Renaming a sheet in Excel VBA is a common task that can be accomplished in several ways. The method you choose will depend on your specific needs and the complexity of your project. In this article, we will explore five different ways to rename a sheet in Excel VBA, providing you with a comprehensive understanding of the process.
The Importance of Renaming Sheets in Excel VBA
Before we dive into the methods, it's essential to understand why renaming sheets in Excel VBA is crucial. Renaming sheets can help you:
- Organize your worksheets more efficiently
- Improve the readability of your code
- Avoid confusion when working with multiple sheets
- Enhance the overall user experience
Now, let's move on to the five ways to rename a sheet in Excel VBA.
Method 1: Using the `Name` Property
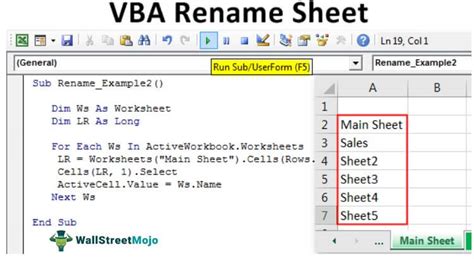
The most straightforward way to rename a sheet in Excel VBA is by using the Name
property. This method involves setting the Name
property of the worksheet object to the new name.
Sub RenameSheetUsingNameProperty()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
ws.Name = "New Sheet Name"
End Sub
Method 2: Using the ActiveSheet
Object
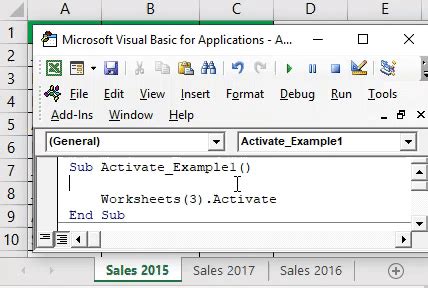
If you want to rename the currently active sheet, you can use the ActiveSheet
object. This method is useful when you need to rename the sheet that is currently being used.
Sub RenameActiveSheet()
ActiveSheet.Name = "New Sheet Name"
End Sub
Method 3: Using the `Worksheets` Collection
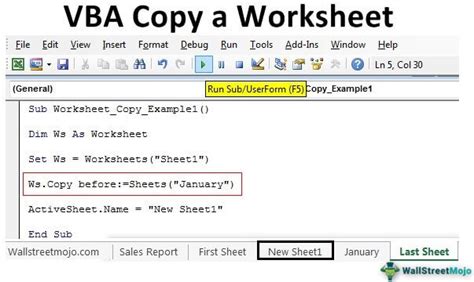
You can also rename a sheet by using the Worksheets
collection. This method involves accessing the worksheet object using its index or name.
Sub RenameSheetUsingWorksheetsCollection()
ThisWorkbook.Worksheets(1).Name = "New Sheet Name"
' or
ThisWorkbook.Worksheets("Sheet1").Name = "New Sheet Name"
End Sub
Method 4: Using the Worksheet
Object with Error Handling
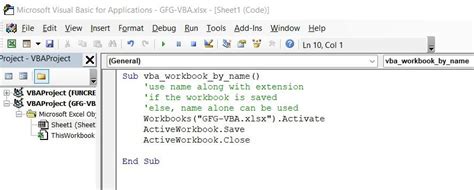
When renaming a sheet, it's essential to handle errors that may occur. You can use the Worksheet
object with error handling to rename a sheet.
Sub RenameSheetWithErrorHandling()
On Error Resume Next
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
ws.Name = "New Sheet Name"
If Err.Number <> 0 Then
MsgBox "Error renaming sheet: " & Err.Description
End If
On Error GoTo 0
End Sub
Method 5: Using a Loop to Rename Multiple Sheets
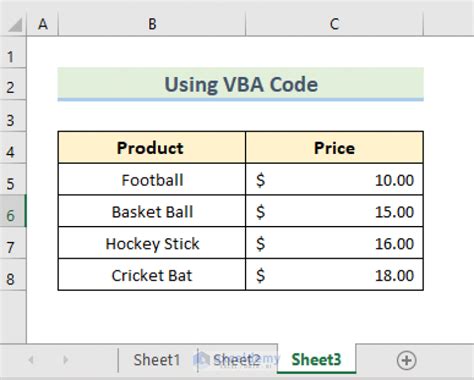
If you need to rename multiple sheets, you can use a loop to iterate through the Worksheets
collection.
Sub RenameMultipleSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Name = "New Sheet Name " & ws.Index
Next ws
End Sub
Gallery of Rename Sheet VBA
Rename Sheet VBA Image Gallery
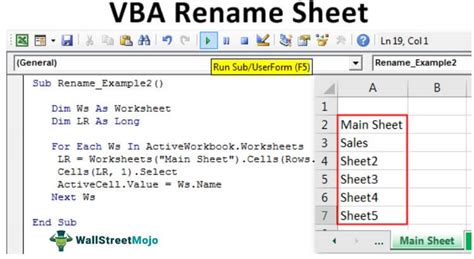
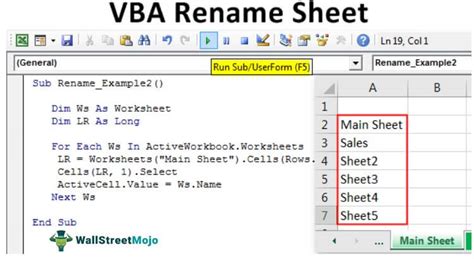
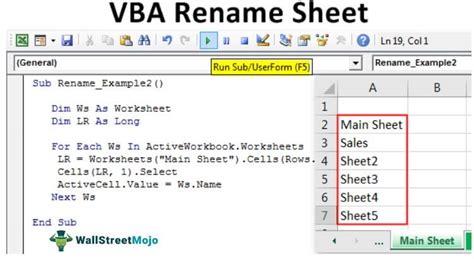
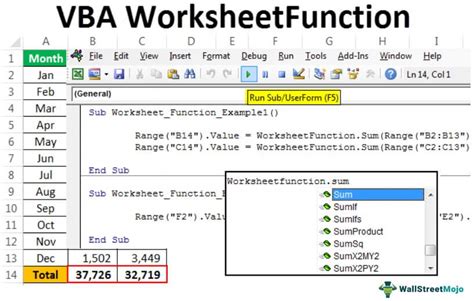
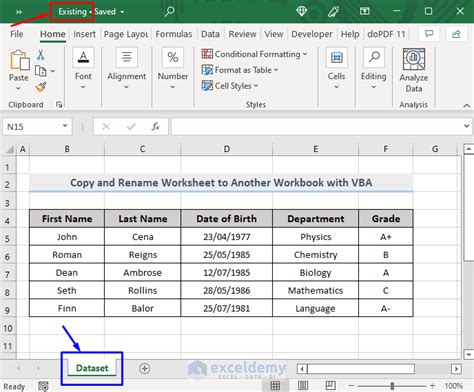
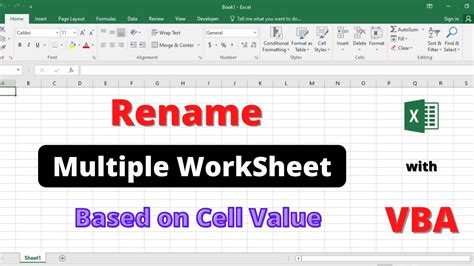
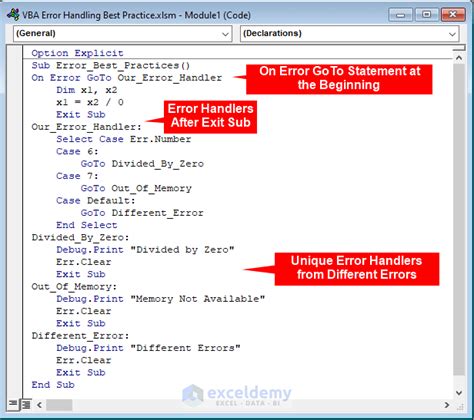
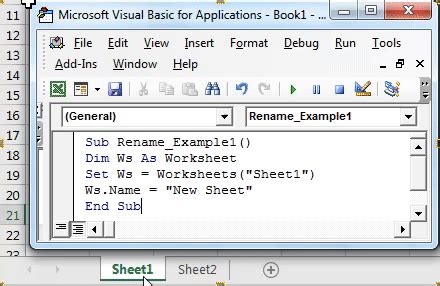
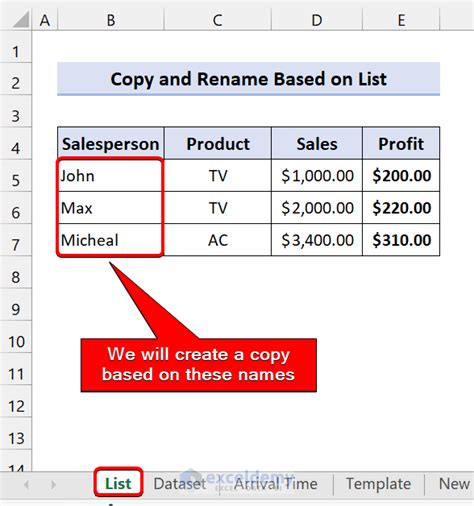
We hope this article has provided you with a comprehensive understanding of the five ways to rename a sheet in Excel VBA. Whether you're a beginner or an advanced user, these methods will help you to efficiently rename your sheets and improve your overall productivity.
If you have any questions or need further assistance, please don't hesitate to ask. Share your thoughts and experiences in the comments section below.