Microsoft Excel is an incredibly powerful tool for data analysis, and when combined with Visual Basic for Applications (VBA), it becomes even more versatile. One of the most common tasks in Excel VBA is pasting values, which is essential for data manipulation and processing. In this article, we will explore five different ways to paste values in VBA Excel, along with their benefits and use cases.
The Importance of Pasting Values in VBA Excel
Before diving into the different methods, it's essential to understand why pasting values is crucial in VBA Excel. When working with data, you often need to copy and paste values from one range to another. However, simply copying and pasting can lead to errors, formatting issues, and even data corruption. By pasting values only, you can ensure that the data is accurately transferred without any unwanted formatting or formulas.
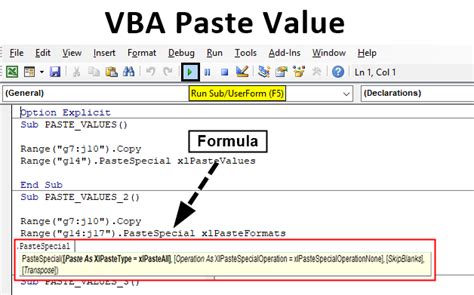
Method 1: Using the PasteSpecial
Method
The PasteSpecial
method is one of the most common ways to paste values in VBA Excel. This method allows you to specify the type of paste operation, including values, formats, and formulas.
Sub PasteValuesUsingPasteSpecial()
Range("A1").Copy
Range("B1").PasteSpecial xlPasteValues
Application.CutCopyMode = False
End Sub
In this example, we copy the value in cell A1 and paste it into cell B1 using the PasteSpecial
method with the xlPasteValues
argument.
Method 2: Using the Value
Property
Another way to paste values is by using the Value
property of the Range
object. This method is more straightforward and efficient than the PasteSpecial
method.
Sub PasteValuesUsingValueProperty()
Range("B1").Value = Range("A1").Value
End Sub
In this example, we set the value of cell B1 to be equal to the value of cell A1, effectively pasting the value.
Method 3: Using the Paste
Method with the xlPasteValues
Argument
The Paste
method is similar to the PasteSpecial
method, but it allows you to specify additional arguments, including the paste type.
Sub PasteValuesUsingPasteMethod()
Range("A1").Copy
Range("B1").Paste xlPasteValues
Application.CutCopyMode = False
End Sub
In this example, we copy the value in cell A1 and paste it into cell B1 using the Paste
method with the xlPasteValues
argument.
Method 4: Using the WorksheetFunction
Object
The WorksheetFunction
object provides a way to access Excel worksheet functions, including the PasteValues
function.
Sub PasteValuesUsingWorksheetFunction()
Range("B1").Value = WorksheetFunction.PasteValues(Range("A1"))
End Sub
In this example, we use the WorksheetFunction
object to paste the value of cell A1 into cell B1.
Method 5: Using the Range.Paste
Method with the xlPasteValues
Argument
The Range.Paste
method is similar to the Paste
method, but it allows you to specify the paste type as an argument.
Sub PasteValuesUsingRangePasteMethod()
Range("A1").Copy
Range("B1").Paste xlPasteValues
Application.CutCopyMode = False
End Sub
In this example, we copy the value in cell A1 and paste it into cell B1 using the Range.Paste
method with the xlPasteValues
argument.
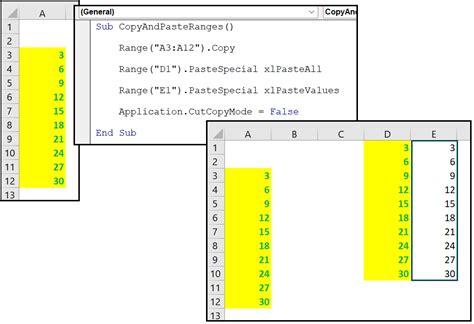
Conclusion
In this article, we explored five different ways to paste values in VBA Excel, each with its own benefits and use cases. Whether you prefer using the PasteSpecial
method, the Value
property, or one of the other methods, you now have the knowledge to accurately paste values in your Excel VBA applications.
Gallery of Excel VBA Paste Values
Excel VBA Paste Values Image Gallery
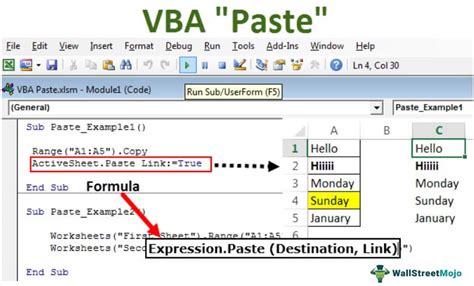
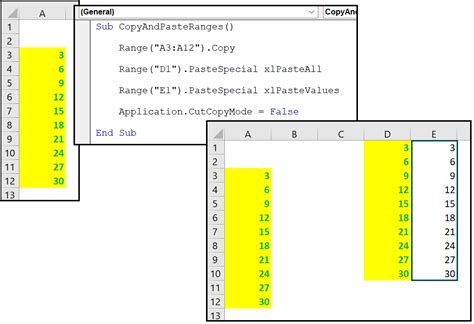
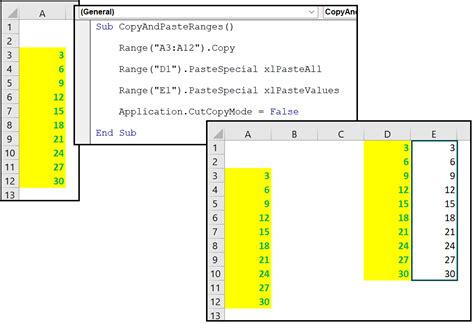
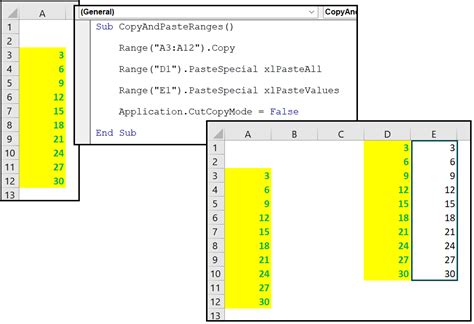
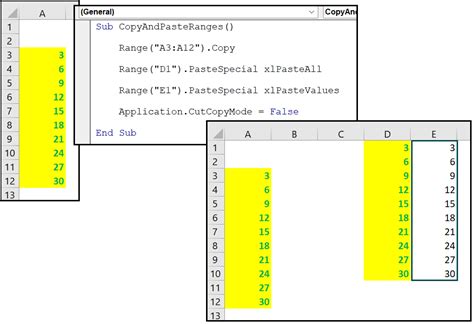
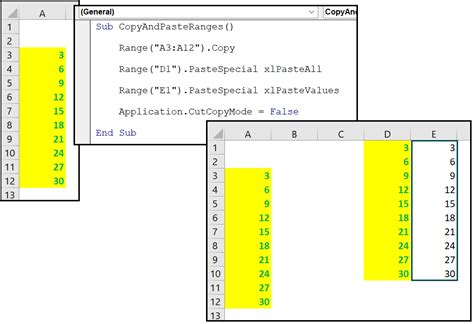
FAQ
Q: What is the difference between the PasteSpecial
method and the Paste
method?
A: The PasteSpecial
method allows you to specify the type of paste operation, including values, formats, and formulas, while the Paste
method allows you to specify additional arguments, including the paste type.
Q: Can I use the Value
property to paste values from one range to another?
A: Yes, you can use the Value
property to paste values from one range to another by setting the value of the destination range to be equal to the value of the source range.
Q: How do I paste values using the WorksheetFunction
object?
A: You can use the WorksheetFunction
object to paste values by calling the PasteValues
function and passing the source range as an argument.