Intro
Master VBA functions with 5 effective ways to return values. Learn how to use Function Return Statements, ByVal and ByRef arguments, and more to efficiently retrieve data from your VBA code. Improve your Visual Basic programming skills and discover best practices for handling function outputs with ease.
Returning values from VBA functions is a crucial aspect of programming in Visual Basic for Applications. When you write a function in VBA, it's essential to understand how to return values to the calling procedure. In this article, we'll explore five different ways to return values from VBA functions, along with examples and best practices.
Understanding VBA Functions
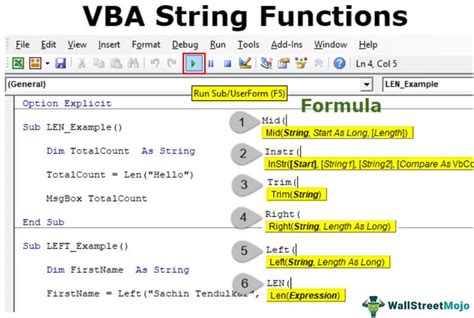
Before we dive into the methods of returning values, let's quickly review what VBA functions are. A VBA function is a block of code that takes arguments, performs calculations or actions, and returns a value. Functions are useful for encapsulating code that performs a specific task, making your code more modular and reusable.
Method 1: Using the Return Statement
One of the most common ways to return a value from a VBA function is by using the Return
statement. This statement is used to exit the function and return a value to the calling procedure.
Example:
Function AddNumbers(a As Integer, b As Integer) As Integer
AddNumbers = a + b
End Function
In this example, the AddNumbers
function takes two integer arguments, adds them together, and returns the result using the Return
statement.
Best Practice: Using the Return Statement
When using the Return
statement, it's essential to assign the return value to the function name, as shown in the example above. This ensures that the function returns the correct value.
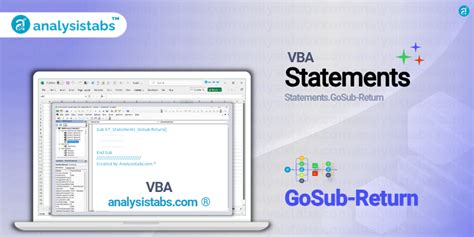
Method 2: Using an Output Parameter
Another way to return values from a VBA function is by using an output parameter. This method involves passing an argument to the function by reference, which allows the function to modify the argument's value.
Example:
Sub AddNumbers(a As Integer, b As Integer, result As Integer)
result = a + b
End Sub
In this example, the AddNumbers
function takes three arguments: two input integers and an output integer. The function adds the input integers together and assigns the result to the output integer.
Best Practice: Using Output Parameters
When using output parameters, it's essential to pass the argument by reference using the ByRef
keyword. This ensures that the function can modify the argument's value.
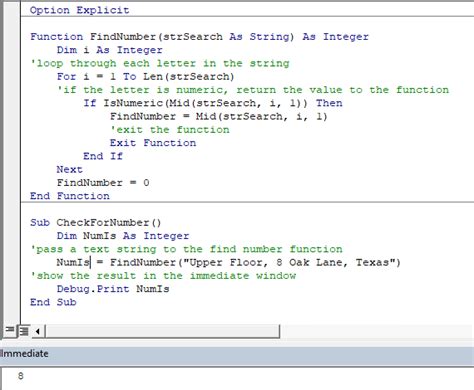
Method 3: Using a Collection or Array
If you need to return multiple values from a VBA function, you can use a collection or array. This method involves creating a collection or array within the function and populating it with values.
Example:
Function GetNumbers() As Variant
Dim numbers As New Collection
numbers.Add 1
numbers.Add 2
numbers.Add 3
GetNumbers = numbers
End Function
In this example, the GetNumbers
function creates a collection, adds three numbers to it, and returns the collection as a variant.
Best Practice: Using Collections or Arrays
When using collections or arrays, it's essential to declare the return type as Variant
to ensure compatibility with different data types.
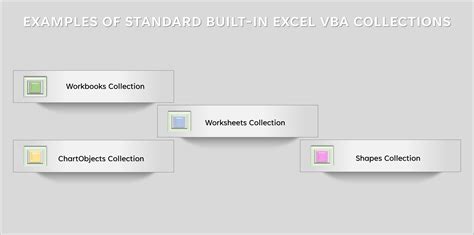
Method 4: Using a Class
If you need to return complex data from a VBA function, you can use a class. This method involves creating a class module, defining properties and methods, and instantiating the class within the function.
Example:
Class Person
Public Name As String
Public Age As Integer
End Class
Function GetPerson() As Person
Dim person As New Person
person.Name = "John"
person.Age = 30
GetPerson = person
End Function
In this example, the GetPerson
function creates a new instance of the Person
class, sets its properties, and returns the object.
Best Practice: Using Classes
When using classes, it's essential to define the class module separately and instantiate the class within the function.
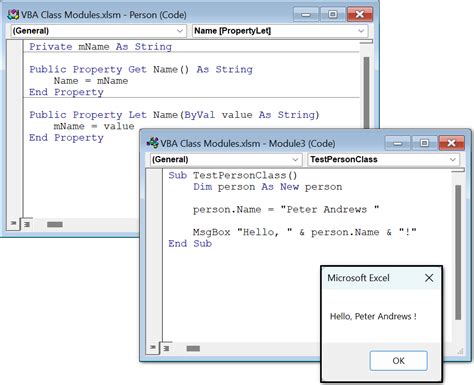
Method 5: Using a DataTable
If you need to return tabular data from a VBA function, you can use a DataTable
. This method involves creating a DataTable
object, populating it with data, and returning it as a variant.
Example:
Function GetDataTable() As Variant
Dim dataTable As New DataTable
dataTable.Columns.Add "Name"
dataTable.Columns.Add "Age"
dataTable.Rows.Add "John", 30
dataTable.Rows.Add "Jane", 25
GetDataTable = dataTable
End Function
In this example, the GetDataTable
function creates a new DataTable
object, adds columns and rows, and returns it as a variant.
Best Practice: Using DataTables
When using DataTables
, it's essential to declare the return type as Variant
to ensure compatibility with different data types.
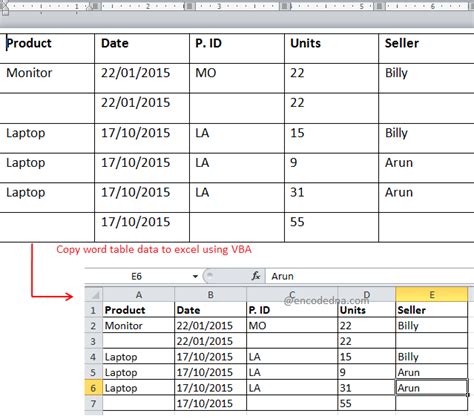
Gallery of VBA Functions
VBA Functions Image Gallery
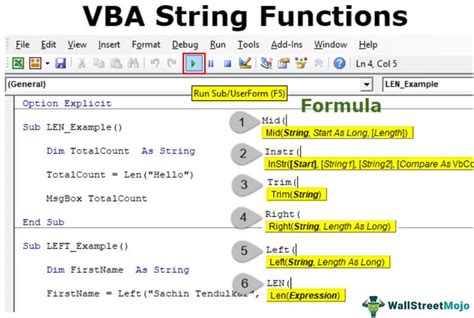
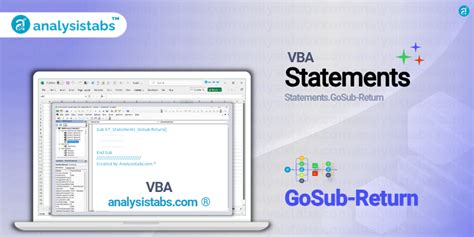
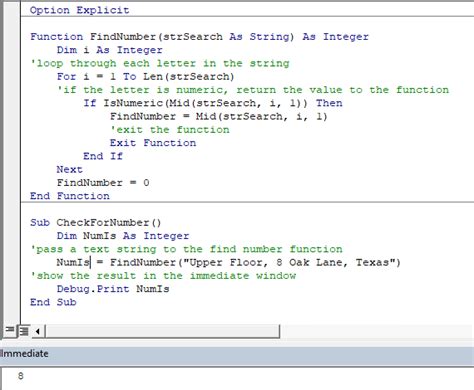
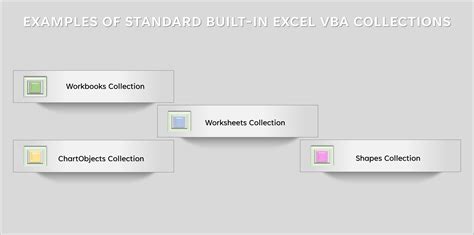
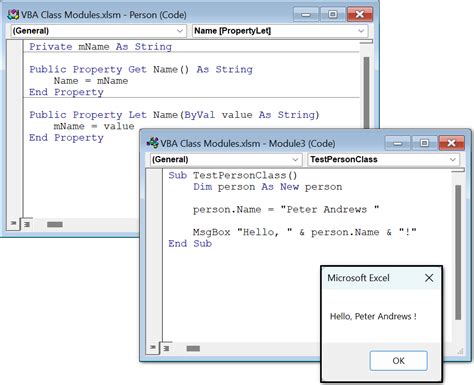
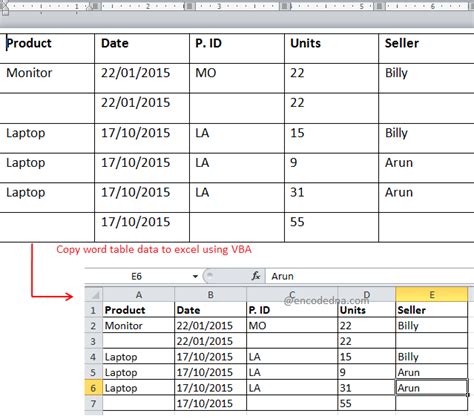
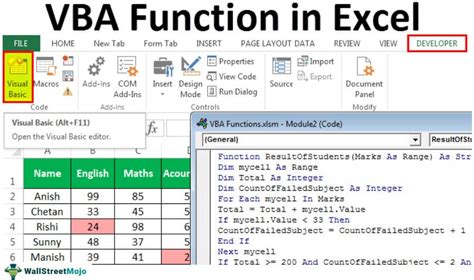
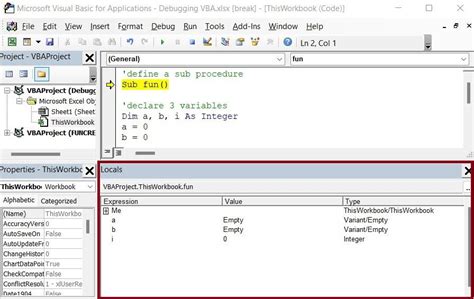
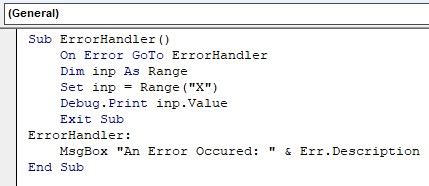
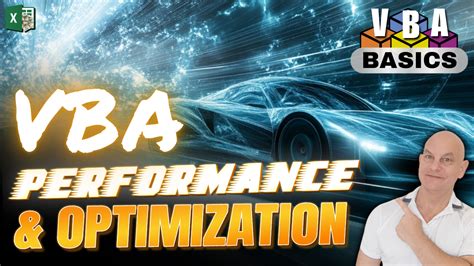
We hope this article has provided you with a comprehensive understanding of the different ways to return values from VBA functions. Whether you're a beginner or an experienced developer, mastering VBA functions is essential for creating efficient and effective code. By following the best practices outlined in this article, you'll be able to write more robust and maintainable code.
If you have any questions or need further clarification on any of the topics discussed in this article, please don't hesitate to ask. We're always here to help.