Intro
Unlock the power of VBA with our easy-to-follow guide on searching for specific text in the next line of a string. Master the art of navigating and manipulating strings in Excel VBA with our expert tips and tricks. Learn how to use InStr, Mid, and Split functions to find and extract data with ease.
VBA Search Next Line in String Made Easy
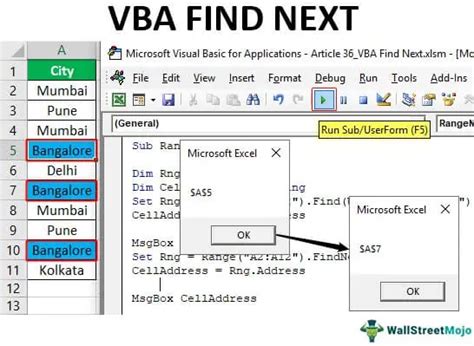
Searching for specific text within a string is a common task in VBA programming. However, when dealing with multi-line strings, finding the next line that contains a specific keyword or phrase can be a bit more challenging. In this article, we will explore how to perform a VBA search next line in string efficiently.
The importance of searching for specific text within a string cannot be overstated. It is a fundamental operation in many programming tasks, such as data manipulation, error handling, and automation. When working with large datasets or complex strings, being able to quickly and accurately locate specific text is crucial.
In VBA, the InStr
function is commonly used to search for a specific substring within a string. However, this function only returns the position of the first occurrence of the substring and does not provide a straightforward way to search for the next line that contains a specific keyword or phrase.
Using the Split Function to Search Next Line in String
One approach to searching for the next line in a string that contains a specific keyword or phrase is to use the `Split` function to split the string into an array of substrings, where each substring represents a line in the original string. Then, you can iterate through the array and check each substring for the presence of the keyword or phrase.Here is an example of how you can use the Split
function to search for the next line in a string that contains a specific keyword or phrase:
Dim strOriginal As String
Dim arrLines() As String
Dim i As Long
Dim strKeyword As String
strOriginal = "This is the first line." & vbCrLf & _
"This is the second line." & vbCrLf & _
"This is the third line."
strKeyword = "second"
arrLines = Split(strOriginal, vbCrLf)
For i = 0 To UBound(arrLines)
If InStr(1, arrLines(i), strKeyword) > 0 Then
MsgBox "The next line that contains the keyword '" & strKeyword & "' is:" & vbCrLf & arrLines(i)
Exit For
End If
Next i
In this example, the Split
function is used to split the original string into an array of substrings, where each substring represents a line in the original string. Then, the code iterates through the array and checks each substring for the presence of the keyword or phrase using the InStr
function. If the keyword or phrase is found, the code displays a message box with the next line that contains the keyword or phrase.
Using Regular Expressions to Search Next Line in String
Another approach to searching for the next line in a string that contains a specific keyword or phrase is to use regular expressions. Regular expressions provide a powerful way to search for patterns within strings and can be used to search for the next line that contains a specific keyword or phrase.Here is an example of how you can use regular expressions to search for the next line in a string that contains a specific keyword or phrase:
Dim strOriginal As String
Dim strKeyword As String
Dim objRegex As Object
Dim objMatch As Object
strOriginal = "This is the first line." & vbCrLf & _
"This is the second line." & vbCrLf & _
"This is the third line."
strKeyword = "second"
Set objRegex = CreateObject("VBScript.RegExp")
objRegex.Pattern = strKeyword & ".*"
objRegex.Multiline = True
Set objMatch = objRegex.Execute(strOriginal)
If objMatch.Count > 0 Then
MsgBox "The next line that contains the keyword '" & strKeyword & "' is:" & vbCrLf & objMatch(0).Value
Else
MsgBox "The keyword '" & strKeyword & "' was not found."
End If
In this example, the regular expression strKeyword & ".*"
is used to search for the next line that contains the keyword or phrase. The .*
pattern matches any characters (including none) until the end of the line, ensuring that the entire line is matched if the keyword or phrase is found. The Multiline
property is set to True
to ensure that the regular expression engine searches across multiple lines.
VBA Search Next Line in String Best Practices
When searching for the next line in a string that contains a specific keyword or phrase, there are several best practices to keep in mind:- Use the Split function or regular expressions: Both the
Split
function and regular expressions provide efficient ways to search for the next line in a string that contains a specific keyword or phrase. - Use the InStr function for simple searches: If you only need to search for a simple substring within a string, the
InStr
function is a good choice. - Consider case sensitivity: When searching for a keyword or phrase, consider whether the search should be case sensitive or not.
- Use MsgBox to display results: Use
MsgBox
to display the results of the search, including the next line that contains the keyword or phrase.
VBA Search Next Line in String Common Errors
When searching for the next line in a string that contains a specific keyword or phrase, there are several common errors to avoid:- Using the wrong search function: Using the wrong search function, such as
InStr
instead ofSplit
or regular expressions, can lead to incorrect results or poor performance. - Not considering case sensitivity: Failing to consider case sensitivity can lead to incorrect results or missed matches.
- Not using MsgBox to display results: Not using
MsgBox
to display the results of the search can make it difficult to verify the correctness of the search.
VBA Search Next Line in String Conclusion
Searching for the next line in a string that contains a specific keyword or phrase is an important task in VBA programming. By using the `Split` function or regular expressions, you can efficiently search for the next line that contains a specific keyword or phrase. Remember to consider case sensitivity and use `MsgBox` to display the results of the search.VBA Search Next Line in String Image Gallery
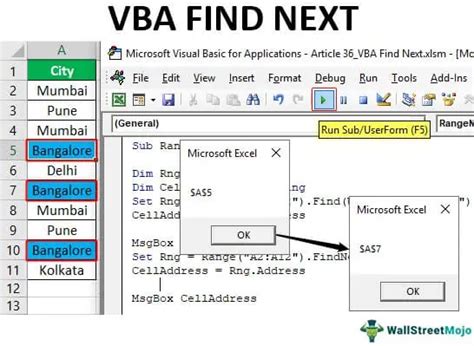
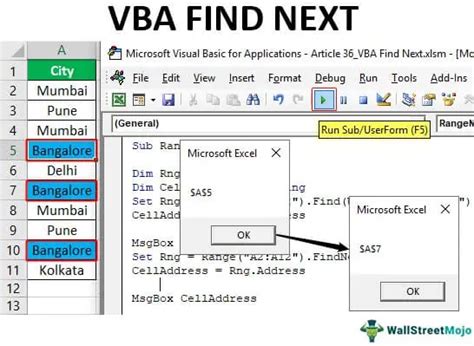
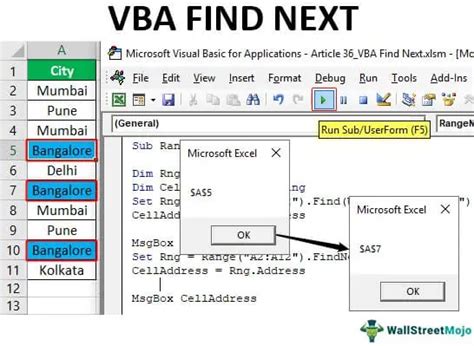
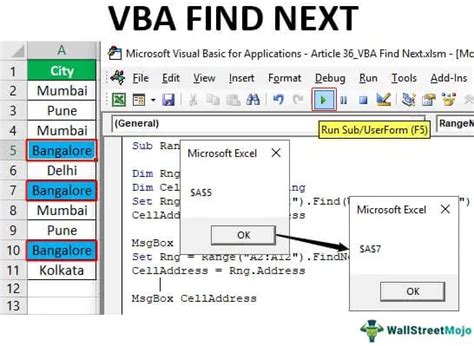
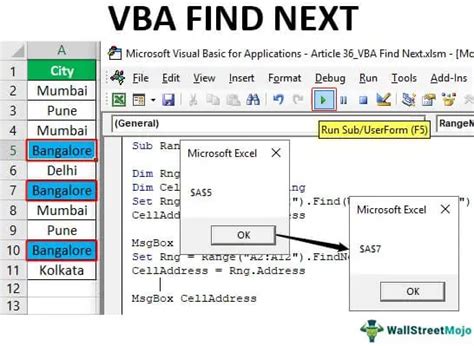
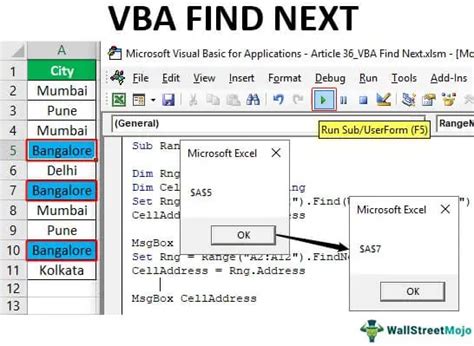
Share your thoughts on VBA search next line in string! Have you used the Split
function or regular expressions to search for specific text within a string? Share your experiences and tips in the comments below!