Intro
Discover the easiest ways to find the last row in Excel using VBA. Learn how to use the VBA Get Last Row method to automate tasks, improve efficiency, and avoid errors. Explore various solutions, including using the Range.Find method, Range.End, and Cells.Find properties, to master Excel VBA programming.
Finding the last row in an Excel spreadsheet can be a daunting task, especially for those who are new to using VBA (Visual Basic for Applications). However, with the right techniques, you can easily identify the last row and utilize it in your VBA code. In this article, we will explore the various methods to find the last row in Excel using VBA, including the most efficient and reliable solutions.
Understanding the Problem
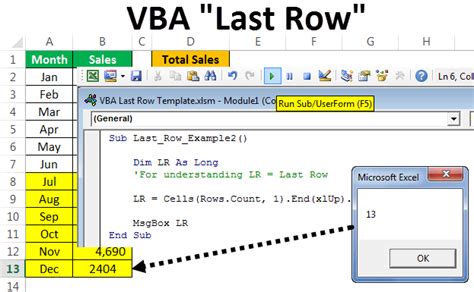
When working with Excel spreadsheets, it's common to encounter situations where you need to find the last row that contains data. This can be particularly challenging when dealing with large datasets or dynamic ranges. Manually scrolling through the spreadsheet to find the last row can be time-consuming and prone to errors. Fortunately, VBA provides several methods to find the last row quickly and accurately.
Method 1: Using the `Cells.Find` Method
One of the most common methods to find the last row is by using the `Cells.Find` method. This method searches for a specific value or format in the spreadsheet and returns the range of the cell that matches the criteria.Sub FindLastRow()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Cells.Find("*", searchorder:=xlByRows, searchdirection:=xlPrevious).Row
MsgBox "The last row is: " & lastRow
End Sub
This method is efficient, but it may not work as expected if the spreadsheet contains formatting or conditional formatting that can interfere with the search.
Method 2: Using the `Range.SpecialCells` Method
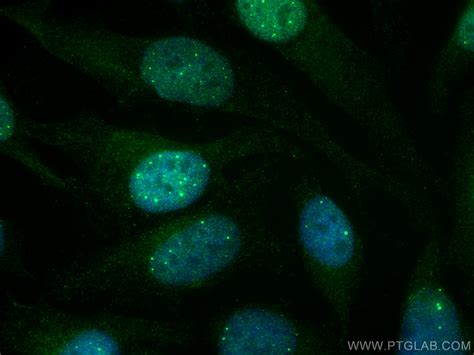
Another method to find the last row is by using the Range.SpecialCells
method. This method returns a range of cells that meet specific criteria, such as blank cells or cells with formulas.
Sub FindLastRow()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Cells.SpecialCells(xlCellTypeLastCell).Row
MsgBox "The last row is: " & lastRow
End Sub
This method is reliable and efficient, but it may not work if the spreadsheet contains a large number of blank cells.
Method 3: Using the `Range.Rows.Count` Property
A simpler method to find the last row is by using the `Range.Rows.Count` property. This method returns the number of rows in a range, which can be used to find the last row.Sub FindLastRow()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Range("A" & ws.Rows.Count).End(xlUp).Row
MsgBox "The last row is: " & lastRow
End Sub
This method is efficient and reliable, but it may not work if the spreadsheet contains a large number of blank cells.
Best Practices and Tips
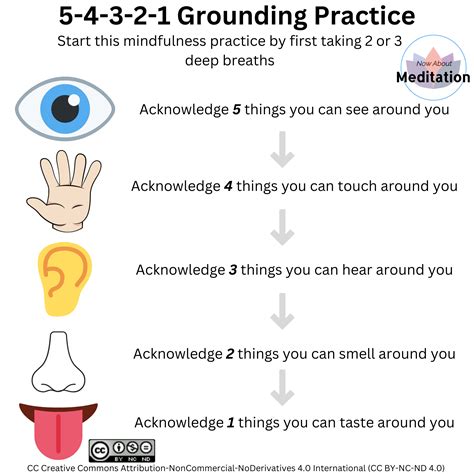
When finding the last row in Excel using VBA, there are several best practices and tips to keep in mind:
- Always specify the worksheet object when finding the last row to avoid errors.
- Use the
Range.SpecialCells
method instead ofCells.Find
to avoid interference from formatting or conditional formatting. - Use the
Range.Rows.Count
property to find the last row when dealing with large datasets. - Avoid using
Select
orActivate
methods when finding the last row, as they can slow down the code and cause errors.
Common Errors and Solutions
When finding the last row in Excel using VBA, you may encounter several errors, including:- Error 1004: "Method 'Find' of object 'Range' failed". Solution: Check if the spreadsheet contains formatting or conditional formatting that can interfere with the search.
- Error 91: "Object variable or With block variable not set". Solution: Check if the worksheet object is properly set before finding the last row.
- Error 13: "Type mismatch". Solution: Check if the variable type is correctly declared before finding the last row.
By following the best practices and tips outlined above, you can avoid these errors and efficiently find the last row in your Excel spreadsheet using VBA.
Conclusion
Finding the last row in an Excel spreadsheet can be a challenging task, but with the right techniques and best practices, you can easily identify the last row and utilize it in your VBA code. In this article, we explored the various methods to find the last row, including the `Cells.Find` method, `Range.SpecialCells` method, and `Range.Rows.Count` property. By following the tips and best practices outlined above, you can efficiently find the last row and take your VBA skills to the next level.Last Row Image Gallery
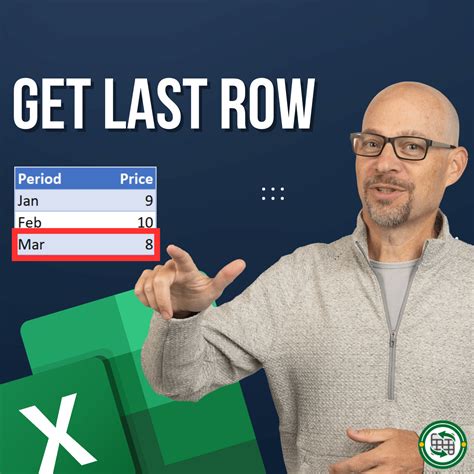
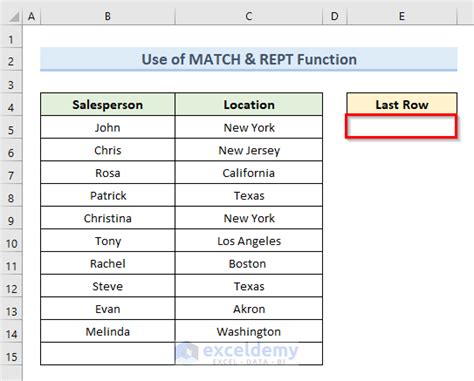
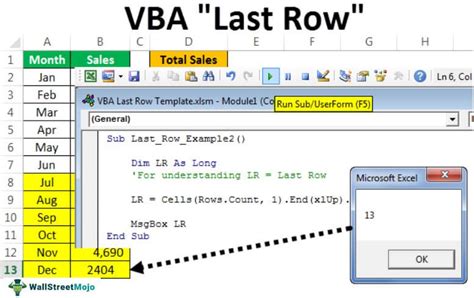
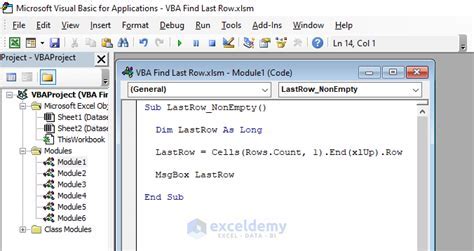
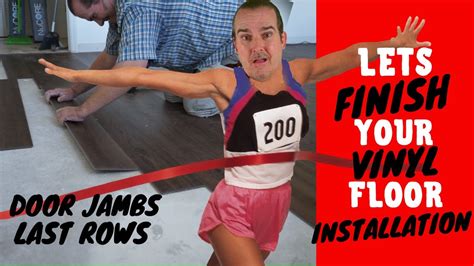
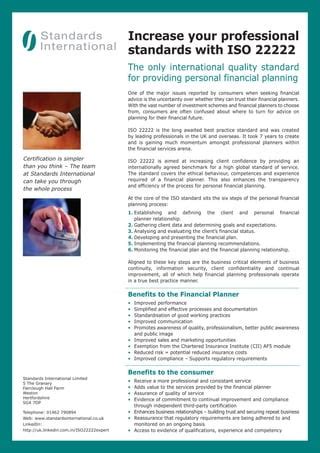
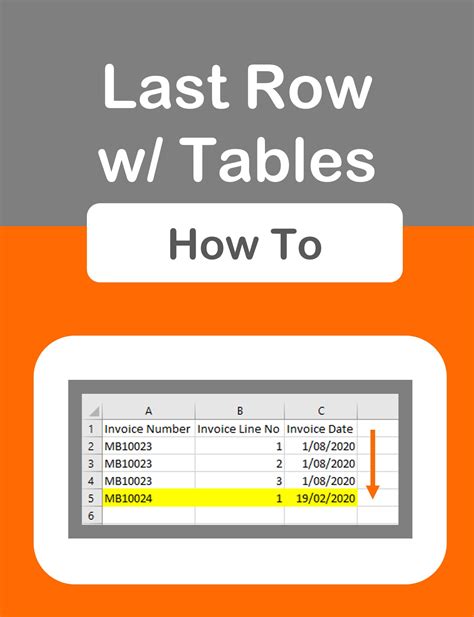
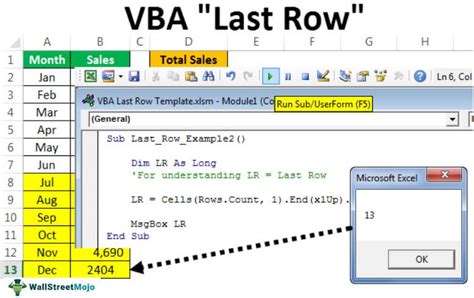
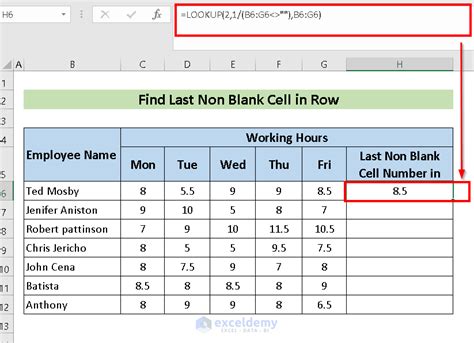
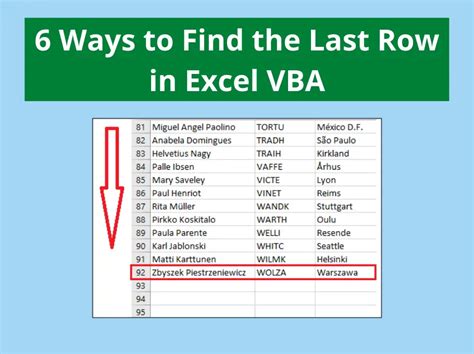
We hope this article has provided you with the necessary knowledge and skills to find the last row in your Excel spreadsheet using VBA. If you have any further questions or need help with implementing these methods, please leave a comment below.