Intro
Master VBA looping techniques with our step-by-step tutorial on how to loop over a range in Excel VBA. Learn to iterate over cells, rows, and columns using For Loops, For Each Loops, and Do Loops. Discover how to manipulate data, automate tasks, and optimize your VBA code with expert tips and examples.
Working with Ranges in VBA: A Comprehensive Guide
Working with ranges is a fundamental aspect of VBA programming in Excel. A range is a cell or a group of cells that can be manipulated as a single unit. In this article, we will explore the various ways to loop over a range in VBA, including using For loops, For Each loops, and Do loops.
Understanding Ranges in VBA
Before we dive into looping over ranges, it's essential to understand how to define and work with ranges in VBA. A range can be defined using the Range object, which can be specified using the range's address, such as "A1:B2" or by using the Cells property, such as Cells(1, 1).
Here's an example of how to define a range in VBA:
Dim myRange As Range
Set myRange = Range("A1:B2")
Looping Over a Range Using For Loops
One of the most common ways to loop over a range is by using a For loop. A For loop allows you to iterate over a range by specifying the starting and ending points of the loop.
Here's an example of how to loop over a range using a For loop:
Dim myRange As Range
Set myRange = Range("A1:B2")
For i = 1 To myRange.Rows.Count
For j = 1 To myRange.Columns.Count
Cells(i, j).Value = "Hello World"
Next j
Next i
In this example, the For loop iterates over the rows and columns of the range, and assigns the value "Hello World" to each cell.
Looping Over a Range Using For Each Loops
Another way to loop over a range is by using a For Each loop. A For Each loop allows you to iterate over a range without having to specify the starting and ending points of the loop.
Here's an example of how to loop over a range using a For Each loop:
Dim myRange As Range
Set myRange = Range("A1:B2")
For Each cell In myRange
cell.Value = "Hello World"
Next cell
In this example, the For Each loop iterates over the cells in the range, and assigns the value "Hello World" to each cell.
Looping Over a Range Using Do Loops
A Do loop is another way to loop over a range in VBA. A Do loop allows you to iterate over a range until a certain condition is met.
Here's an example of how to loop over a range using a Do loop:
Dim myRange As Range
Set myRange = Range("A1:B2")
i = 1
Do Until i > myRange.Rows.Count
For j = 1 To myRange.Columns.Count
Cells(i, j).Value = "Hello World"
Next j
i = i + 1
Loop
In this example, the Do loop iterates over the rows of the range, and assigns the value "Hello World" to each cell.
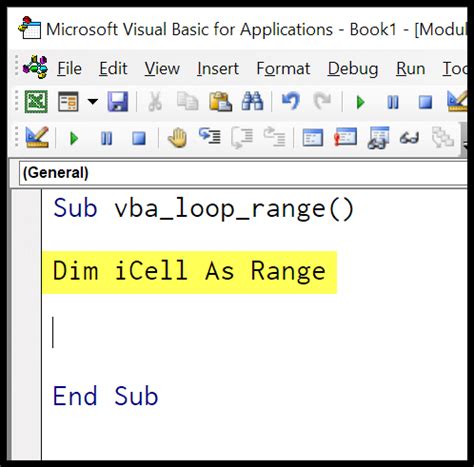
Tips and Best Practices
When looping over a range in VBA, there are several tips and best practices to keep in mind:
- Always define the range explicitly, rather than relying on implicit definitions.
- Use the Range object to specify the range, rather than using the Cells property.
- Use For loops or For Each loops instead of Do loops, unless there is a specific reason to use a Do loop.
- Avoid using Select and Selection when looping over a range, as this can cause performance issues.
Common Errors and Debugging
When looping over a range in VBA, there are several common errors to watch out for:
- Undefined range: Make sure to define the range explicitly before looping over it.
- Out of range error: Make sure to check the range bounds before looping over it.
- Infinite loop: Make sure to specify a condition for the loop to exit, otherwise it will run indefinitely.
To debug your code, use the VBA debugger to step through the code and identify the error.
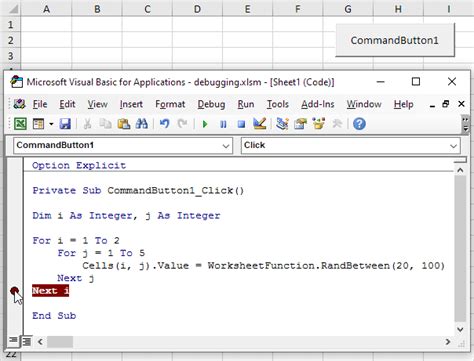
Conclusion
Looping over a range is a fundamental aspect of VBA programming in Excel. By using For loops, For Each loops, and Do loops, you can iterate over a range and perform tasks on each cell. Remember to define the range explicitly, use the Range object, and avoid using Select and Selection. With these tips and best practices, you'll be able to write efficient and effective VBA code that loops over ranges with ease.
Gallery of VBA Loop Over Range
VBA Loop Over Range Gallery
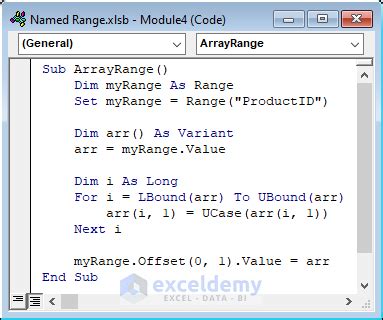
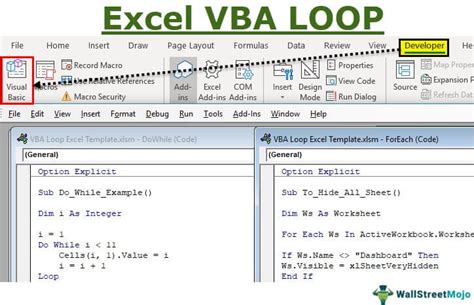
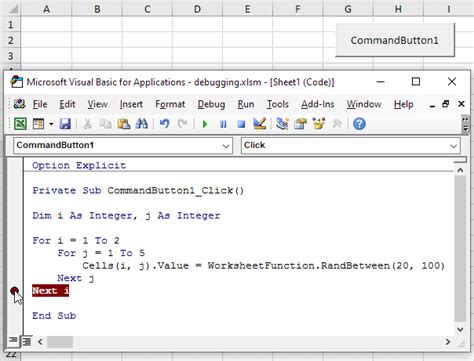
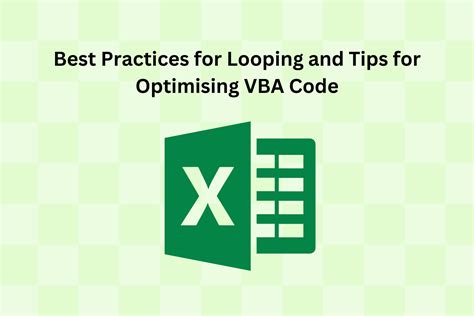
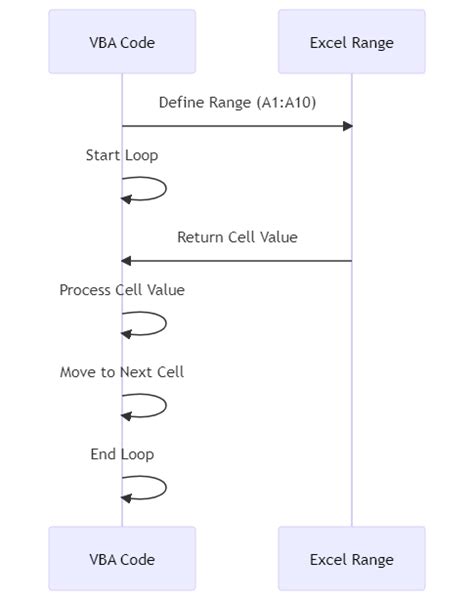
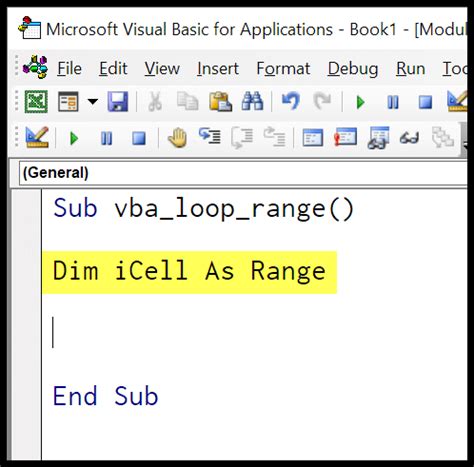
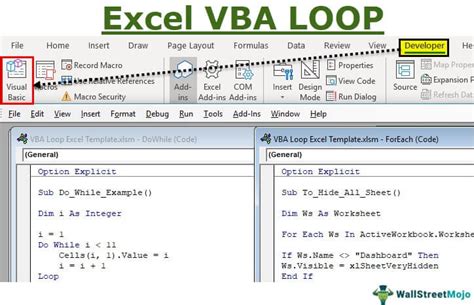
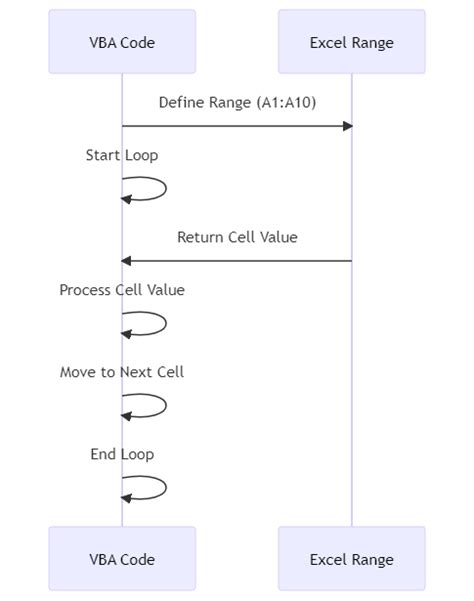
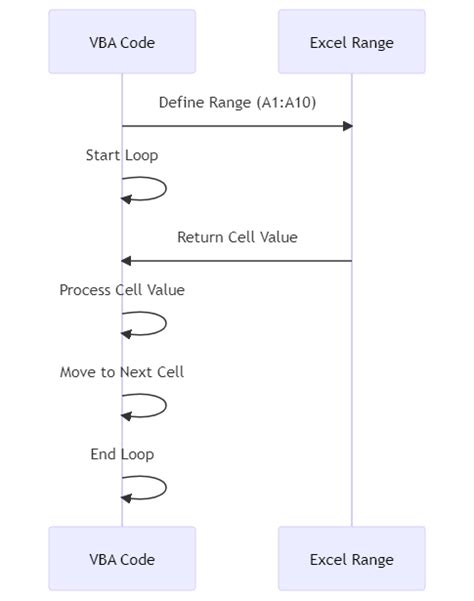
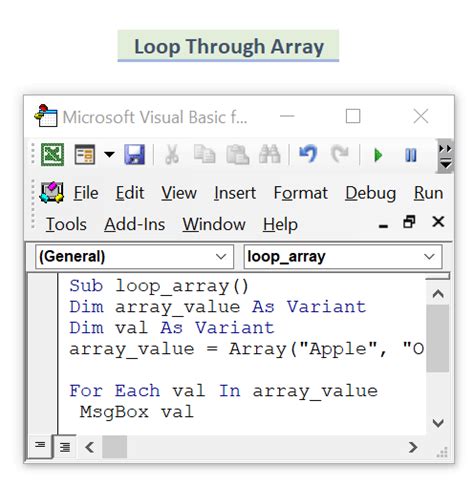