Intro
Master VBA string manipulation with our expert guide on how to quickly and easily replace characters in strings using VBA. Learn efficient methods to replace characters, substrings, and patterns in VBA strings, including using the Replace function, regular expressions, and more. Boost your VBA skills and automate tasks with ease.
VBA, or Visual Basic for Applications, is a powerful tool used in Microsoft Office applications, such as Excel, Word, and Outlook. It allows users to automate tasks, create custom functions, and interact with the application's environment. One common task in VBA programming is manipulating strings, which includes replacing characters within a string. In this article, we will delve into how to replace characters in a string quickly and easily using VBA.
Understanding VBA Strings
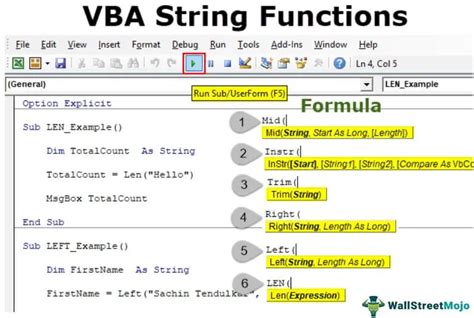
Before diving into the methods of replacing characters in a string, it's essential to understand how VBA handles strings. In VBA, a string is a sequence of characters, such as letters, numbers, spaces, and punctuation marks. Strings can be enclosed in double quotes ("") or, less commonly, in single quotes (''). The choice of delimiter (quotes or single quotes) does not affect the content of the string itself.
Why Replace Characters in Strings?
There are several reasons why you might need to replace characters in a string in VBA:
- Cleaning up data by removing unwanted characters
- Standardizing formats, such as changing all commas to semicolons
- Preparing data for export or import by converting special characters
- Enhancing the readability of text by replacing abbreviations with their full forms
Methods for Replacing Characters in Strings
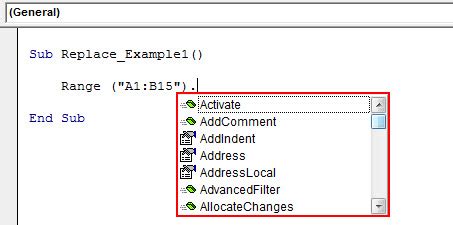
VBA offers several methods to replace characters in strings, each with its own set of advantages and use cases.
1. Using the Replace
Function
The Replace
function is the most straightforward method to replace characters in a string. Its syntax is as follows:
Replace(expression, find, replace, [start, [count]])
Where:
expression
is the string you want to modifyfind
is the character(s) you want to replacereplace
is the character(s) you want to use as a replacementstart
is an optional argument specifying the starting position for the replacementcount
is an optional argument specifying how many replacements to make
Example:
Dim originalString As String
originalString = "Hello, World!"
Dim modifiedString As String
modifiedString = Replace(originalString, ",", ".")
MsgBox modifiedString
2. Using Regular Expressions
For more complex string manipulation, VBA can leverage regular expressions (regex) through the RegExp
object. This requires adding a reference to the "Microsoft VBScript Regular Expressions" library in your VBA project.
Dim regex As Object
Set regex = CreateObject("VBScript.RegExp")
regex.Pattern = "," ' Find comma
regex.IgnoreCase = True
Dim originalString As String
originalString = "Hello, World!"
Dim modifiedString As String
modifiedString = regex.Replace(originalString, ".")
MsgBox modifiedString
3. Looping Through Characters
Sometimes, you might want to replace characters based on specific conditions that are not easily handled by the Replace
function or regex. In such cases, looping through each character of the string is a viable option.
Dim originalString As String
originalString = "Hello, World!"
Dim modifiedString As String
modifiedString = ""
For i = 1 To Len(originalString)
If Mid(originalString, i, 1) = "," Then
modifiedString = modifiedString & "."
Else
modifiedString = modifiedString & Mid(originalString, i, 1)
End If
Next i
MsgBox modifiedString
Tips for Efficient Character Replacement
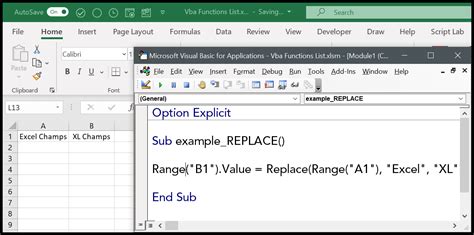
When working with large datasets or complex string manipulation tasks, efficiency becomes a critical factor. Here are some tips to improve your VBA string replacement efficiency:
- Use the
Replace
Function for Simple Tasks: For straightforward replacements, theReplace
function is the most efficient method. - Leverage Regular Expressions for Complex Patterns: When dealing with complex replacement patterns, especially those involving multiple conditions, consider using regex for its flexibility and power.
- Minimize Loop Iterations: When looping through characters, ensure that each iteration performs the necessary checks to avoid redundant operations.
Common Issues and Troubleshooting
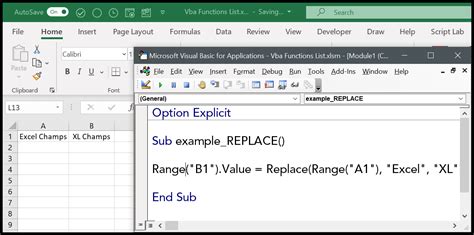
Despite the straightforward nature of string replacement in VBA, several common issues can arise, especially for those new to VBA programming. Here are some of the most frequent problems and their solutions:
- Inconsistent Case Sensitivity: Be aware that the
Replace
function is case-sensitive. If you need case-insensitive replacement, use theLCase
orUCase
function to convert both the string and the find parameter to the same case. - Regular Expression Pattern Errors: When using regex, ensure that your pattern is correctly defined. Misplacing a single character can lead to unexpected results or errors.
Gallery of VBA String Replacement Examples
VBA String Replacement Examples
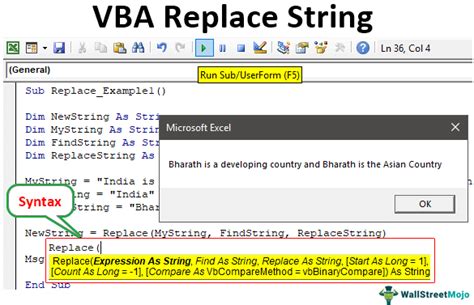
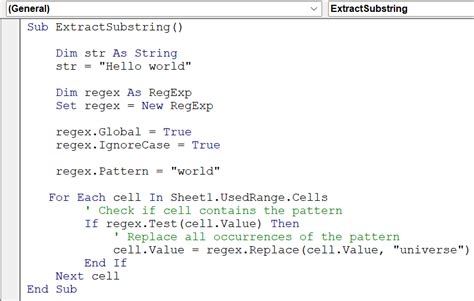
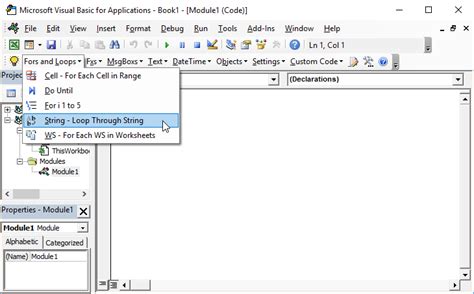
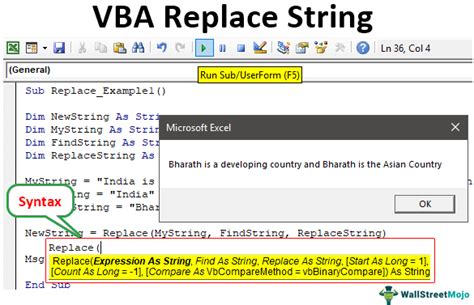
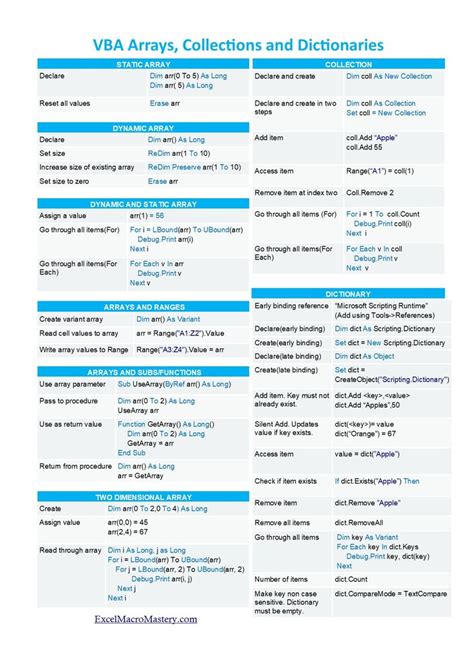
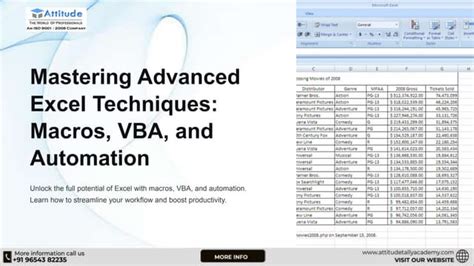
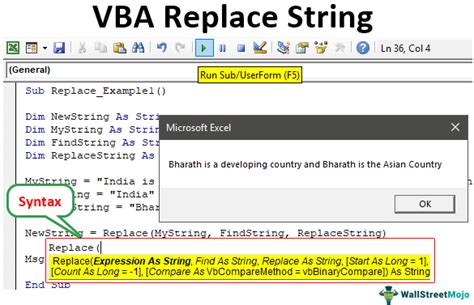
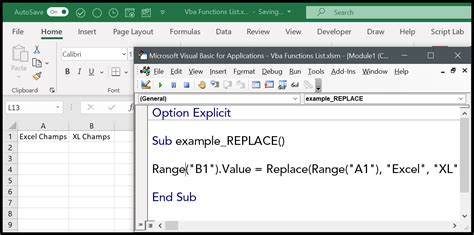
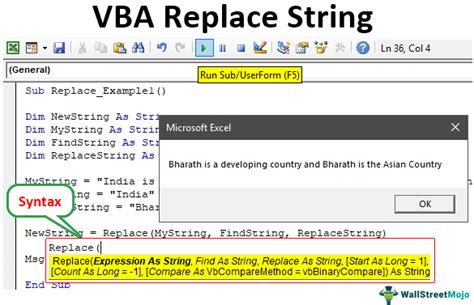
We hope this comprehensive guide to replacing characters in strings using VBA has been informative and helpful. Whether you're a beginner or an advanced user, mastering string manipulation is crucial for automating tasks in Microsoft Office applications.