Intro
Discover how to efficiently locate specific text within your VBA projects with our expert guide. Learn 5 effective ways to find a string in VBA, including using the InStr function, looping through arrays, and utilizing regular expressions. Master VBA string search techniques and boost your coding productivity with these actionable tips.
Finding a specific string within a larger text or dataset is a common task in programming, and VBA (Visual Basic for Applications) is no exception. Whether you're working with Excel, Word, or another Office application, being able to efficiently locate specific strings can greatly enhance your productivity and the effectiveness of your macros. Here are five ways to find a string in VBA, each with its own strengths and best use cases.
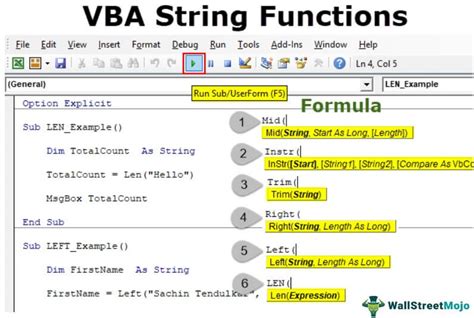
1. Using the InStr
Function
The InStr
function is one of the most straightforward methods for finding a string within another string in VBA. It returns the position of the first occurrence of the specified string within the text.
Dim text As String
Dim search As String
Dim position As Integer
text = "This is a sample text."
search = "sample"
position = InStr(text, search)
If position > 0 Then
MsgBox "The string '" & search & "' is found at position " & position
Else
MsgBox "The string '" & search & "' is not found."
End If
2. Utilizing the Like
Operator
The Like
operator in VBA allows you to use wildcards to find strings that match a pattern. While it doesn't return the position of the match, it's useful for determining if a string contains a certain substring or matches a specific pattern.
Dim text As String
Dim pattern As String
text = "This is a sample text."
pattern = "*sample*"
If text Like pattern Then
MsgBox "The text contains the specified pattern."
Else
MsgBox "The text does not contain the specified pattern."
End If
3. Employing Regular Expressions
Regular expressions (regex) offer a powerful method for finding complex patterns within strings. VBA supports regex through the RegExp
object in the Microsoft VBScript Regular Expressions
library.
Dim text As String
Dim regex As Object
Dim match As Object
Set regex = CreateObject("VBScript.RegExp")
regex.Pattern = "sample"
regex.IgnoreCase = True
text = "This is a sample text."
If regex.Test(text) Then
MsgBox "The text contains the specified pattern."
Else
MsgBox "The text does not contain the specified pattern."
End If
Set regex = Nothing
4. Looping Through Characters
For certain situations, especially when you need to perform actions based on the position of the found string, looping through each character of the text can be an effective approach.
Dim text As String
Dim search As String
Dim i As Integer
Dim found As Boolean
text = "This is a sample text."
search = "sample"
found = False
For i = 1 To Len(text)
If Mid(text, i, Len(search)) = search Then
found = True
MsgBox "The string '" & search & "' is found at position " & i
Exit For
End If
Next i
If Not found Then
MsgBox "The string '" & search & "' is not found."
End If
5. Array Methods
If you're working with arrays of strings, you can leverage array methods, combined with the Filter
function or looping through the array, to find specific strings.
Dim arr() As String
Dim search As String
Dim i As Integer
arr = Split("This is a sample text.", " ")
search = "sample"
For i = LBound(arr) To UBound(arr)
If arr(i) Like search Then
MsgBox "The string '" & search & "' is found."
Exit For
End If
Next i
Gallery of VBA String Manipulation
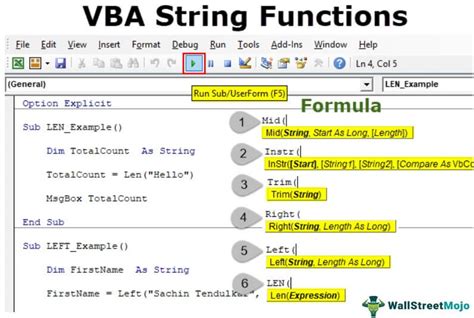
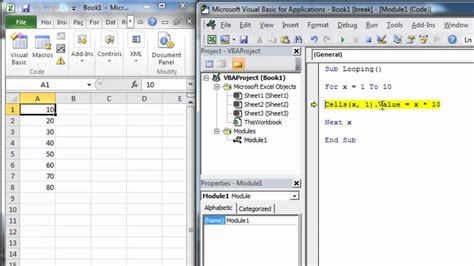
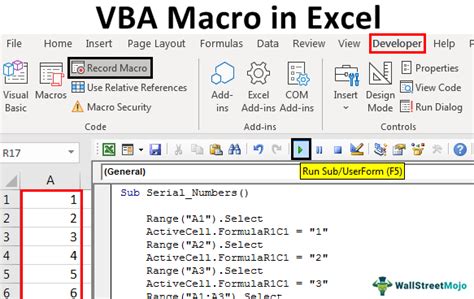
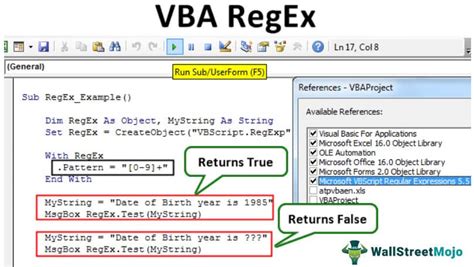
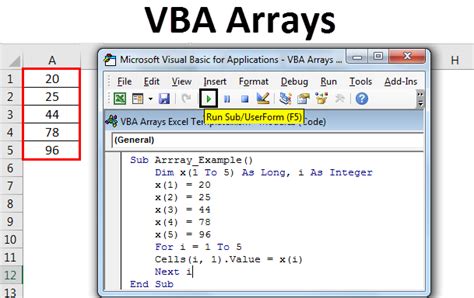
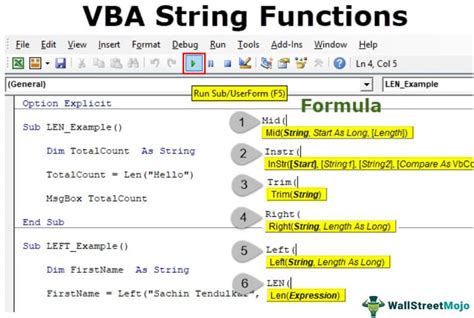
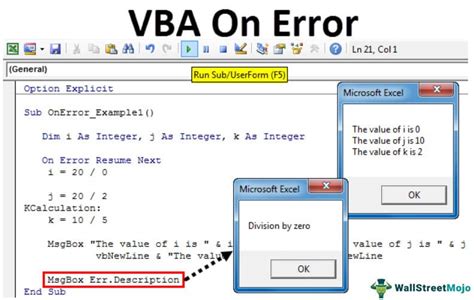
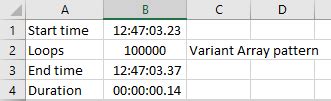
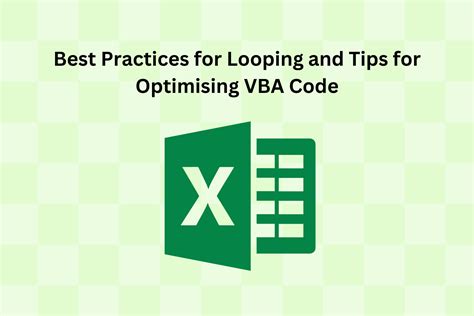
Each of these methods has its place in the world of VBA programming, and mastering them can significantly improve your skills in automating tasks in Office applications. Whether you're a beginner looking to automate simple tasks or an advanced user seeking to optimize complex workflows, the ability to efficiently find and manipulate strings is fundamental.