Intro
Discover how to efficiently test for null date parameters in VBA. Learn three effective methods to check if a date parameter is null, including using the IsNull function, comparing with an empty date, and utilizing the Nz function. Master VBA date parameter testing with these simple yet powerful techniques and improve your coding efficiency.
Understanding the Importance of Handling Null Date Parameters in VBA
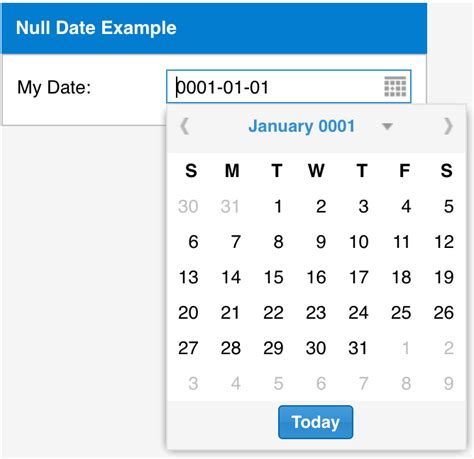
When working with dates in VBA, it's not uncommon to encounter null or missing values. Failing to properly handle these null values can lead to errors, crashes, and incorrect results. In this article, we'll explore three ways to test if a date parameter is null in VBA, ensuring your code is robust and reliable.
The Consequences of Not Handling Null Date Parameters
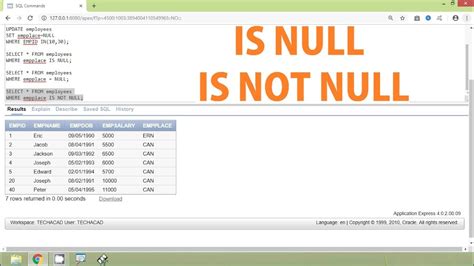
Ignoring null date parameters can have serious consequences, including:
- Errors and exceptions: Null values can cause errors when used in calculations or comparisons.
- Incorrect results: Null values can lead to incorrect results, especially when used in aggregate functions or summaries.
- Code crashes: Unhandled null values can cause your code to crash or become unresponsive.
Method 1: Using the IsNull Function
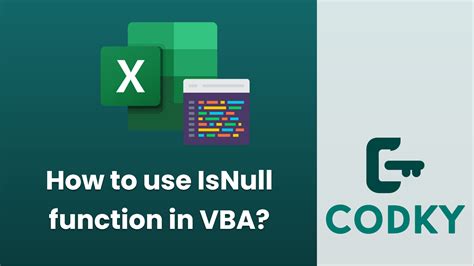
The IsNull function is a simple and straightforward way to test if a date parameter is null. Here's an example:
Sub TestIsNull()
Dim dt As Date
dt = Null
If IsNull(dt) Then
MsgBox "Date is null"
Else
MsgBox "Date is not null"
End If
End Sub
Advantages and Limitations
The IsNull function is easy to use and understand, but it has some limitations. For example, it only works with Variant data types, and it can be slower than other methods.
Method 2: Using the IsMissing Function
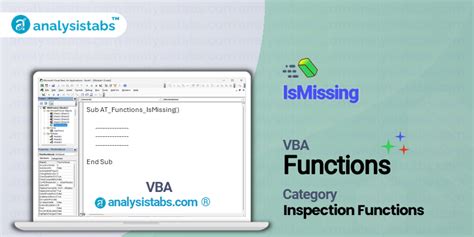
The IsMissing function is another way to test if a date parameter is null. Here's an example:
Sub TestIsMissing()
Dim dt As Date
dt = Null
If IsMissing(dt) Then
MsgBox "Date is null"
Else
MsgBox "Date is not null"
End If
End Sub
Advantages and Limitations
The IsMissing function is similar to IsNull, but it only works with parameters that have a default value of Empty. This can be a limitation in some cases.
Method 3: Using the VarType Function
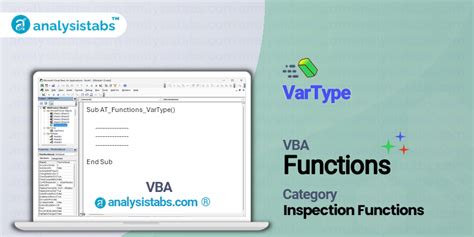
The VarType function is a more advanced way to test if a date parameter is null. Here's an example:
Sub TestVarType()
Dim dt As Date
dt = Null
If VarType(dt) = vbNull Then
MsgBox "Date is null"
Else
MsgBox "Date is not null"
End If
End Sub
Advantages and Limitations
The VarType function is more flexible than IsNull and IsMissing, as it can handle different data types. However, it requires a good understanding of the VarType function and its return values.
Conclusion
Testing if a date parameter is null is crucial in VBA programming. By using one of the three methods outlined above, you can ensure your code is robust and reliable. Remember to choose the method that best fits your needs and consider the advantages and limitations of each approach.
Which method do you prefer? Share your thoughts in the comments below!
VBA Date Parameter Testing Gallery
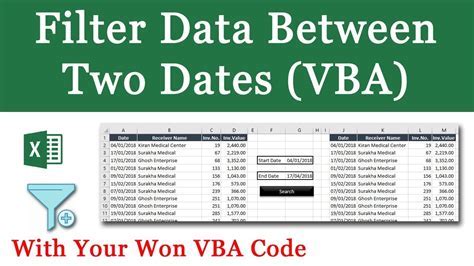
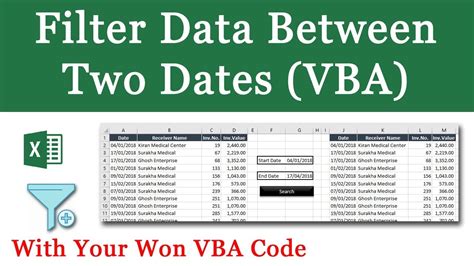
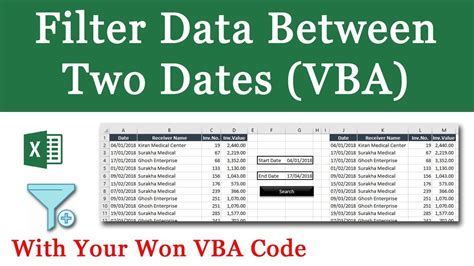
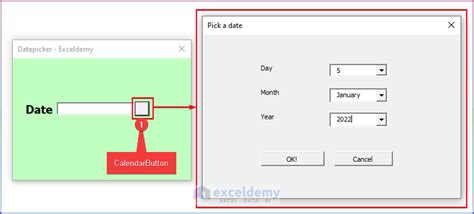
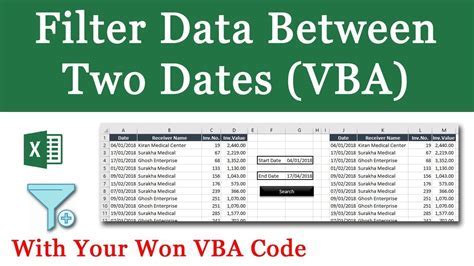
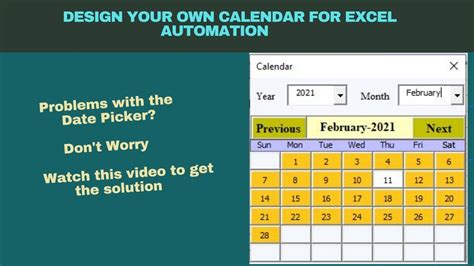
Note: The gallery images are just examples and not actual code.