Intro
Master Excel VBA with ease! Learn how to trim strings in Excel VBA effortlessly, removing unwanted spaces and characters. Discover the most efficient methods, including using the Trim function, regular expressions, and VBA coding techniques. Optimize your data cleaning process with these expert tips and boost your spreadsheet productivity.
Trimming strings is an essential task in Excel VBA, and there are several ways to do it. In this article, we will explore the different methods to trim strings in Excel VBA, including using the Trim function, regular expressions, and other techniques.
Why Trim Strings in Excel VBA?
Trimming strings is necessary to remove unwanted characters, such as spaces, tabs, or line breaks, from the beginning or end of a string. This can improve the accuracy of data analysis, data validation, and data manipulation. In Excel VBA, trimming strings can also help to prevent errors when working with text data.
Method 1: Using the Trim Function
The Trim function is a built-in function in Excel VBA that removes spaces from the beginning and end of a string. The syntax is simple:
Trim(string)
Where string
is the input string.
Here is an example:
Sub TrimString()
Dim originalString As String
originalString = " Hello World "
Debug.Print Trim(originalString) ' Outputs: "Hello World"
End Sub
Method 2: Using Regular Expressions
Regular expressions (RegEx) are a powerful tool for text manipulation. In Excel VBA, you can use the RegExp object to trim strings.
First, you need to set a reference to the RegExp object in the Visual Basic Editor. To do this, go to Tools
> References
and check the box next to Microsoft VBScript Regular Expressions
.
Then, you can use the following code:
Sub TrimStringRegEx()
Dim originalString As String
originalString = " Hello World "
Dim regEx As Object
Set regEx = CreateObject("VBScript.RegExp")
regEx.Pattern = "^\s+|\s+$"
Debug.Print regEx.Replace(originalString, "") ' Outputs: "Hello World"
End Sub
Method 3: Using the LTrim and RTrim Functions
The LTrim and RTrim functions are also built-in functions in Excel VBA. The LTrim function removes spaces from the beginning of a string, while the RTrim function removes spaces from the end of a string.
Here is an example:
Sub TrimStringLTrimRTrim()
Dim originalString As String
originalString = " Hello World "
Debug.Print LTrim(RTrim(originalString)) ' Outputs: "Hello World"
End Sub
Method 4: Using the WorksheetFunction.Trim Function
The WorksheetFunction.Trim function is a built-in function in Excel that removes spaces from the beginning and end of a string. You can use this function in Excel VBA like this:
Sub TrimStringWorksheetFunction()
Dim originalString As String
originalString = " Hello World "
Debug.Print Application.WorksheetFunction.Trim(originalString) ' Outputs: "Hello World"
End Sub
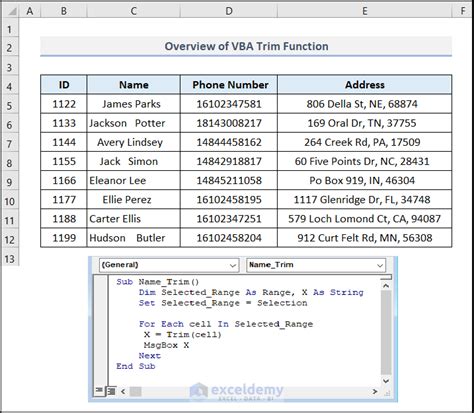
Best Practices for Trimming Strings in Excel VBA
Here are some best practices to keep in mind when trimming strings in Excel VBA:
- Always check the input string for null or empty values before trimming.
- Use the Trim function or WorksheetFunction.Trim function for simple trimming tasks.
- Use regular expressions for more complex trimming tasks.
- Use the LTrim and RTrim functions for trimming specific sides of the string.
Conclusion
Trimming strings is an essential task in Excel VBA, and there are several ways to do it. By using the Trim function, regular expressions, or other techniques, you can remove unwanted characters from your strings and improve the accuracy of your data analysis. Remember to follow best practices and use the right technique for your specific task.
Gallery of Trim String in Excel VBA
Trim String in Excel VBA Image Gallery
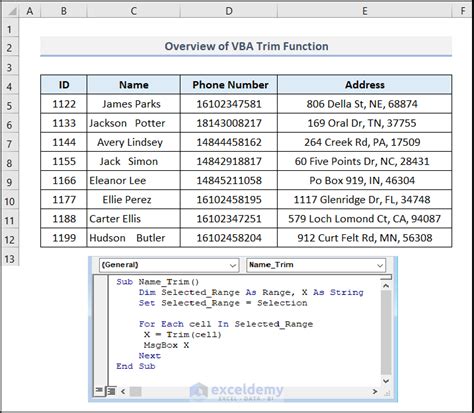

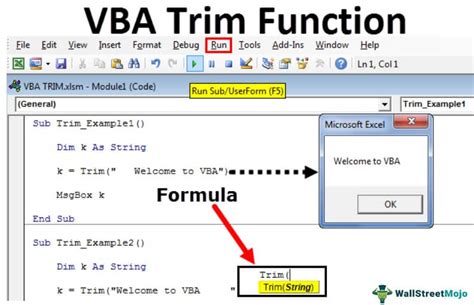
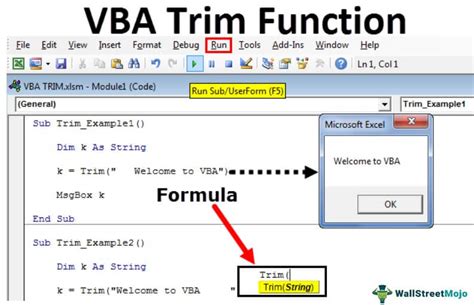
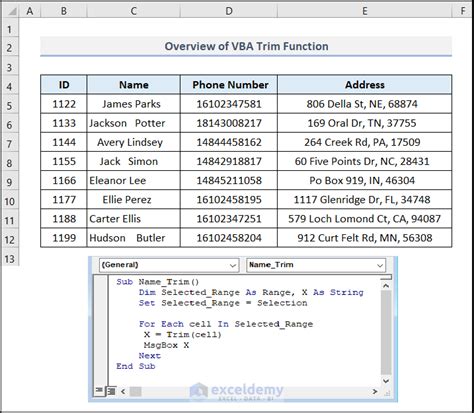
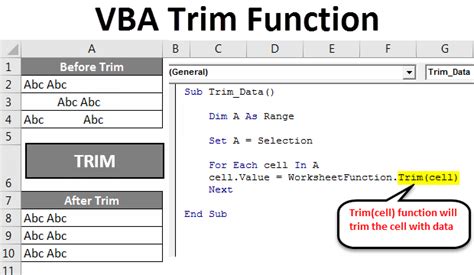
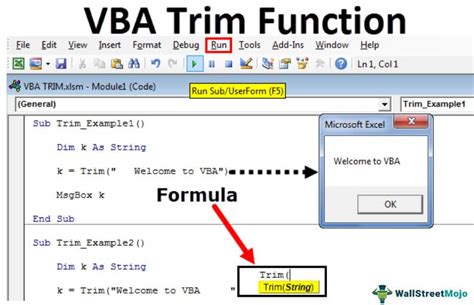
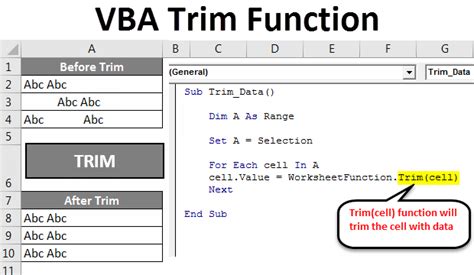
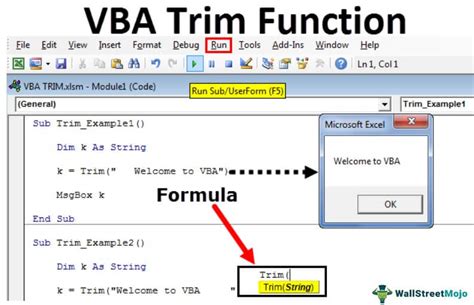
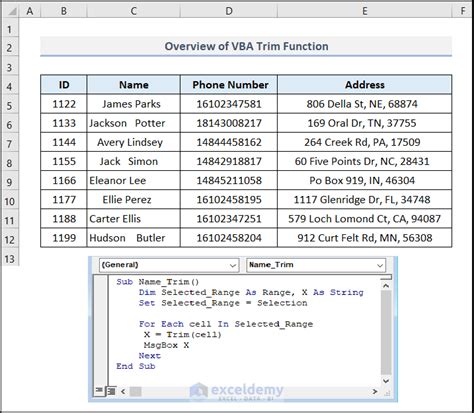