Intro
Unlock the power of C++ template member functions with our expert guide. Mastering these techniques is crucial for efficient and reusable code. Discover five actionable ways to improve your skills, including template metaprogramming, SFINAE, and template specialization. Take your C++ programming to the next level with these advanced template member function techniques.
Mastering C++ template member functions is a crucial aspect of becoming proficient in the C++ programming language. Template member functions are a powerful tool in C++ that allows for generic programming, enabling developers to write reusable and flexible code. In this article, we will explore five ways to master C++ template member functions, including understanding the basics, using template metaprogramming, avoiding common pitfalls, leveraging type traits, and utilizing std::enable_if.
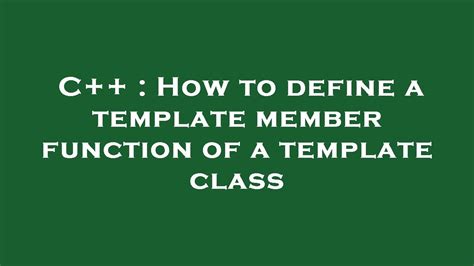
Understanding the Basics of Template Member Functions
Template member functions are a type of member function that belongs to a class template. They are defined inside a class template and can be instantiated with different types. To understand the basics of template member functions, it's essential to know how to declare and define them.
Declaring Template Member Functions
A template member function is declared using the template
keyword followed by a template parameter list. The template parameter list is a comma-separated list of template parameters, which are placeholders for types or values.
template
class MyClass {
public:
template
void myFunction(U param);
};
Defining Template Member Functions
A template member function is defined outside the class template definition. The definition of a template member function is similar to a regular member function, except that it uses the template
keyword and template parameter list.
template
template
void MyClass::myFunction(U param) {
// function body
}
Using Template Metaprogramming
Template metaprogramming is a technique that allows developers to perform computations at compile-time using templates. Template metaprogramming can be used to create complex data structures, perform type manipulation, and optimize code.
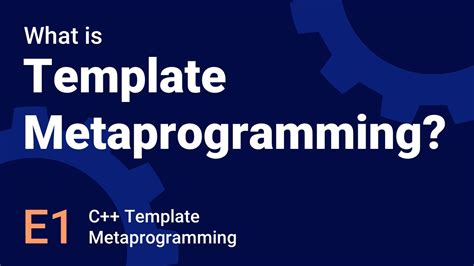
One common use of template metaprogramming is to create a recursive template that calculates the factorial of a number at compile-time.
template
struct Factorial {
enum { value = N * Factorial::value };
};
template <>
struct Factorial<0> {
enum { value = 1 };
};
int main() {
int result = Factorial<5>::value;
return 0;
}
Avoiding Common Pitfalls
There are several common pitfalls to avoid when using template member functions. One common pitfall is to forget to use the template
keyword when defining a template member function outside the class template definition.
Another common pitfall is to use the wrong syntax when calling a template member function. The correct syntax is to use the template
keyword followed by the template parameter list.
MyClass obj;
obj.myFunction(10.5); // incorrect syntax
obj.template myFunction(10.5); // correct syntax
Leveraging Type Traits
Type traits are a set of classes and functions that provide information about types at compile-time. Type traits can be used to manipulate types, check type properties, and optimize code.
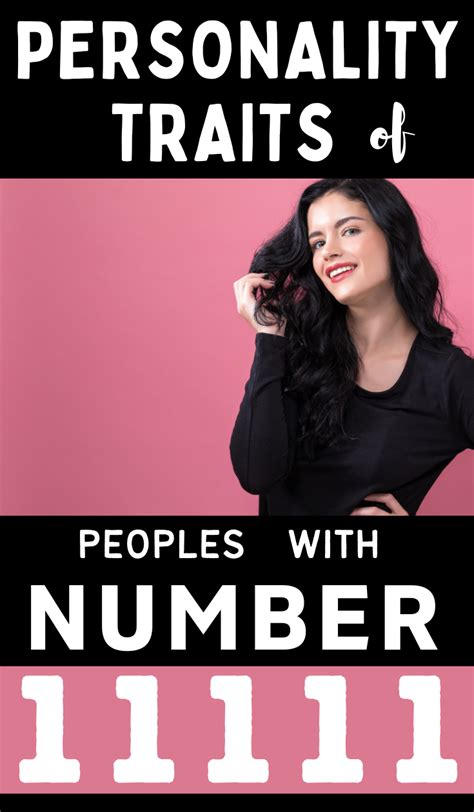
One common use of type traits is to check if a type is a pointer or not.
#include
template
void myFunction(T param) {
if (std::is_pointer::value) {
// param is a pointer
} else {
// param is not a pointer
}
}
Utilizing std::enable_if
std::enable_if is a type trait that allows developers to selectively enable or disable functions based on certain conditions. std::enable_if can be used to optimize code, reduce compilation time, and improve code readability.
#include
template
typename std::enable_if::value, void>::type
myFunction(T param) {
// param is a pointer
}
template
typename std::enable_if::value, void>::type
myFunction(T param) {
// param is not a pointer
}
Template Member Functions Image Gallery
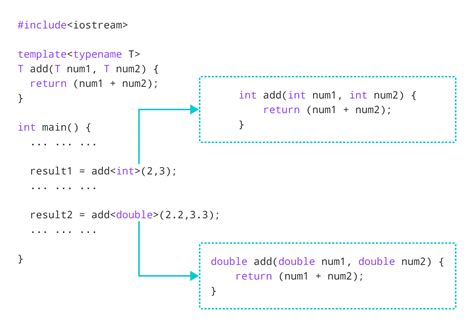
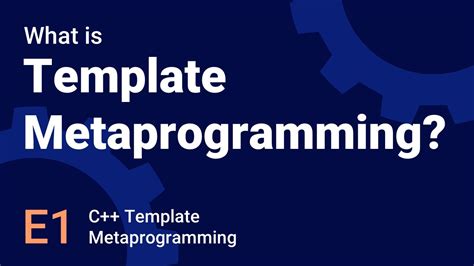
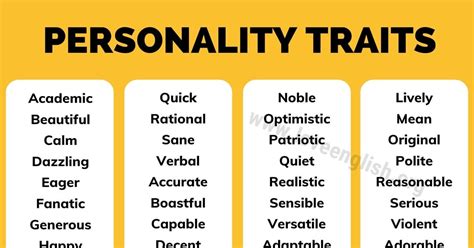
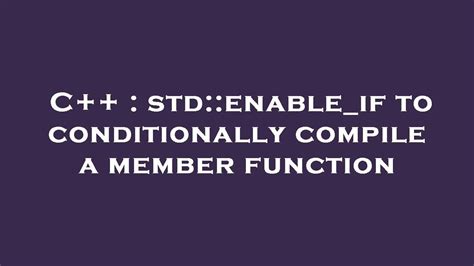
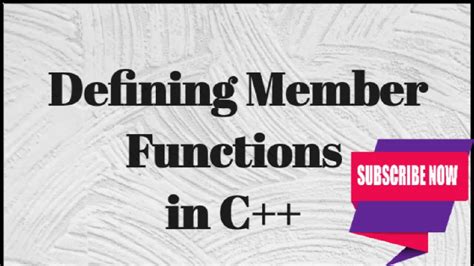
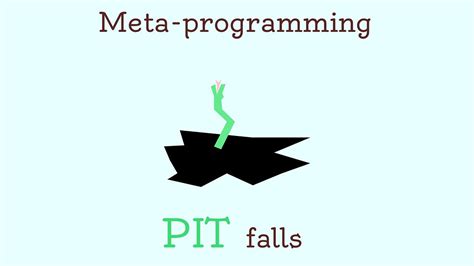
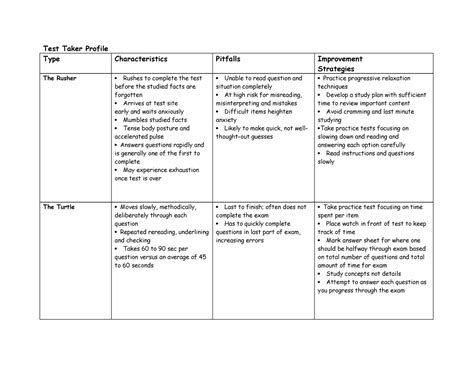
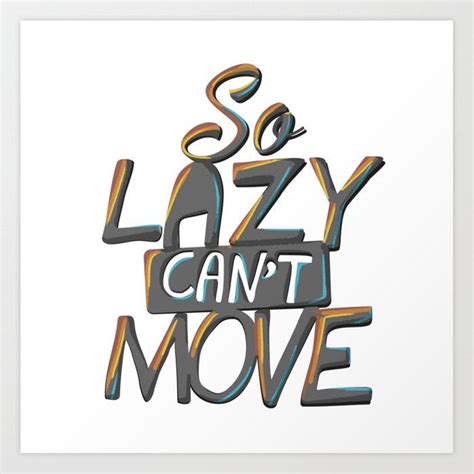
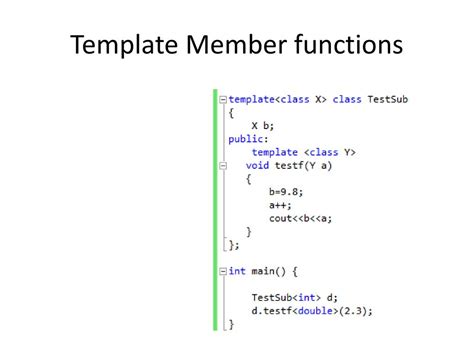
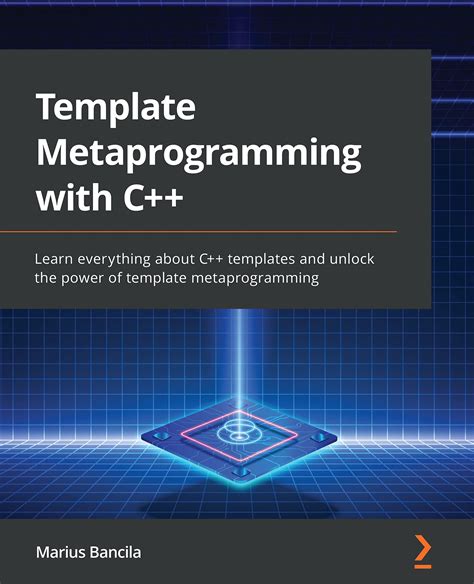
In conclusion, mastering C++ template member functions requires a deep understanding of the basics, template metaprogramming, type traits, and std::enable_if. By avoiding common pitfalls and leveraging these techniques, developers can write more efficient, flexible, and maintainable code. We encourage you to share your experiences and tips on using template member functions in the comments below.