Intro
Pivot tables are a powerful tool in Excel, allowing users to summarize and analyze large datasets with ease. However, one of the most frustrating aspects of working with pivot tables is the need to constantly refresh them to reflect changes in the underlying data. Fortunately, VBA (Visual Basic for Applications) provides a solution to this problem, enabling users to refresh pivot tables automatically with just a few lines of code.
The Importance of Refreshing Pivot Tables
Before diving into the world of VBA, it's essential to understand why refreshing pivot tables is crucial. Pivot tables are dynamic, meaning they update automatically when the data changes. However, this dynamic behavior only works when the data is within the same worksheet or workbook. If the data is located in a different workbook or external data source, the pivot table will not update automatically. This is where VBA comes in, providing a way to programmatically refresh pivot tables and ensure they always reflect the latest data.
Understanding VBA
For those new to VBA, it's a programming language developed by Microsoft that allows users to create and automate tasks in Excel. VBA is accessed through the Visual Basic Editor, which can be opened by pressing Alt + F11 or navigating to Developer > Visual Basic in the ribbon. VBA code is written in modules, which are essentially containers for code.
Refreshing Pivot Tables with VBA
To refresh a pivot table using VBA, you'll need to use the PivotTable.RefreshTable
method. This method updates the pivot table with the latest data from the data source. Here's an example of how to use this method:
Sub RefreshPivotTable()
Dim pt As PivotTable
Set pt = ThisWorkbook.Worksheets("PivotTableWorksheet").PivotTables("PivotTableName")
pt.RefreshTable
End Sub
In this example, ThisWorkbook.Worksheets("PivotTableWorksheet")
refers to the worksheet containing the pivot table, and PivotTables("PivotTableName")
refers to the pivot table itself. The RefreshTable
method is then called on the pivot table object to refresh the data.
Automating Pivot Table Refresh
To automate the pivot table refresh process, you'll need to use a combination of VBA and Excel events. One way to do this is to use the Worksheet_Change
event, which fires whenever a change is made to the worksheet. Here's an example of how to use this event to refresh a pivot table:
Private Sub Worksheet_Change(ByVal Target As Range)
Dim pt As PivotTable
Set pt = ThisWorkbook.Worksheets("PivotTableWorksheet").PivotTables("PivotTableName")
pt.RefreshTable
End Sub
In this example, the Worksheet_Change
event is triggered whenever a change is made to the worksheet. The code then refreshes the pivot table using the RefreshTable
method.
Scheduling Pivot Table Refresh
Another way to automate pivot table refresh is to schedule it using VBA. This can be done using the Application.OnTime
method, which schedules a procedure to run at a specific time or interval. Here's an example of how to use this method to refresh a pivot table every hour:
Sub SchedulePivotTableRefresh()
Dim pt As PivotTable
Set pt = ThisWorkbook.Worksheets("PivotTableWorksheet").PivotTables("PivotTableName")
Application.OnTime TimeValue("14:00:00"), "RefreshPivotTable" ' 2:00 PM
End Sub
In this example, the Application.OnTime
method is used to schedule the RefreshPivotTable
procedure to run at 2:00 PM every day.
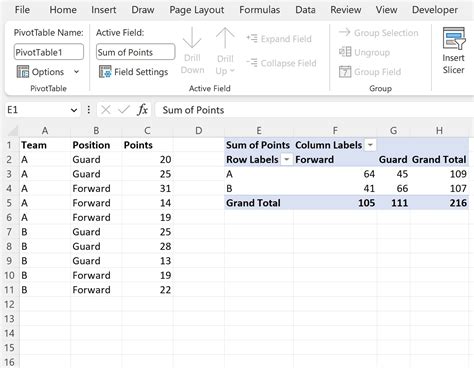
Tips and Variations
- To refresh multiple pivot tables, simply loop through the
PivotTables
collection and call theRefreshTable
method on each pivot table. - To refresh pivot tables in multiple workbooks, use the
Workbooks
collection and loop through each workbook. - To schedule a pivot table refresh using a specific interval (e.g., every 10 minutes), use the
Application.OnTime
method with a time interval (e.g.,TimeValue("00:10:00")
).
Gallery of Pivot Table Refresh Examples
Pivot Table Refresh VBA Examples
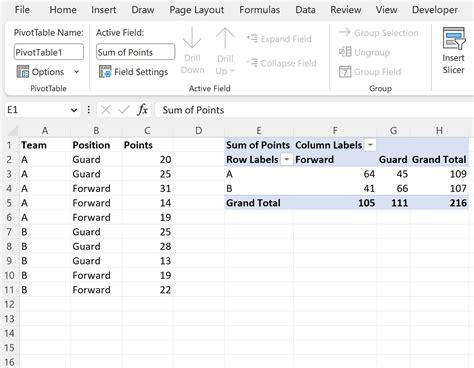
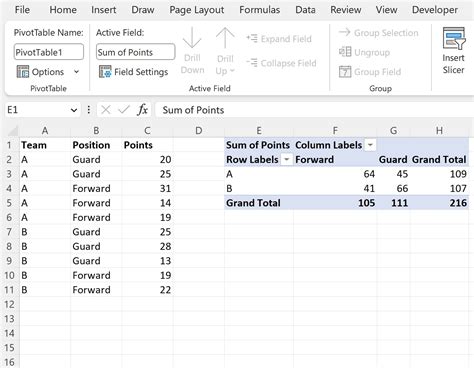
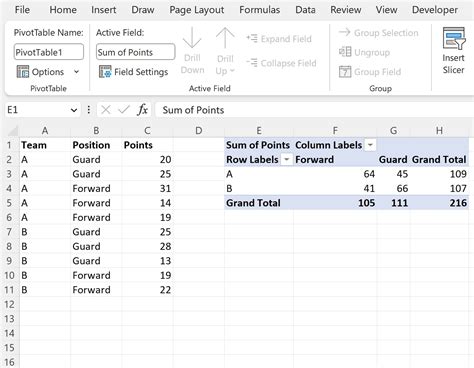
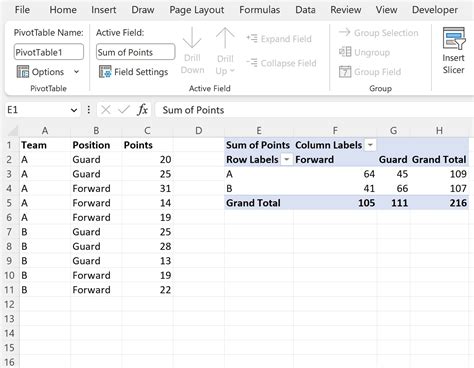
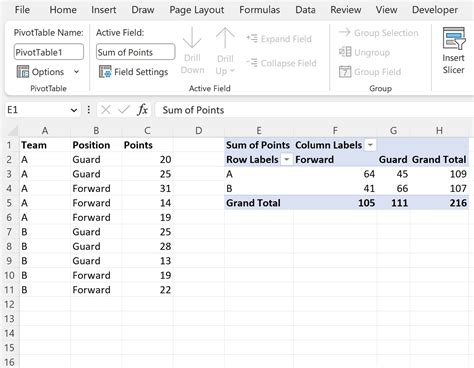
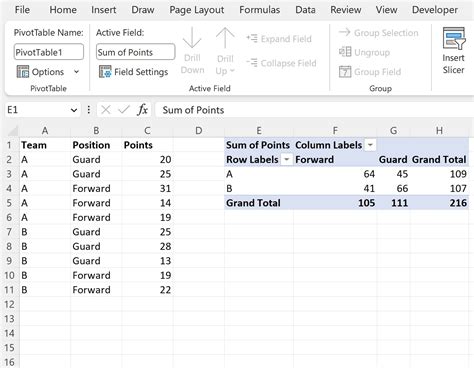
By using VBA to refresh pivot tables, you can automate the process of updating your pivot tables with the latest data, saving you time and effort. Whether you're working with a single pivot table or multiple tables across multiple workbooks, VBA provides the tools you need to streamline your workflow and ensure your data is always up-to-date.
Take Action!
Take the first step towards automating your pivot table refresh process by trying out the code examples in this article. Share your experiences and tips in the comments below!