Sending emails with VBA (Visual Basic for Applications) is a powerful tool that can automate tasks and streamline workflows in Microsoft Office applications, particularly in Excel. This guide will walk you through the process of sending emails using VBA, covering the basics, advanced techniques, and providing practical examples.
Setting Up Your Environment
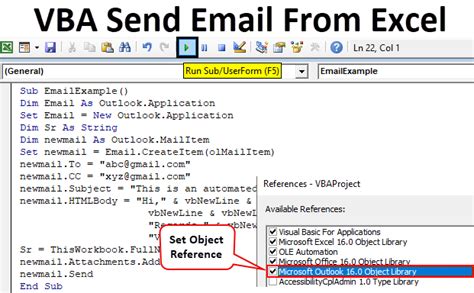
Before you can start sending emails with VBA, you need to set up your environment. This involves enabling the Microsoft Outlook Object Library in your VBA editor and creating a new module.
- Open the Visual Basic Editor in Excel by pressing
Alt + F11
or navigating toDeveloper
>Visual Basic
. - In the VBA editor, click
Tools
>References
and check ifMicrosoft Outlook Object Library
is selected. If not, select it and clickOK
. - Create a new module by clicking
Insert
>Module
or pressAlt + F11
again.
Understanding the Outlook Object Model
The Outlook Object Model is a set of objects, properties, and methods that allow you to interact with Outlook from VBA. Understanding this model is crucial to sending emails with VBA.
- The
Application
object represents the Outlook application. - The
Namespace
object represents the Outlook session. - The
MailItem
object represents a single email message.
Sending a Simple Email with VBA
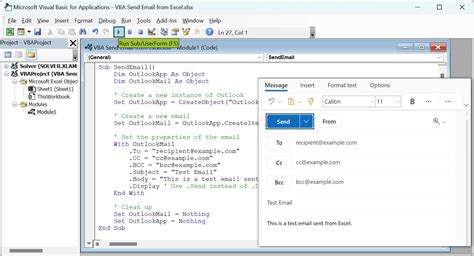
Now that you have set up your environment and understand the Outlook Object Model, let's create a simple VBA script to send an email.
- In the new module, paste the following code:
Sub SendSimpleEmail()
Dim olApp As Object
Dim olMail As Object
Set olApp = CreateObject("Outlook.Application")
Set olMail = olApp.CreateItem(0)
With olMail
.To = "recipient@example.com"
.Subject = "Simple Email from VBA"
.Body = "This is a simple email sent from VBA."
.Send
End With
Set olMail = Nothing
Set olApp = Nothing
End Sub
- Press
F5
to run the script.
This script creates a new email message, sets the recipient, subject, and body, and sends the email.
Adding Attachments and Formatting
You can add attachments and formatting to your email by using the Attachments
property and the HTMLBody
property.
- To add an attachment, use the
Attachments.Add
method:
.Attachments.Add "C:\Path\To\Attachment.xlsx"
- To format the email body as HTML, use the
HTMLBody
property:
.HTMLBody = "This is a formatted email
This is a paragraph.
"
Advanced Email Techniques
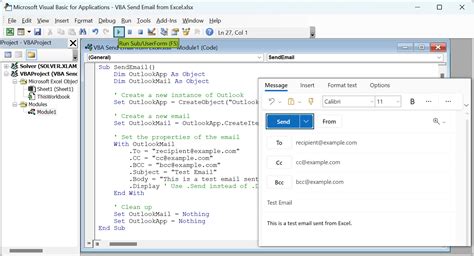
Once you have mastered the basics, you can move on to advanced email techniques, such as sending emails to multiple recipients, using templates, and scheduling emails.
- To send an email to multiple recipients, use the
To
property with a semicolon-separated list of email addresses:
.To = "recipient1@example.com; recipient2@example.com"
- To use a template, use the
OpenSharedItem
method:
Dim olTemplate As Object
Set olTemplate = olApp.CreateItemFromTemplate("C:\Path\To\Template.oft")
- To schedule an email, use the
SendOnBehalfOfName
property and theSendUsingAccount
property:
.SendOnBehalfOfName = " sender@example.com"
.SendUsingAccount = olApp.Session.Accounts.Item(" sender@example.com")
Error Handling and Debugging
Error handling and debugging are essential when working with VBA. You can use the On Error
statement to handle errors and the Debug.Print
statement to debug your code.
- To handle errors, use the
On Error
statement:
On Error GoTo ErrorHandler
- To debug your code, use the
Debug.Print
statement:
Debug.Print "Email sent successfully"
Conclusion
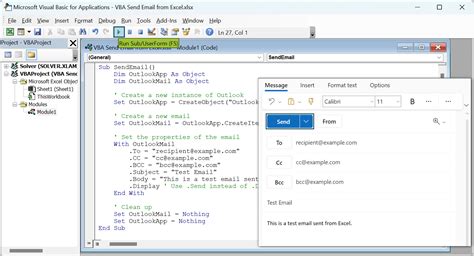
Sending emails with VBA is a powerful tool that can automate tasks and streamline workflows. By mastering the basics and advanced techniques, you can take your email automation to the next level.
Gallery of VBA Email Examples
VBA Email Examples
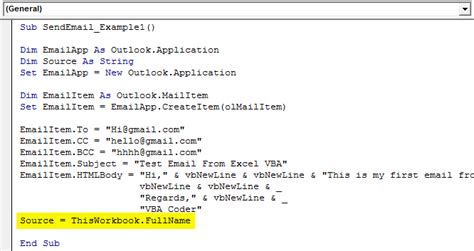
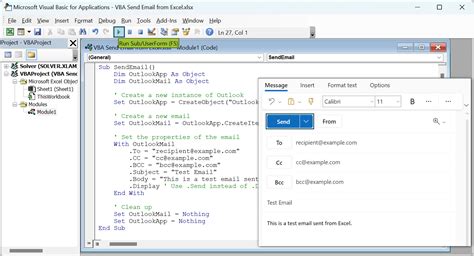
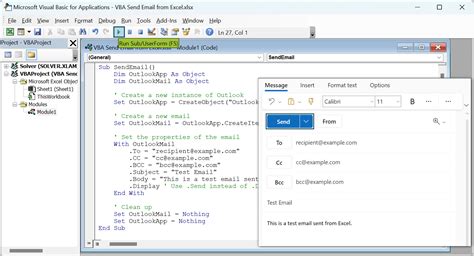
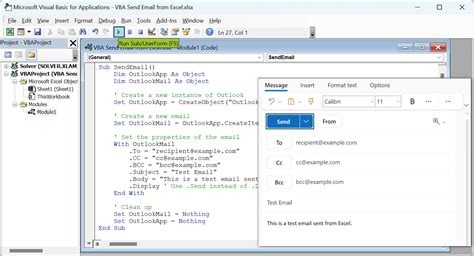
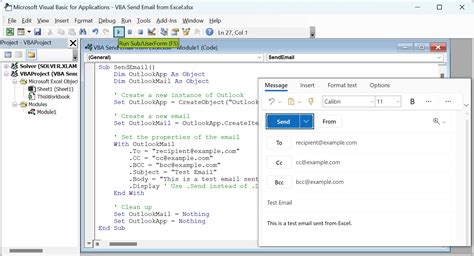
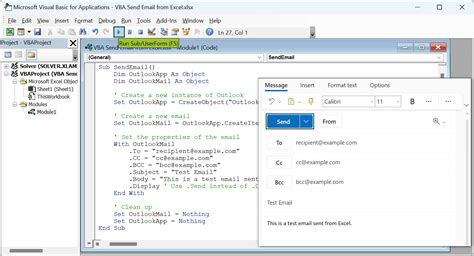
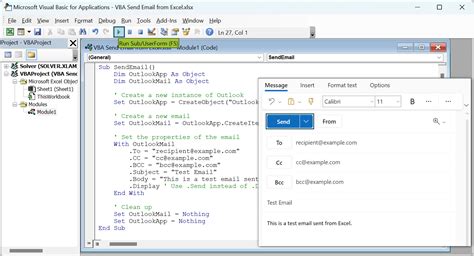
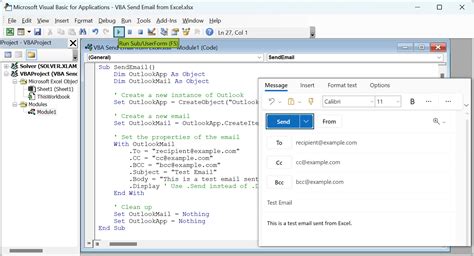
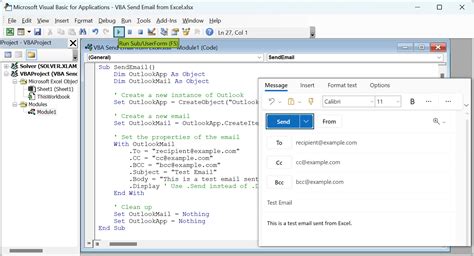
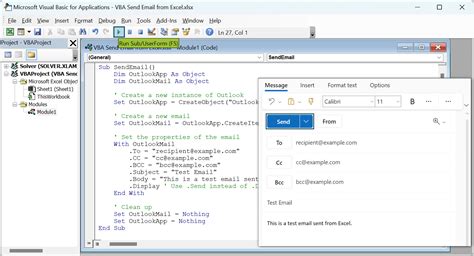
We hope this comprehensive guide has helped you master the art of sending emails with VBA. Share your thoughts, questions, or feedback in the comments below!