Intro
Learn how to collect user input with ease using VBA MsgBox text input. Discover how to create interactive MsgBox prompts, retrieve user responses, and incorporate input validation in your VBA applications. Master the art of user interaction with VBA and streamline your workflows with efficient text input techniques.
Visual Basic for Applications (VBA) is a powerful tool used in Microsoft Office applications, such as Excel, Word, and Access, to automate tasks and create custom interfaces. One of the most useful features in VBA is the ability to collect user input through various methods, including the MsgBox
function. In this article, we will explore how to use the MsgBox
function to collect user input in VBA, specifically focusing on text input.
The Importance of User Input in VBA
Collecting user input is a crucial aspect of creating interactive and dynamic applications in VBA. By allowing users to provide input, you can create applications that are tailored to their specific needs and preferences. User input can be used to drive calculations, update databases, or trigger specific actions within an application. In this article, we will focus on collecting text input using the MsgBox
function, which is a simple and effective way to gather user input.
Using the MsgBox Function for Text Input
The MsgBox
function is a built-in VBA function that displays a message box with a specified message and returns a value indicating the user's response. While the MsgBox
function is often used to display information or warnings, it can also be used to collect text input from users. To use the MsgBox
function for text input, you need to specify the vbInputBox
argument, which allows users to enter text.
Here is a basic example of how to use the MsgBox
function for text input:
Sub CollectTextInput()
Dim userInput As String
userInput = InputBox("Please enter your name:", "User Input")
MsgBox "Hello, " & userInput & "!"
End Sub
In this example, the InputBox
function is used to display a message box with a prompt asking the user to enter their name. The user's input is stored in the userInput
variable, which is then used to display a greeting message.
Customizing the MsgBox Function for Text Input
While the basic MsgBox
function for text input is useful, you may want to customize it to suit your specific needs. Here are some ways to customize the MsgBox
function:
- Default Value: You can specify a default value for the text input by adding a third argument to the
InputBox
function. For example:userInput = InputBox("Please enter your name:", "User Input", "John Doe")
- Title: You can specify a custom title for the message box by adding a fourth argument to the
InputBox
function. For example:userInput = InputBox("Please enter your name:", "User Input", "John Doe", "Enter Your Name")
- Cancel Button: You can specify whether the cancel button is displayed by adding a fifth argument to the
InputBox
function. For example:userInput = InputBox("Please enter your name:", "User Input", "John Doe", "Enter Your Name", True)
Here is an example of a customized MsgBox
function for text input:
Sub CollectTextInput()
Dim userInput As String
userInput = InputBox("Please enter your name:", "User Input", "John Doe", "Enter Your Name", True)
MsgBox "Hello, " & userInput & "!"
End Sub
Practical Examples of Using the MsgBox Function for Text Input
Here are some practical examples of using the MsgBox
function for text input:
- Data Validation: You can use the
MsgBox
function to collect user input and validate it against specific criteria. For example: ```vb Sub ValidateTextInput() Dim userInput As String userInput = InputBox("Please enter your email address:", "User Input") If ValidateEmail(userInput) Then MsgBox "Thank you for entering a valid email address!" Else MsgBox "Please enter a valid email address!" End If End Sub
* **User Preferences**: You can use the `MsgBox` function to collect user input and store it in a database or worksheet. For example: ```vb
Sub CollectUserPreferences()
Dim userInput As String
userInput = InputBox("Please enter your favorite color:", "User Preferences")
Range("A1").Value = userInput
End Sub
Gallery of VBA MsgBox Text Input Examples
Here are some examples of using the MsgBox
function for text input in VBA:
VBA MsgBox Text Input Gallery
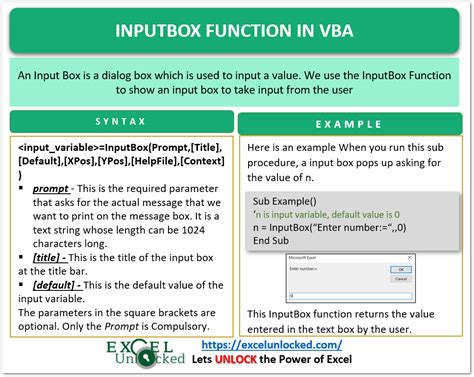
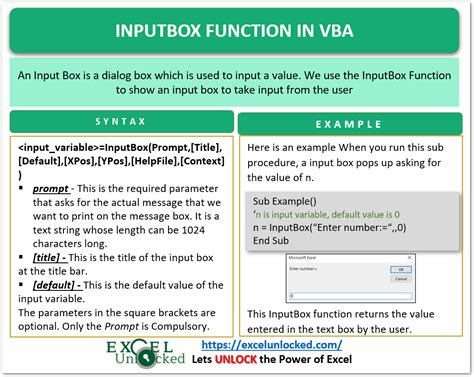
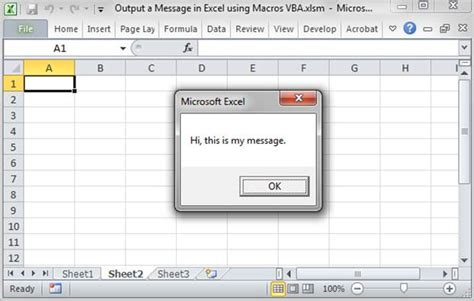
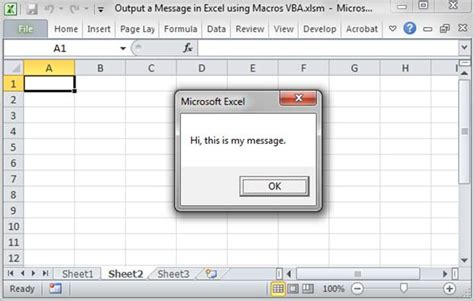
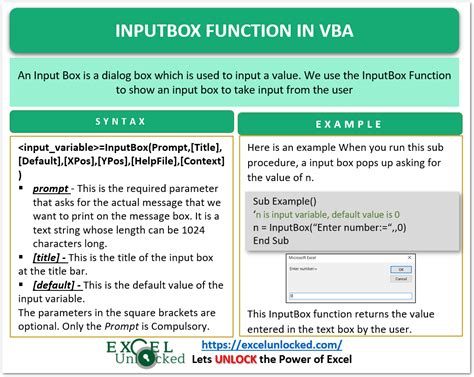
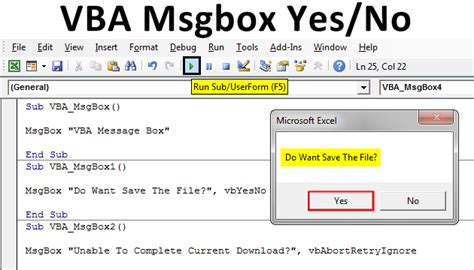
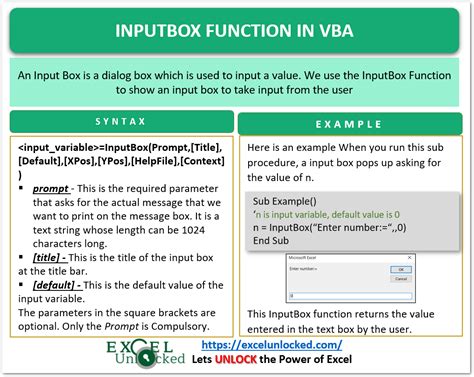
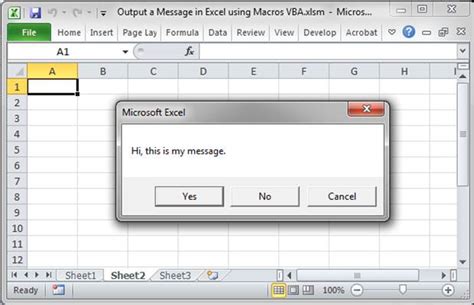
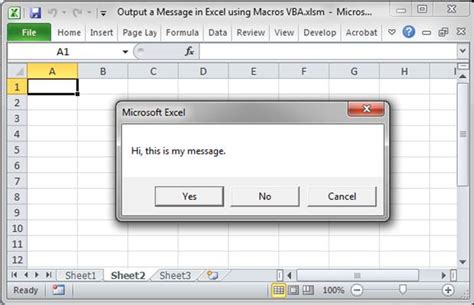
Conclusion
In conclusion, the MsgBox
function is a powerful tool in VBA that allows you to collect user input with ease. By using the InputBox
function, you can create interactive applications that gather text input from users and perform various tasks based on their input. Whether you need to validate data, collect user preferences, or simply display a message, the MsgBox
function is an essential tool in your VBA toolkit. We hope this article has provided you with a comprehensive understanding of how to use the MsgBox
function for text input in VBA.
Share Your Thoughts!
We would love to hear your thoughts on using the MsgBox
function for text input in VBA. Have you used this function in your own projects? Do you have any tips or tricks to share? Please leave a comment below and let's start a conversation!