Intro
Learn how to print to PDF with ease using VBA. Discover the simplest methods to convert your Excel files to PDF format, including using the Acrobat PDFMaker and late binding techniques. Master VBA print to PDF tricks and streamline your workflow with our expert guide, covering PDF creation, formatting, and automation.
Saving time and increasing productivity are essential goals for many professionals, especially those who work extensively with Microsoft Office applications like Excel. One of the most common tasks that can consume a significant amount of time is printing and sharing documents, especially when it comes to creating Portable Document Format (PDF) files. Fortunately, Visual Basic for Applications (VBA) can greatly simplify this process.
For Excel users, printing to PDF can be a bit tricky without the right tools or knowledge. However, with VBA, you can automate this process, making it quicker and more efficient. In this article, we will explore the world of VBA printing to PDF, covering the benefits, how it works, and providing a step-by-step guide on implementing this solution in your workflow.
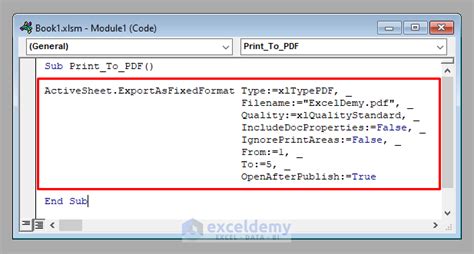
Benefits of Using VBA to Print to PDF
Before diving into the specifics of how to use VBA for printing to PDF, it's essential to understand the benefits of this approach. Here are a few key advantages:
- Time-Saving: Automating the process of printing to PDF can save you a considerable amount of time, especially when dealing with multiple documents or regular reports.
- Consistency: VBA ensures that your PDFs are generated consistently, with the same layout and settings each time, reducing errors and variability.
- Customization: With VBA, you can customize the PDF generation process to fit your specific needs, including selecting page ranges, adding headers or footers, and more.
Understanding How VBA Works with PDFs
To print to PDF using VBA, you need to understand the basic mechanism behind this process. Here's a simplified overview:
- Identify the PDF Creator: First, you need a PDF creator installed on your system. This could be Adobe Acrobat, PDFCreator, or any other compatible software.
- Accessing VBA: Open Excel and navigate to the Developer tab. If you don't see the Developer tab, you can enable it from the Excel Options menu.
- Write the VBA Code: In the Visual Basic Editor, you will write a script that tells Excel how to print your document to PDF. This involves specifying the printer (in this case, the PDF creator), the file path and name for the PDF, and any other settings you want to apply.
A Step-by-Step Guide to Printing to PDF with VBA
Now that you understand the basics, let's walk through the process step by step:
Step 1: Enable the Developer Tab
- Open Excel.
- Go to File > Options.
- In the Excel Options window, click on Customize Ribbon.
- Check the box next to "Developer" in the list of available main tabs.
- Click OK.
Step 2: Open the Visual Basic Editor
- Go to the Developer tab.
- Click on Visual Basic.
Step 3: Write the VBA Code
- In the Visual Basic Editor, insert a new module by right-clicking on any of the objects for your workbook listed in the "Project" window on the left side of the screen. Choose Insert > Module.
- Copy and paste the following code into the module:
Sub PrintToPDF()
Dim ws As Worksheet
Dim filename As String
' Change this to the sheet you want to print
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Change this to the desired file path and name
filename = "C:\Users\YourUsername\Documents\example.pdf"
' Print the worksheet to PDF
ws.ExportAsFixedFormat _
Type:=xlTypePDF, _
Filename:=filename, _
Quality:=xlMediumQuality, _
IncludeDocProps:=True, _
IgnorePrintAreas:=False, _
OpenAfterPublish:=False
End Sub
- Customize the code as needed. Specifically, you will need to change "Sheet1" to the name of the worksheet you want to print, and update the file path and name for the PDF output.
Step 4: Run the Macro
- Close the Visual Basic Editor.
- Save your workbook with the macro enabled. It's recommended to save the workbook as an Excel Macro-Enabled Workbook (.xlsm).
- Go back to the Developer tab.
- Click on Macros.
- In the Macro dialog box, find and select "PrintToPDF," then click Run.
The specified worksheet will now be printed to PDF according to the settings you defined in the VBA code.
Troubleshooting Common Issues
While using VBA to print to PDF is generally straightforward, you might encounter a few common issues:
- PDF Creator Not Found: Ensure that a PDF creator is installed and configured properly on your system.
- Permission Errors: Check the file path and ensure that you have the necessary permissions to write files to that location.
- Macro Security: Make sure that macros are enabled in your Excel settings. You may need to adjust the security settings to allow macros to run.
Conclusion
Printing to PDF with VBA is a powerful tool for automating tasks in Excel, saving you time and effort. By following the steps outlined in this guide, you can integrate this functionality into your workflow and enjoy the benefits of streamlined document management. Remember to customize the VBA code to suit your specific needs, whether it's adjusting the PDF quality, adding metadata, or specifying page ranges.
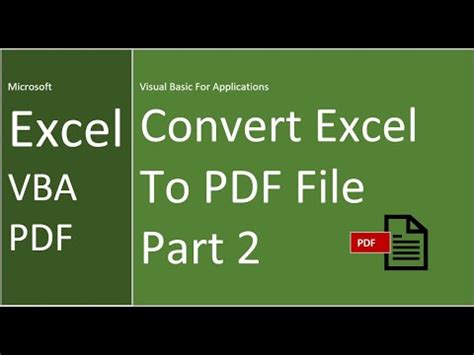
FAQs
Q: Do I need to have Adobe Acrobat installed to use VBA for printing to PDF?
A: No, you don't necessarily need Adobe Acrobat. Any PDF creator that is compatible with VBA can be used. However, Adobe Acrobat is a popular choice due to its comprehensive features.
Q: How do I customize the PDF settings in the VBA code?
A: You can customize various settings such as the PDF quality, whether to include document properties, and whether to open the PDF after publishing by adjusting the parameters in the ExportAsFixedFormat
method.
Q: Can I use VBA to print multiple worksheets to a single PDF?
A: Yes, you can modify the VBA code to loop through multiple worksheets and print them to a single PDF file. This involves adjusting the ws
variable to iterate over different worksheets and possibly adjusting the file naming convention to avoid overwriting files.
Excel VBA Print to PDF Image Gallery
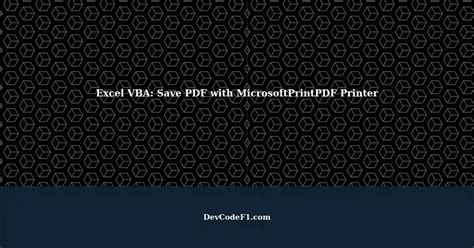
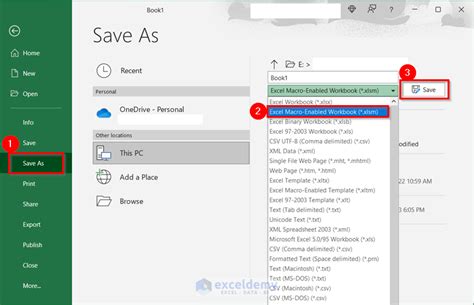
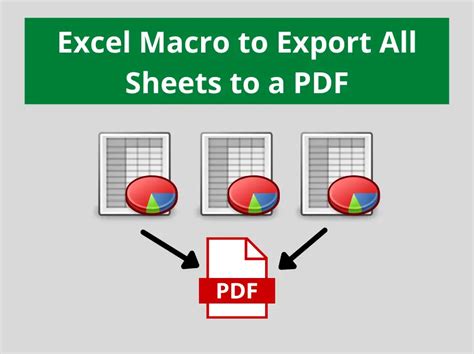
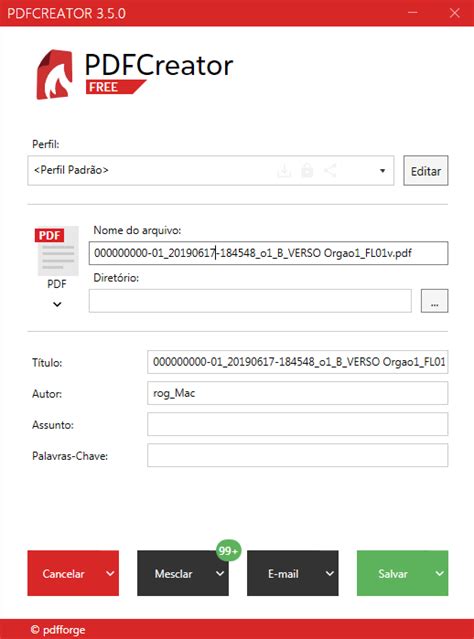
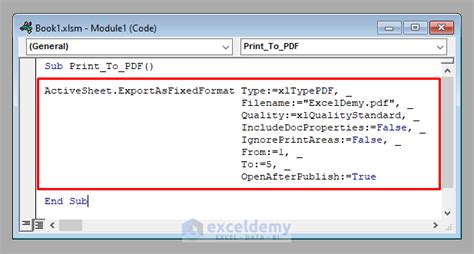
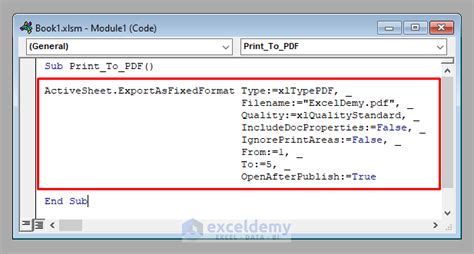
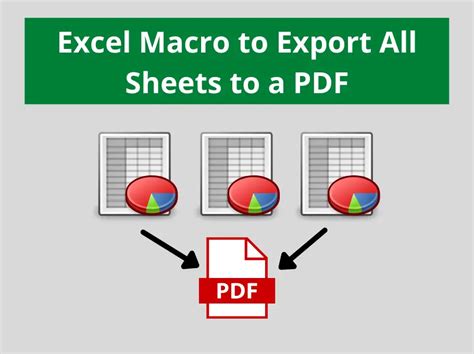
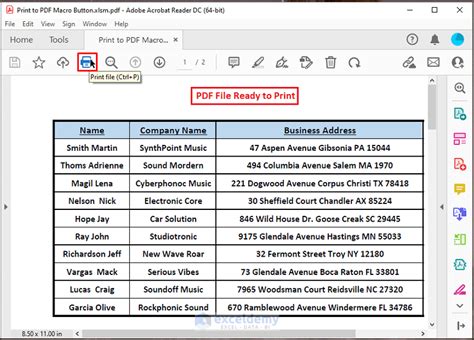
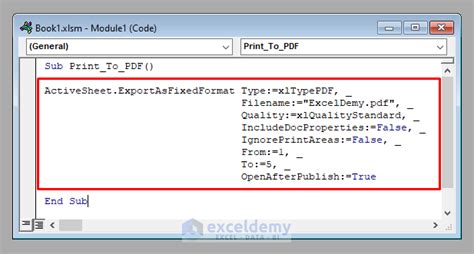
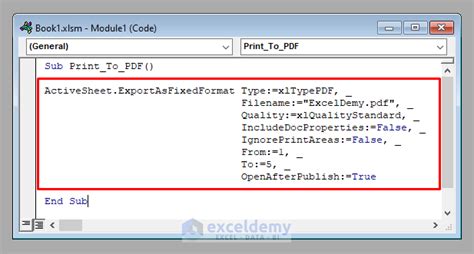