Intro
Master VBA search column for value with ease. Learn how to quickly find specific data in Excel using VBA macros. Discover how to search for values in columns, rows, and entire worksheets. Get step-by-step code examples and expert tips to boost your productivity. Simplify your VBA search tasks and make data analysis a breeze.
Searching for a specific value in a column using VBA can be a daunting task, especially for those who are new to programming. However, with the right techniques and tools, it can be made easy and efficient. In this article, we will explore the various methods of searching for a value in a column using VBA, and provide a step-by-step guide on how to do it.
Understanding the Problem
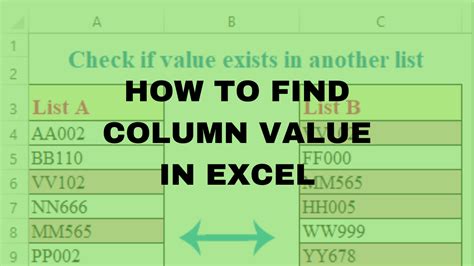
Before we dive into the solution, it's essential to understand the problem. When working with large datasets in Excel, it's common to need to find a specific value within a column. This can be a tedious task, especially if the dataset is massive. VBA provides a powerful solution to this problem by allowing you to automate the search process.
Method 1: Using the Find Method
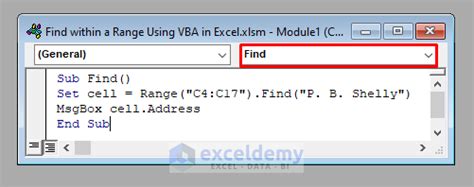
The Find method is a built-in VBA function that allows you to search for a value within a range. Here's an example of how to use the Find method to search for a value in a column:
Sub SearchColumn()
Dim rng As Range
Dim cell As Range
Dim searchValue As String
' Define the search value
searchValue = "John"
' Define the range to search
Set rng = Range("A:A")
' Use the Find method to search for the value
Set cell = rng.Find(searchValue)
' Check if the value is found
If cell Is Nothing Then
MsgBox "Value not found"
Else
MsgBox "Value found in cell " & cell.Address
End If
End Sub
This code defines a subroutine that searches for the value "John" in column A. If the value is found, it displays a message box with the address of the cell where the value is located.
Advantages and Disadvantages
The Find method is a powerful tool for searching for values in a column. However, it has some limitations. Here are some advantages and disadvantages of using the Find method:
Advantages:
- Easy to use
- Fast search results
- Can be used to search for exact matches or partial matches
Disadvantages:
- Can be slow for large datasets
- Can return incorrect results if the dataset is not sorted
Method 2: Using the Loop Method
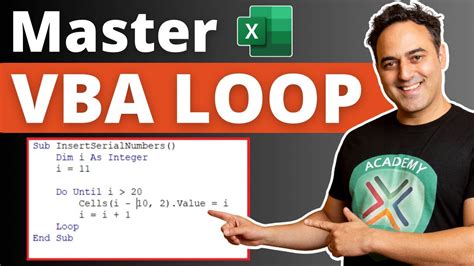
The Loop method involves iterating through each cell in the range and checking if the value matches the search value. Here's an example of how to use the Loop method to search for a value in a column:
Sub SearchColumn()
Dim rng As Range
Dim cell As Range
Dim searchValue As String
Dim found As Boolean
' Define the search value
searchValue = "John"
' Define the range to search
Set rng = Range("A:A")
' Initialize the found variable
found = False
' Loop through each cell in the range
For Each cell In rng
If cell.Value = searchValue Then
found = True
MsgBox "Value found in cell " & cell.Address
Exit For
End If
Next cell
' Check if the value is found
If Not found Then
MsgBox "Value not found"
End If
End Sub
This code defines a subroutine that searches for the value "John" in column A using the Loop method. If the value is found, it displays a message box with the address of the cell where the value is located.
Advantages and Disadvantages
The Loop method is a flexible tool for searching for values in a column. However, it has some limitations. Here are some advantages and disadvantages of using the Loop method:
Advantages:
- Can be used to search for exact matches or partial matches
- Can be used to search for values in multiple columns
- Can be used to perform additional tasks when the value is found
Disadvantages:
- Can be slow for large datasets
- Can be tedious to implement
Method 3: Using the Filter Method
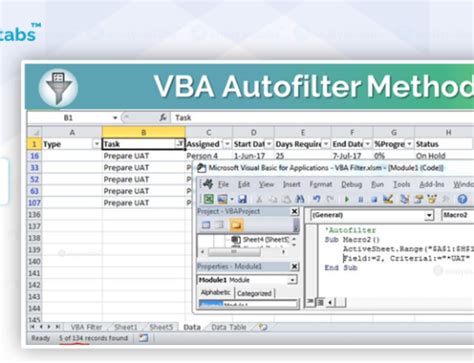
The Filter method involves using the AutoFilter feature to filter the data and find the value. Here's an example of how to use the Filter method to search for a value in a column:
Sub SearchColumn()
Dim rng As Range
Dim searchValue As String
' Define the search value
searchValue = "John"
' Define the range to search
Set rng = Range("A:A")
' Apply the filter
rng.AutoFilter Field:=1, Criteria1:=searchValue
' Check if the value is found
If rng.SpecialCells(xlCellTypeVisible).Count > 1 Then
MsgBox "Value found"
Else
MsgBox "Value not found"
End If
' Remove the filter
rng.AutoFilter
End Sub
This code defines a subroutine that searches for the value "John" in column A using the Filter method. If the value is found, it displays a message box indicating that the value is found.
Advantages and Disadvantages
The Filter method is a powerful tool for searching for values in a column. However, it has some limitations. Here are some advantages and disadvantages of using the Filter method:
Advantages:
- Fast search results
- Can be used to search for exact matches or partial matches
- Can be used to filter data based on multiple criteria
Disadvantages:
- Can be slow for large datasets
- Can be affected by the filter settings
VBA Search Column for Value Made Easy Image Gallery
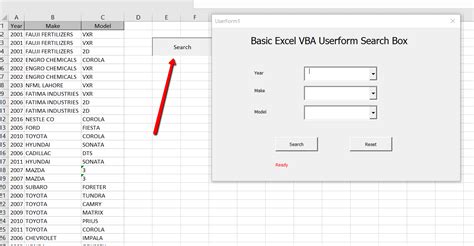
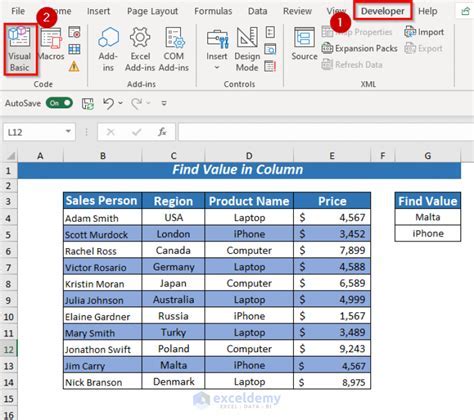
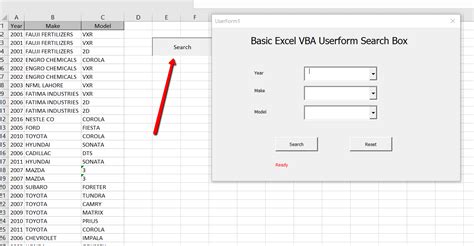
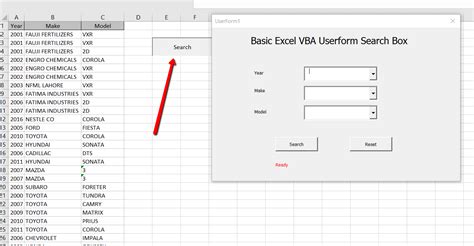
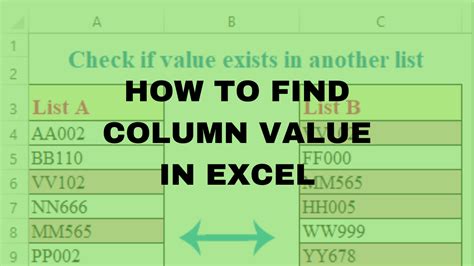
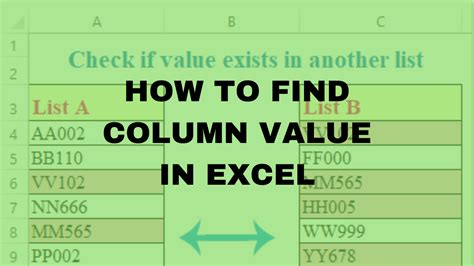
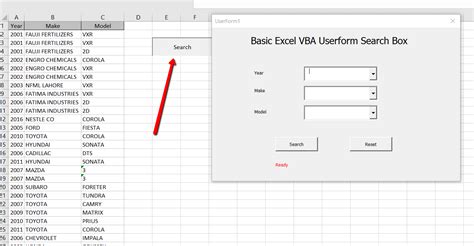
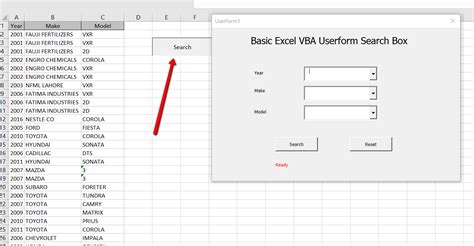
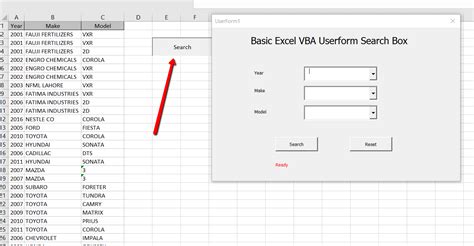
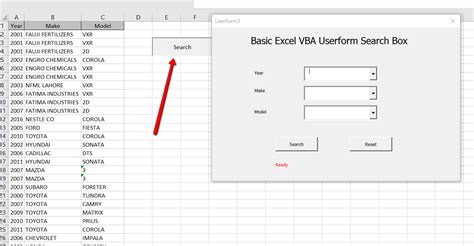
In conclusion, searching for a value in a column using VBA can be made easy and efficient using the right techniques and tools. The Find method, Loop method, and Filter method are all powerful tools that can be used to search for values in a column. By understanding the advantages and disadvantages of each method, you can choose the best approach for your specific needs.