Automating tasks in Excel can significantly enhance your productivity and efficiency. One common task that can be automated using VBA (Visual Basic for Applications) is hiding rows based on specific conditions. This can help declutter your worksheets and improve focus on relevant data. In this article, we will explore five ways to hide rows in Excel using VBA, covering different scenarios and approaches.
Understanding the Importance of Hiding Rows
Hiding rows in Excel can be beneficial in various situations. For instance, you might want to hide rows that contain errors, duplicates, or data that's not relevant to the current analysis. By automating this process with VBA, you can save time and reduce the risk of human error.
Method 1: Hiding Rows Based on a Specific Condition
Imagine you have a dataset where you want to hide all rows where the value in a specific column is "Yes". You can achieve this using the following VBA code:
Sub HideRowsBasedOnCondition()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("YourSheetName")
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
For i = lastRow To 1 Step -1
If ws.Cells(i, "A").Value = "Yes" Then
ws.Rows(i).Hidden = True
End If
Next i
End Sub
Replace "YourSheetName"
with the name of your sheet and adjust the column reference in ws.Cells(i, "A")
to match the column you want to check.
Method 2: Hiding Rows with Errors
To hide rows that contain errors, such as #N/A or #VALUE!, you can use the IsError
function in VBA. Here's how you can do it:
Sub HideRowsWithErrors()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("YourSheetName")
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
For i = lastRow To 1 Step -1
If IsError(ws.Cells(i, "A").Value) Then
ws.Rows(i).Hidden = True
End If
Next i
End Sub
This script hides rows where the value in column A is an error.
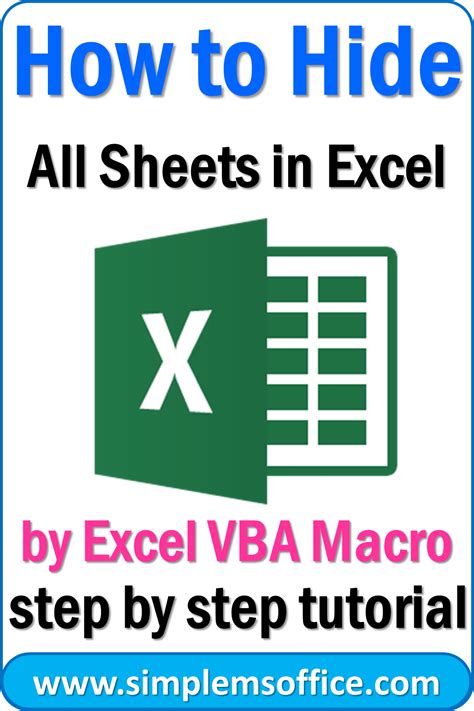
Method 3: Hiding Duplicate Rows
If you want to hide duplicate rows based on a specific column, you can use a combination of the RemoveDuplicates
method and error handling to hide rows instead of deleting them. However, for simplicity and adherence to the hiding rows requirement, you can use the following script to mark and then hide duplicates:
Sub HideDuplicateRows()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("YourSheetName")
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
ws.Cells(1, "B").Value = "Duplicate"
For i = lastRow To 2 Step -1
If Application.WorksheetFunction.CountIf(ws.Range(ws.Cells(1, "A"), ws.Cells(i, "A")), ws.Cells(i, "A").Value) > 1 Then
ws.Cells(i, "B").Value = "Duplicate"
End If
Next i
For i = lastRow To 1 Step -1
If ws.Cells(i, "B").Value = "Duplicate" Then
ws.Rows(i).Hidden = True
End If
Next i
End Sub
This script marks duplicate values in column A and then hides those rows.
Method 4: Hiding Rows Based on User Input
Sometimes, you might want to hide rows based on a value input by the user. You can use an InputBox
to get the value from the user and then hide rows accordingly.
Sub HideRowsBasedOnUserInput()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("YourSheetName")
Dim userInput As String
userInput = InputBox("Enter the value to hide rows for")
If userInput <> "" Then
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
For i = lastRow To 1 Step -1
If ws.Cells(i, "A").Value = userInput Then
ws.Rows(i).Hidden = True
End If
Next i
Else
MsgBox "No value entered", vbExclamation
End If
End Sub
This script prompts the user to enter a value and then hides rows in column A that match the input value.
Method 5: Hiding Rows Using AutoFilter
Finally, you can also use AutoFilter to hide rows. Although this method is more about filtering than directly hiding, it achieves a similar visual effect and is quite powerful for dynamic datasets.
Sub HideRowsUsingAutoFilter()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("YourSheetName")
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
ws.Range(ws.Cells(1, "A"), ws.Cells(lastRow, "A")).AutoFilter Field:=1, Criteria1:="<>Yes"
End Sub
This script applies an AutoFilter to column A to hide rows that do not contain "Yes".
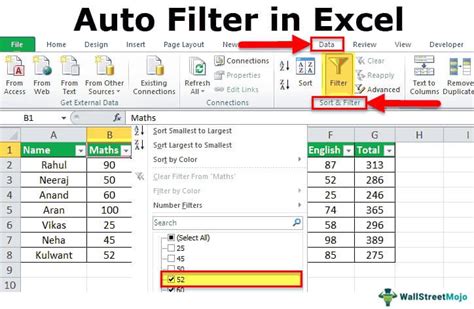
Gallery of Excel VBA Images
Excel VBA Image Gallery
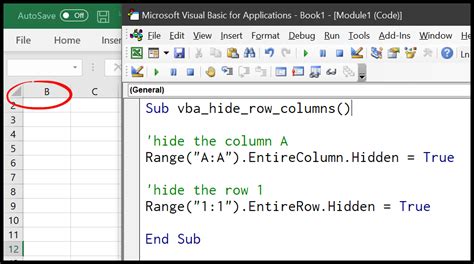
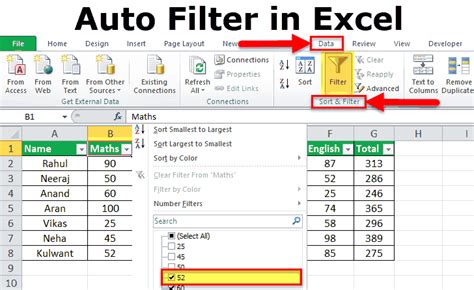
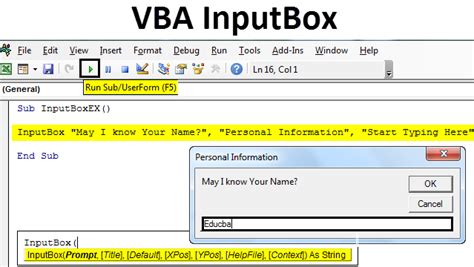
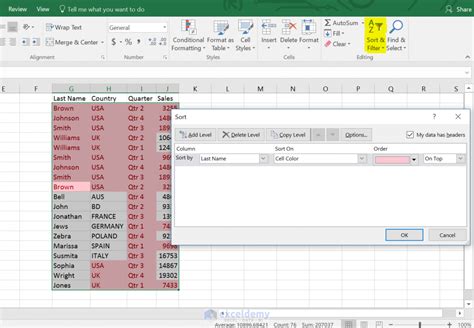
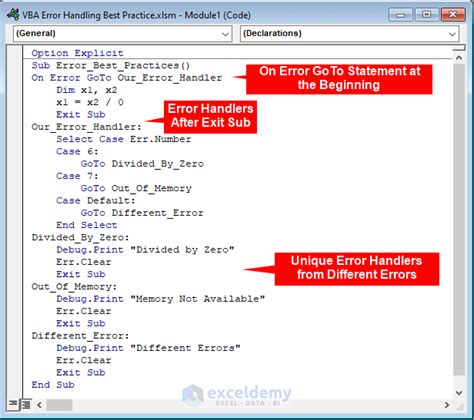
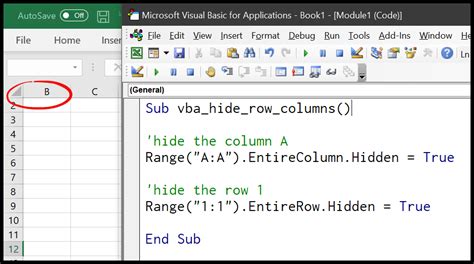
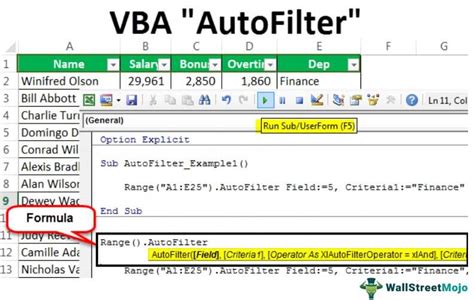
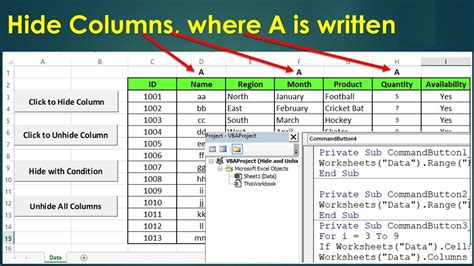
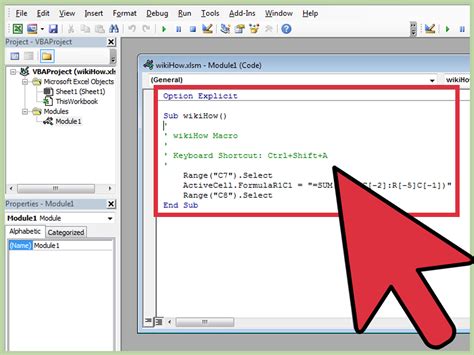
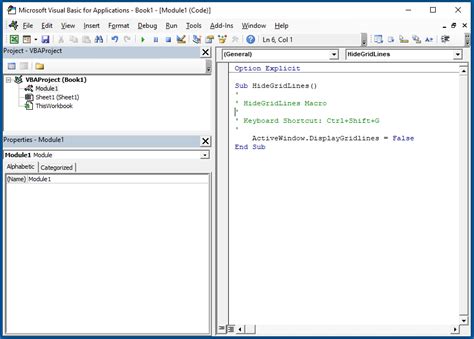
Final Thoughts
Hiding rows in Excel using VBA can greatly simplify your workflow and improve data visualization. By applying the methods outlined in this article, you can automate the process of hiding rows based on various conditions, user inputs, and AutoFilter criteria. Remember to adjust the code snippets to fit your specific needs and explore more advanced VBA techniques to further enhance your Excel skills.
If you have any questions or need further assistance with hiding rows or any other Excel VBA topics, feel free to ask in the comments below. Share this article with others who might benefit from learning how to hide rows in Excel with VBA, and consider subscribing for more Excel and VBA tutorials.