Intro
Discover how to return values from VBA functions with ease. Learn three effective methods to pass values back to your calling code, including using function return types, ByRef arguments, and class properties. Master VBA functions and improve your Excel automation skills with these practical tips and examples.
Writing efficient and effective VBA functions is crucial for any Excel developer. One of the most fundamental aspects of VBA functions is returning values. In this article, we will explore three ways to return values from VBA functions, providing you with the knowledge to improve your coding skills.
VBA functions are an essential part of automating tasks in Excel. By creating reusable blocks of code, you can simplify complex tasks and make your worksheets more efficient. However, without the ability to return values, VBA functions would be severely limited. Fortunately, VBA provides several ways to return values from functions, and we will examine three of the most common methods.
Understanding VBA Functions
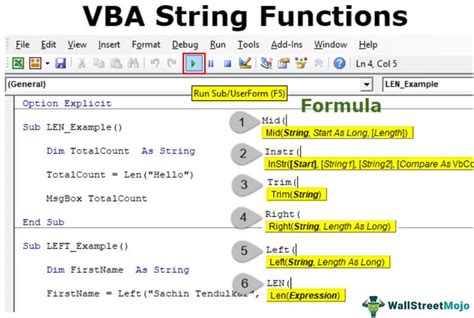
Before we dive into the three ways to return values from VBA functions, let's quickly review the basics of VBA functions. A VBA function is a block of code that performs a specific task and returns a value. Functions can take arguments, which are values passed to the function when it is called. The function can then use these arguments to perform calculations or execute tasks.
Function Syntax
The basic syntax of a VBA function is as follows:
Function FunctionName(arg1 As DataType, arg2 As DataType) As ReturnType
' Code to execute
End Function
In this syntax, FunctionName
is the name of the function, arg1
and arg2
are the arguments passed to the function, and ReturnType
is the data type of the value returned by the function.
Method 1: Using the Return Statement
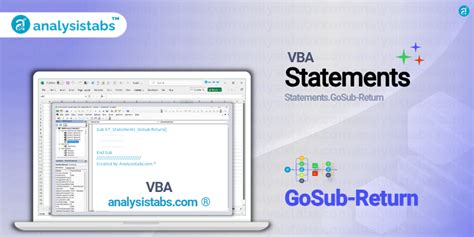
The first method for returning values from VBA functions is by using the Return
statement. This statement is used to specify the value that the function should return.
For example, consider the following function:
Function AddNumbers(a As Integer, b As Integer) As Integer
AddNumbers = a + b
End Function
In this function, the Return
statement is not explicitly used. Instead, the value of a + b
is assigned to the function name AddNumbers
. This is a common way to return values from VBA functions.
However, you can also use the Return
statement explicitly, like this:
Function AddNumbers(a As Integer, b As Integer) As Integer
Return a + b
End Function
Both methods are equivalent and will return the same value.
Method 2: Using an Output Parameter
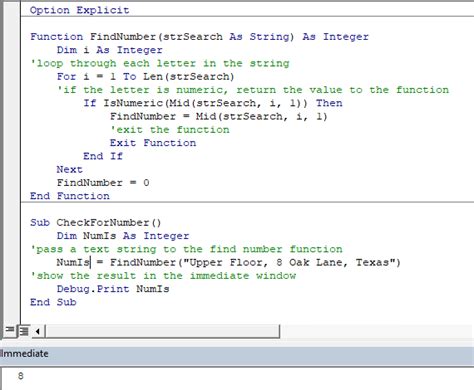
The second method for returning values from VBA functions is by using an output parameter. An output parameter is a variable that is passed to the function by reference, allowing the function to modify its value.
For example, consider the following function:
Sub AddNumbers(a As Integer, b As Integer, ByRef result As Integer)
result = a + b
End Sub
In this function, the result
parameter is declared as ByRef
, which means that it is passed to the function by reference. The function can then modify the value of result
, which will be reflected in the calling code.
To call this function and retrieve the result, you would use the following code:
Dim result As Integer
AddNumbers 2, 3, result
Debug.Print result
This code calls the AddNumbers
function, passing 2
and 3
as arguments, and result
as the output parameter. The function modifies the value of result
, which is then printed to the debug console.
Method 3: Using a Class Property
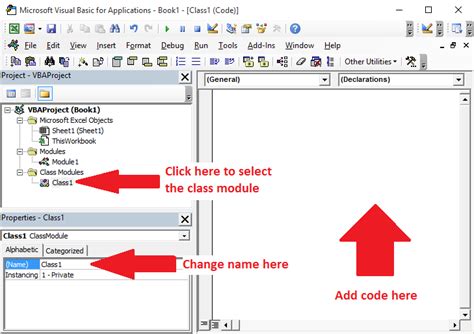
The third method for returning values from VBA functions is by using a class property. A class property is a variable that is defined within a class module and can be accessed through an instance of the class.
For example, consider the following class module:
Public MyClass
Private pResult As Integer
Public Function AddNumbers(a As Integer, b As Integer) As MyClass
pResult = a + b
Set AddNumbers = Me
End Function
Public Property Get Result() As Integer
Result = pResult
End Property
End Class
In this class module, the AddNumbers
function is used to calculate the sum of two numbers and store the result in the pResult
variable. The Result
property is then used to retrieve the value of pResult
.
To use this class and retrieve the result, you would use the following code:
Dim obj As New MyClass
Set obj = obj.AddNumbers(2, 3)
Debug.Print obj.Result
This code creates an instance of the MyClass
class and calls the AddNumbers
function, passing 2
and 3
as arguments. The Result
property is then used to retrieve the value of pResult
, which is printed to the debug console.
VBA Functions Image Gallery
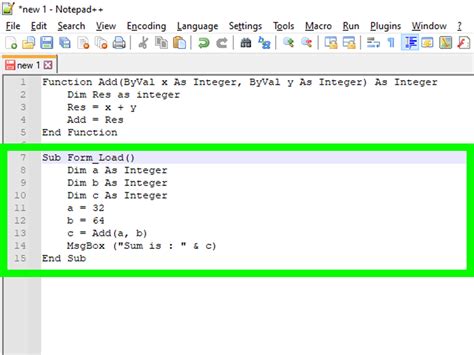
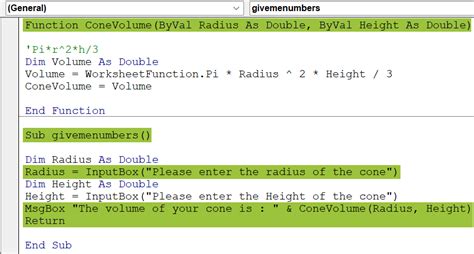
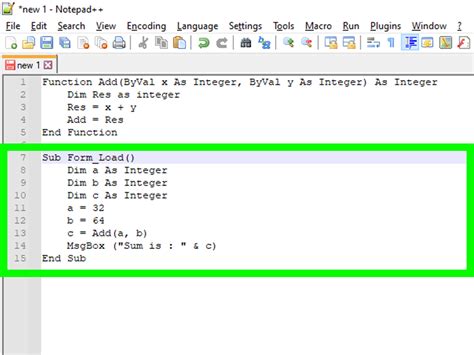
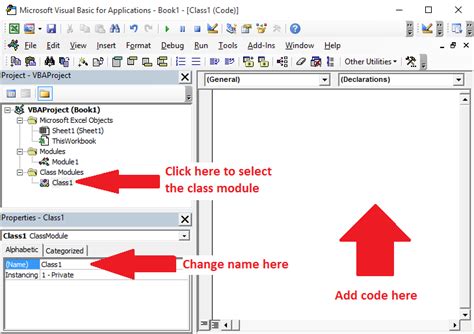
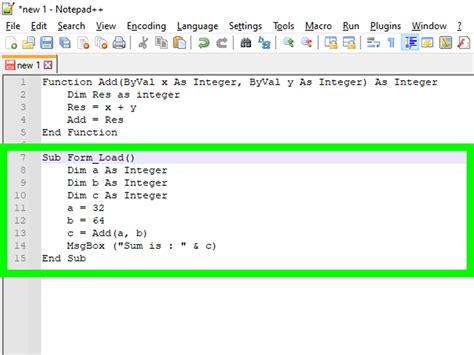
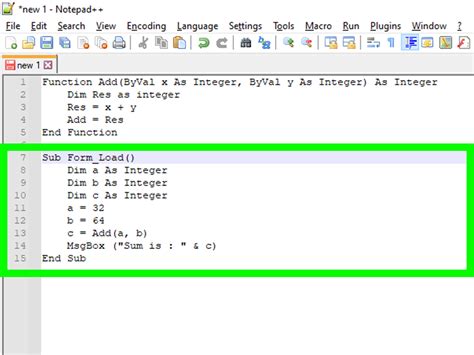
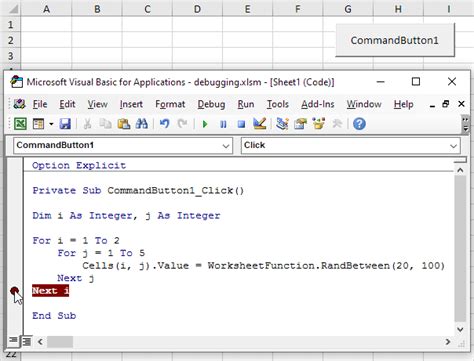
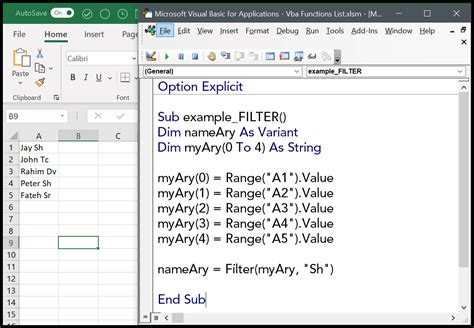
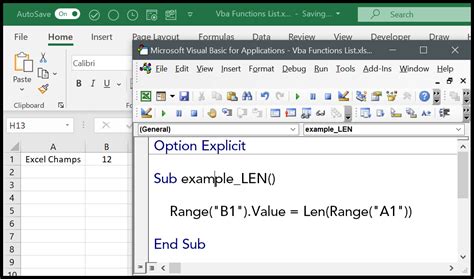
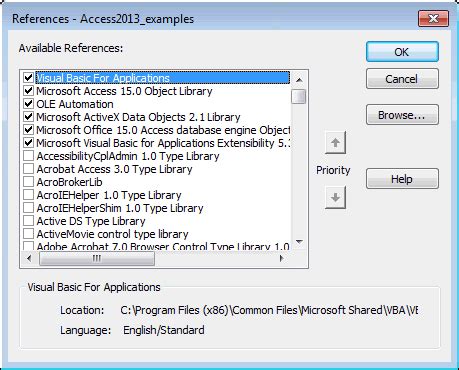
In conclusion, returning values from VBA functions is an essential aspect of Excel development. By using the Return
statement, output parameters, or class properties, you can create efficient and effective functions that simplify complex tasks. Whether you're a seasoned developer or just starting out, mastering these techniques will take your VBA skills to the next level.
We hope this article has provided you with a comprehensive understanding of the three ways to return values from VBA functions. If you have any questions or need further clarification, please don't hesitate to ask. Share your thoughts and experiences in the comments below, and don't forget to share this article with your fellow developers. Happy coding!