Finding Value in Excel Column with VBA
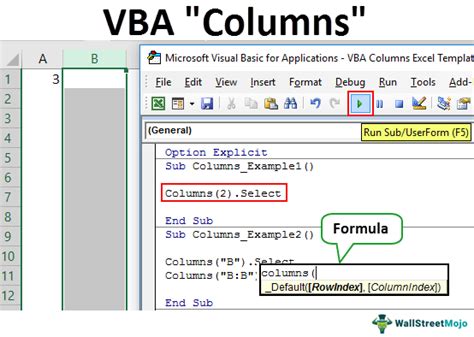
In Microsoft Excel, finding a specific value within a large dataset can be a daunting task, especially when dealing with multiple columns and rows. However, with the help of Visual Basic for Applications (VBA), you can automate this process and make it more efficient. In this article, we will explore how to find a value in an Excel column using VBA.
Finding a value in an Excel column is a common task that can be accomplished using various methods. One approach is to use the built-in Excel function, VLOOKUP
or INDEX/MATCH
. However, these functions have limitations and may not always provide the desired results. VBA, on the other hand, offers a more flexible and powerful solution.
Why Use VBA to Find Value in Excel Column?
There are several reasons why you might want to use VBA to find a value in an Excel column:
- Flexibility: VBA allows you to customize the search process to suit your specific needs. You can search for values in a specific column, row, or range, and perform actions based on the results.
- Speed: VBA can search large datasets quickly and efficiently, making it ideal for big data applications.
- Accuracy: VBA can perform exact matches, partial matches, or wildcard searches, depending on your requirements.
Basic VBA Code to Find Value in Excel Column
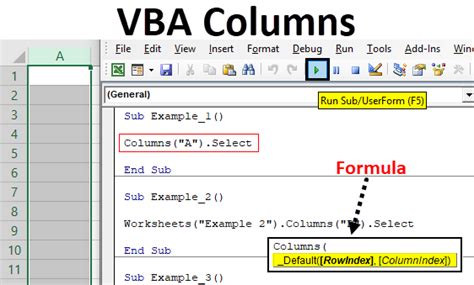
To get started with finding a value in an Excel column using VBA, you can use the following basic code:
Sub FindValueInColumn()
Dim ws As Worksheet
Dim column As Range
Dim valueToFind As String
Dim foundCell As Range
' Set the worksheet and column
Set ws = ThisWorkbook.Worksheets("Sheet1")
Set column = ws.Range("A:A")
' Set the value to find
valueToFind = "Your Value"
' Find the value in the column
Set foundCell = column.Find(valueToFind)
' Check if the value is found
If Not foundCell Is Nothing Then
MsgBox "Value found in cell " & foundCell.Address
Else
MsgBox "Value not found"
End If
End Sub
This code searches for a value in the first column (A) of the active worksheet and displays a message box with the address of the found cell.
How to Use VBA to Find Value in Excel Column
To use this code, follow these steps:
- Open the Visual Basic Editor in Excel by pressing
Alt + F11
or by navigating toDeveloper > Visual Basic
in the ribbon. - In the Visual Basic Editor, click
Insert > Module
to insert a new module. - Paste the code into the module and modify the worksheet and column references to suit your needs.
- Set the value to find by replacing
"Your Value"
with the desired value. - Run the code by clicking
Run > Run Sub/UserForm
or by pressingF5
.
Advanced VBA Techniques for Finding Value in Excel Column
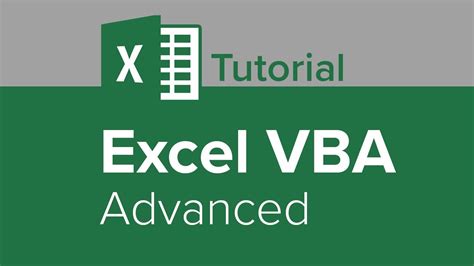
While the basic code provides a good starting point, you may need to perform more advanced searches or actions. Here are some techniques to enhance your VBA code:
- Partial Matches: To search for partial matches, use the
LookAt
argument in theFind
method. For example:Set foundCell = column.Find(valueToFind, LookAt:=xlPart)
- Wildcard Searches: To perform wildcard searches, use the
Like
operator. For example:If foundCell.Value Like "*" & valueToFind & "*" Then
- Multiple Values: To search for multiple values, use an array or a collection. For example:
Dim valuesToFind As Variant: valuesToFind = Array("Value1", "Value2")
- Actions: To perform actions based on the search results, use conditional statements or loops. For example:
If Not foundCell Is Nothing Then Range(foundCell.Address).Interior.Color = vbGreen
Common Errors and Troubleshooting
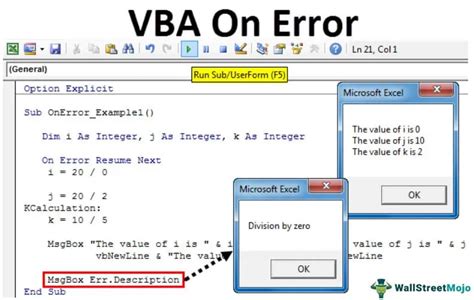
When working with VBA, you may encounter errors or unexpected results. Here are some common issues and troubleshooting tips:
- Object Not Set: Ensure that the worksheet and column references are correct.
- Value Not Found: Verify that the value to find is correct and exists in the column.
- Runtime Errors: Check for syntax errors or incorrect logic in the code.
Best Practices for Using VBA to Find Value in Excel Column
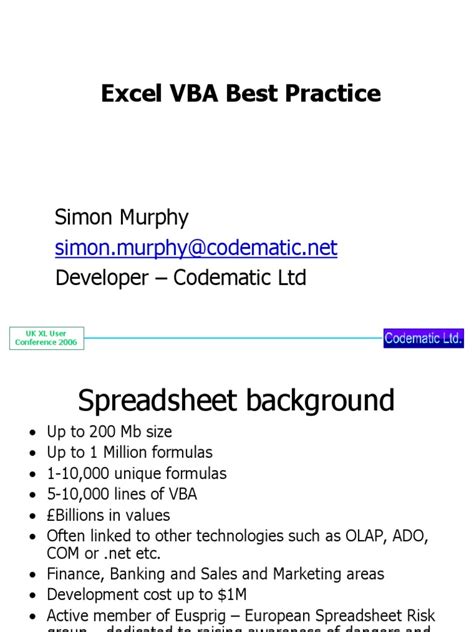
To ensure efficient and effective use of VBA to find value in Excel column, follow these best practices:
- Use Meaningful Variable Names: Use descriptive variable names to improve code readability.
- Comment Your Code: Add comments to explain the logic and purpose of the code.
- Test Thoroughly: Test the code with different inputs and edge cases to ensure accuracy.
- Optimize Performance: Use efficient algorithms and data structures to optimize performance.
Gallery of Excel VBA Column Search Examples
Excel VBA Column Search Examples
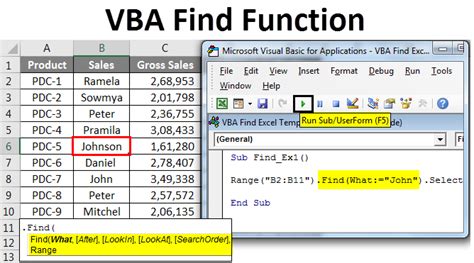
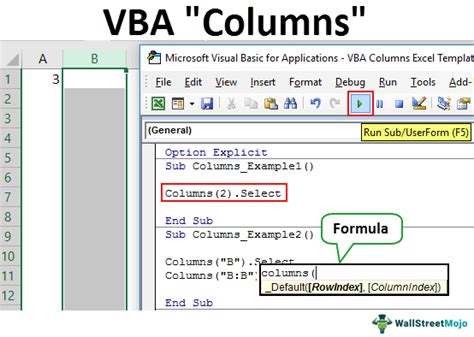
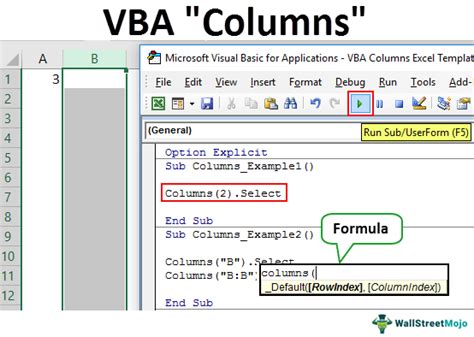
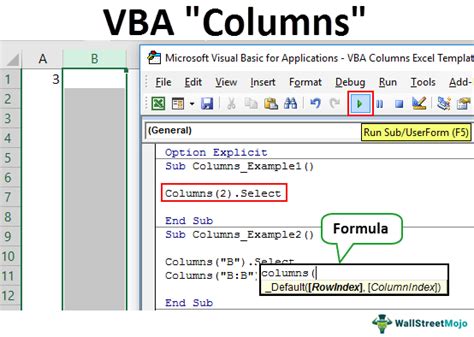
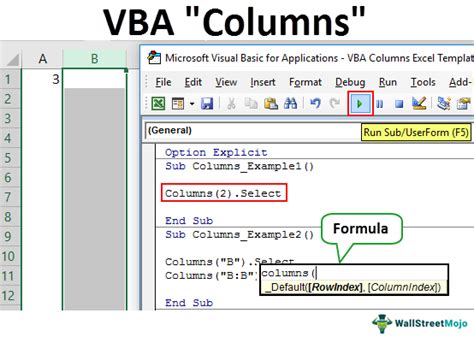
In conclusion, finding a value in an Excel column using VBA is a powerful technique that can save you time and improve productivity. By following the best practices and using advanced techniques, you can create efficient and effective VBA code to meet your needs.
We hope this article has provided you with valuable insights and examples to help you master VBA column search. If you have any questions or need further assistance, please don't hesitate to comment below.