Intro
Master formatting in VBA with our expert guide. Learn 3 easy ways to format numbers to 2 decimal places, including using the Format function, NumberFormat property, and VBA rounding functions. Improve your Excel skills and precision with these simple yet powerful techniques.
Formatting numbers to display a specific number of decimal places is a common task in Excel VBA programming. Displaying two decimal places is particularly useful for monetary values or other quantities where precision to two decimal places is sufficient. Here are three ways to format numbers to 2 decimal places in VBA:
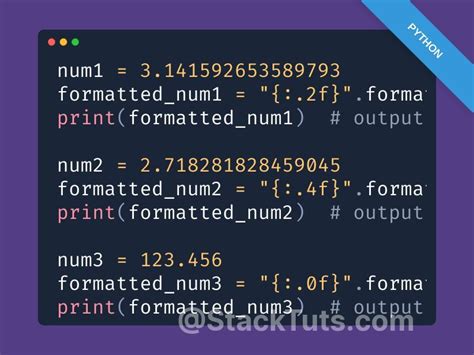
1. Using the Format
Function
The Format
function is one of the most straightforward methods to format a number to 2 decimal places. This function allows you to specify a format string that defines how the number should be displayed.
Dim myNumber As Double
myNumber = 10.1234
Dim formattedNumber As String
formattedNumber = Format(myNumber, "0.00")
Debug.Print formattedNumber ' Outputs: 10.12
In the format string "0.00"
, the 0
before the decimal point is a digit placeholder that will display all digits from the left, and the 00
after the decimal point specifies that you want two decimal places. If the number has fewer than two decimal places, the remaining places will be padded with zeros.
2. Using the Round
Function with Format
While the Format
function changes the display of the number without altering its underlying value, using the Round
function in conjunction with Format
ensures that the number itself is rounded to two decimal places before formatting. This can prevent issues where calculations might rely on the formatted (not the actual) value.
Dim myNumber As Double
myNumber = 10.1234
myNumber = Round(myNumber, 2) ' Rounds to 10.12
Dim formattedNumber As String
formattedNumber = Format(myNumber, "0.00")
Debug.Print formattedNumber ' Outputs: 10.12
Rounding the number before formatting ensures that any further calculations will be based on the rounded value.
3. Using the NumberFormat
Property
If you are working with Excel cells directly and want to format the cell's value to display two decimal places, you can use the NumberFormat
property. This method doesn't require converting the number to a string but rather changes how the value is displayed in the cell.
Dim rng As Range
Set rng = Range("A1")
rng.Value = 10.1234
rng.NumberFormat = "0.00"
Debug.Print rng.Text ' Outputs: 10.12
This method is particularly useful when you want to change the display format of many cells at once or when working with Excel worksheets directly from VBA.
Choosing the Right Method
- Use the
Format
function when you need to display a number in a specific format but don't necessarily need to change the underlying value. - Use
Round
followed byFormat
if you're working in a context where the actual value needs to be rounded before being formatted for display or further calculation. - Use the
NumberFormat
property when working directly with Excel cells and you want to change the display format without converting the value to a string.
Each of these methods serves a slightly different purpose, but they all contribute to maintaining clear and accurate numerical displays in your VBA applications.
Image Gallery for VBA Formatting
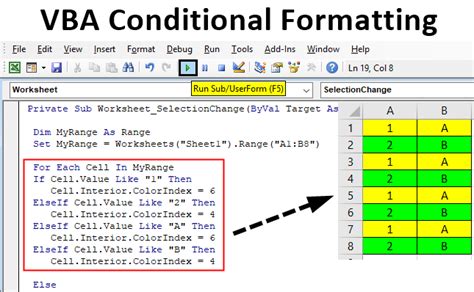
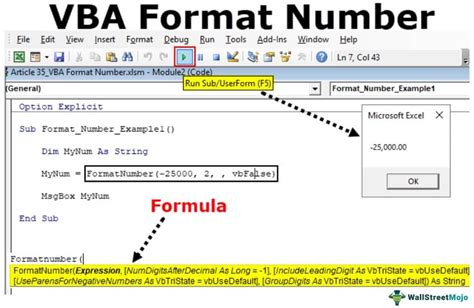
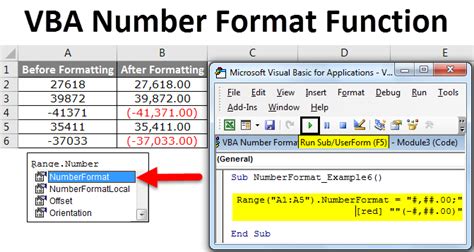
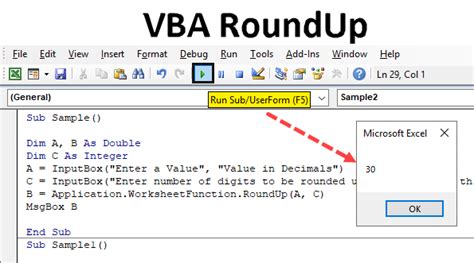
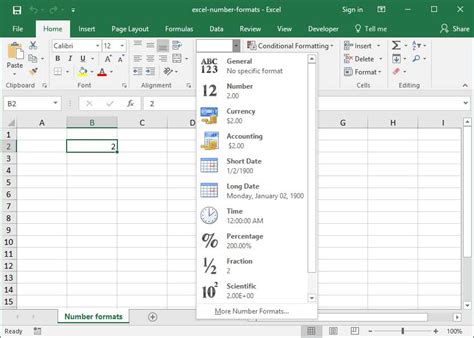
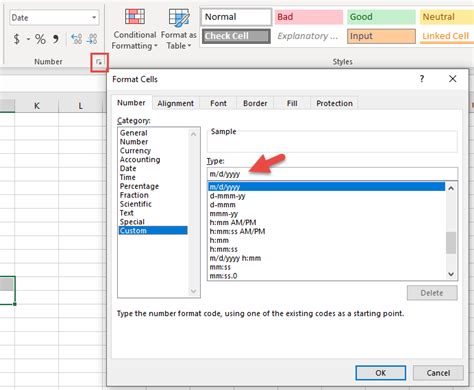
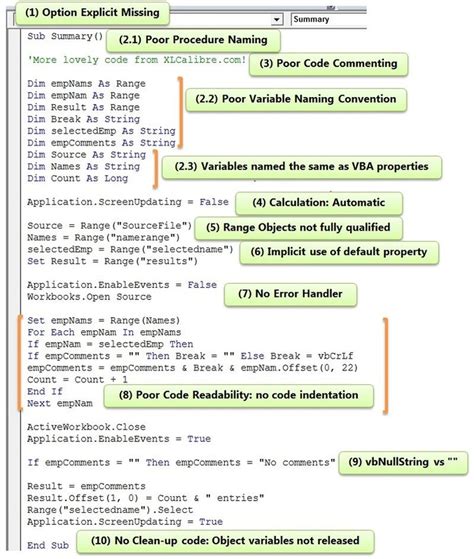
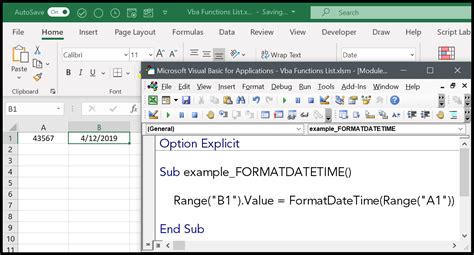
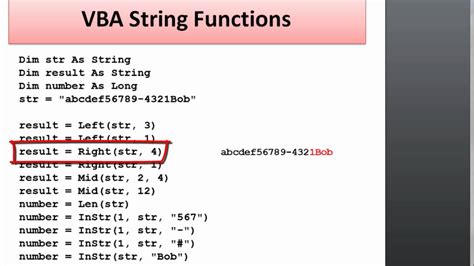
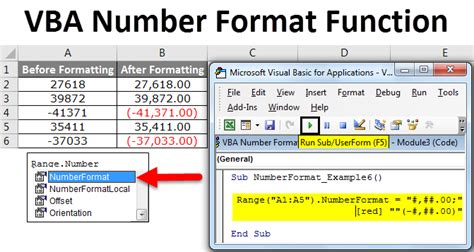
Feel free to ask more questions or explore other topics related to VBA and Excel.