Intro
Effortlessly convert VBA strings to integers with these 5 proven methods. Discover how to use the CInt, CLng, and Val functions, as well as error handling techniques, to ensure accurate data type conversions in your Visual Basic for Applications (VBA) code. Simplify your VBA programming with these expert-approved string to integer conversion solutions.
Converting a string to an integer in VBA (Visual Basic for Applications) is a common requirement when working with data that contains numeric values stored as text. This can happen for various reasons, such as when importing data from an external source or when dealing with user input. VBA offers several methods to achieve this conversion, each with its own set of advantages and potential pitfalls. Here, we will explore five ways to convert a VBA string to an integer, highlighting their uses and considerations.
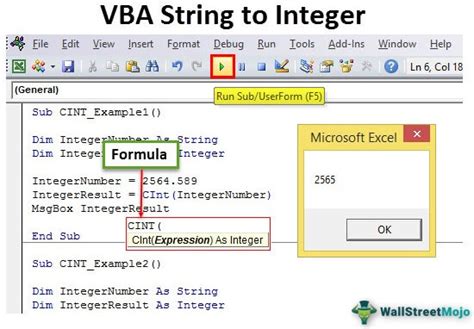
1. Using the CInt()
Function
The most straightforward method to convert a string to an integer in VBA is by using the CInt()
function. This function attempts to convert a specified value to an integer.
Dim myString As String
Dim myInteger As Integer
myString = "10"
myInteger = CInt(myString)
MsgBox "The integer value is: " & myInteger
Important Consideration: Using CInt()
can lead to runtime errors if the string cannot be converted to an integer. Always validate or handle potential errors.
2. Using the CLng()
Function and Then Converting
While CLng()
converts to a long integer, in many cases, you can use it as an intermediate step if CInt()
fails due to the value being too large for an integer but still within the long integer range.
Dim myString As String
Dim myLong As Long
Dim myInteger As Integer
myString = "32767"
myLong = CLng(myString)
myInteger = CInt(myLong)
MsgBox "The integer value is: " & myInteger
3. Using the Val()
Function
The Val()
function is another method to convert a string to a numeric value. It stops converting at the first character it cannot recognize as part of a number.
Dim myString As String
Dim myNumber As Double
Dim myInteger As Integer
myString = "123.45"
myNumber = Val(myString)
myInteger = CInt(myNumber)
MsgBox "The integer value is: " & myInteger
Important Note: Val()
returns a double, which then needs to be converted to an integer using CInt()
. Be cautious of rounding errors.
4. Using Application.Evaluate()
For more complex scenarios, or when working within Excel and needing to evaluate a string as if it were a formula, Application.Evaluate()
can be used.
Dim myString As String
Dim myValue As Variant
myString = "10*2"
myValue = Application.Evaluate(myString)
MsgBox "The value is: " & myValue
Caution: Be extremely cautious with Evaluate
due to its potential to evaluate any VBA expression, posing a significant security risk if used with untrusted input.
5. Using Regular Expressions to Validate and Extract Numbers
For strings containing text mixed with numbers, or when you need more control over what is extracted, regular expressions can be a powerful tool.
Dim myString As String
Dim regex As Object
Dim matches As Object
myString = "abc123def"
Set regex = CreateObject("VBScript.RegExp")
regex.Pattern = "\d+"
Set matches = regex.Execute(myString)
For Each match In matches
MsgBox "Found: " & match.Value
Next
Important: Regular expressions require the "Microsoft VBScript Regular Expressions" library to be enabled in the VBA Editor (Tools > References).
Gallery of Converting String to Integer
Converting String to Integer Gallery
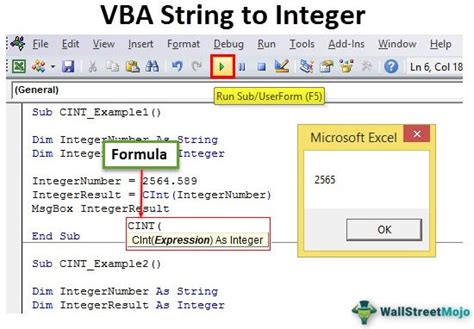
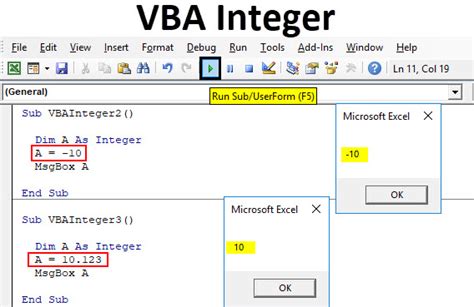
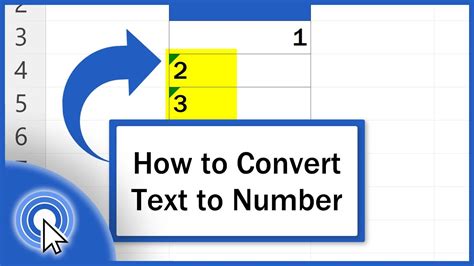
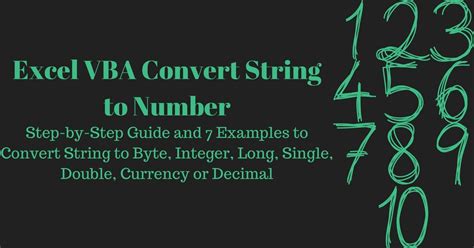
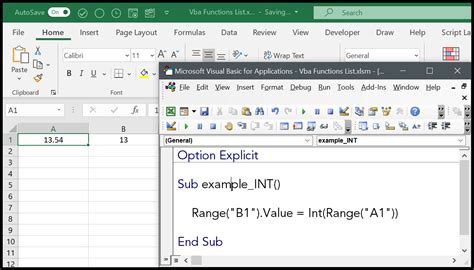
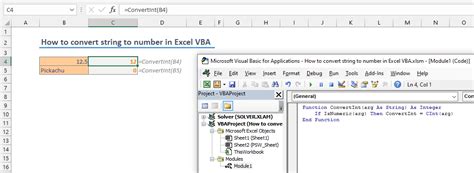
When dealing with string to integer conversions in VBA, it's crucial to validate the input to avoid errors, especially when using functions like CInt()
or CLng()
. Each method has its place depending on the complexity of the string and the requirements of the task at hand.