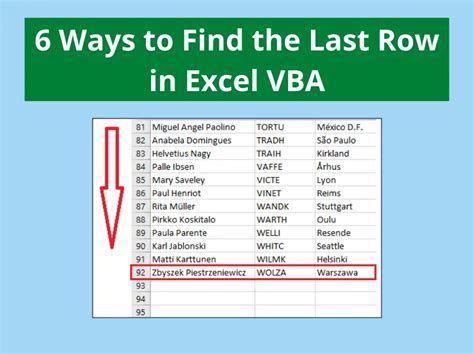
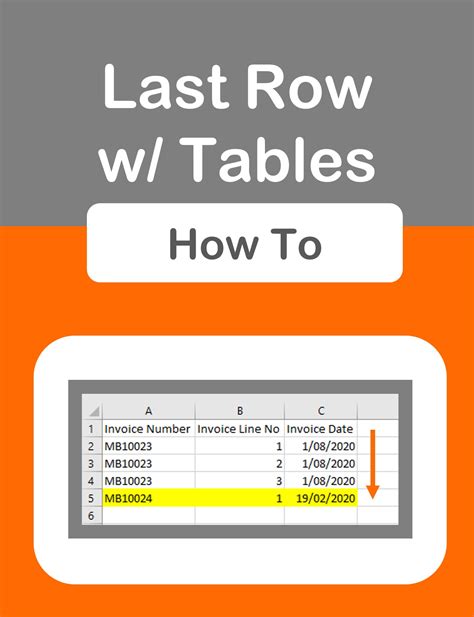
We hope you found this article helpful. If you have any questions or need further assistance, please don't hesitate to ask.