Intro
Unlock the power of VBA loops with our expert guide. Discover 5 essential ways to master the Loop Do While statement, including syntax, examples, and troubleshooting tips. Improve your Excel automation skills with VBA loop tutorials, error handling, and optimization techniques. Learn how to write efficient VBA code and streamline your workflow today!
VBA loops are an essential part of any VBA programmer's toolkit, and the Loop Do While is one of the most versatile and powerful loops available. In this article, we will explore the ins and outs of the Loop Do While, and provide you with 5 ways to master it.
The Loop Do While is a type of loop that continues to execute a block of code as long as a certain condition is true. It is commonly used to iterate over a range of cells, perform repetitive tasks, and to manipulate data. But, as with any powerful tool, it can be tricky to use effectively.
Here are 5 ways to master the Loop Do While:
Understanding the Syntax
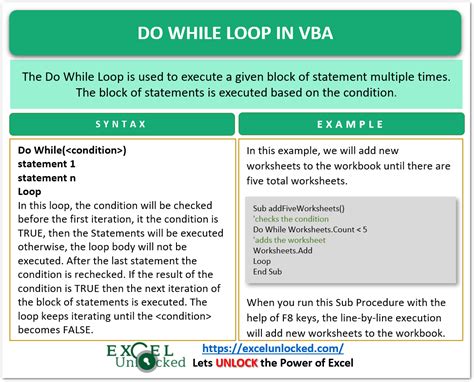
The syntax for a Loop Do While is simple:
Do While [Condition]
[Code to execute]
Loop
The [Condition]
is the key to the Loop Do While. It is the expression that determines whether the loop will continue to execute or not. As long as the condition is true, the loop will continue to execute. When the condition becomes false, the loop will exit.
Example: A Simple Loop Do While
Here is an example of a simple Loop Do While:
Sub SimpleLoopDoWhile()
Dim i As Integer
i = 1
Do While i <= 10
Debug.Print i
i = i + 1
Loop
End Sub
This loop will print the numbers 1 through 10 to the Immediate window.
Using the Loop Do While to Iterate Over a Range of Cells
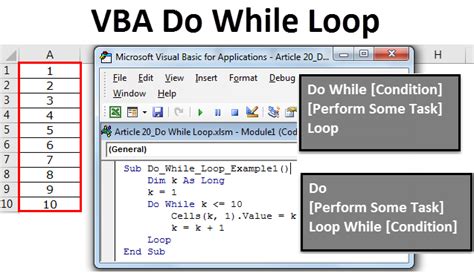
One of the most common uses of the Loop Do While is to iterate over a range of cells. This can be done using the Range
object and the Cells
property.
Sub LoopOverRange()
Dim cell As Range
Dim i As Integer
i = 1
Do While i <= 10
Set cell = Range("A" & i)
Debug.Print cell.Value
i = i + 1
Loop
End Sub
This loop will print the values in cells A1 through A10 to the Immediate window.
Example: Using the Loop Do While to Iterate Over a Range of Cells with a Condition
Here is an example of a Loop Do While that iterates over a range of cells with a condition:
Sub LoopOverRangeWithCondition()
Dim cell As Range
Dim i As Integer
i = 1
Do While i <= 10 And cell.Value <> ""
Set cell = Range("A" & i)
Debug.Print cell.Value
i = i + 1
Loop
End Sub
This loop will print the values in cells A1 through A10 to the Immediate window, as long as the cell value is not empty.
Using the Loop Do While to Perform Repetitive Tasks
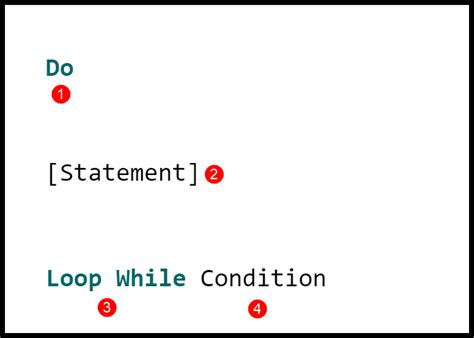
The Loop Do While can also be used to perform repetitive tasks, such as formatting cells or inserting data.
Sub PerformRepetitiveTasks()
Dim i As Integer
i = 1
Do While i <= 10
Range("A" & i).Interior.ColorIndex = 6
i = i + 1
Loop
End Sub
This loop will format the cells in column A to have a yellow background.
Example: Using the Loop Do While to Perform Repetitive Tasks with a Condition
Here is an example of a Loop Do While that performs repetitive tasks with a condition:
Sub PerformRepetitiveTasksWithCondition()
Dim i As Integer
i = 1
Do While i <= 10 And Range("A" & i).Value <> ""
Range("A" & i).Interior.ColorIndex = 6
i = i + 1
Loop
End Sub
This loop will format the cells in column A to have a yellow background, as long as the cell value is not empty.
Using the Loop Do While to Manipulate Data
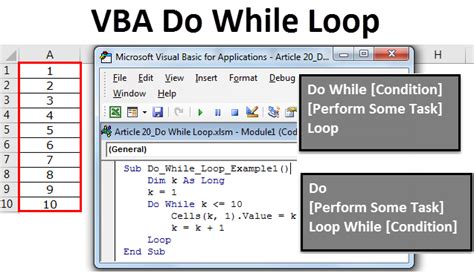
The Loop Do While can also be used to manipulate data, such as copying and pasting values.
Sub ManipulateData()
Dim i As Integer
i = 1
Do While i <= 10
Range("A" & i).Value = Range("B" & i).Value
i = i + 1
Loop
End Sub
This loop will copy the values in column B and paste them into column A.
Example: Using the Loop Do While to Manipulate Data with a Condition
Here is an example of a Loop Do While that manipulates data with a condition:
Sub ManipulateDataWithCondition()
Dim i As Integer
i = 1
Do While i <= 10 And Range("A" & i).Value <> ""
Range("A" & i).Value = Range("B" & i).Value
i = i + 1
Loop
End Sub
This loop will copy the values in column B and paste them into column A, as long as the cell value in column A is not empty.
Conclusion
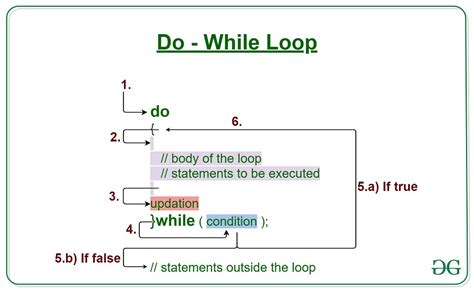
The Loop Do While is a powerful and versatile loop that can be used to iterate over a range of cells, perform repetitive tasks, and manipulate data. By mastering the Loop Do While, you can take your VBA skills to the next level and become more efficient and effective in your work.
We hope this article has provided you with a comprehensive understanding of the Loop Do While and has given you the tools you need to master it.
Do you have any questions about the Loop Do While or any other VBA topic? Please leave a comment below and we will do our best to assist you.
VBA Loop Do While Image Gallery
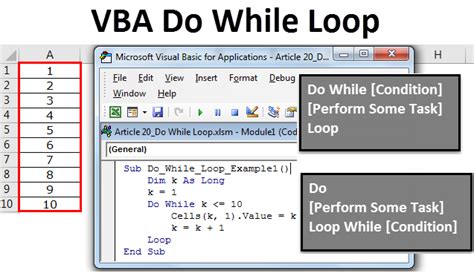
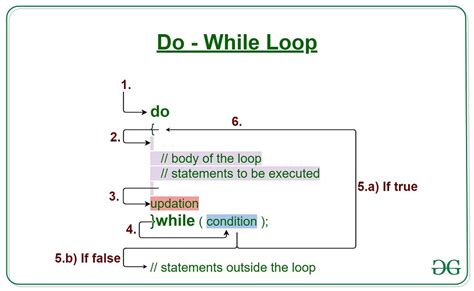
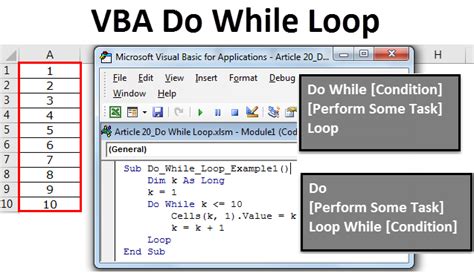
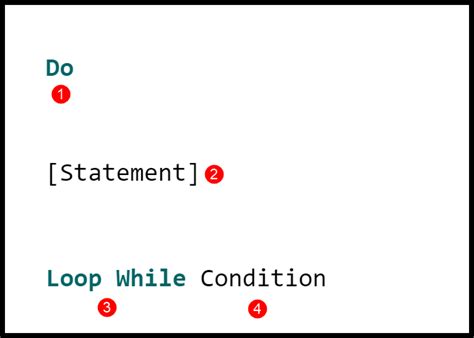
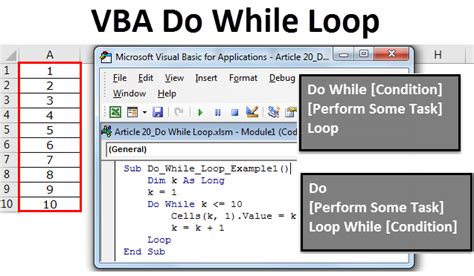
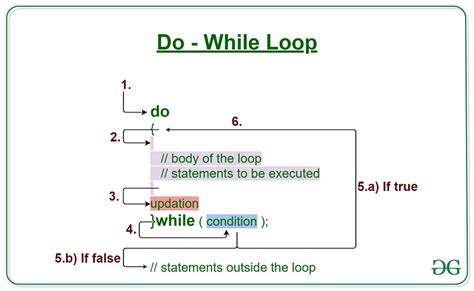
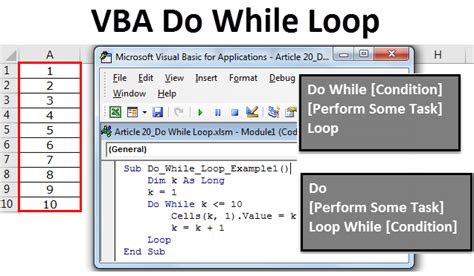
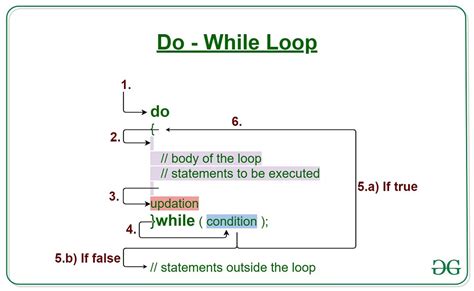
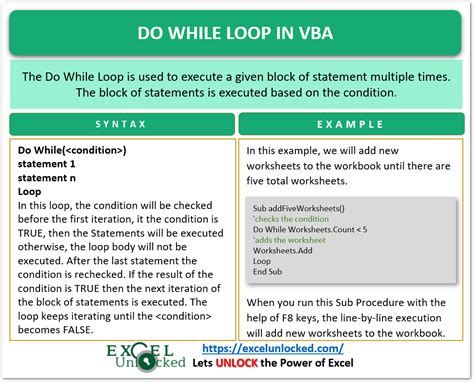
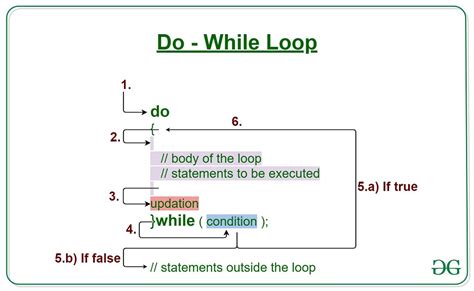
We hope you found this article informative and helpful. Please feel free to share it with your friends and colleagues.