Intro
Master VBA sheet selection with 5 expert methods. Discover how to activate, select, and manipulate sheets in Excel using VBA. Learn to iterate through worksheets, use sheet names, and apply VBA sheet selection techniques for efficient data management. Improve your VBA skills and automate tasks with ease.
Understanding the Importance of Selecting Sheets in VBA
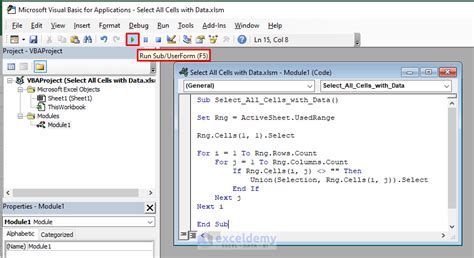
When working with Excel VBA, selecting the correct sheet is crucial for accurate data manipulation and analysis. A single mistake can lead to incorrect results, errors, or even data loss. In this article, we will explore five ways to select a sheet in VBA, highlighting the benefits and potential pitfalls of each method.
The Risks of Not Selecting the Correct Sheet
Not selecting the correct sheet can lead to a range of issues, including:
- Incorrect data analysis and reporting
- Data loss or corruption
- Errors in formula calculations
- Inability to perform tasks due to incorrect sheet selection
Method 1: Using the Sheets
Collection
One of the most common ways to select a sheet in VBA is by using the Sheets
collection. This method involves referencing the sheet by its index number or name.
Sub SelectSheetByIndex()
Sheets(1).Select
End Sub
Sub SelectSheetByName()
Sheets("Sheet1").Select
End Sub
Method 2: Using the Worksheets
Collection
Another way to select a sheet is by using the Worksheets
collection. This method is similar to the Sheets
collection, but it only includes worksheets, excluding chart sheets and other types of sheets.
Sub SelectWorksheetByIndex()
Worksheets(1).Select
End Sub
Sub SelectWorksheetByName()
Worksheets("Sheet1").Select
End Sub
Method 3: Using the Activate
Method
The Activate
method is another way to select a sheet. This method activates the specified sheet, making it the active sheet.
Sub ActivateSheet()
Sheets("Sheet1").Activate
End Sub
Method 4: Using the Select
Method with Range
Object
You can also select a sheet by using the Select
method with a Range
object. This method selects the range on the specified sheet.
Sub SelectRangeOnSheet()
Range("A1").Select
Sheets("Sheet1").Select
End Sub
Method 5: Using the Application.Goto
Method
The Application.Goto
method is another way to select a sheet. This method navigates to the specified range on the specified sheet.
Sub GotoRangeOnSheet()
Application.Goto Range("A1"), Scroll:=True
Sheets("Sheet1").Select
End Sub
Choosing the Right Method
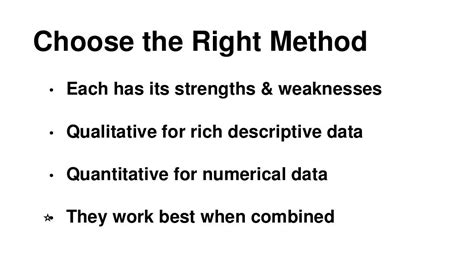
When selecting a sheet in VBA, it's essential to choose the right method for your specific needs. Consider the following factors:
- Performance: The
Sheets
andWorksheets
collections are generally faster than theActivate
andApplication.Goto
methods. - Readability: The
Select
method with aRange
object can make your code more readable by specifying the range on the sheet. - Flexibility: The
Application.Goto
method offers more flexibility by allowing you to navigate to a specific range on a sheet.
Best Practices for Selecting Sheets in VBA
To ensure accurate and efficient sheet selection in VBA, follow these best practices:
- Use the
Sheets
orWorksheets
collection: These collections are generally faster and more reliable than other methods. - Specify the sheet name or index: Always specify the sheet name or index to avoid selecting the wrong sheet.
- Avoid using
Select
method: TheSelect
method can lead to errors and slow performance. Instead, use theActivate
orApplication.Goto
methods. - Test your code: Always test your code to ensure that the correct sheet is selected.
Gallery of VBA Sheet Selection Methods
VBA Sheet Selection Methods
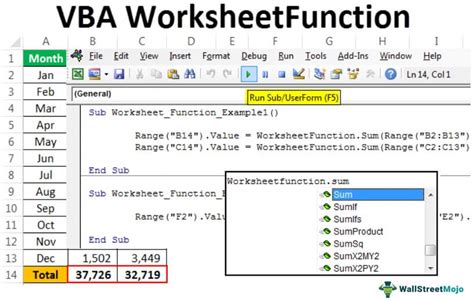
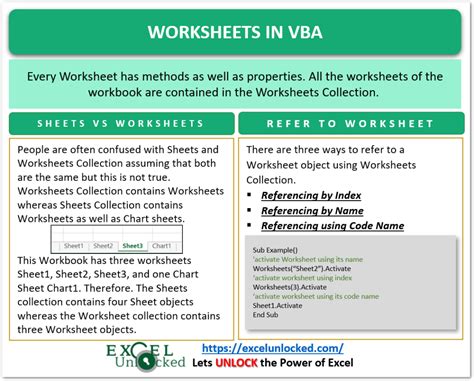
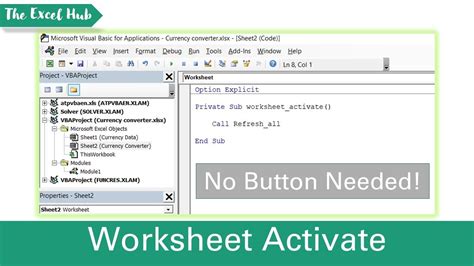
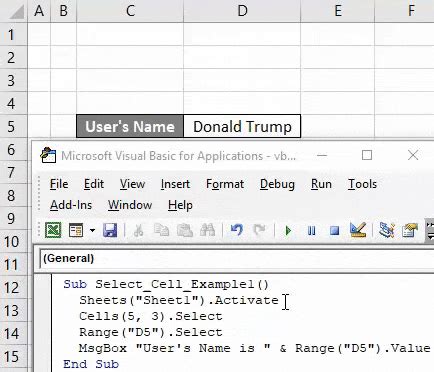
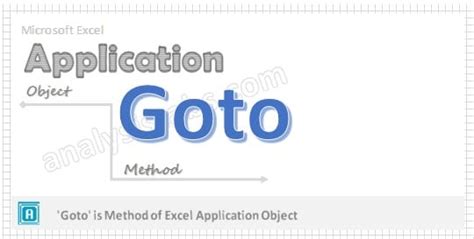
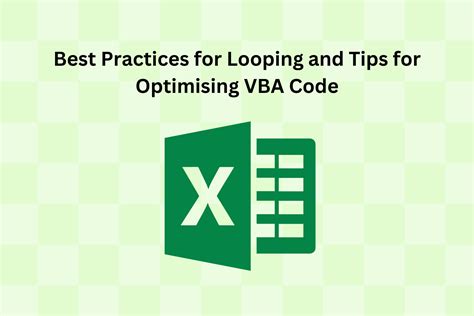
We hope this article has provided you with a comprehensive understanding of the different methods for selecting sheets in VBA. By following best practices and choosing the right method for your specific needs, you can ensure accurate and efficient sheet selection in your VBA code. If you have any questions or comments, please feel free to share them below.