Intro
Resolve VBA Runtime Error 91 with ease! Discover the causes and solutions for the Object Variable Not Set error in VBA. Learn how to troubleshoot, debug, and fix this common error with expert tips and tricks. Master VBA error handling and improve your coding skills with this comprehensive guide.
VBA Runtime Error 91 is a common error that occurs when trying to access or manipulate an object that hasn't been set or initialized. This error can be frustrating, but it's usually easy to fix. Here's a step-by-step guide to help you resolve the issue.
What causes VBA Runtime Error 91?
The error occurs when your code tries to access an object that doesn't exist or hasn't been properly initialized. This can happen in several situations:
- Uninitialized object: You're trying to use an object variable that hasn't been set to a specific object.
- Object not created: You're trying to access an object that hasn't been created or instantiated.
- Object no longer exists: You're trying to access an object that has been deleted or no longer exists.
How to fix VBA Runtime Error 91?
To resolve the error, follow these steps:
1. Check your object variables
Make sure you have declared and initialized all object variables correctly. Ensure that you have set the object variable to a specific object using the Set
keyword.
Dim myWorksheet As Worksheet
Set myWorksheet = ThisWorkbook.Worksheets("Sheet1")
2. Verify object existence
Before accessing an object, check if it exists using the If
statement.
If Not myWorksheet Is Nothing Then
' Code to access myWorksheet
End If
3. Use the Set
keyword
When assigning an object to a variable, use the Set
keyword to ensure proper initialization.
Set myWorksheet = ThisWorkbook.Worksheets.Add
4. Avoid using late binding
Late binding can lead to errors if the object doesn't exist. Try to use early binding by declaring the object variable with the correct type.
Dim myWorksheet As Worksheet
Instead of:
Dim myWorksheet As Object
5. Check for typos and incorrect references
Typos or incorrect references can cause the error. Verify that the object variable name matches the one used in the code.
6. Restart your application
Sometimes, restarting your application can resolve the issue.
Example code to avoid VBA Runtime Error 91
Here's an example code that demonstrates how to avoid the error:
Sub AvoidRuntimeError91()
Dim myWorksheet As Worksheet
' Set the object variable
Set myWorksheet = ThisWorkbook.Worksheets.Add
' Verify object existence
If Not myWorksheet Is Nothing Then
' Code to access myWorksheet
myWorksheet.Range("A1").Value = "Hello, World!"
End If
End Sub
Additional tips
- Always declare and initialize object variables before using them.
- Use the
Set
keyword when assigning objects to variables. - Verify object existence before accessing them.
- Avoid using late binding and opt for early binding instead.
By following these steps and tips, you should be able to fix VBA Runtime Error 91 and avoid similar errors in the future.
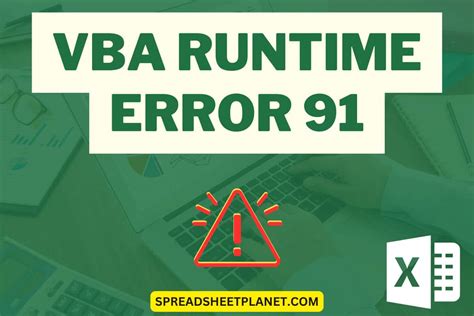
Gallery of VBA Runtime Error 91
VBA Runtime Error 91 Images
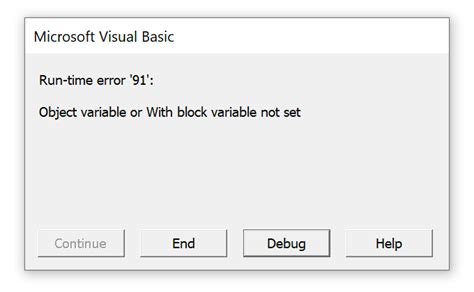
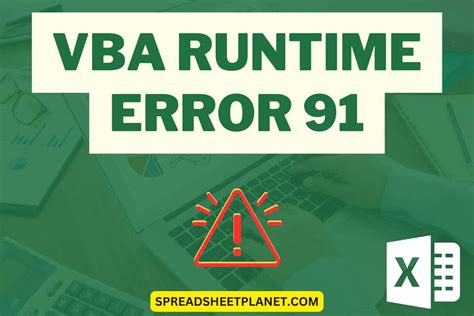
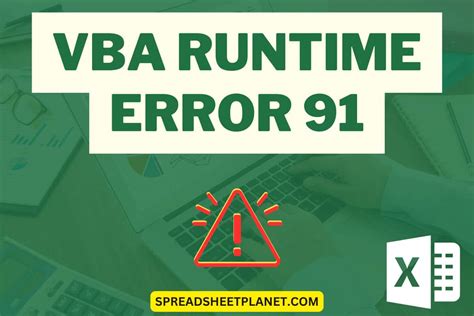
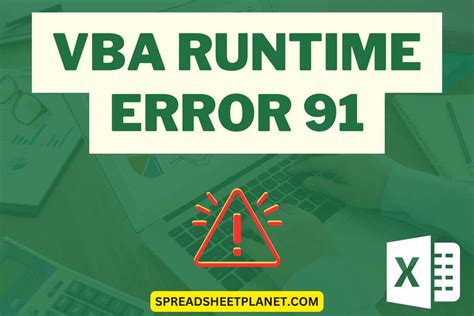
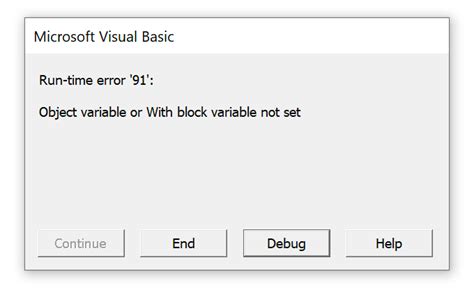
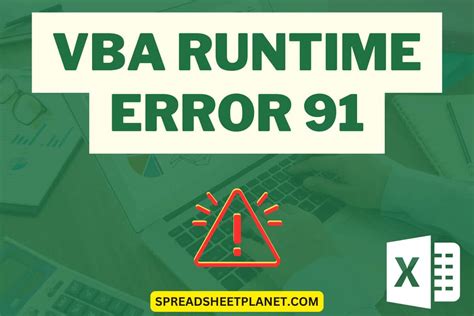
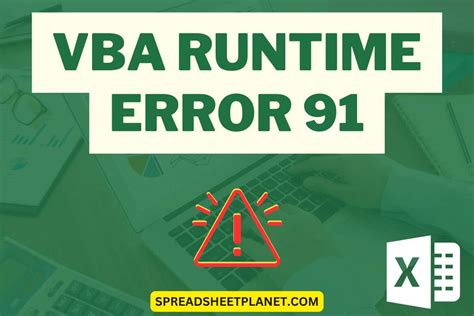
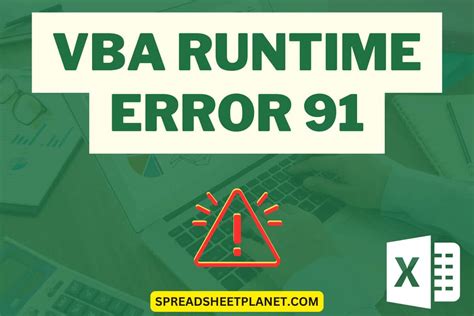
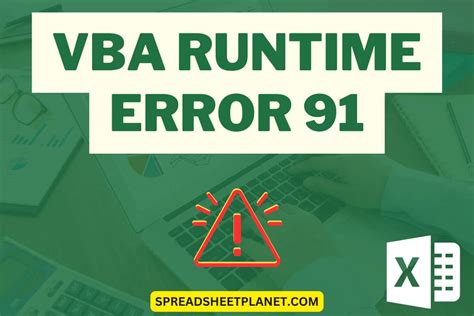
I hope this article helps you fix VBA Runtime Error 91 and understand how to avoid similar errors in the future. If you have any further questions or need additional assistance, please feel free to ask in the comments section below!