Intro
Master VBA sheet looping techniques with our expert guide. Discover 5 efficient ways to loop through sheets in VBA, including using For Each, For Next, and Do While loops. Learn how to navigate worksheets, workbooks, and Excel sheets with ease, and boost your VBA programming skills with practical examples and code snippets.
Working with multiple sheets in Excel can be a daunting task, especially when it comes to automating tasks using VBA. Looping through sheets is a common requirement for many Excel VBA projects. In this article, we will explore five different ways to loop through sheets in VBA, highlighting the benefits and drawbacks of each method.
The Importance of Looping Through Sheets in VBA
Before we dive into the different methods, it's essential to understand why looping through sheets is crucial in VBA. When working with multiple sheets, you may need to perform tasks such as:
- Formatting cells or ranges on each sheet
- Copying data from one sheet to another
- Running calculations or formulas on each sheet
- Hiding or unhiding sheets based on certain conditions
Looping through sheets allows you to automate these tasks, saving you time and reducing the risk of human error.
Method 1: Using the For Each
Loop
The For Each
loop is one of the most common methods used to loop through sheets in VBA. This method is straightforward and easy to implement.
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
' Code to execute on each sheet
Next ws
This method is useful when you need to perform tasks on every sheet in the workbook. However, it can be slower than other methods, especially when working with large workbooks.
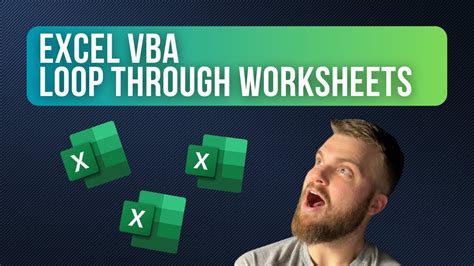
Method 2: Using the For
Loop with the Worksheets
Collection
This method uses a For
loop to iterate through the Worksheets
collection.
Dim i As Integer
For i = 1 To ThisWorkbook.Worksheets.Count
' Code to execute on each sheet
Next i
This method is faster than the For Each
loop, especially when working with large workbooks. However, it requires you to keep track of the sheet index, which can be error-prone.
Method 3: Using the For
Loop with the Worksheet
Object
This method uses a For
loop to iterate through the Worksheet
object.
Dim ws As Worksheet
For Each ws In ThisWorkbook.Sheets
' Code to execute on each sheet
Next ws
This method is similar to the For Each
loop, but it uses the Sheets
collection instead of the Worksheets
collection. The Sheets
collection includes both worksheets and chart sheets, whereas the Worksheets
collection only includes worksheets.
Method 4: Using the While
Loop
This method uses a While
loop to iterate through the sheets.
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(1)
While ws IsNot Nothing
' Code to execute on each sheet
Set ws = ws.Next
Wend
This method is less common than the other methods, but it can be useful when you need to perform tasks on a specific range of sheets.
Method 5: Using the Do While
Loop
This method uses a Do While
loop to iterate through the sheets.
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(1)
Do While ws IsNot Nothing
' Code to execute on each sheet
Set ws = ws.Next
Loop
This method is similar to the While
loop, but it uses a Do While
loop instead.
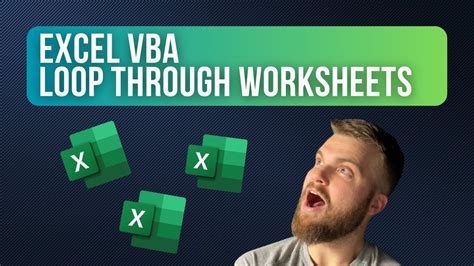
Conclusion
Looping through sheets in VBA is a crucial task that can save you time and reduce errors. In this article, we explored five different methods to loop through sheets in VBA, highlighting the benefits and drawbacks of each method. By understanding these methods, you can choose the best approach for your specific project requirements.
We hope this article has been helpful in providing you with a comprehensive understanding of looping through sheets in VBA. If you have any questions or need further assistance, please don't hesitate to ask.
Gallery of VBA Loop Through Sheets
VBA Loop Through Sheets Image Gallery
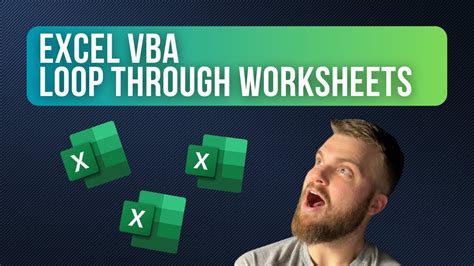
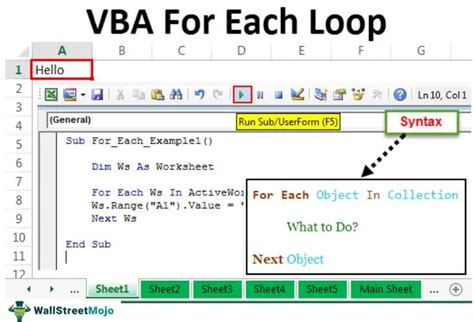
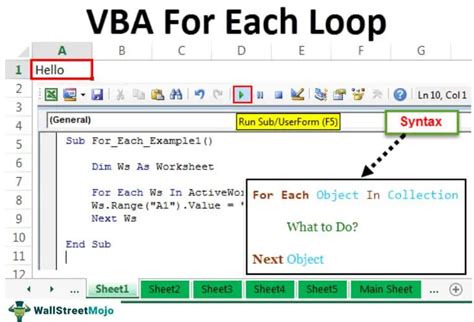
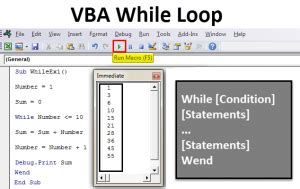
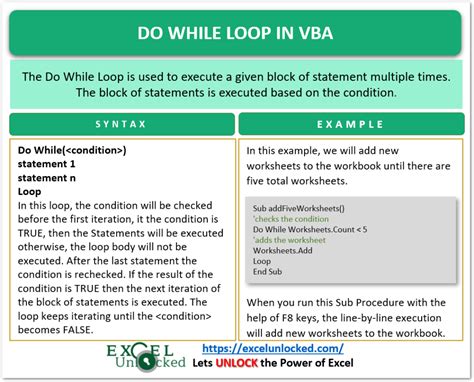
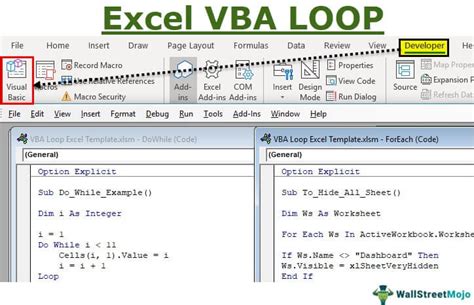
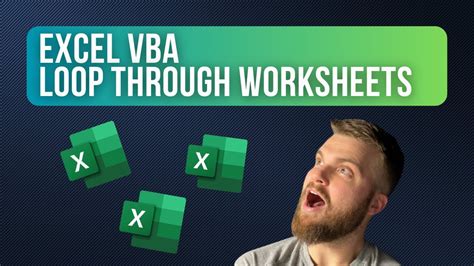
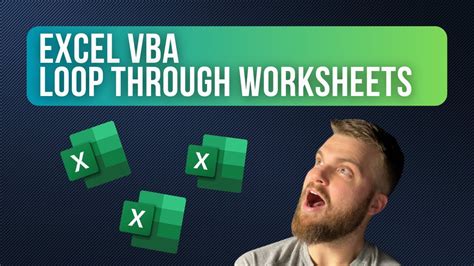
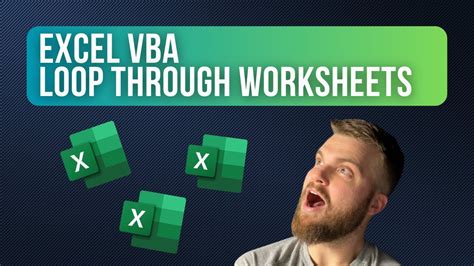
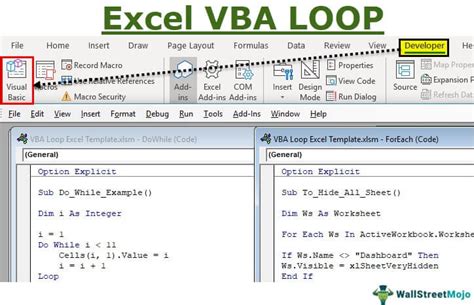
FAQ
Q: What is the best method to loop through sheets in VBA?
A: The best method depends on your specific project requirements. The For Each
loop is a good starting point, but you may need to use other methods depending on your needs.
Q: How do I loop through sheets in VBA without using the For Each
loop?
A: You can use the For
loop with the Worksheets
collection, the While
loop, or the Do While
loop.
Q: Can I use the Sheets
collection instead of the Worksheets
collection?
A: Yes, but the Sheets
collection includes both worksheets and chart sheets, whereas the Worksheets
collection only includes worksheets.