Intro
Master VBA Select Whole Column with ease! Learn how to efficiently select entire columns in Excel using VBA, including how to reference columns, loop through data, and avoid common pitfalls. Simplify your workflow with expert tips and code examples, perfect for automating tasks and improving productivity.
VBA Select Whole Column Made Easy
When working with Excel, selecting an entire column can be a mundane task, especially when dealing with large datasets. Fortunately, VBA provides an efficient way to achieve this task with just a few lines of code. In this article, we will explore the different methods to select a whole column using VBA, making it easier for you to work with your Excel spreadsheets.
Why Select a Whole Column?
Before diving into the VBA code, let's understand why selecting a whole column is useful. Here are a few scenarios where this comes in handy:
- Data analysis: When working with large datasets, selecting an entire column allows you to perform calculations, formatting, or data manipulation on the entire range of cells.
- Data visualization: Selecting a whole column enables you to create charts, graphs, or other visualizations that represent the entire dataset.
- Data formatting: Selecting an entire column makes it easy to apply formatting changes, such as font styles, colors, or number formatting, to the entire range of cells.
Method 1: Using the Range
Object
The most straightforward way to select a whole column using VBA is by utilizing the Range
object. Here's an example code snippet:
Sub SelectWholeColumn()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
ws.Range("A:A").Select
End Sub
In this code, we first declare a worksheet object ws
and set it to the active sheet. Then, we use the Range
object to select the entire column A by specifying the range as "A:A".
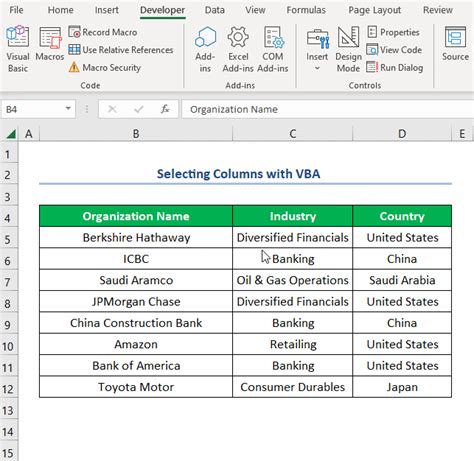
Method 2: Using the Cells
Property
Another way to select a whole column is by using the Cells
property. Here's an example code snippet:
Sub SelectWholeColumn()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
ws.Cells(1, 1).EntireColumn.Select
End Sub
In this code, we use the Cells
property to select the cell at row 1, column 1 (cell A1). Then, we use the EntireColumn
property to select the entire column.
Method 3: Using the Columns
Property
You can also select a whole column using the Columns
property. Here's an example code snippet:
Sub SelectWholeColumn()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
ws.Columns(1).Select
End Sub
In this code, we use the Columns
property to select the first column (column A).
Tips and Variations
Here are some additional tips and variations to keep in mind:
- Selecting multiple columns: To select multiple columns, you can modify the range or column index. For example,
ws.Range("A:C").Select
selects columns A to C. - Selecting a specific column: To select a specific column, you can use the
Cells
property with the column index. For example,ws.Cells(1, 3).EntireColumn.Select
selects the third column (column C). - Selecting a range of columns: To select a range of columns, you can use the
Range
object with the column indices. For example,ws.Range("B:E").Select
selects columns B to E.
Common Errors and Solutions
When working with VBA, it's not uncommon to encounter errors. Here are some common errors and solutions related to selecting a whole column:
- Error: "Select method of range class failed": This error occurs when the range is not valid or the worksheet is not active. Solution: Ensure the worksheet is active and the range is valid.
- Error: "Object required": This error occurs when the
Range
orCells
object is not properly set. Solution: Ensure the object is properly set and referenced.
Conclusion
Selecting a whole column in Excel using VBA is a straightforward process that can save you time and effort. By using the Range
, Cells
, or Columns
property, you can easily select an entire column and perform various tasks such as data analysis, visualization, or formatting. Remember to use the tips and variations provided to customize your code and avoid common errors.
VBA Select Whole Column Made Easy Image Gallery
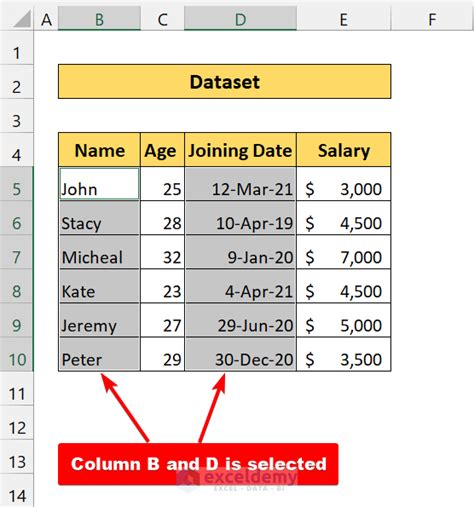
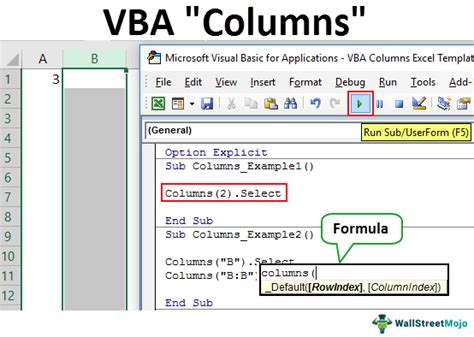
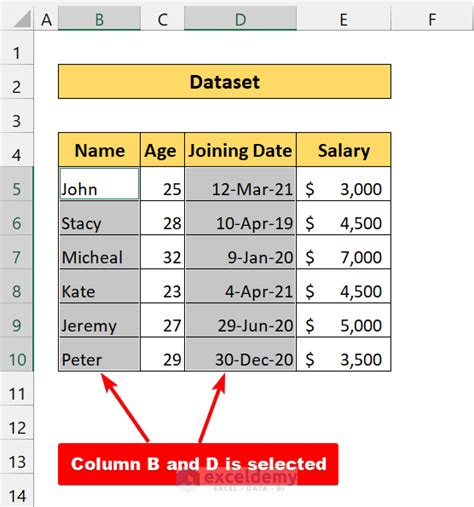
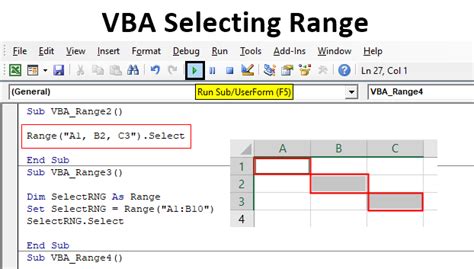
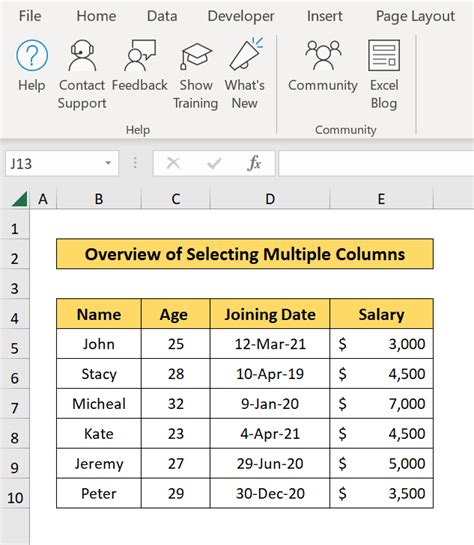
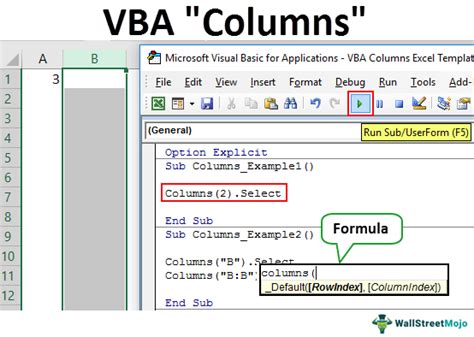
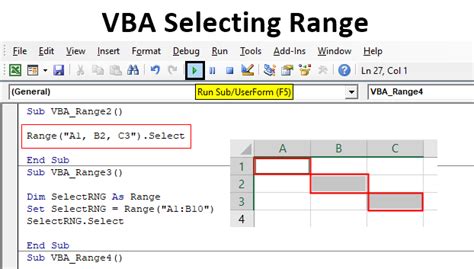
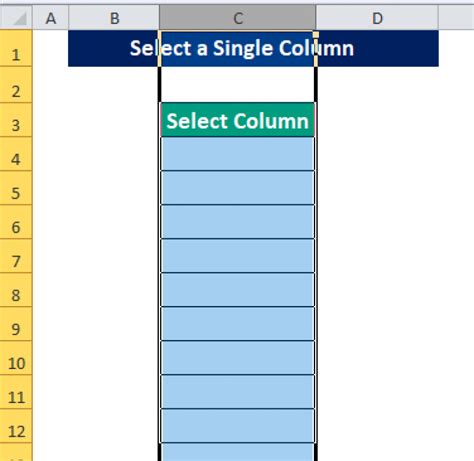
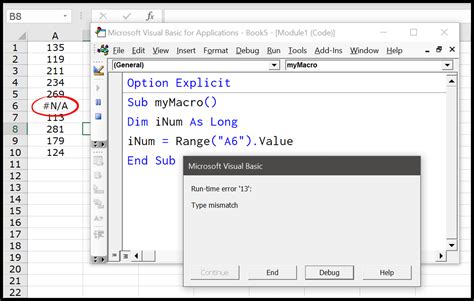
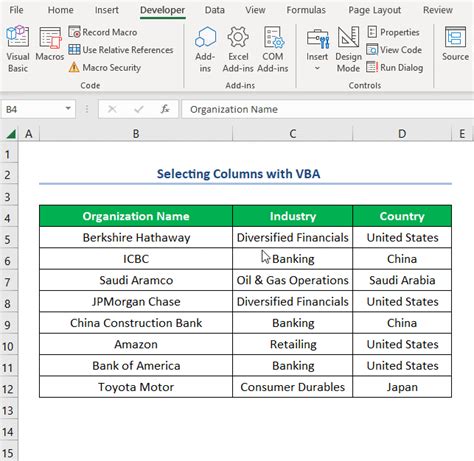
We hope this article has provided you with a comprehensive guide on selecting a whole column using VBA. If you have any further questions or need assistance with your VBA code, feel free to ask in the comments below!