Intro
Master the art of dynamically adding sheets in VBA Excel with ease. Learn how to automate worksheet creation, manipulate sheet properties, and streamline your workflow with efficient VBA code. Discover tips and tricks for adding sheets, renaming, and deleting them programmatically, using VBAs powerful worksheet object model.
In Microsoft Excel, managing worksheets can be a daunting task, especially when dealing with multiple sheets and large datasets. Fortunately, VBA (Visual Basic for Applications) provides a simple and efficient way to add sheets dynamically, making it easier to organize and maintain your Excel workbooks. In this article, we'll explore the various ways to add sheets dynamically in VBA Excel, providing you with step-by-step instructions and examples to get you started.
Why Add Sheets Dynamically?
Before we dive into the nitty-gritty of adding sheets dynamically, let's consider why this feature is so useful. Dynamic sheet addition can help:
- Streamline your worksheet management by creating sheets as needed
- Automate repetitive tasks, such as creating reports or summaries
- Enhance collaboration by allowing multiple users to add sheets without manual intervention
- Improve data organization by creating separate sheets for different datasets or scenarios
Method 1: Adding Sheets Using the Worksheets.Add
Method
One of the simplest ways to add sheets dynamically in VBA Excel is by using the Worksheets.Add
method. This method allows you to add a new worksheet to the active workbook.
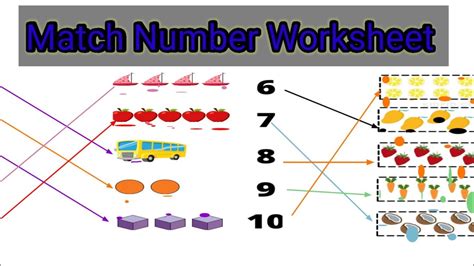
Here's an example code snippet that demonstrates how to use the Worksheets.Add
method:
Sub AddSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets.Add
ws.Name = "NewSheet"
End Sub
In this example, we create a new subroutine called AddSheet
and set a variable ws
to the new worksheet added using the Worksheets.Add
method. We then name the new worksheet "NewSheet".
Method 2: Adding Sheets Using the Worksheets.Insert
Method
Another way to add sheets dynamically is by using the Worksheets.Insert
method. This method allows you to insert a new worksheet at a specific position within the workbook.
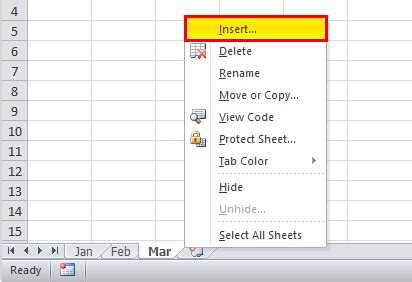
Here's an example code snippet that demonstrates how to use the Worksheets.Insert
method:
Sub InsertSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets.Insert(ThisWorkbook.Worksheets.Count + 1)
ws.Name = "NewSheet"
End Sub
In this example, we create a new subroutine called InsertSheet
and set a variable ws
to the new worksheet inserted using the Worksheets.Insert
method. We then name the new worksheet "NewSheet".
Method 3: Adding Sheets Using the Worksheets.Copy
Method
You can also add sheets dynamically by copying an existing worksheet. This method is useful when you want to create a new worksheet with the same layout and formatting as an existing sheet.
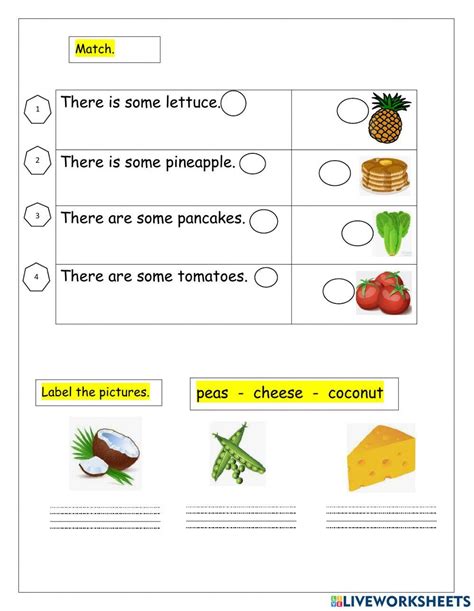
Here's an example code snippet that demonstrates how to use the Worksheets.Copy
method:
Sub CopySheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("ExistingSheet")
ws.Copy After:=ThisWorkbook.Worksheets(ThisWorkbook.Worksheets.Count)
ThisWorkbook.Worksheets(ThisWorkbook.Worksheets.Count).Name = "NewSheet"
End Sub
In this example, we create a new subroutine called CopySheet
and set a variable ws
to an existing worksheet named "ExistingSheet". We then copy the existing worksheet and name the new worksheet "NewSheet".
Method 4: Adding Sheets Using the Worksheets.AddWithDialog
Method
If you want to prompt the user to select a template or provide a name for the new worksheet, you can use the Worksheets.AddWithDialog
method.
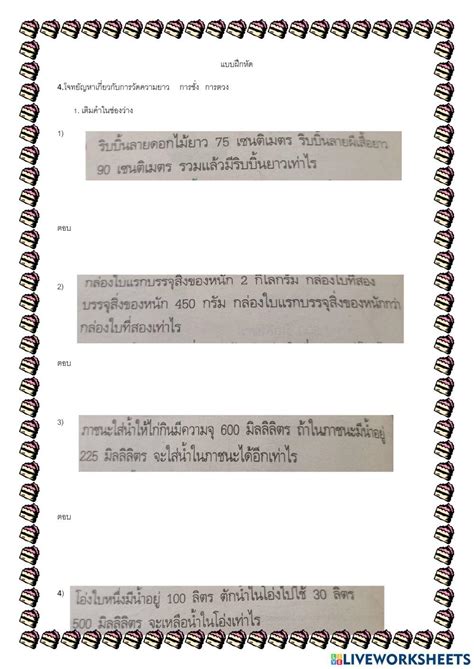
Here's an example code snippet that demonstrates how to use the Worksheets.AddWithDialog
method:
Sub AddSheetWithDialog()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets.AddWithDialog
ws.Name = "NewSheet"
End Sub
In this example, we create a new subroutine called AddSheetWithDialog
and set a variable ws
to the new worksheet added using the Worksheets.AddWithDialog
method. We then name the new worksheet "NewSheet".
Conclusion
Adding sheets dynamically in VBA Excel is a powerful feature that can streamline your worksheet management and automate repetitive tasks. By using the Worksheets.Add
, Worksheets.Insert
, Worksheets.Copy
, and Worksheets.AddWithDialog
methods, you can create new worksheets with ease and efficiency.
Whether you're a beginner or an advanced VBA user, this article has provided you with the necessary knowledge to add sheets dynamically in VBA Excel. With practice and experimentation, you'll become proficient in using these methods to manage your worksheets and create powerful Excel applications.
Gallery of VBA Excel Worksheet Management
VBA Excel Worksheet Management Gallery
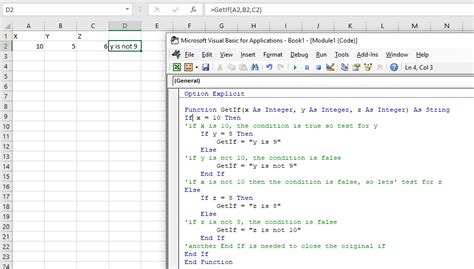
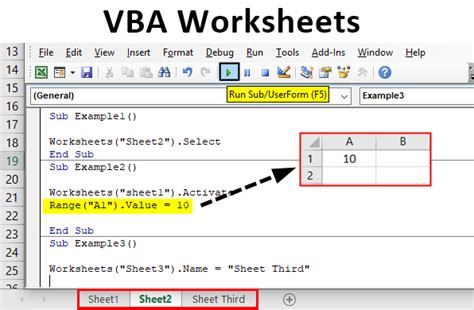
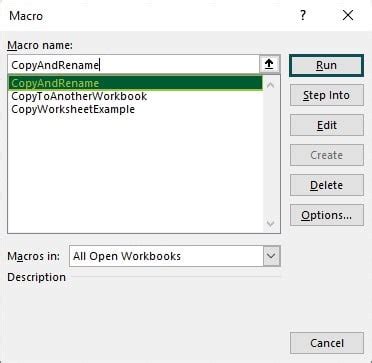
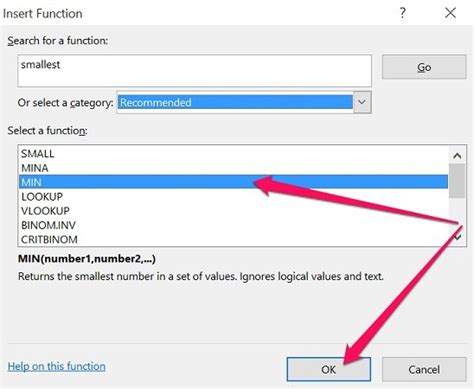
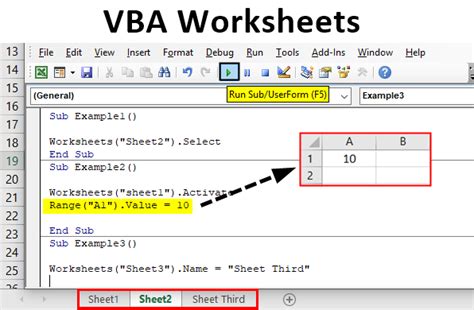
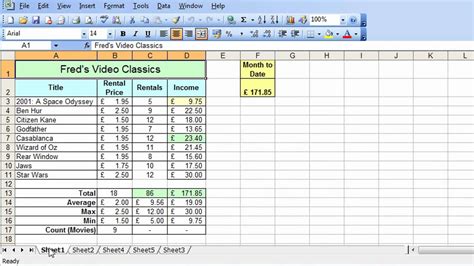