Intro
Discover how to find the last row in Excel VBA with ease. Learn efficient methods to determine the last row with data using VBA code, including using the End property, Find method, and SpecialCells approach. Master Excel automation and streamline your workflows with these simple yet powerful VBA techniques.
Finding the last row in Excel VBA is a common task that can be accomplished in several ways, depending on the specific requirements of your project. The method you choose will often depend on the layout of your data, including whether it is in a table format, if there are blank rows or columns, and whether you are working with a specific range or the entire worksheet.
Why Finding the Last Row is Important
Determining the last row with data in Excel VBA is crucial for many operations, such as:
- Looping through a range of cells to perform actions on each row.
- Copying or moving data from one range to another.
- Avoiding errors by ensuring you're working within the bounds of your data.
- Optimizing code by reducing the number of iterations in loops.
Methods to Find the Last Row
1. Using the End
Property
One of the simplest and most commonly used methods to find the last row with data is by utilizing the End
property in combination with the xlDown
parameter. This method is effective for finding the last row in a continuous range of data starting from a specific cell, often A1.
Sub FindLastRow_EndProperty()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
MsgBox "The last row with data is: " & lastRow
End Sub
This method works well when you have a solid block of data. However, if your data has gaps, it might not give you the expected results.
2. Using the UsedRange
Property
Another method is to use the UsedRange
property, which returns the range of cells that have been used in a worksheet. This can be useful but might include blank rows if your data has gaps.
Sub FindLastRow_UsedRange()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lastRow As Long
lastRow = ws.UsedRange.Rows(ws.UsedRange.Rows.Count).Row
MsgBox "The last row with data is: " & lastRow
End Sub
3. Using the Find
Method
The Find
method can also be used to find the last row with data, particularly useful when you're looking for a specific value or format in your data.
Sub FindLastRow_FindMethod()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Cells.Find("*", searchorder:=xlByRows, searchdirection:=xlPrevious).Row
MsgBox "The last row with data is: " & lastRow
End Sub
4. Using Range
with xlCellTypeLastCell
For more precise control or when working with specific ranges, you can use a combination of Range
and xlCellTypeLastCell
.
Sub FindLastRow_Range()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Cells.Find("*", searchorder:=xlByRows, searchdirection:=xlPrevious).Row
'Or use ws.Cells.SpecialCells(xlCellTypeLastCell).Row
MsgBox "The last row with data is: " & lastRow
End Sub
Choosing the Right Method
- Continuous Data Blocks: The
End
property withxlDown
is efficient. - Gapped Data or Specific Values: Consider using
UsedRange
,Find
, orRange
withxlCellTypeLastCell
. - Performance-Critical Applications: Avoid
UsedRange
if possible, as it can be slower with large datasets.
Final Thoughts
Finding the last row in Excel VBA is a fundamental skill that can significantly simplify and optimize your code. By choosing the right method for your specific task, you can improve the efficiency and reliability of your macros.
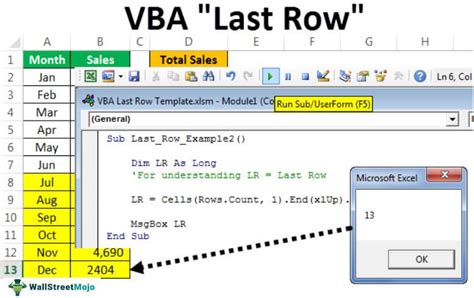
Gallery of Excel VBA Last Row Images
Excel VBA Last Row Gallery
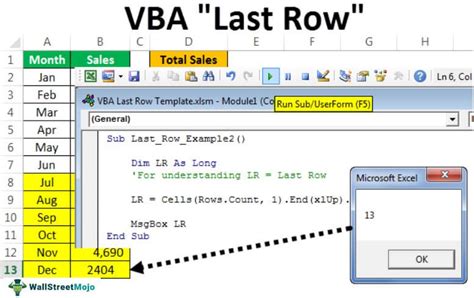
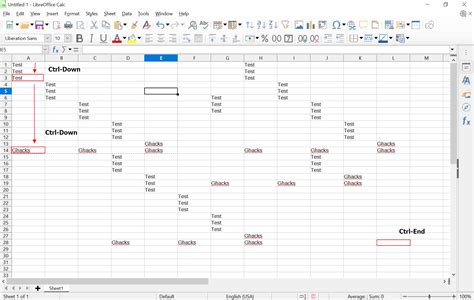
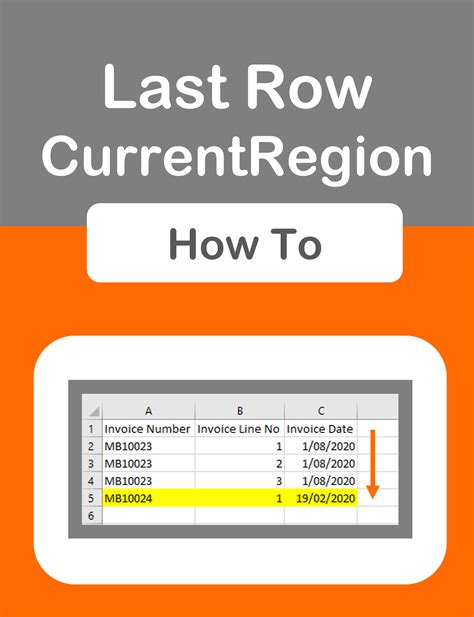
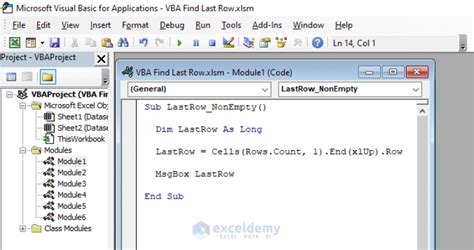
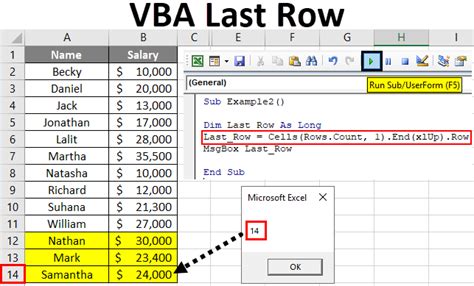
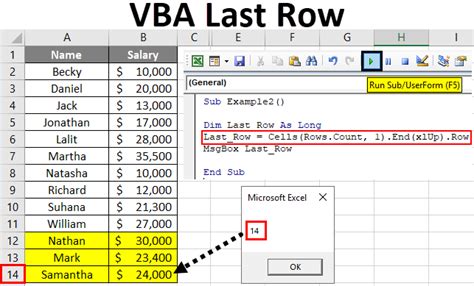
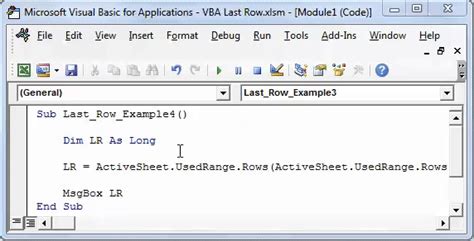
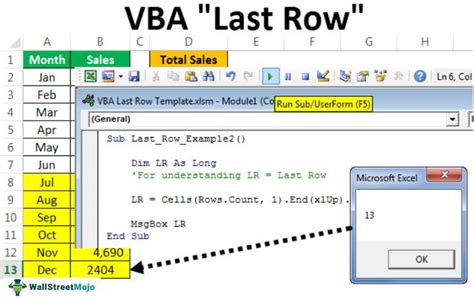
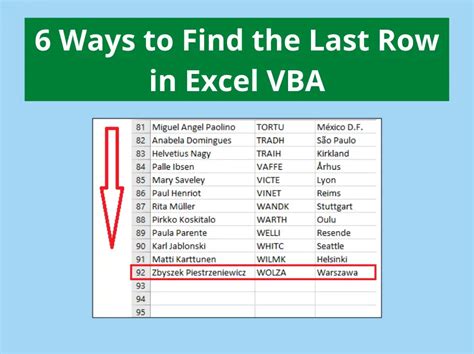
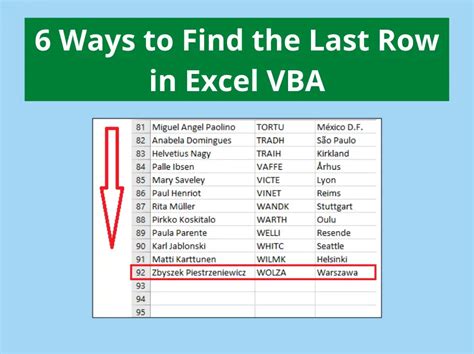
Feel free to comment below with any questions or tips related to finding the last row in Excel VBA!