Intro
Unlock the power of VBA with our expert guide on writing to text files made easy. Learn how to efficiently create, write, and append text files using VBA code, including practical examples and troubleshooting tips. Master VBA text file manipulation and streamline your workflows with our comprehensive tutorial.
Writing to a text file using VBA (Visual Basic for Applications) is a fundamental task that can be accomplished with ease. In this article, we will delve into the world of VBA and explore the various methods of writing to a text file, making it a straightforward process for you.
Why Write to a Text File?
Before we dive into the nitty-gritty of writing to a text file, let's briefly discuss why this is a useful skill to have. Text files are an excellent way to store and share data, especially when working with automation tasks or data analysis. By writing data to a text file, you can easily export data from Excel, Word, or other Office applications, and then import it into other programs or systems.
Methods for Writing to a Text File
There are several methods for writing to a text file using VBA. We will explore the most common methods, including the Open
statement, the Write
statement, and the Print
statement.
Method 1: Using the Open
Statement
The Open
statement is a simple way to open a text file and write data to it. Here is an example:
Sub WriteToFile()
Dim filePath As String
filePath = "C:\Example\TextFile.txt"
Open filePath For Output As #1
Write #1, "Hello, World!"
Close #1
End Sub
In this example, we open a text file called "TextFile.txt" located in the "C:\Example" directory. We then use the Write
statement to write the string "Hello, World!" to the file.
Image:
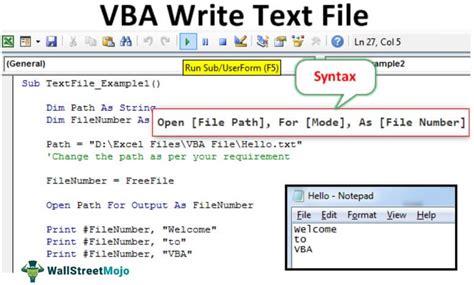
Method 2: Using the Write
Statement
The Write
statement is similar to the Open
statement, but it allows you to write data to a text file without explicitly opening the file. Here is an example:
Sub WriteToFile()
Dim filePath As String
filePath = "C:\Example\TextFile.txt"
Write #1, filePath, "Hello, World!"
Close #1
End Sub
In this example, we use the Write
statement to write data to the text file, and then close the file using the Close
statement.
Image:
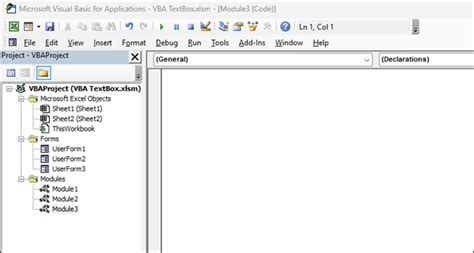
Method 3: Using the Print
Statement
The Print
statement is another way to write data to a text file. Here is an example:
Sub WriteToFile()
Dim filePath As String
filePath = "C:\Example\TextFile.txt"
Print #1, filePath, "Hello, World!"
Close #1
End Sub
In this example, we use the Print
statement to write data to the text file, and then close the file using the Close
statement.
Image:
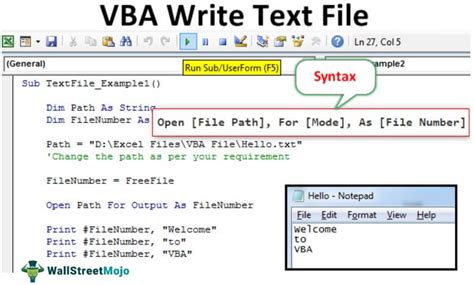
Tips and Tricks
- Always close the text file after writing data to it to avoid errors and data loss.
- Use the
FreeFile
function to get the next available file number when using theOpen
statement. - Use the
FileExists
function to check if a file exists before writing to it.
Gallery of VBA Write to Text File Examples
VBA Write to Text File Gallery
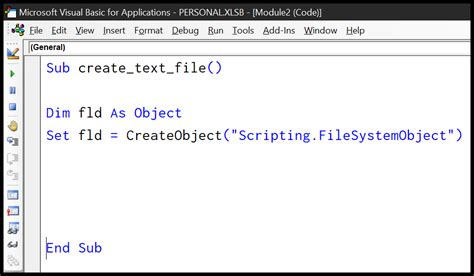
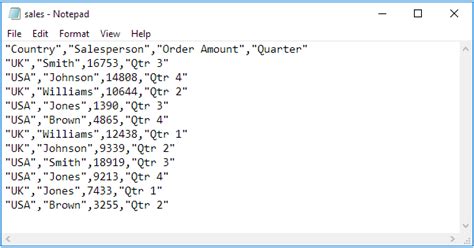
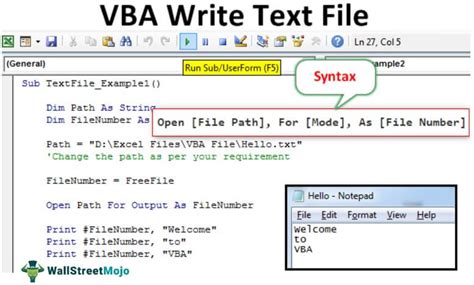
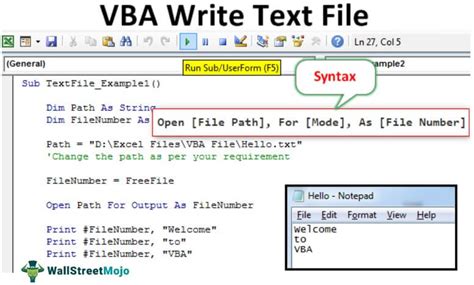
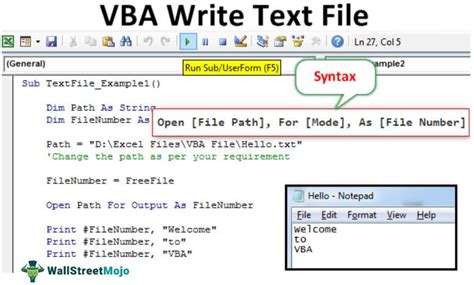
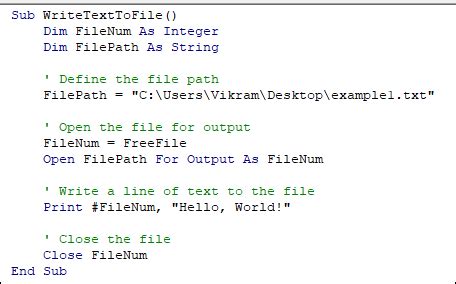
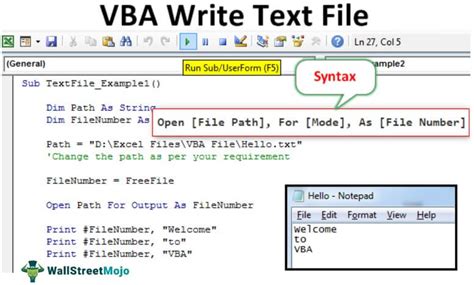
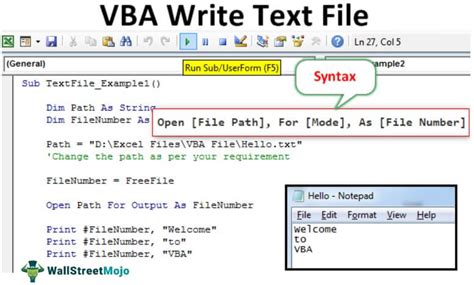
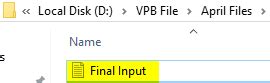
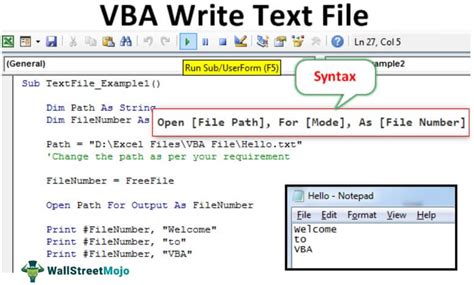
Get Started with VBA Write to Text File
Writing to a text file using VBA is a simple yet powerful skill that can be used in a variety of applications. By following the methods outlined in this article, you can easily write data to a text file and take your automation tasks to the next level.
Share your experiences and tips for writing to text files using VBA in the comments section below. Don't forget to share this article with your friends and colleagues who may find it useful.
Stay tuned for more VBA tutorials and tips!